Intro
Remove non-numeric characters with 5 easy methods, including regex, formatting, and parsing techniques to clean numeric data, ensuring accurate numeric extraction and data validation for seamless processing and analysis.
Removing non-numeric characters from a string can be a common task in various programming and data processing contexts. This operation is crucial for ensuring that only numbers are processed, analyzed, or stored, which can be particularly important in applications such as data cleaning, numerical computations, and input validation. Here, we'll explore five ways to remove non-numeric characters, focusing on approaches that can be applied in different programming environments.
The importance of removing non-numeric characters cannot be overstated. In many applications, especially those involving numerical analysis or database operations, the presence of non-numeric characters can lead to errors, incorrect results, or system crashes. For instance, in a database, ensuring that a field meant to hold numerical values does indeed only contain numbers is vital for maintaining data integrity and facilitating accurate queries and analyses.
Moreover, in programming, when dealing with user input or data from external sources, it's common to encounter strings that contain a mix of numeric and non-numeric characters. Cleaning such data by removing the non-numeric parts is a prerequisite for many subsequent operations, such as calculations, sorting, or storing the data in a structured format.
Given the versatility required in handling different types of data and the variety of programming environments, it's beneficial to have multiple strategies for removing non-numeric characters. These strategies can range from using regular expressions, which offer a powerful and flexible way to match patterns in strings, to leveraging built-in string manipulation functions available in most programming languages.
Introduction to Removing Non-Numeric Characters
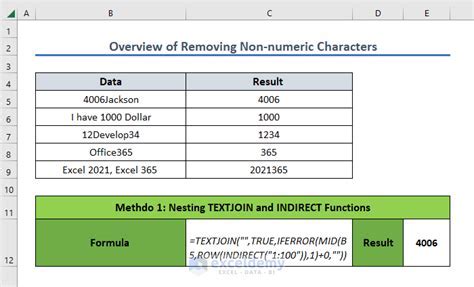
Removing non-numeric characters is a fundamental operation that involves filtering out all characters in a string that are not numbers. This can be achieved through various methods, including the use of regular expressions, loops that iterate over each character in the string, and built-in functions provided by programming languages for string manipulation.
Method 1: Using Regular Expressions
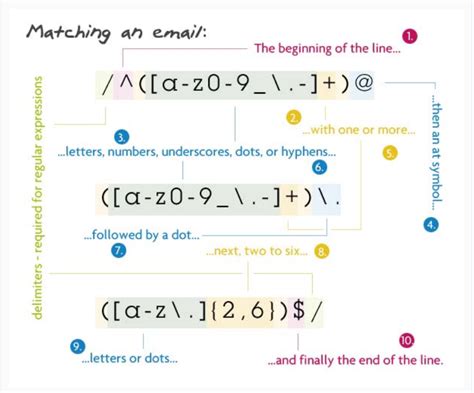
Regular expressions (regex) are a powerful tool for matching patterns in strings, making them ideal for removing non-numeric characters. The pattern \D
matches any non-numeric character, and by using the replace
method with this pattern, you can effectively remove all non-numeric characters from a string.
Here's an example in JavaScript:
let str = "abc123def456";
let numericStr = str.replace(/\D/g, '');
console.log(numericStr); // Outputs: "123456"
Method 2: Looping Through Characters
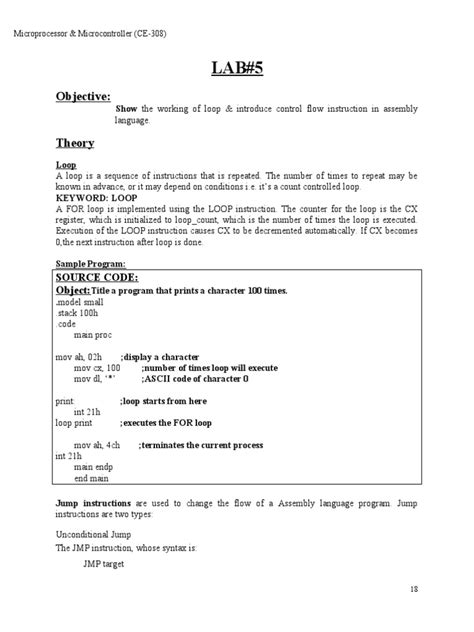
Another approach is to manually loop through each character in the string and check if it's a number. If it is, you append it to a new string. This method, although more verbose than using regex, provides a clear understanding of the process and can be implemented in any programming language.
