Intro
Learn to copy worksheets to new workbooks using VBA, with expert tips on worksheet duplication, Excel automation, and macro scripting for efficient data transfer and workbook management.
The ability to copy a worksheet to a new workbook using VBA (Visual Basic for Applications) is a powerful feature in Excel that can greatly enhance your productivity and workflow. This capability is especially useful when you need to distribute specific worksheets to different teams or individuals, or when you're organizing your data into separate workbooks for better management. Below, we'll explore how to accomplish this task using VBA, including step-by-step instructions and example code.
To start working with VBA in Excel, you first need to access the Visual Basic Editor. You can do this by pressing Alt
+ F11
or by navigating to the Developer
tab (if available) and clicking on Visual Basic
. If you don't see the Developer
tab, you can add it by going to File
> Options
> Customize Ribbon
, checking the Developer
checkbox, and clicking OK
.
Copying a Worksheet to a New Workbook
The following VBA code snippet demonstrates how to copy the active worksheet to a new workbook. This example assumes you want to copy the entire worksheet, including all data, formatting, and any other elements it might contain.
Sub CopyWorksheetToNewWorkbook()
Dim newWorkbook As Workbook
Dim sourceWorksheet As Worksheet
' Set the source worksheet to the active sheet
Set sourceWorksheet = ActiveSheet
' Copy the worksheet to a new workbook
sourceWorksheet.Copy
' The ActiveWorkbook will now be the new workbook that was created
' by the copy operation. We set this to our newWorkbook variable.
Set newWorkbook = ActiveWorkbook
' Optionally, you can save the new workbook immediately
' newWorkbook.SaveAs "Path\To\Save\NewWorkbook.xlsx"
MsgBox "Worksheet copied to a new workbook."
End Sub
This script defines a subroutine named CopyWorksheetToNewWorkbook
that performs the following actions:
- Sets the source worksheet to the currently active sheet.
- Copies the source worksheet. Excel automatically creates a new workbook and pastes the copied worksheet into it.
- Optionally, you can uncomment the
SaveAs
line to save the new workbook to a specified location.
Specifying the Source and Destination
If you need more control over which worksheet is copied and where it's copied to, you can modify the script to accept specific worksheet names or references. For example, to copy a worksheet named "Sheet1" to a new workbook, you could modify the script like this:
Sub CopySpecificWorksheetToNewWorkbook()
Dim newWorkbook As Workbook
Dim sourceWorksheet As Worksheet
' Set the source worksheet to a specific sheet
Set sourceWorksheet = ThisWorkbook.Worksheets("Sheet1")
' Copy the worksheet to a new workbook
sourceWorksheet.Copy
' Set the new workbook
Set newWorkbook = ActiveWorkbook
' Optionally, save the new workbook
' newWorkbook.SaveAs "Path\To\Save\NewWorkbook.xlsx"
MsgBox "Specific worksheet copied to a new workbook."
End Sub
Gallery of Copying Worksheets
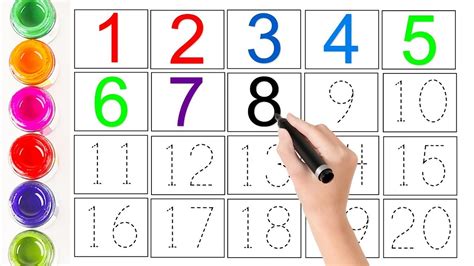
Copying Multiple Worksheets
If you need to copy multiple worksheets to a new workbook, you can loop through the worksheets you wish to copy and use the Worksheets.Copy
method to copy them. Here's an example:
Sub CopyMultipleWorksheetsToNewWorkbook()
Dim newWorkbook As Workbook
Dim sourceWorkbook As Workbook
Dim worksheet As Worksheet
Dim worksheetsToCopy As Variant
Dim i As Long
' Set the source workbook
Set sourceWorkbook = ThisWorkbook
' Specify the worksheets to copy by name
worksheetsToCopy = Array("Sheet1", "Sheet2", "Sheet3")
' Create a new workbook
Set newWorkbook = Workbooks.Add
' Loop through each worksheet to copy and copy it
For i = LBound(worksheetsToCopy) To UBound(worksheetsToCopy)
Set worksheet = sourceWorkbook.Worksheets(worksheetsToCopy(i))
worksheet.Copy After:=newWorkbook.Worksheets(newWorkbook.Worksheets.Count)
Next i
' Remove the initial worksheet in the new workbook if necessary
Application.DisplayAlerts = False
newWorkbook.Worksheets(1).Delete
Application.DisplayAlerts = True
' Optionally, save the new workbook
' newWorkbook.SaveAs "Path\To\Save\NewWorkbook.xlsx"
MsgBox "Multiple worksheets copied to a new workbook."
End Sub
This example shows how to copy multiple worksheets specified by their names to a new workbook, and then optionally save the new workbook.
