Intro
Learn to change Excel macro button color when clicked using VBA, conditional formatting, and macro settings to enhance user experience and interaction.
The use of macros in Excel can greatly enhance the functionality and user experience of a spreadsheet. One common requirement is to change the color of a macro button when it is clicked, providing visual feedback to the user. This can be particularly useful in scenarios where the button's action triggers a process that takes some time to complete, or when the button's state needs to reflect its current status (e.g., enabled or disabled).
To achieve this, you can use Excel VBA (Visual Basic for Applications), which allows you to create and manage macros, including changing the properties of form controls like buttons. Below is a step-by-step guide on how to change the color of a macro button when clicked in Excel.
First, ensure you have a button in your Excel sheet. If you don't have one, you can add it by going to the "Developer" tab, clicking on "Insert" under the "Controls" group, and then selecting the "Command Button" from the "ActiveX Controls" group. If the "Developer" tab is not visible, you can enable it by going to "File" > "Options" > "Customize Ribbon" and checking the "Developer" checkbox.
Enabling the Developer Tab and Adding a Button
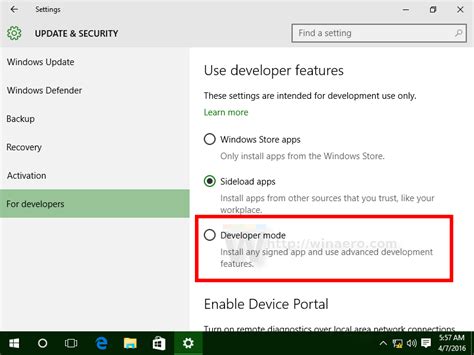
Once you have your button, you can proceed to write the VBA code that changes its color when clicked. To access the VBA editor, press "Alt" + "F11" or navigate to the "Developer" tab and click on "Visual Basic".
Accessing the VBA Editor
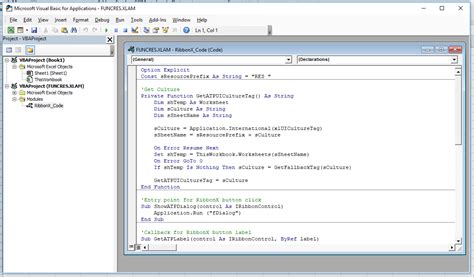
In the VBA editor, you'll see the "Project Explorer" on the left. Find your workbook and the sheet where your button is located. If you double-click on the button, it will open a code module where you can write the code for the button's click event.
Writing the Button Click Event Code
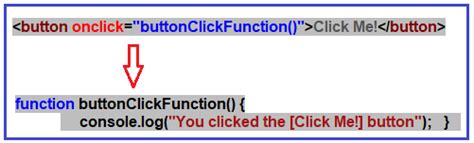
Here's an example of VBA code that changes the button's color when clicked:
Private Sub CommandButton1_Click()
CommandButton1.BackColor = vbRed ' Change the background color to red
' Add your macro code here
End Sub
Replace CommandButton1
with the actual name of your button. The BackColor
property is used to change the background color of the button. vbRed
is a predefined constant for the color red, but you can use other constants or specify the color using its RGB value.
Understanding Color Constants and RGB Values
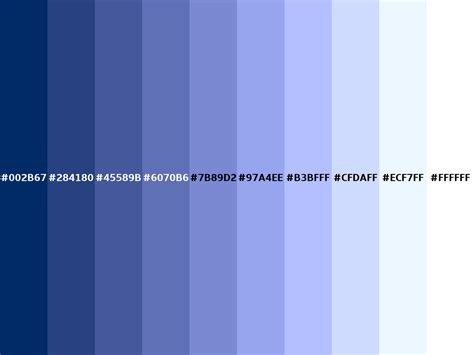
For example, to change the color to a specific shade of blue, you could use:
CommandButton1.BackColor = RGB(0, 0, 255) ' Pure blue
After writing your code, close the VBA editor and save your workbook as a macro-enabled file (.xlsm
).