Example in Python:
def remove_non_numeric(s):
result = ""
for char in s:
if char.isdigit():
result += char
return result
print(remove_non_numeric("hello123world456")) # Outputs: "123456"
Method 3: Using Built-in String Functions
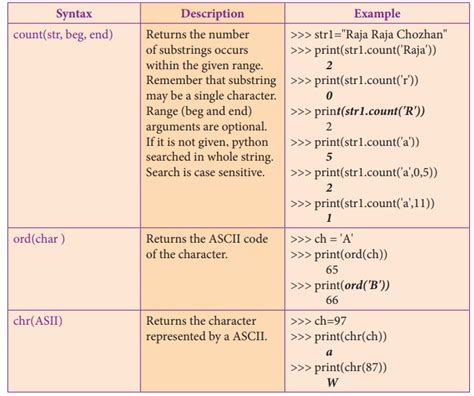
Many programming languages offer built-in functions or methods that can be used to filter out non-numeric characters. For example, in Python, you can use a list comprehension with the isdigit
method to achieve this.
Example:
s = "abc123def456"
numeric_s = "".join([c for c in s if c.isdigit()])
print(numeric_s) # Outputs: "123456"
Method 4: Using Filter Function

The filter
function in Python can also be used in combination with a lambda function to remove non-numeric characters.
Example:
s = "hello123world456"
numeric_s = "".join(filter(lambda x: x.isdigit(), s))
print(numeric_s) # Outputs: "123456"
Method 5: Using Isnumeric() Method
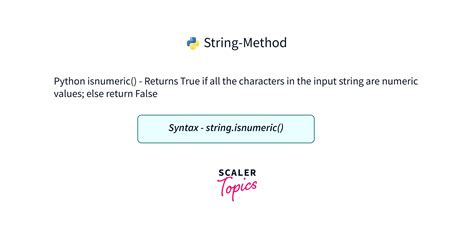
Lastly, Python's isnumeric()
method can be utilized within a loop or a list comprehension to filter out characters that are not numeric.
Example:
s = "abc123def456"
numeric_s = "".join(c for c in s if c.isnumeric())
print(numeric_s) # Outputs: "123456"
Gallery of Removing Non-Numeric Characters
Removing Non-Numeric Characters Image Gallery
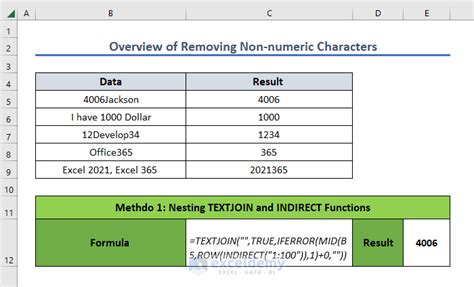
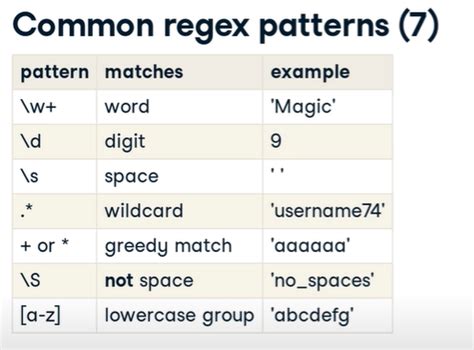

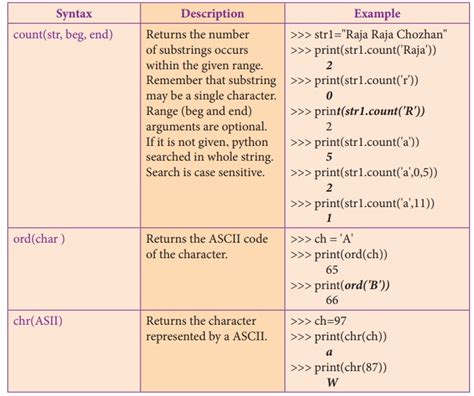
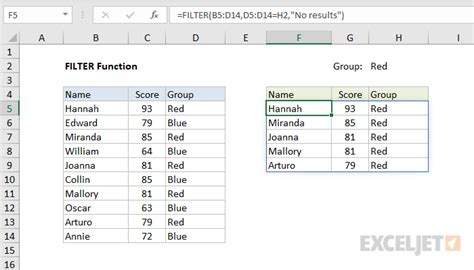
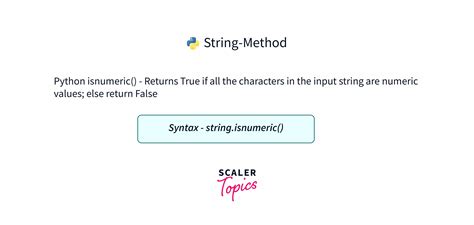
