Intro
Master VBA referencing cells with ease. Learn how to reference cells, ranges, and worksheets using VBA variables, loops, and Excel object models, optimizing your macros with cell references, range offsets, and worksheet interactions.
When working with Visual Basic for Applications (VBA) in Excel, referencing cells is a fundamental aspect of creating effective macros and interacting with the worksheet. Understanding how to reference cells is essential for performing various tasks, such as data manipulation, calculations, and automation. In this article, we will delve into the world of VBA cell referencing, exploring its importance, the different methods available, and providing practical examples to help you master this crucial skill.
The ability to accurately reference cells in VBA is vital for several reasons. Firstly, it allows you to access and manipulate data within your worksheets, enabling you to automate tasks and improve efficiency. Secondly, proper cell referencing helps prevent errors, ensuring that your macros operate as intended and reducing the risk of data corruption. Finally, understanding cell referencing is a building block for more complex VBA operations, such as looping through ranges, creating charts, and interacting with other Excel objects.
Methods of Referencing Cells in VBA
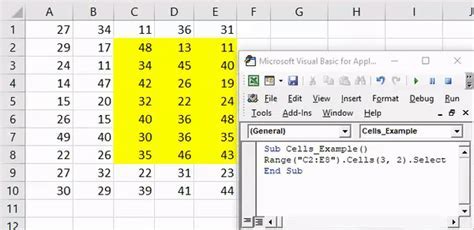
There are several methods to reference cells in VBA, each with its own advantages and use cases. The most common methods include using the Range
object, the Cells
property, and the Offset
property. The Range
object is the most versatile, allowing you to reference a single cell or a range of cells using the Range("A1")
or Range("A1:B2")
syntax. The Cells
property is useful for referencing cells based on their row and column numbers, such as Cells(1, 1)
for the cell at row 1, column 1. The Offset
property enables you to reference cells relative to a specified range, for example, Range("A1").Offset(1, 0)
to reference the cell one row below A1.
Using the Range Object
The `Range` object is the most common method for referencing cells in VBA. It provides a flexible way to access and manipulate cells, allowing you to specify a single cell or a range of cells. To use the `Range` object, you simply need to specify the range address in quotes, such as `Range("A1")` or `Range("A1:B2")`. You can also use the `Range` object to reference named ranges, which can be useful for making your code more readable and maintainable.Using the Cells Property
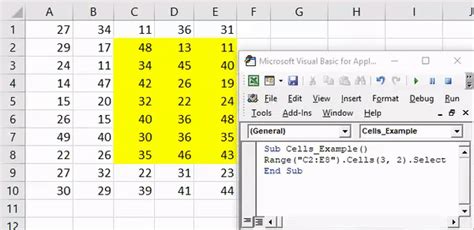
The Cells
property is another popular method for referencing cells in VBA. It allows you to specify the row and column numbers of the cell you want to reference, such as Cells(1, 1)
for the cell at row 1, column 1. This method is particularly useful when you need to reference cells dynamically, such as when looping through a range of cells. You can also use the Cells
property to reference a range of cells by specifying the row and column numbers of the top-left and bottom-right cells, such as Range(Cells(1, 1), Cells(2, 2))
.
Practical Examples of Cell Referencing in VBA
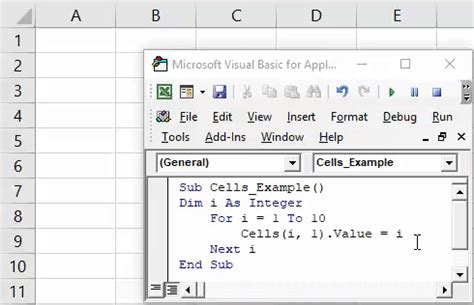
To illustrate the concepts discussed above, let's consider a few practical examples of cell referencing in VBA. Suppose you want to write a macro that copies the value from cell A1 to cell B1. You can use the Range
object to reference the cells, like this: Range("B1").Value = Range("A1").Value
. Alternatively, you can use the Cells
property to achieve the same result: Cells(1, 2).Value = Cells(1, 1).Value
.
Another example might involve looping through a range of cells and performing a calculation on each cell. You can use the Cells
property to reference each cell in the loop, like this:
For i = 1 To 10
Cells(i, 1).Value = Cells(i, 1).Value * 2
Next i
This code loops through the cells in column A, rows 1 to 10, and multiplies the value in each cell by 2.