Gallery of VBA Scripts
VBA Scripts Gallery
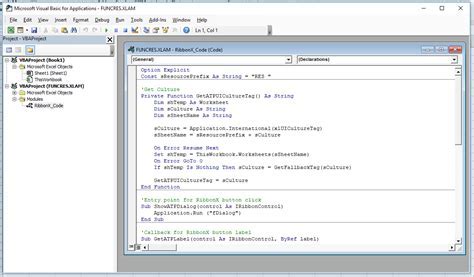
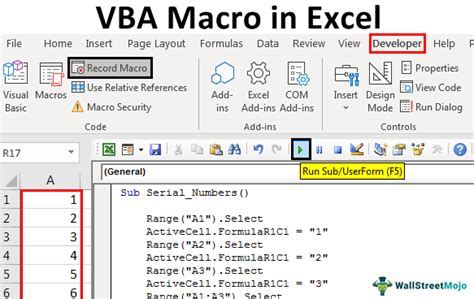
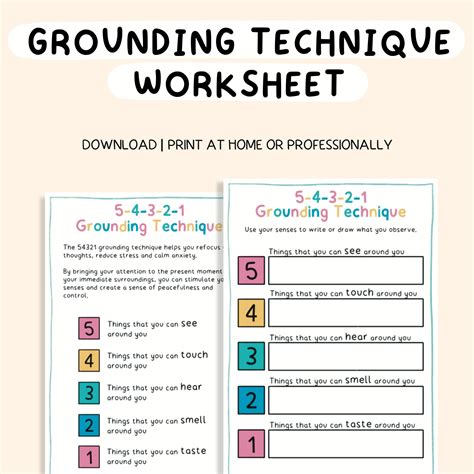
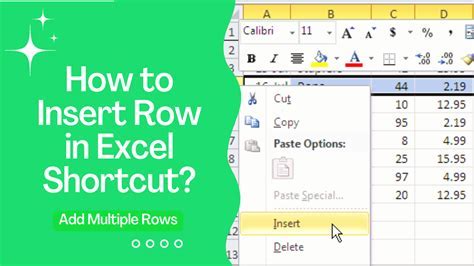
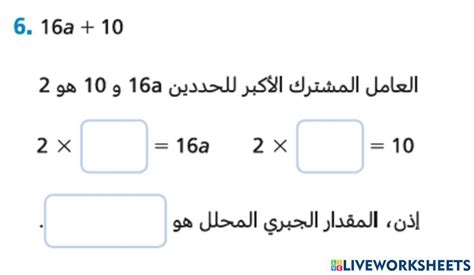
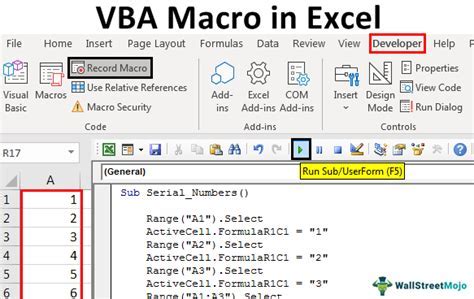
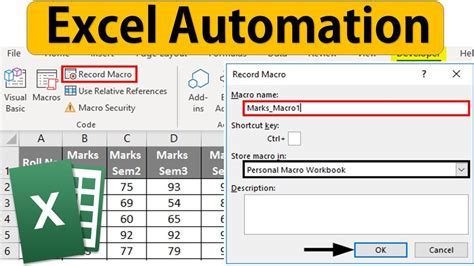
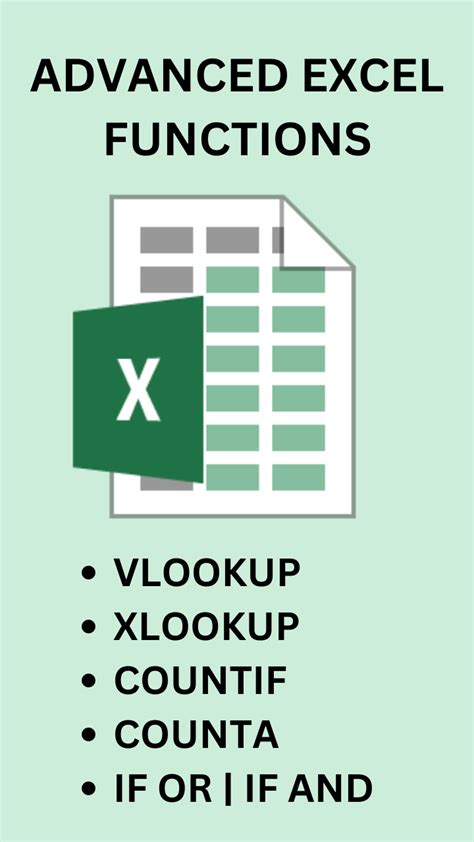
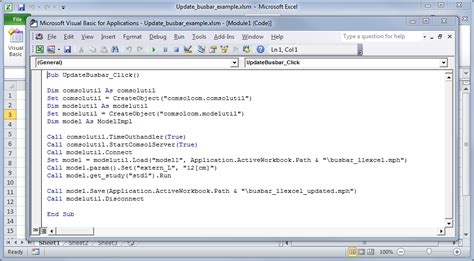
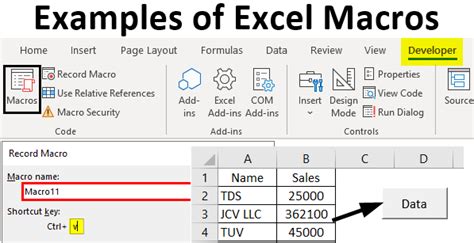
FAQs
How do I access the VBA editor in Excel?
+You can access the VBA editor by pressing `Alt` + `F11` or by navigating to the `Developer` tab and clicking on `Visual Basic`.
Can I copy multiple worksheets to a new workbook using VBA?
+Yes, you can copy multiple worksheets by looping through the worksheets you wish to copy and using the `Worksheets.Copy` method.
How do I specify which worksheets to copy in my VBA script?
+You can specify worksheets by their names or by their index. Using names is more readable and less prone to errors if the worksheet order changes.
In conclusion, copying worksheets to new workbooks using VBA is a straightforward yet powerful technique for managing and distributing your Excel data. By mastering this skill, you can significantly streamline your workflow and improve your productivity. Whether you're working with a single worksheet or multiple worksheets, VBA provides the flexibility and automation you need to get the job done efficiently. So, don't hesitate to explore the world of VBA scripting and discover how it can revolutionize the way you work with Excel. Feel free to share your experiences, ask questions, or provide feedback on using VBA for worksheet manipulation in the comments below.