Intro
Master Excel VBA with 5 ways to select sheets, using macros, worksheets, and ranges, to automate tasks and improve workflow efficiency with Visual Basic for Applications.
In the world of Excel, Visual Basic for Applications (VBA) is a powerful tool that allows users to automate tasks, create custom interfaces, and interact with Excel sheets in a more dynamic way. One of the fundamental actions in VBA is selecting a sheet, which can be crucial for performing operations on specific data sets or for presenting information to the user. The process of selecting a sheet in Excel VBA can be accomplished in several ways, each with its own set of advantages and use cases. Here, we'll delve into five methods to select a sheet using Excel VBA, exploring their syntax, practical applications, and examples.
Introduction to Excel VBA
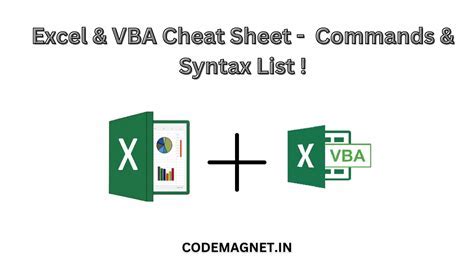
Before diving into the methods of selecting a sheet, it's essential to understand the basics of Excel VBA. VBA is a programming language developed by Microsoft, designed to be used for creating and automating tasks in Microsoft Office applications, including Excel. It allows users to create macros, which are sequences of instructions that can be executed with a single command, making repetitive tasks easier and faster.
Method 1: Using the Sheets Collection
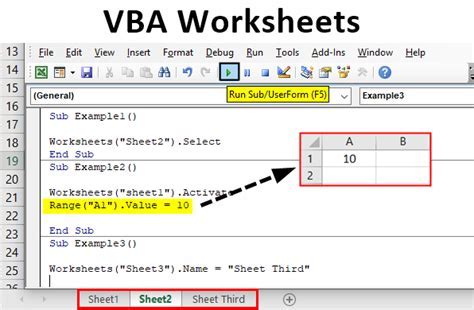
The Sheets collection in Excel VBA refers to all the sheets in a workbook. You can select a sheet by its index number or its name. Here's how you can do it:
- By Index:
Sheets(1).Select
- This selects the first sheet in the workbook. - By Name:
Sheets("Sheet1").Select
- This selects the sheet named "Sheet1".
This method is straightforward and useful when you know the exact name or position of the sheet you want to select.
Example Usage
Sub SelectSheetByName()
Sheets("Sheet1").Select
End Sub
Sub SelectSheetByIndex()
Sheets(1).Select
End Sub
Method 2: Using the Worksheets Collection
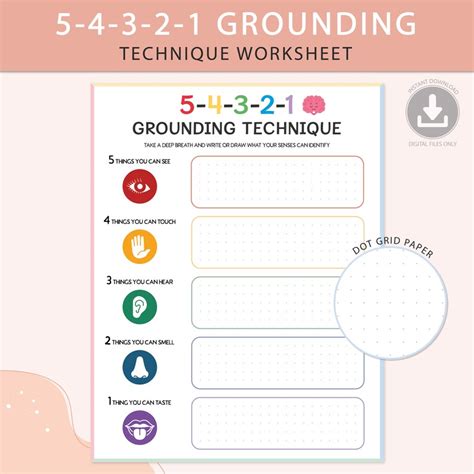
Similar to the Sheets collection, the Worksheets collection can be used to select worksheets. However, this collection only includes worksheets and does not include chart sheets. The syntax is similar:
- By Index:
Worksheets(1).Select
- By Name:
Worksheets("Sheet1").Select
This method is preferred when you are certain you are working with worksheets and want to exclude chart sheets.
Example Usage
Sub SelectWorksheetByName()
Worksheets("Sheet1").Select
End Sub
Sub SelectWorksheetByIndex()
Worksheets(1).Select
End Sub
Method 3: Using the ActiveWorkbook Object
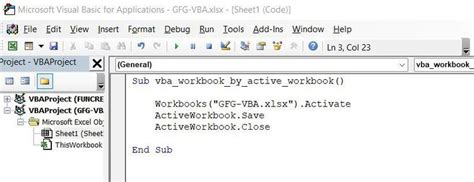
The ActiveWorkbook object refers to the workbook that is currently active. You can use this object to select a sheet:
ActiveWorkbook.Sheets("Sheet1").Select
Or,
ActiveWorkbook.Worksheets("Sheet1").Select
This method is useful when you're working with multiple workbooks and want to ensure you're selecting a sheet from the currently active workbook.
Example Usage
Sub SelectSheetInActiveWorkbook()
ActiveWorkbook.Sheets("Sheet1").Select
End Sub
Method 4: Looping Through Sheets
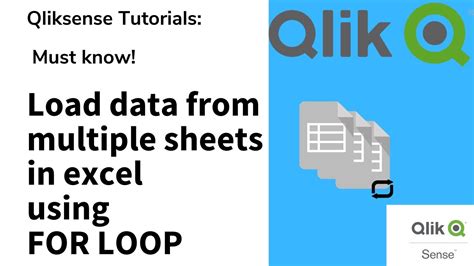
Sometimes, you might need to perform an action on every sheet in a workbook or select a sheet based on certain conditions. You can loop through all sheets using a For Each loop:
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Select
' Perform actions here
Next ws
This method is particularly useful for batch processing tasks across multiple sheets.
