Intro
Discover 5 ways VBA send value efficiently, leveraging macros, Excel automation, and data transfer techniques to streamline workflows and boost productivity with Visual Basic for Applications.
Visual Basic for Applications (VBA) is a powerful tool used for creating and automating tasks in Microsoft Office applications, particularly in Excel. One of the fundamental operations in VBA is sending values from one place to another, whether it's to a worksheet, a user form, or even an external application. There are several ways to achieve this, each with its own set of advantages and scenarios where they are most appropriately used. Below, we'll explore five ways to send values using VBA, along with examples and explanations to help you understand how and when to use each method.
Firstly, let's consider the importance of VBA in automating tasks and enhancing productivity. By leveraging VBA, users can create customized solutions that streamline processes, reduce manual errors, and improve overall efficiency. Whether you're a beginner or an advanced user, understanding how to send values using VBA is a crucial skill that can significantly enhance your ability to work with Microsoft Office applications.
Introduction to VBA and Value Transfer
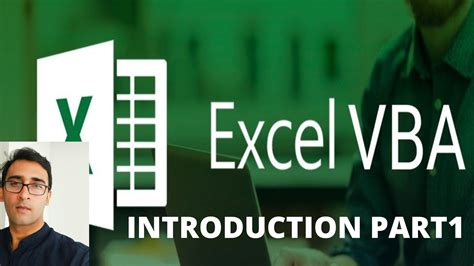
Before diving into the methods of sending values, it's essential to have a basic understanding of VBA and its environment. VBA stands for Visual Basic for Applications, and it's used to create and automate tasks within Microsoft Office applications. The VBA editor is where you write your code, and it provides a comprehensive set of tools and features to help you develop, debug, and execute your VBA scripts.
1. Using the Range Object
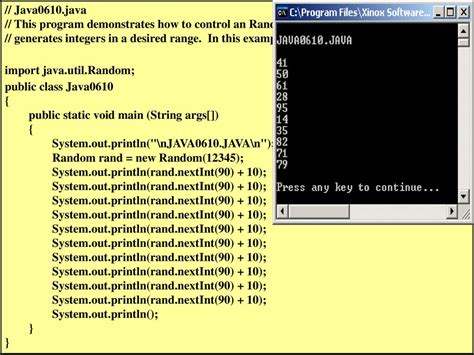
One of the most common ways to send values in VBA is by using the Range object. This object represents a cell or a range of cells in a worksheet. You can assign a value to a Range object directly, which then updates the corresponding cell(s) in the worksheet.
Sub SendValueUsingRange()
Range("A1").Value = "Hello, World!"
End Sub
This example demonstrates how to send the string "Hello, World!" to cell A1 in the active worksheet.
2. Using Variables
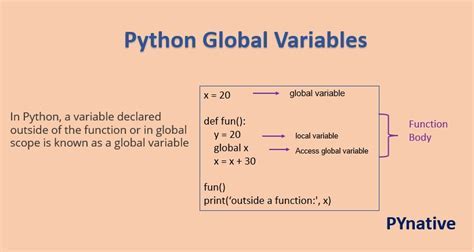
Variables are another fundamental way to send values in VBA. By declaring variables, you can store and manipulate data before assigning it to a specific location, such as a worksheet cell or a control on a user form.
Sub SendValueUsingVariable()
Dim myValue As String
myValue = "Hello, again!"
Range("B1").Value = myValue
End Sub
In this example, a variable named myValue
is declared and assigned the string "Hello, again!". This value is then sent to cell B1.
3. Using User Forms
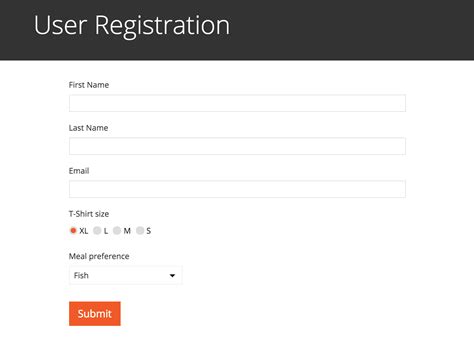
User forms in VBA provide a graphical user interface (GUI) that allows users to interact with your application. You can send values from a user form to a worksheet or use them within the form itself for calculations or other operations.
Sub SendValueFromUserForm()
Dim frm As New UserForm1
frm.Show
' Assuming UserForm1 has a TextBox named TextBox1
Range("C1").Value = frm.TextBox1.Value
End Sub
This example shows how to display a user form and then send the value from a text box on the form to cell C1.
4. Using Arrays
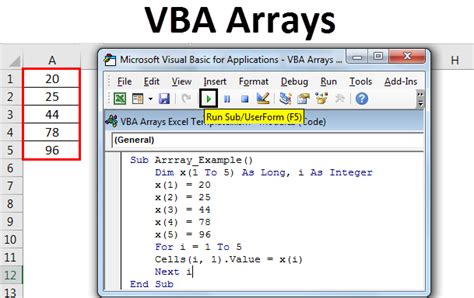
Arrays are useful when you need to send multiple values at once. You can store several values in an array and then write this array to a range of cells in a worksheet.
Sub SendValuesUsingArray()
Dim myArray(1 To 3) As Variant
myArray(1) = "Value 1"
myArray(2) = "Value 2"
myArray(3) = "Value 3"
Range("D1:D3").Value = Application.Transpose(myArray)
End Sub
In this example, an array myArray
is populated with three values, which are then sent to cells D1 through D3.
