Intro
When working with Visual Basic for Applications (VBA) in Microsoft Office, particularly in Excel, you might encounter an error that reads "Argument Not Optional." This error typically occurs when you're trying to use a VBA function or subroutine but haven't provided all the required arguments. Understanding and fixing this error is crucial for smooth execution of your VBA scripts. Let's delve into the details of what this error means and how to fix it.
The "Argument Not Optional" error in VBA is essentially a compiler error that stops your code from running because it detects a missing argument in a function or subroutine call. This can happen for several reasons, such as misspelling the function name, forgetting to include a required argument, or passing arguments in the wrong order. To resolve this issue, you need to identify the line of code causing the problem and ensure that all necessary arguments are provided correctly.
Understanding VBA Errors
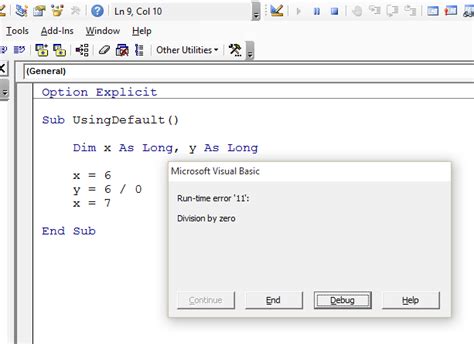
Before diving into the specifics of fixing the "Argument Not Optional" error, it's helpful to understand how VBA errors are categorized and handled. VBA errors can be either compile-time errors, which prevent your code from compiling, or runtime errors, which occur while your code is executing. The "Argument Not Optional" error falls into the compile-time category because it's detected by the VBA compiler before your code even runs.
VBA Error Types
- **Compile-time errors** are detected by the VBA compiler and prevent your code from running. Examples include syntax errors, undefined variables, and the "Argument Not Optional" error. - **Runtime errors** occur during the execution of your code. These can be due to various reasons such as attempting to divide by zero, trying to access an array out of its bounds, or other logical errors.Fixing the Argument Not Optional Error

To fix the "Argument Not Optional" error, follow these steps:
- Identify the Error Line: The first step is to locate the line of code that's causing the error. VBA will typically highlight this line in red or display an error message indicating the problematic line.
- Check the Function/Subroutine Definition: Look at the definition of the function or subroutine you're trying to call. Check what arguments it requires and ensure you're providing all of them.
- Review Argument Order: Make sure you're passing the arguments in the correct order. The order of arguments in your function call must match the order in which they're defined in the function or subroutine.
- Provide Optional Arguments: If an argument is truly optional, it should be defined as such in the function or subroutine definition by using the "Optional" keyword. If you're calling a function and don't want to provide a value for an optional argument, you can omit it, but you must still provide values for all required arguments that come before it.
- Use Named Arguments: To avoid confusion, especially when dealing with functions that have many arguments, consider using named arguments. This involves specifying the name of the argument followed by its value in the function call, like
MyFunction(requiredArg1:="value1", optionalArg2:=123)
.
Example of Fixing the Error
Consider a simple VBA subroutine that requires two arguments: ```vba Sub MySubroutine(arg1 As String, arg2 As Integer) ' Code here End Sub ``` If you try to call this subroutine with only one argument, like `MySubroutine "Hello"`, you'll get the "Argument Not Optional" error because `arg2` is required. To fix this, you must provide a value for `arg2`, like `MySubroutine "Hello", 123`.Best Practices for Avoiding VBA Errors
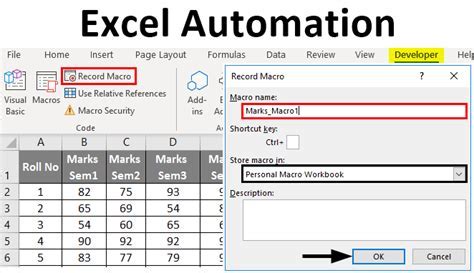
While the "Argument Not Optional" error is specific, following best practices can help minimize errors in your VBA code:
- Use Option Explicit: This directive at the top of your module forces you to declare all variables, reducing errors due to typos or undefined variables.
- Declare Variables: Always declare your variables with specific data types to avoid type mismatch errors.
- Test Thoroughly: Before considering your code complete, test it extensively with different inputs and scenarios to catch and fix runtime errors.
