Intro
Learn to create a new worksheet in Excel using VBA, with step-by-step guides on worksheet creation, VBA macros, and Excel automation techniques.
Creating a new worksheet in Excel using VBA (Visual Basic for Applications) can be a straightforward process, especially when you understand the basics of VBA programming. This process involves opening the Visual Basic Editor, writing a simple script, and then executing that script to add a new worksheet to your workbook. Below is a step-by-step guide on how to achieve this, including explanations and examples to help you understand the process better.
First, let's understand why you might want to create a new worksheet using VBA. This could be part of a larger automation task, where you need to generate reports, track data, or perform analysis on a regular basis. By automating the creation of new worksheets, you can save time and reduce the chance of human error.
Step 1: Access the Visual Basic Editor
To start working with VBA, you need to open the Visual Basic Editor. You can do this by pressing Alt
+ F11
while in Excel, or by navigating to the Developer
tab and clicking on Visual Basic
. If you don't see the Developer
tab, you might need to activate it through Excel's settings.
Step 2: Insert a New Module
Once the Visual Basic Editor is open, you'll need to insert a new module to write your VBA code. To do this:
- In the Visual Basic Editor, right-click on any of the objects for your workbook listed in the "Project" window on the left side.
- Choose
Insert
>Module
from the context menu. This action creates a new module.
Step 3: Write the VBA Code
Now, you can write the VBA code to create a new worksheet. Here's a simple example:
Sub CreateNewWorksheet()
' Declare a variable for the new worksheet
Dim newWorksheet As Worksheet
' Create a new worksheet
Set newWorksheet = ThisWorkbook.Worksheets.Add
' Name the new worksheet
newWorksheet.Name = "MyNewSheet"
' Optionally, you can move the new worksheet to a specific position
' newWorksheet.Move Before:=ThisWorkbook.Worksheets("Sheet1")
End Sub
This code defines a subroutine called CreateNewWorksheet
that adds a new worksheet to the active workbook and names it "MyNewSheet". You can adjust the name as needed.
Step 4: Run the VBA Code
To execute the code and create the new worksheet:
- Press
F5
while in the Visual Basic Editor with the module containing your code active. - Alternatively, you can run the code by clicking
Run
>Run Sub/UserForm
and selectingCreateNewWorksheet
from the list.
Step 5: Understanding the Code
Let's break down what the code does:
Sub CreateNewWorksheet()
: This line begins the definition of a subroutine namedCreateNewWorksheet
.Dim newWorksheet As Worksheet
: This line declares a variable namednewWorksheet
of typeWorksheet
, which will be used to refer to the new worksheet.Set newWorksheet = ThisWorkbook.Worksheets.Add
: This line creates a new worksheet in the current workbook and assigns it to thenewWorksheet
variable.newWorksheet.Name = "MyNewSheet"
: This line names the new worksheet "MyNewSheet". You can change"MyNewSheet"
to any valid worksheet name you prefer.newWorksheet.Move Before:=ThisWorkbook.Worksheets("Sheet1")
: This line is commented out but shows how you can move the new worksheet to a specific position in the workbook, in this case, before a worksheet named "Sheet1".
Additional Tips
- Error Handling: When working with VBA, especially in a production environment, consider adding error handling to your code. This can help manage unexpected issues, such as a worksheet with the desired name already existing.
- Worksheet Existence Check: Before creating a new worksheet, you might want to check if a worksheet with the same name already exists to avoid errors or overwriting data unintentionally.
- Customization: The code provided is basic. Depending on your needs, you might want to add more functionality, such as formatting the new worksheet, adding data, or setting up specific page layouts.
