Intro
Fix Runtime Error 9: Subscript Out of Range with expert solutions, troubleshooting steps, and code optimization tips to resolve VBA errors, debugging issues, and array index problems.
Runtime Error 9, also known as a "Subscript Out of Range" error, is a common issue that occurs when working with arrays, collections, or other data structures in programming. This error happens when you try to access an element that is outside the defined bounds of the array or collection. In this article, we will delve into the causes of this error, provide steps to fix it, and offer best practices to avoid it in the future.
The importance of understanding and addressing Runtime Error 9 cannot be overstated. It is a fundamental aspect of programming that can significantly impact the performance, reliability, and security of software applications. By grasping the concepts and techniques outlined in this article, developers can improve their coding skills, reduce debugging time, and create more robust and efficient programs.
The "Subscript Out of Range" error is not unique to any particular programming language; it can occur in various languages, including Visual Basic, C++, Java, and Python. The error message may vary slightly depending on the language and development environment, but the underlying cause is typically the same. To illustrate this point, consider a simple example in Visual Basic: if you declare an array with 10 elements ( indexed from 0 to 9) and then try to access the 11th element, you will encounter a Runtime Error 9.
Understanding the Causes of Runtime Error 9
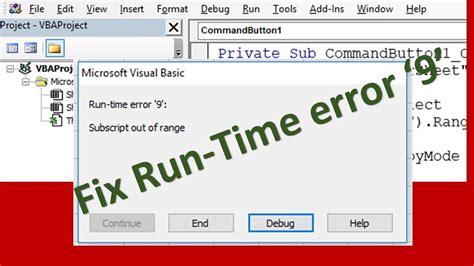
To fix a Runtime Error 9, it's essential to understand what causes it. The primary reasons for this error include:
- Attempting to access an array or collection element that does not exist.
- Using an incorrect index or key to access an element.
- Failing to initialize or properly declare an array or collection before use.
- Incorrectly assuming the size or bounds of an array or collection.
Common Scenarios Leading to Runtime Error 9
Several common scenarios can lead to a "Subscript Out of Range" error: - Looping through an array with a counter that exceeds the array's upper bound. - Using user-input data as an index without validating its range. - Copying code from one part of a program to another without adjusting array indices.Steps to Fix Runtime Error 9
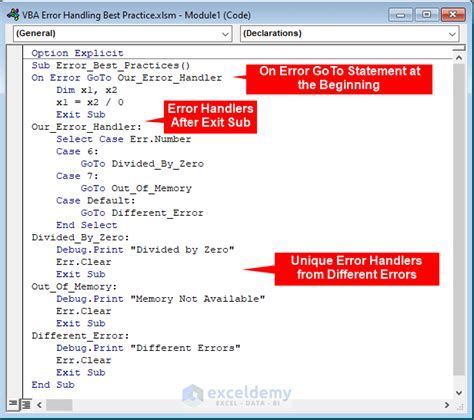
Fixing a Runtime Error 9 involves a systematic approach to identify and correct the issue:
- Review the Code: Carefully examine the line of code where the error occurs, focusing on array or collection accesses.
- Check Array Bounds: Verify that the array or collection is properly declared and initialized, and that you are not attempting to access an element outside its defined bounds.
- Validate Indices: Ensure that any indices or keys used to access elements are within the valid range.
- Use Debugging Tools: Utilize the debugger to step through the code, inspect variables, and identify the point at which the error occurs.
- Test Thoroughly: Once corrections are made, thoroughly test the application to ensure the error is resolved and no new issues are introduced.
Best Practices to Avoid Runtime Error 9
Adopting certain best practices can significantly reduce the likelihood of encountering a "Subscript Out of Range" error: - Always validate user input before using it to access arrays or collections. - Use built-in functions or methods to determine the size of arrays or collections. - Implement bounds checking for array accesses, especially in loops. - Keep code organized and well-documented to facilitate easier debugging.Example Code and Solutions
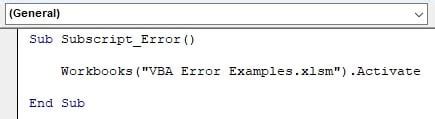
Consider the following example in Visual Basic, which demonstrates how to avoid a Runtime Error 9:
Dim myArray(10) As Integer
For i As Integer = 0 To myArray.Length - 1
myArray(i) = i * 2
Next
In this example, the loop iterates from 0 to the last valid index of myArray
, preventing a "Subscript Out of Range" error.
Additional Tips for Debugging
When debugging a Runtime Error 9, consider the following tips: - Use print statements or the debugger to display the values of indices and array bounds. - Test the application with different inputs to see if the error is consistent or dependent on specific conditions. - Consult documentation or online resources for the programming language or development environment being used.Conclusion and Future Directions
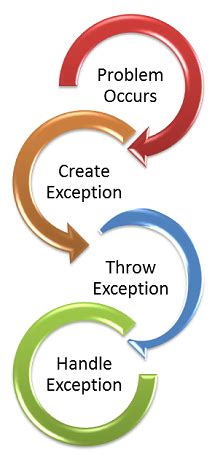
In conclusion, Runtime Error 9 is a common issue that can be addressed through a combination of understanding its causes, following best practices for coding, and systematically debugging applications. By adopting these strategies, developers can reduce the occurrence of "Subscript Out of Range" errors, improve the reliability of their software, and enhance their overall programming skills.
