Intro
Troubleshoot VBA errors with 5 expert fixes, resolving macros issues, debugging code, and runtime errors to optimize Excel performance and stability.
Visual Basic for Applications (VBA) is a powerful tool used for creating and automating tasks in Microsoft Office applications, such as Excel, Word, and Access. However, like any programming language, VBA is not immune to errors. In this article, we will delve into five common VBA error fixes, providing you with the knowledge and skills to troubleshoot and resolve issues that may arise in your VBA projects.
VBA errors can be frustrating, especially for those who are new to programming. These errors can range from syntax errors to runtime errors, and understanding how to fix them is crucial for the successful execution of your VBA code. Whether you are a beginner or an experienced developer, being able to identify and resolve errors is an essential part of the VBA development process.
The importance of learning VBA error fixes cannot be overstated. Not only does it save time and reduce frustration, but it also enables you to create more robust and reliable VBA applications. By mastering the art of error fixing, you can ensure that your VBA projects run smoothly, efficiently, and without interruptions. In this article, we will explore five common VBA error fixes, providing you with practical examples, step-by-step instructions, and valuable tips to help you overcome the most common VBA errors.
Understanding VBA Errors
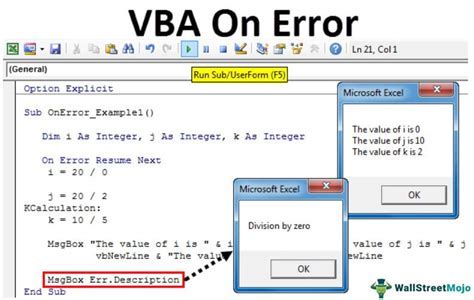
Before we dive into the five VBA error fixes, it is essential to understand the different types of errors that can occur in VBA. VBA errors can be broadly categorized into two main types: syntax errors and runtime errors. Syntax errors occur when there is a mistake in the code syntax, such as a missing or mismatched bracket, a typo, or an incorrect keyword. Runtime errors, on the other hand, occur during the execution of the code, often due to issues such as division by zero, out-of-range values, or missing references.
VBA Error Types
- Syntax errors: These errors occur when there is a mistake in the code syntax, such as a missing or mismatched bracket, a typo, or an incorrect keyword.
- Runtime errors: These errors occur during the execution of the code, often due to issues such as division by zero, out-of-range values, or missing references.
Error Fix 1: Syntax Error Fix
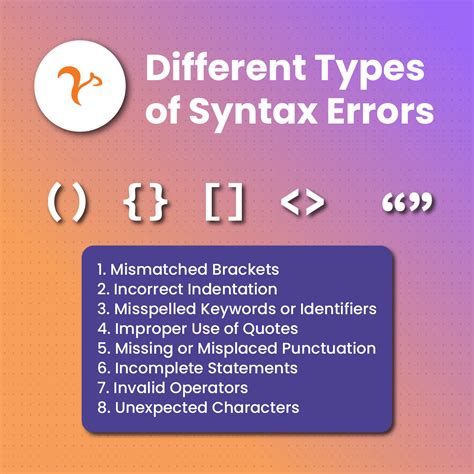
One of the most common VBA errors is the syntax error. Syntax errors can be frustrating, especially for beginners, as they can be difficult to identify and fix. To fix a syntax error, you need to carefully review your code, checking for any mistakes in the syntax, such as missing or mismatched brackets, typos, or incorrect keywords. Here are some steps to help you fix a syntax error:
- Review your code: Carefully review your code, checking for any mistakes in the syntax.
- Check for missing or mismatched brackets: Make sure that all brackets are properly matched and closed.
- Check for typos: Typos can be a common cause of syntax errors, so make sure to check your code for any spelling mistakes.
- Check for incorrect keywords: Ensure that you are using the correct keywords and syntax for the VBA function or statement you are trying to use.
