Intro
Master Setting Range in VBA with ease. Learn range objects, cell references, and worksheet interactions using Visual Basic for Applications, Excel VBA programming, and macros.
Setting a range in VBA is a fundamental skill that is essential for any Excel automation task. Ranges in VBA refer to a selection of cells or a group of cells that you want to perform an action on. In this article, we will explore the different ways to set a range in VBA, including using the Range
object, Cells
property, and Union
method.
When working with ranges in VBA, it's essential to understand the different types of ranges that you can work with. You can set a range to a single cell, a group of cells, an entire row or column, or even a non-contiguous range of cells. Each type of range has its own unique characteristics and uses, and understanding these differences is crucial for writing effective VBA code.
One of the most common ways to set a range in VBA is by using the Range
object. The Range
object is a built-in VBA object that allows you to select a range of cells. You can use the Range
object to set a range to a single cell, a group of cells, or an entire row or column. For example, to set a range to the cell A1, you can use the following code: Dim myRange As Range: Set myRange = Range("A1")
.
Using the Range Object
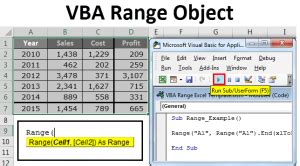
Another way to set a range in VBA is by using the Cells
property. The Cells
property allows you to select a range of cells based on their row and column numbers. For example, to set a range to the cell A1, you can use the following code: Dim myRange As Range: Set myRange = Cells(1, 1)
. The Cells
property is useful when you need to work with ranges that are based on specific row and column numbers.
Using the Cells Property

In addition to the Range
object and Cells
property, you can also use the Union
method to set a range in VBA. The Union
method allows you to combine multiple ranges into a single range. For example, to set a range to the cells A1 and B2, you can use the following code: Dim myRange As Range: Set myRange = Union(Range("A1"), Range("B2"))
. The Union
method is useful when you need to work with non-contiguous ranges of cells.
Using the Union Method
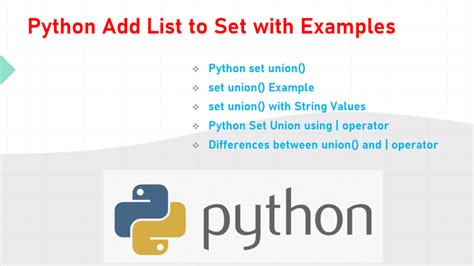
When setting a range in VBA, it's essential to consider the different types of ranges that you can work with. You can set a range to a single cell, a group of cells, an entire row or column, or even a non-contiguous range of cells. Each type of range has its own unique characteristics and uses, and understanding these differences is crucial for writing effective VBA code.
Types of Ranges
There are several types of ranges that you can work with in VBA, including: * Single cell range: A range that consists of a single cell. * Multiple cell range: A range that consists of multiple cells. * Row range: A range that consists of an entire row of cells. * Column range: A range that consists of an entire column of cells. * Non-contiguous range: A range that consists of multiple ranges that are not adjacent to each other.Setting a Range to a Single Cell
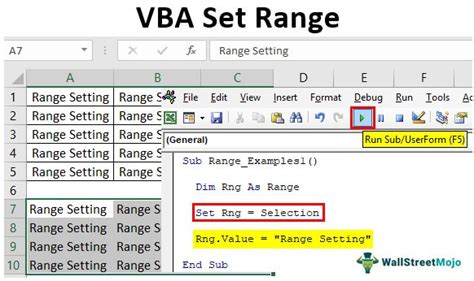
To set a range to a single cell, you can use the Range
object or the Cells
property. For example, to set a range to the cell A1, you can use the following code: Dim myRange As Range: Set myRange = Range("A1")
. Alternatively, you can use the Cells
property to set a range to the cell A1: Dim myRange As Range: Set myRange = Cells(1, 1)
.
Setting a Range to a Group of Cells
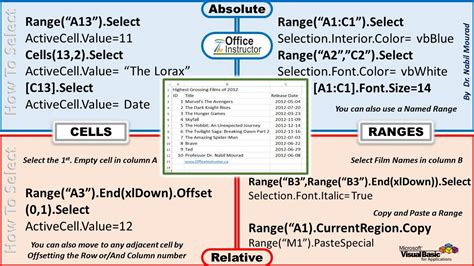
To set a range to a group of cells, you can use the Range
object or the Cells
property. For example, to set a range to the cells A1:B2, you can use the following code: Dim myRange As Range: Set myRange = Range("A1:B2")
. Alternatively, you can use the Cells
property to set a range to the cells A1:B2: Dim myRange As Range: Set myRange = Range(Cells(1, 1), Cells(2, 2))
.
Setting a Range to an Entire Row or Column
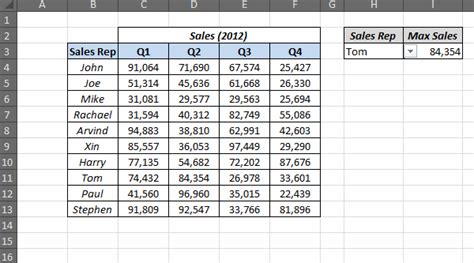
To set a range to an entire row or column, you can use the Rows
or Columns
property. For example, to set a range to the entire first row, you can use the following code: Dim myRange As Range: Set myRange = Rows(1)
. Alternatively, you can use the Columns
property to set a range to the entire first column: Dim myRange As Range: Set myRange = Columns(1)
.
Setting a Range to a Non-Contiguous Range of Cells
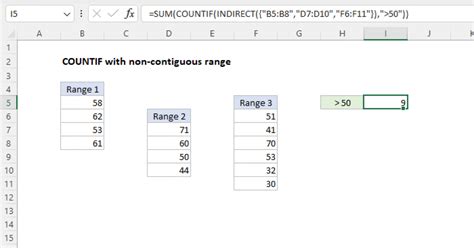
To set a range to a non-contiguous range of cells, you can use the Union
method. For example, to set a range to the cells A1 and B2, you can use the following code: Dim myRange As Range: Set myRange = Union(Range("A1"), Range("B2"))
.
Setting Range in VBA Image Gallery