Final Thoughts on Error Handling
Effective error handling is crucial for the development of robust and user-friendly applications. It involves not only fixing errors like Runtime Error 9 but also anticipating and preventing potential issues through meticulous coding practices and comprehensive testing.Runtime Error 9 Image Gallery
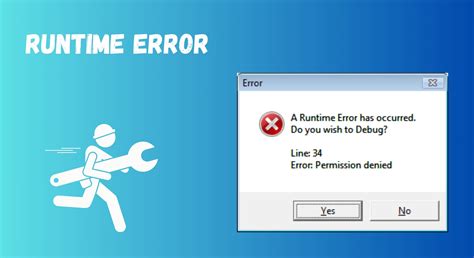
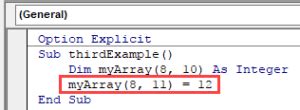
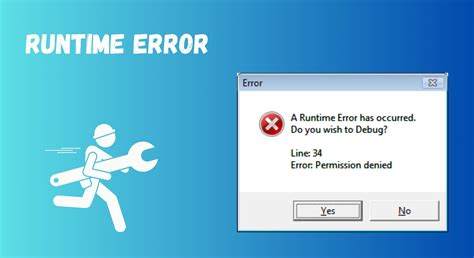
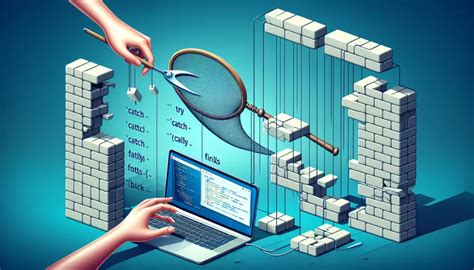
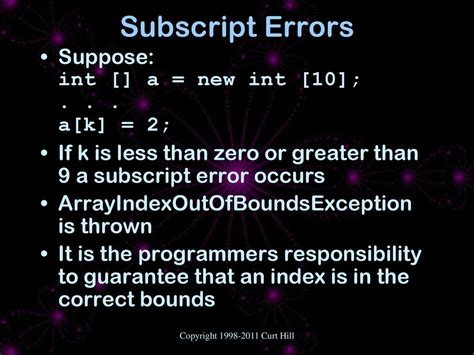
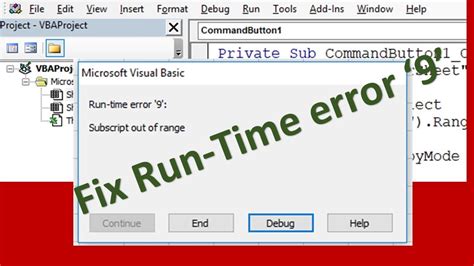
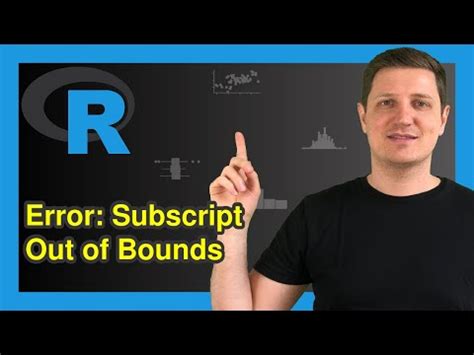
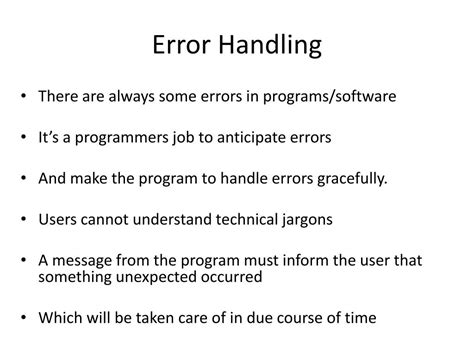
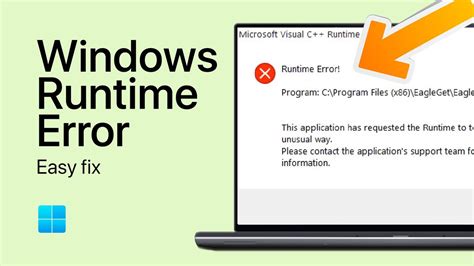
What is Runtime Error 9?
+Runtime Error 9, or "Subscript Out of Range," occurs when you attempt to access an element outside the bounds of an array or collection.
How do I fix a Runtime Error 9?
+To fix a Runtime Error 9, review your code, check array bounds, validate indices, use debugging tools, and test your application thoroughly.
Can I prevent Runtime Error 9?
+Yes, you can prevent Runtime Error 9 by following best practices such as validating user input, using built-in functions to determine array sizes, and implementing bounds checking.
We hope this comprehensive guide to Runtime Error 9 has been informative and helpful. Whether you are a seasoned developer or just starting out, understanding how to identify, fix, and prevent "Subscript Out of Range" errors is essential for creating reliable and efficient software applications. If you have any questions, comments, or would like to share your experiences with Runtime Error 9, please don't hesitate to reach out. Your feedback and insights are valuable to us and can help others in the programming community.