Best Practices for Cell Referencing in VBA
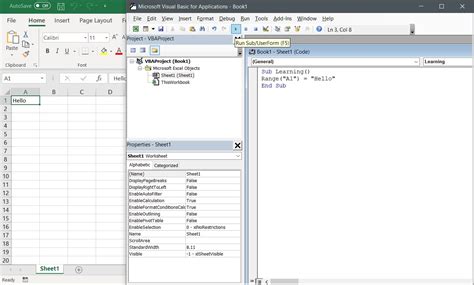
To ensure that your VBA code is efficient, readable, and maintainable, it's essential to follow best practices for cell referencing. Here are a few tips to keep in mind:
- Always use explicit references to cells and ranges, rather than relying on implicit references.
- Use the
Range
object orCells
property to reference cells, rather than usingActiveCell
orSelection
. - Avoid using
Select
andActivate
methods, as they can slow down your code and cause errors. - Use named ranges to make your code more readable and maintainable.
- Always test your code thoroughly to ensure that it works as intended.
Common Errors in Cell Referencing
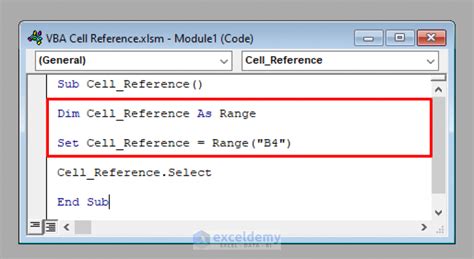
When working with cell referencing in VBA, it's common to encounter errors, especially if you're new to programming. Here are a few common errors to watch out for:
- Referencing a cell that doesn't exist, such as a cell outside the bounds of the worksheet.
- Using an incorrect range address or cell reference.
- Forgetting to specify the worksheet or workbook when referencing a cell.
- Using
Select
andActivate
methods, which can cause errors and slow down your code.
To avoid these errors, it's essential to test your code thoroughly and use explicit references to cells and ranges.
Debugging Cell Referencing Issues
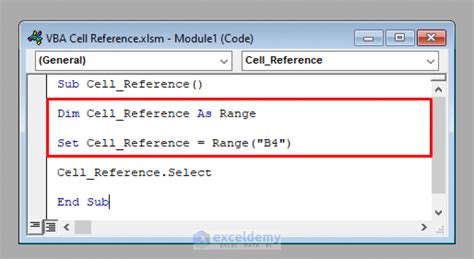
When debugging cell referencing issues, it's essential to use the correct tools and techniques. Here are a few tips to help you debug your code:
- Use the VBA debugger to step through your code and identify errors.
- Use the
Debug.Print
statement to print values to the Immediate window. - Use the
MsgBox
function to display error messages and debug information. - Always test your code thoroughly to ensure that it works as intended.
VBA Cell Referencing Image Gallery
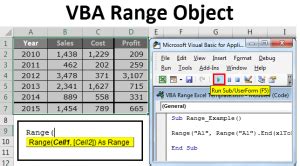
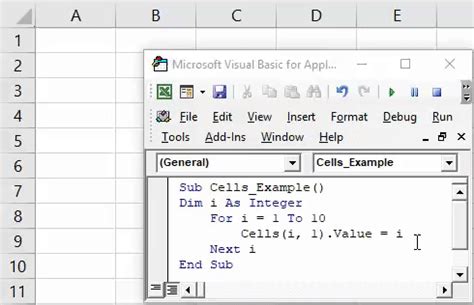
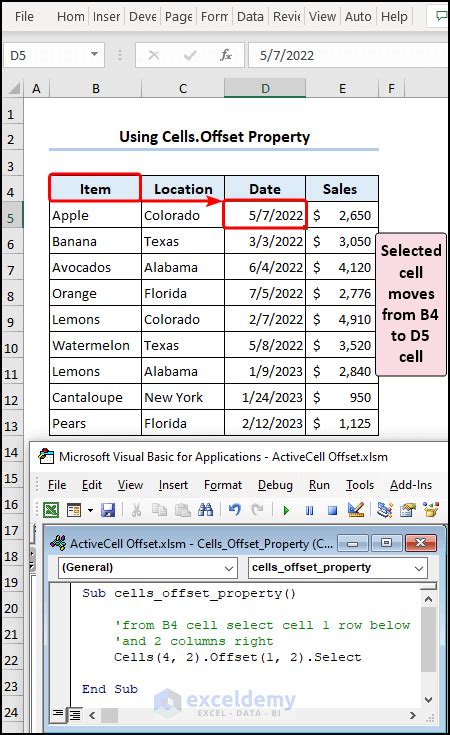
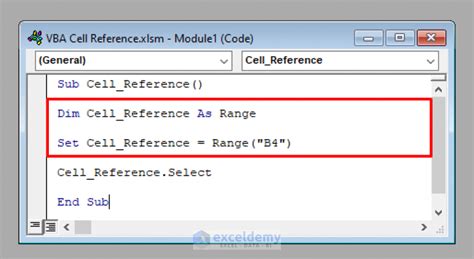
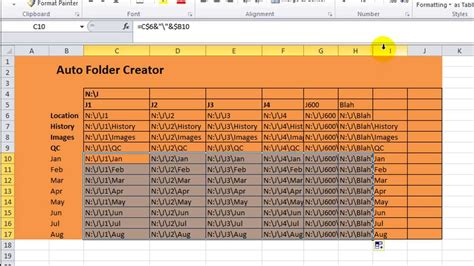
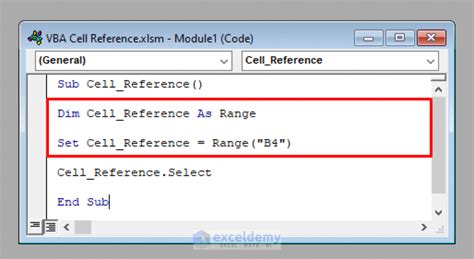
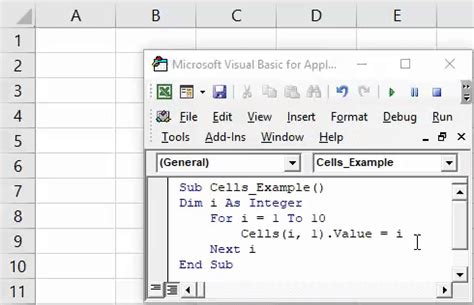