Example Usage
Sub LoopThroughSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Select
MsgBox ws.Name
Next ws
End Sub
Method 5: Selecting a Sheet Without Activating It
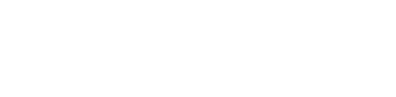
In some scenarios, you might want to select a range or perform an operation on a sheet without actually activating it. This can be achieved by directly referencing the sheet and range:
ThisWorkbook.Sheets("Sheet1").Range("A1").Select
However, selecting a range on a sheet that is not active can be tricky and might not work as expected in all situations. It's often more reliable to activate the sheet first.
Example Usage
Sub SelectRangeOnSheet()
ThisWorkbook.Sheets("Sheet1").Activate
ThisWorkbook.Sheets("Sheet1").Range("A1").Select
End Sub
Gallery of Excel VBA Select Sheet Methods
Excel VBA Select Sheet Methods
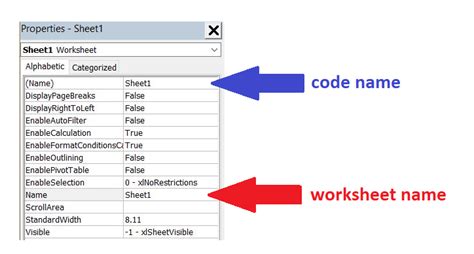
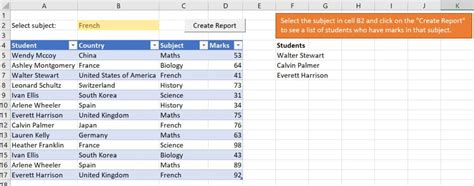
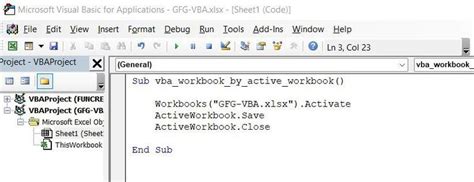
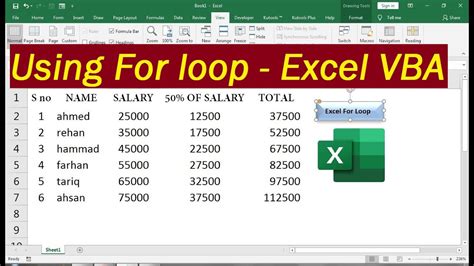
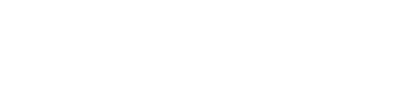
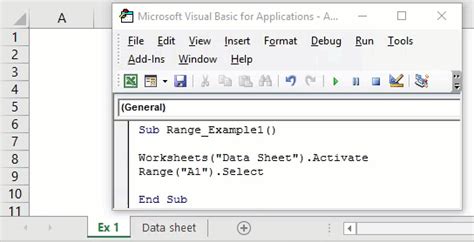
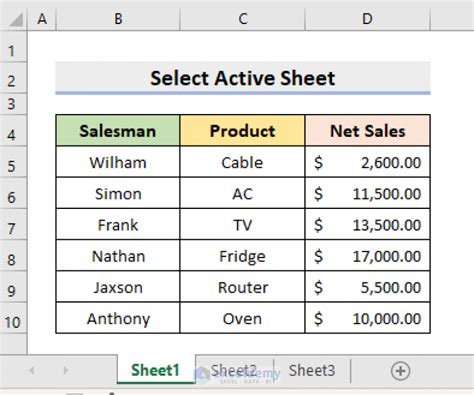
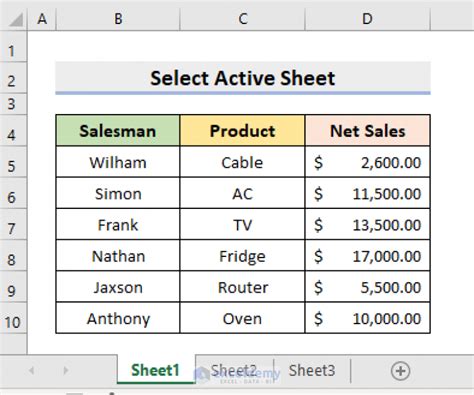
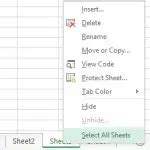

What is the difference between the Sheets and Worksheets collections in Excel VBA?
+The Sheets collection includes all types of sheets in a workbook, such as worksheets and chart sheets. The Worksheets collection, on the other hand, only includes worksheets, excluding chart sheets.
How do I select a sheet in Excel VBA without activating it?
+You can select a range on a sheet without activating it by directly referencing the sheet and range, such as `ThisWorkbook.Sheets("Sheet1").Range("A1").Select`. However, this might not work as expected in all situations, and it's often more reliable to activate the sheet first.
Can I loop through all sheets in a workbook using Excel VBA?
+Yes, you can loop through all sheets in a workbook using a For Each loop, such as `For Each ws In ThisWorkbook.Worksheets`. This allows you to perform actions on every sheet or select a sheet based on certain conditions.
To summarize, selecting a sheet in Excel VBA can be accomplished through various methods, each with its own advantages and use cases. Whether you're using the Sheets collection, the Worksheets collection, the ActiveWorkbook object, looping through sheets, or selecting a sheet without activating it, understanding these methods can help you automate tasks and interact with Excel sheets more efficiently. We invite you to explore these methods further, practice using them in your VBA projects, and share your experiences or questions in the comments below.