Example of Syntax Error Fix
For example, suppose you have the following code:
If x > 5 Then
MsgBox "x is greater than 5"
This code will result in a syntax error because the If
statement is not properly closed. To fix this error, you need to add the End If
statement to close the If
block:
If x > 5 Then
MsgBox "x is greater than 5"
End If
Error Fix 2: Runtime Error Fix
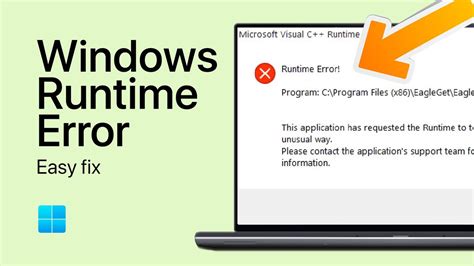
Runtime errors can be more challenging to fix than syntax errors, as they often occur during the execution of the code. To fix a runtime error, you need to identify the cause of the error and modify your code to prevent it from occurring. Here are some steps to help you fix a runtime error:
- Identify the error: Use the VBA error message to identify the cause of the error.
- Check for division by zero: Make sure that you are not dividing by zero, as this can result in a runtime error.
- Check for out-of-range values: Ensure that your variables are not exceeding their maximum or minimum values.
- Check for missing references: Make sure that all references are properly set and that there are no missing references.
Example of Runtime Error Fix
For example, suppose you have the following code:
x = 5 / 0
This code will result in a runtime error because you are dividing by zero. To fix this error, you need to add a check to ensure that you are not dividing by zero:
If y <> 0 Then
x = 5 / y
Else
MsgBox "Cannot divide by zero"
End If
Error Fix 3: Object Reference Error Fix
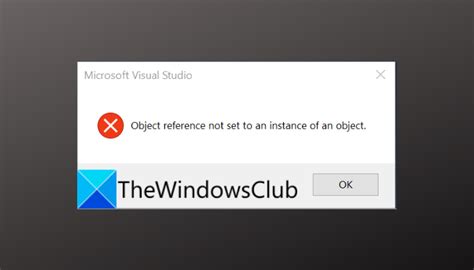
Object reference errors occur when you try to access an object that does not exist or is not properly referenced. To fix an object reference error, you need to ensure that the object is properly declared and referenced. Here are some steps to help you fix an object reference error:
- Declare the object: Make sure that the object is properly declared using the
Dim
statement. - Set the object reference: Use the
Set
statement to set the object reference. - Check for typos: Ensure that the object name is spelled correctly and that there are no typos.
Example of Object Reference Error Fix
For example, suppose you have the following code:
Dim ws As Worksheet
ws.Range("A1").Value = 5
This code will result in an object reference error because the ws
object is not properly set. To fix this error, you need to set the ws
object reference using the Set
statement:
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
ws.Range("A1").Value = 5
Error Fix 4: Type Mismatch Error Fix
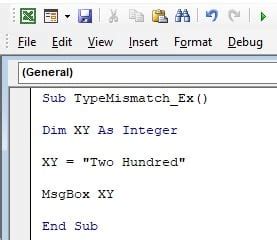
Type mismatch errors occur when you try to assign a value to a variable that is not of the same data type. To fix a type mismatch error, you need to ensure that the variable is declared with the correct data type. Here are some steps to help you fix a type mismatch error:
- Declare the variable: Make sure that the variable is properly declared using the
Dim
statement. - Check the data type: Ensure that the variable is declared with the correct data type.
- Use type conversion functions: Use type conversion functions, such as
CInt
orCDbl
, to convert the value to the correct data type.