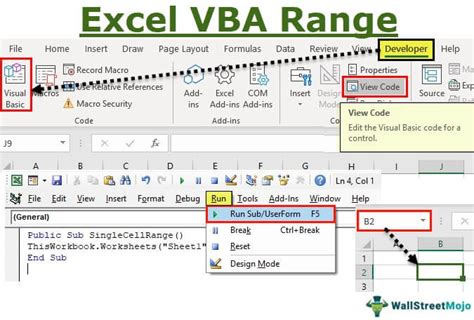
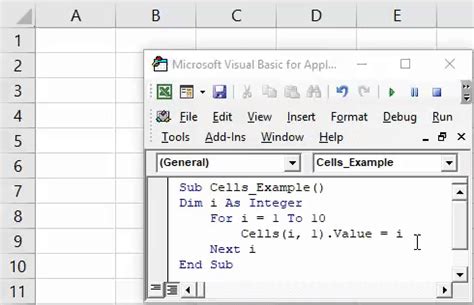
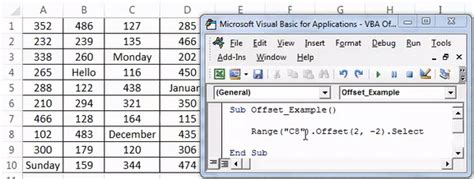
What is the difference between the Range object and the Cells property in VBA?
+The Range object and the Cells property are both used to reference cells in VBA, but they have different syntax and use cases. The Range object is more versatile and allows you to reference a single cell or a range of cells using the Range("A1") or Range("A1:B2") syntax. The Cells property, on the other hand, allows you to reference cells based on their row and column numbers, such as Cells(1, 1) for the cell at row 1, column 1.
How do I reference a cell in a different worksheet or workbook in VBA?
+To reference a cell in a different worksheet or workbook, you need to specify the worksheet or workbook name in your code. For example, to reference a cell in a worksheet named "Sheet2", you can use the syntax Range("Sheet2!A1"). To reference a cell in a different workbook, you need to open the workbook and specify the workbook name in your code, such as Workbooks("Workbook2.xlsx").Worksheets("Sheet1").Range("A1").
What are some common errors to watch out for when referencing cells in VBA?
+Some common errors to watch out for when referencing cells in VBA include referencing a cell that doesn't exist, using an incorrect range address or cell reference, forgetting to specify the worksheet or workbook, and using Select and Activate methods, which can cause errors and slow down your code.
In conclusion, mastering cell referencing in VBA is a crucial skill for any Excel power user or developer. By understanding the different methods available, following best practices, and avoiding common errors, you can create efficient, readable, and maintainable code that automates tasks and improves productivity. We hope this article has provided you with a comprehensive understanding of cell referencing in VBA and has inspired you to explore the world of Excel automation. If you have any questions or comments, please don't hesitate to reach out. Share this article with your colleagues and friends who may benefit from learning about VBA cell referencing.