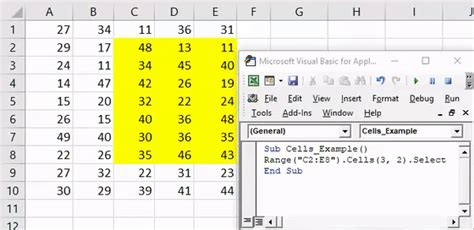
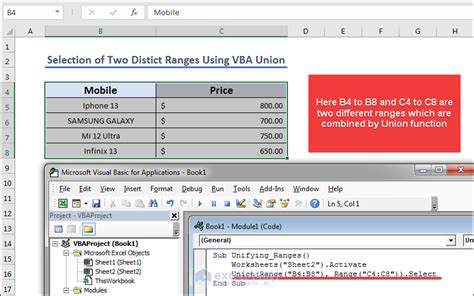
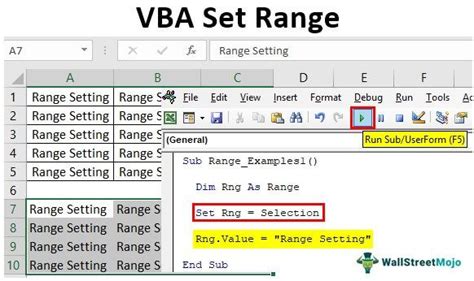
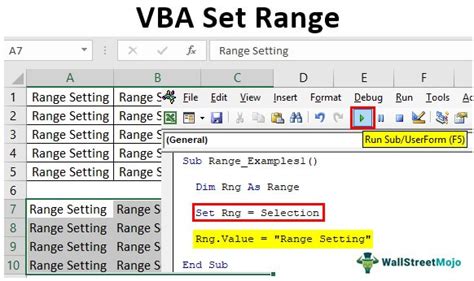
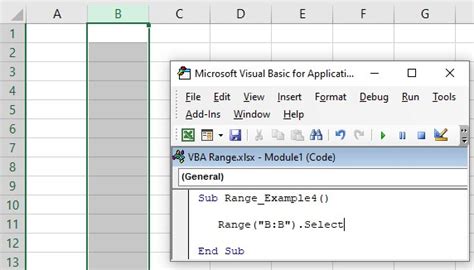

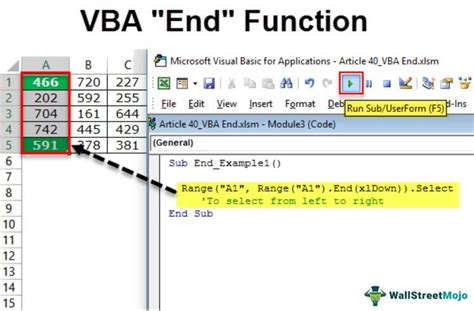
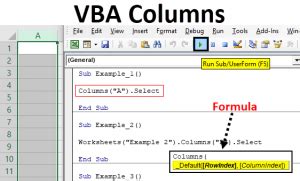
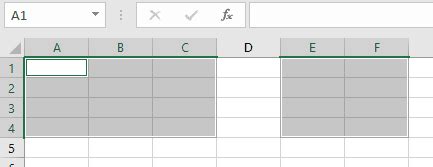
What is the purpose of setting a range in VBA?
+The purpose of setting a range in VBA is to select a group of cells or a single cell that you want to perform an action on.
How do I set a range to a single cell in VBA?
+To set a range to a single cell in VBA, you can use the `Range` object or the `Cells` property. For example, to set a range to the cell A1, you can use the following code: `Dim myRange As Range: Set myRange = Range("A1")`.
How do I set a range to a group of cells in VBA?
+To set a range to a group of cells in VBA, you can use the `Range` object or the `Cells` property. For example, to set a range to the cells A1:B2, you can use the following code: `Dim myRange As Range: Set myRange = Range("A1:B2")`.
What is the difference between the `Range` object and the `Cells` property in VBA?
+The `Range` object and the `Cells` property are both used to select a range of cells in VBA. However, the `Range` object is more flexible and can be used to select a range of cells based on their address, while the `Cells` property is used to select a range of cells based on their row and column numbers.
How do I set a range to an entire row or column in VBA?
+To set a range to an entire row or column in VBA, you can use the `Rows` or `Columns` property. For example, to set a range to the entire first row, you can use the following code: `Dim myRange As Range: Set myRange = Rows(1)`.
Setting a range in VBA is a fundamental skill that is essential for any Excel automation task. By understanding the different ways to set a range, including using the Range
object, Cells
property, and Union
method, you can write more effective VBA code and automate tasks with ease. Whether you're working with single cells, groups of cells, or entire rows and columns, setting a range in VBA is a crucial step in achieving your automation goals. We hope this article has provided you with a comprehensive understanding of how to set a range in VBA and has given you the confidence to start automating your Excel tasks today. If you have any questions or need further assistance, please don't hesitate to comment below.