Additional Tips
- **Comment Your Code**: Comments can help you and others understand what your code is supposed to do, making it easier to identify and fix errors. - **Use Error Handling**: Implement error handling mechanisms, such as `On Error GoTo` statements, to gracefully manage runtime errors and provide useful feedback instead of crashing.Gallery of VBA Error Fixes
VBA Error Fixes Image Gallery
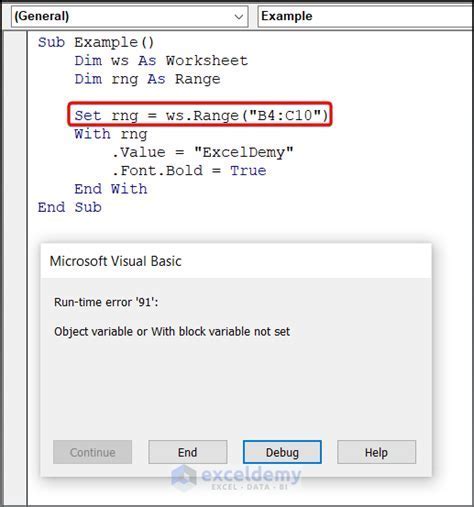
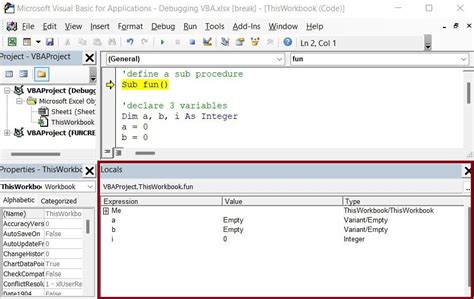
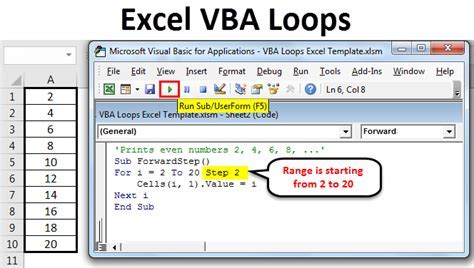
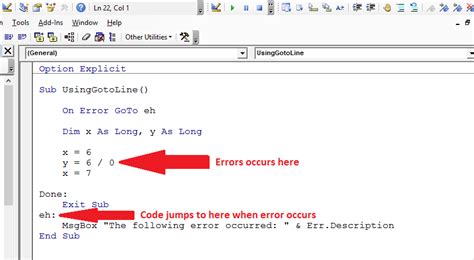
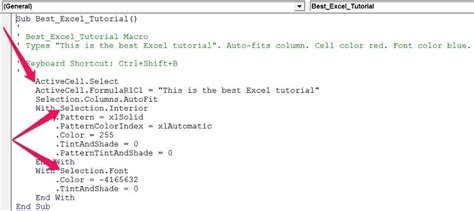
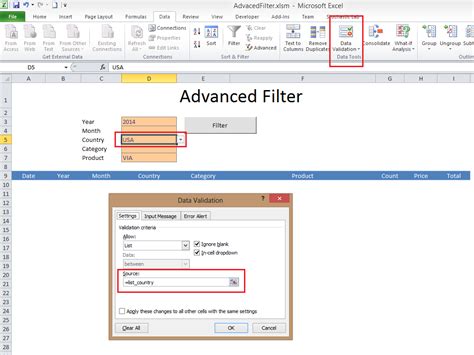
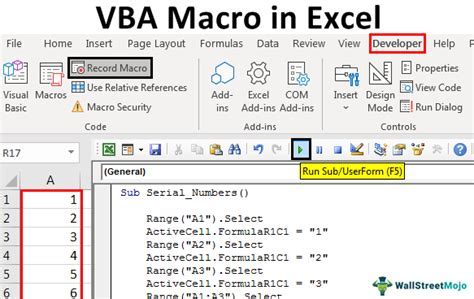
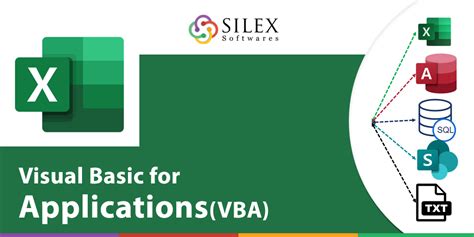
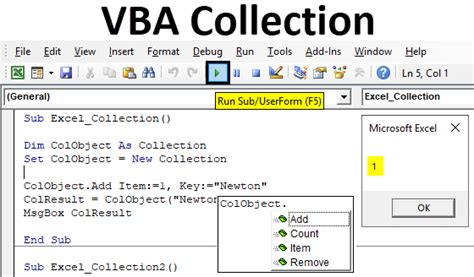
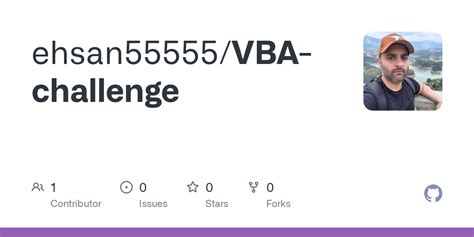
Frequently Asked Questions
What is the "Argument Not Optional" error in VBA?
+The "Argument Not Optional" error occurs when you try to call a VBA function or subroutine without providing all the required arguments.
How do I fix the "Argument Not Optional" error?
+To fix this error, ensure you're providing all required arguments for the function or subroutine you're calling. Check the order and types of arguments as well.
What are some best practices for avoiding VBA errors?
+Best practices include using Option Explicit, declaring variables, testing your code thoroughly, and implementing error handling mechanisms.
If you've encountered the "Argument Not Optional" error in your VBA journey, understanding its cause and applying the fixes outlined above should help resolve the issue. Remember, practice and patience are key to mastering VBA and troubleshooting its errors. Feel free to share your experiences or ask further questions in the comments below, and don't forget to share this article with anyone who might benefit from learning about VBA error fixes.