Embedding Images
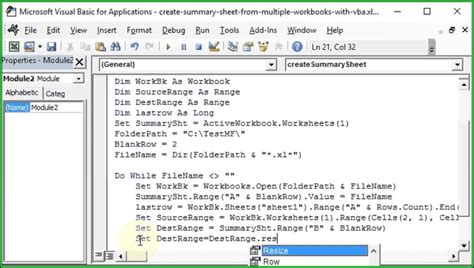
Gallery of VBA Worksheets
VBA Worksheet Gallery
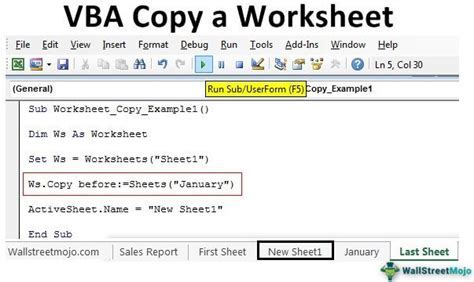
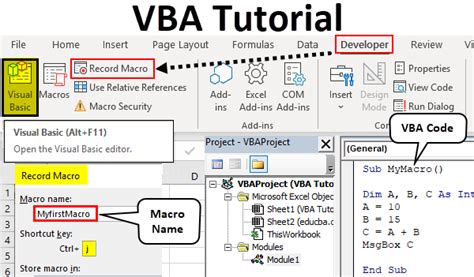
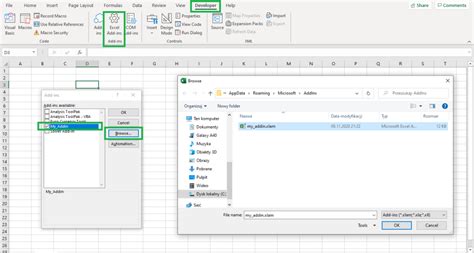
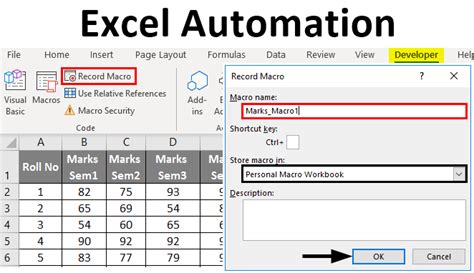
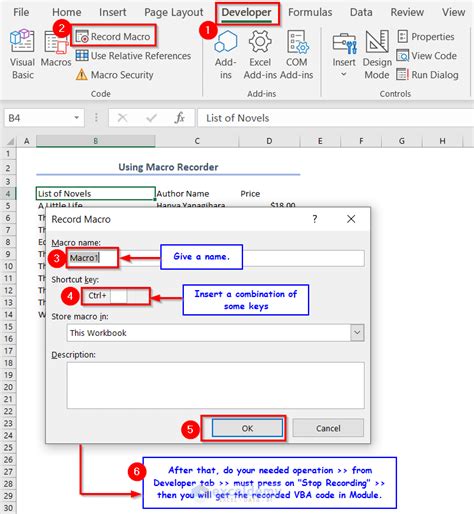
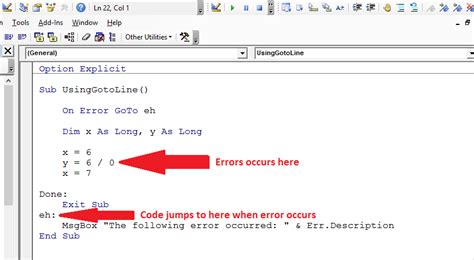
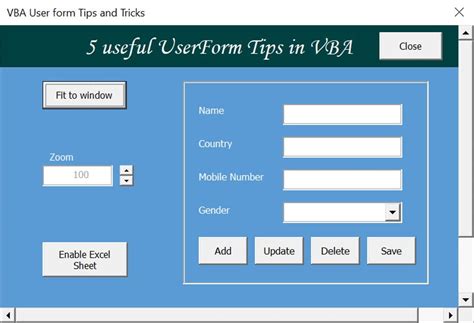
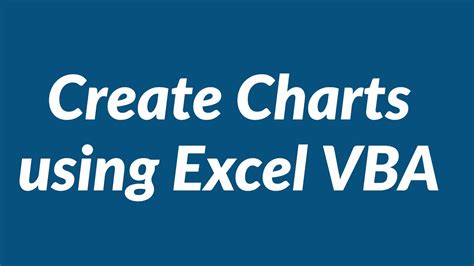
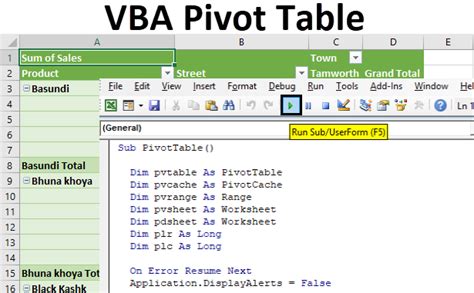
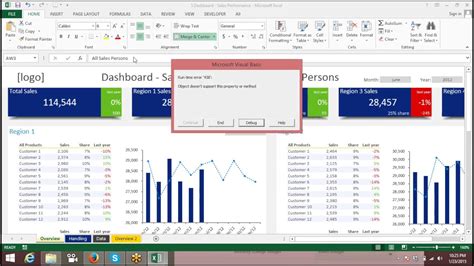
FAQs
How do I open the Visual Basic Editor in Excel?
+You can open the Visual Basic Editor by pressing Alt + F11 or by navigating to the Developer tab and clicking on Visual Basic.
What is the purpose of declaring variables in VBA?
+Declaring variables helps in making the code more readable, reduces errors, and improves performance by explicitly defining the data type of the variable.
Can I create multiple new worksheets at once using VBA?
+Yes, you can create multiple new worksheets by using a loop in your VBA code. This allows you to add multiple worksheets in a single execution of the code.
In conclusion, creating a new worksheet in Excel using VBA is a powerful tool for automating tasks and customizing your workbook to fit your specific needs. By following the steps outlined above and experimenting with different aspects of VBA programming, you can unlock a wide range of possibilities for managing and analyzing data in Excel. Whether you're a beginner looking to automate simple tasks or an advanced user seeking to create complex applications, VBA offers a flexible and robust platform for achieving your goals. So, take the first step today, and discover how VBA can transform the way you work with Excel.