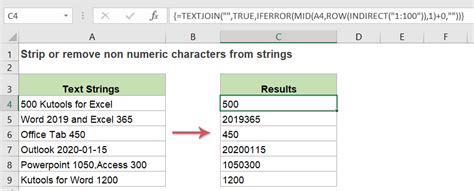
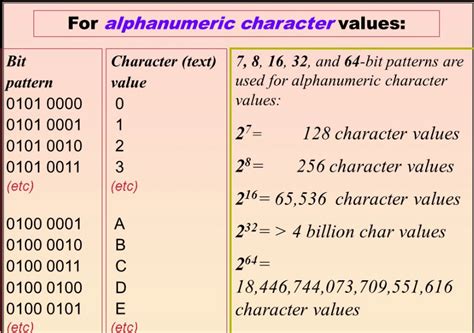
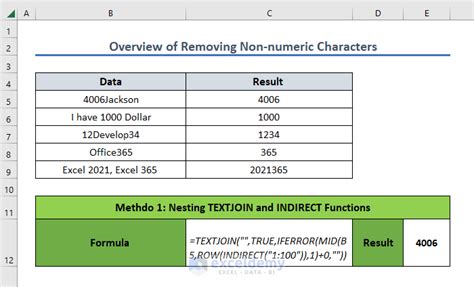
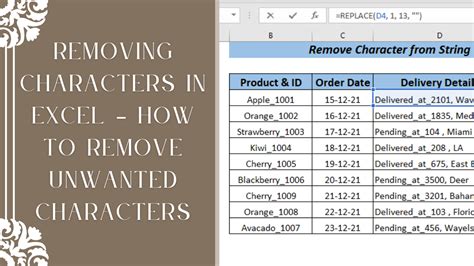
What are non-numeric characters?
+Non-numeric characters are any characters that are not numbers, including letters, symbols, and whitespace.
Why is it important to remove non-numeric characters?
+Removing non-numeric characters is crucial for ensuring data integrity, especially in numerical computations, data analysis, and input validation.
How can I remove non-numeric characters from a string in Python?
+You can use regular expressions, loop through characters and check if they are digits, use built-in string functions, the filter function, or the isnumeric method.
In conclusion, removing non-numeric characters from strings is a fundamental task that can be accomplished through various methods, each with its own advantages and use cases. Whether you're working with user input, processing data for analysis, or ensuring the integrity of numerical fields in a database, being able to efficiently remove non-numeric characters is a valuable skill. By understanding and applying the methods outlined above, you can more effectively handle and manipulate string data in your programming projects. If you have any questions or would like to discuss further the best approach for your specific scenario, please don't hesitate to comment or share this article with others who might find it useful.