Saving the Workbook with Macros
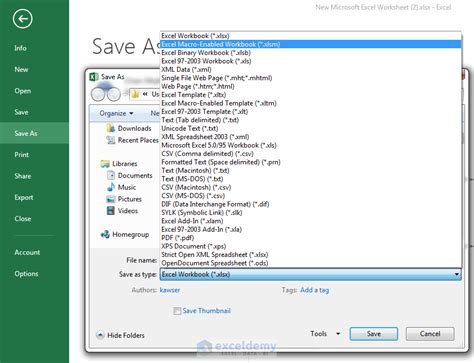
Now, when you click the button, it should change color according to the code you've written. This is a basic example, but you can expand upon it by adding more functionality or changing the button's appearance in other ways, such as its font, size, or even making it invisible under certain conditions.
Expanding Button Functionality
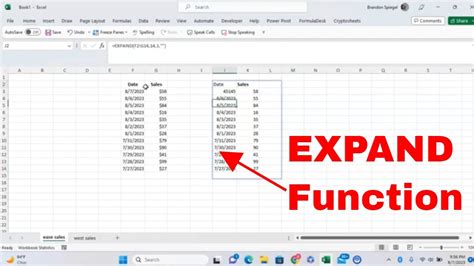
Practical Applications
Changing the color of a macro button when clicked has numerous practical applications, including:
- Visual Feedback: Providing immediate visual feedback to the user that an action has been triggered.
- Status Indication: Indicating the status of a process or the state of the button (e.g., enabled/disabled).
- User Guidance: Guiding the user through a series of steps by changing the button's color as each step is completed.
Benefits of Visual Feedback
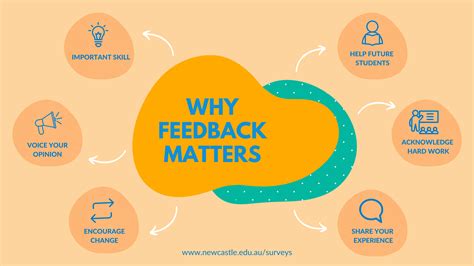
Troubleshooting
If your button's color doesn't change as expected, ensure:
- The button is an ActiveX control and not a Form control.
- The VBA code is correctly written and associated with the button's click event.
- Macros are enabled in your Excel settings.
Troubleshooting Common Issues
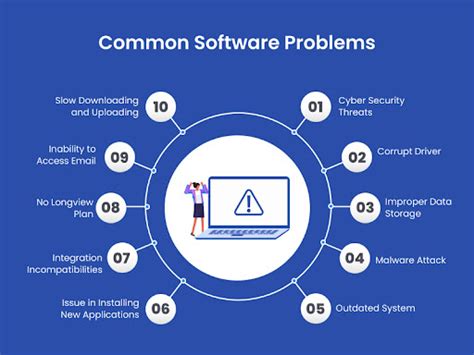
Gallery of Excel Macro Buttons
Excel Macro Button Gallery