Example of Type Mismatch Error Fix
For example, suppose you have the following code:
Dim x As Integer
x = "hello"
This code will result in a type mismatch error because you are trying to assign a string value to an integer variable. To fix this error, you need to declare the variable with the correct data type:
Dim x As String
x = "hello"
Error Fix 5: Loop Error Fix
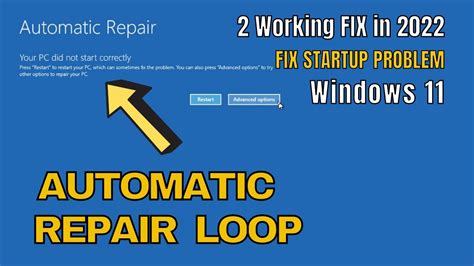
Loop errors occur when a loop is not properly terminated or when the loop counter is not properly incremented. To fix a loop error, you need to ensure that the loop is properly terminated and that the loop counter is properly incremented. Here are some steps to help you fix a loop error:
- Check the loop termination: Ensure that the loop is properly terminated using the
Exit
statement. - Check the loop counter: Ensure that the loop counter is properly incremented using the
Increment
statement. - Use a
For
loop: Consider using aFor
loop instead of aWhile
loop to avoid loop errors.
Example of Loop Error Fix
For example, suppose you have the following code:
x = 0
While x < 10
x = x + 1
Wend
This code will result in a loop error because the loop is not properly terminated. To fix this error, you need to add an Exit
statement to terminate the loop:
x = 0
While x < 10
x = x + 1
If x = 10 Then Exit While
Wend
Gallery of VBA Error Fixes
VBA Error Fixes Image Gallery
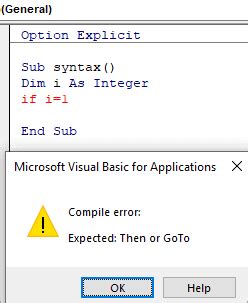
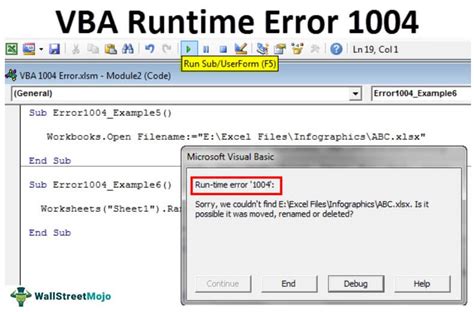
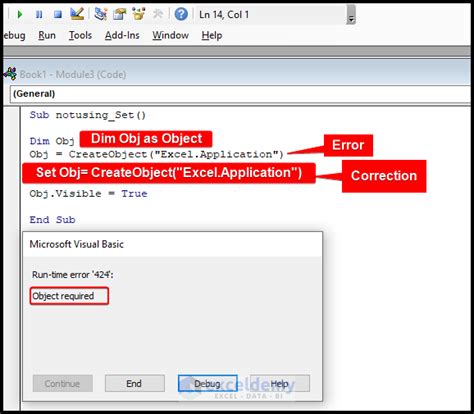
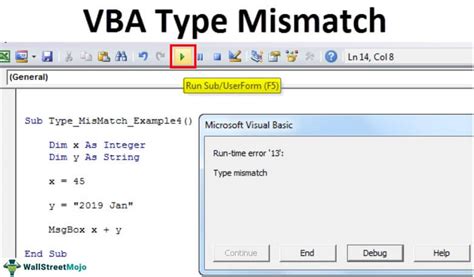
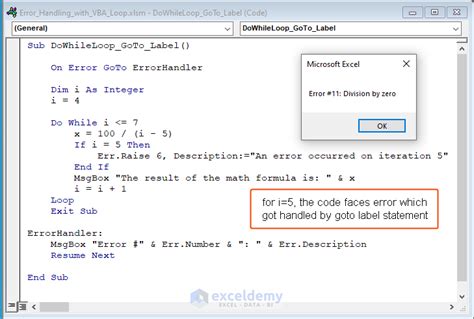
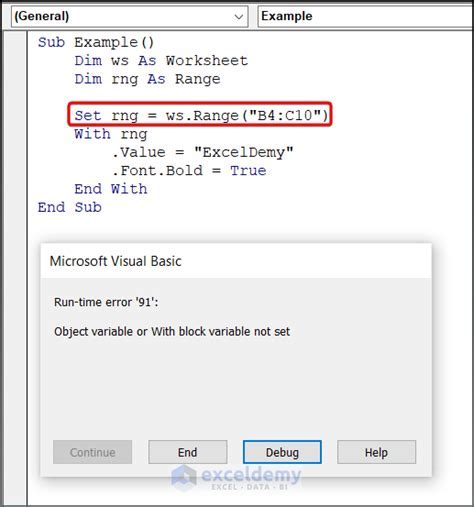
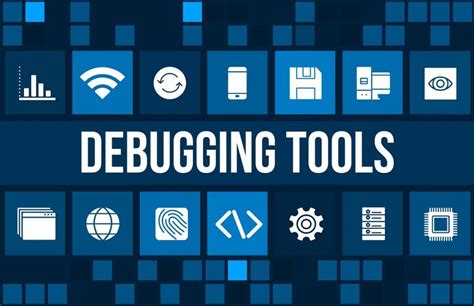
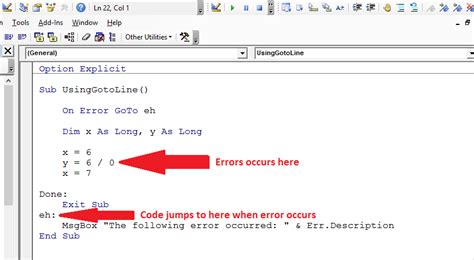
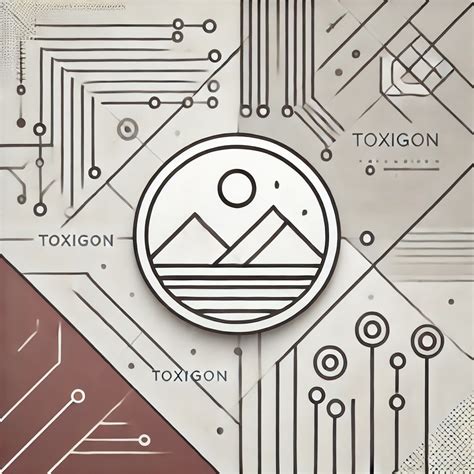
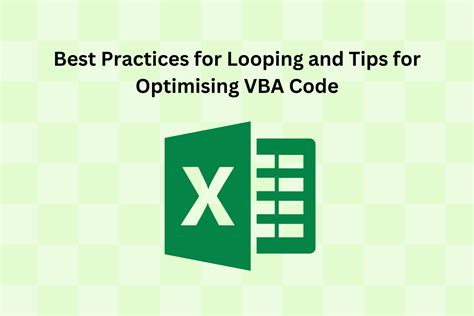
What are the most common VBA errors?