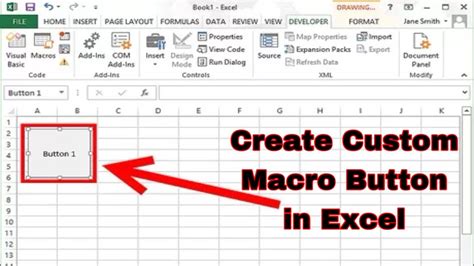
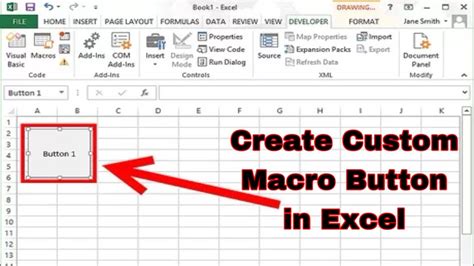
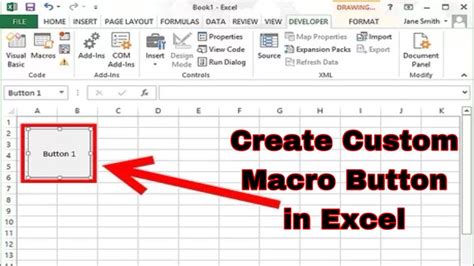
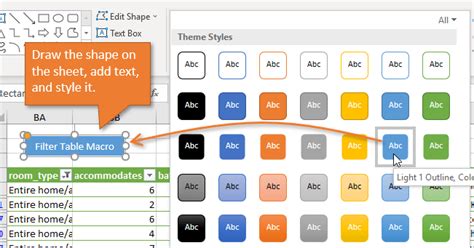
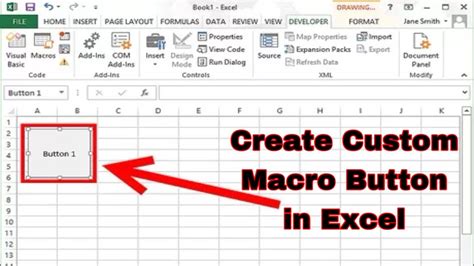
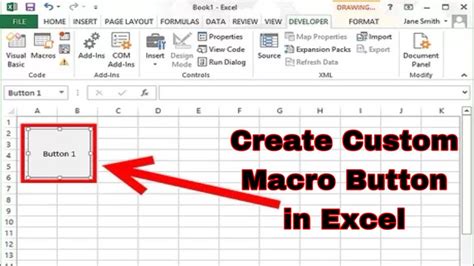
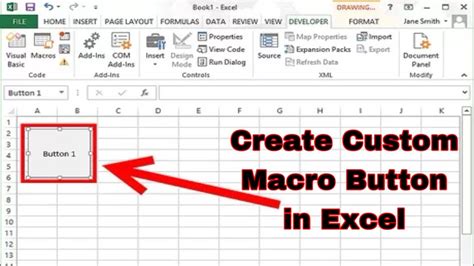
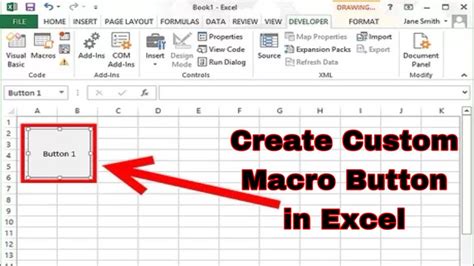
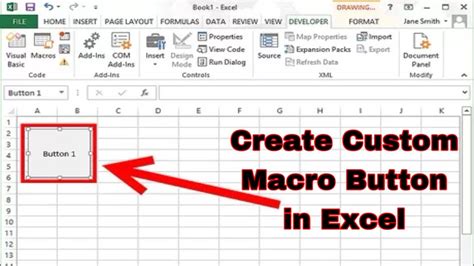
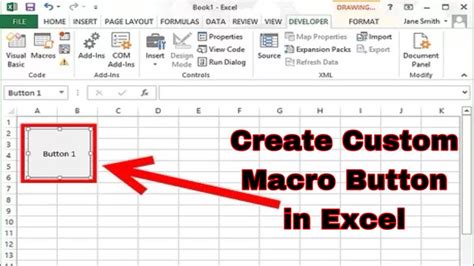
FAQs
How do I enable macros in Excel?
+To enable macros, go to "File" > "Options" > "Trust Center" > "Trust Center Settings" > "Macro Settings" and select "Enable all macros" or "Disable all macros except digitally signed macros".
What is the difference between an ActiveX control and a Form control in Excel?
+ActiveX controls are more flexible and can be used with VBA, while Form controls are simpler and primarily used for basic interactions like buttons and checkboxes.
How do I change the font color of a macro button in Excel?
+You can change the font color of a macro button by using the `ForeColor` property in VBA, e.g., `CommandButton1.ForeColor = vbBlue`.
If you have any questions or need further assistance with changing the color of a macro button in Excel or any other Excel-related queries, feel free to ask in the comments below. Your feedback and suggestions are also welcome, helping us to improve and provide more useful content in the future.