+The most common VBA errors include syntax errors, runtime errors, object reference errors, type mismatch errors, and loop errors.
How do I fix a syntax error in VBA?
+To fix a syntax error in VBA, you need to carefully review your code, checking for any mistakes in the syntax, such as missing or mismatched brackets, typos, or incorrect keywords.
What is the difference between a syntax error and a runtime error?
+A syntax error occurs when there is a mistake in the code syntax, while a runtime error occurs during the execution of the code, often due to issues such as division by zero, out-of-range values, or missing references.
How do I troubleshoot a VBA error?
+To troubleshoot a VBA error, you need to identify the cause of the error, check the error message, and use debugging tools, such as the VBA debugger, to step through the code and identify the source of the error.
What are some best practices for avoiding VBA errors?
+Some best practices for avoiding VBA errors include using descriptive variable names, commenting your code, using error handling routines, and testing your code thoroughly.
In conclusion, VBA errors can be frustrating, but by understanding the different types of errors and how to fix them, you can create more robust and reliable VBA applications. By following the five VBA error fixes outlined in this article, you can troubleshoot and resolve common errors, such as syntax errors, runtime errors, object reference errors, type mismatch errors, and loop errors. Remember to always test your code thoroughly, use descriptive variable names, and comment your code to avoid errors and make it easier to debug. With practice and experience, you will become proficient in fixing VBA errors and creating efficient and effective VBA applications. If you have any questions or need further assistance, please don't hesitate to comment or share this article with others.