5. Using Loops
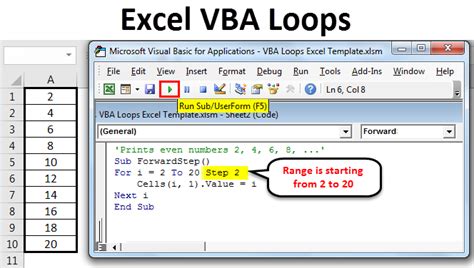
Loops are essential in VBA for repetitive tasks, including sending values to multiple locations. You can use a loop to iterate over a range of cells or an array and send values accordingly.
Sub SendValuesUsingLoop()
Dim i As Integer
For i = 1 To 5
Cells(i, 5).Value = "Value " & i
Next i
End Sub
This example demonstrates a loop that sends values to cells E1 through E5, with each value being "Value " followed by the loop counter.
Gallery of VBA Examples
VBA Examples Image Gallery
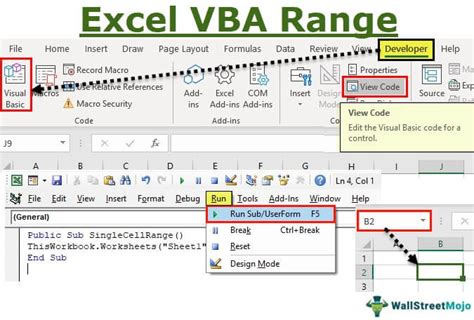
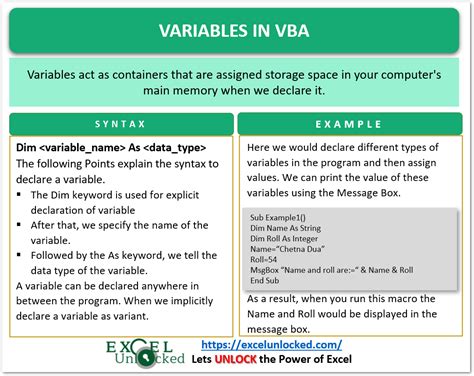
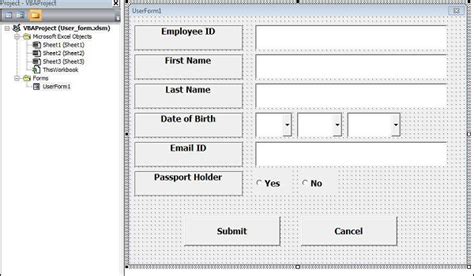
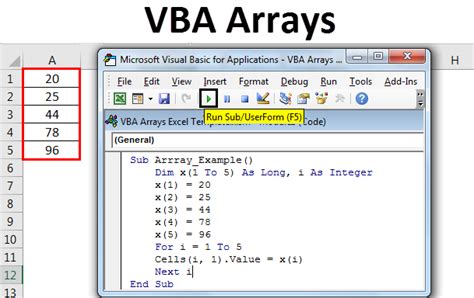
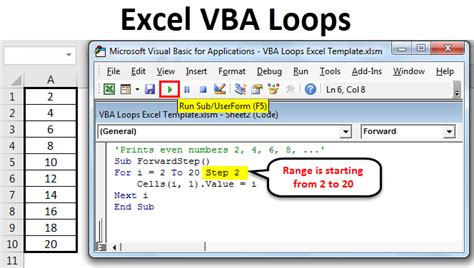
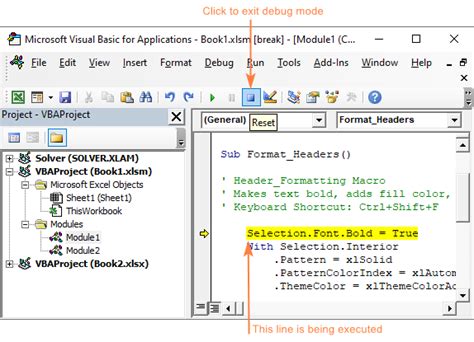
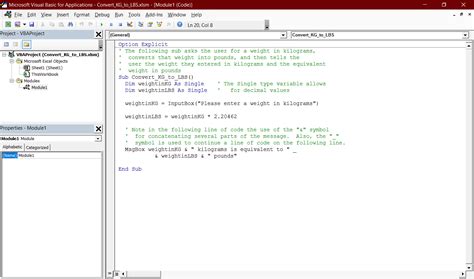
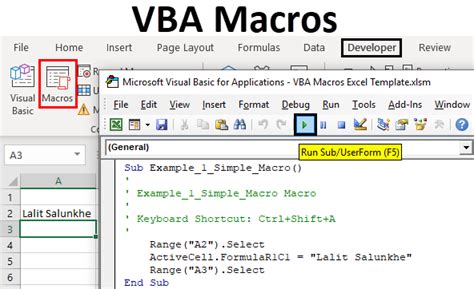
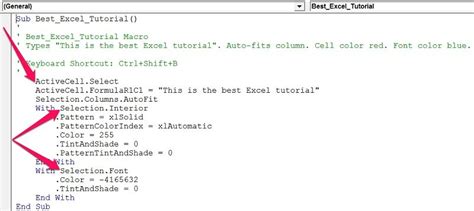
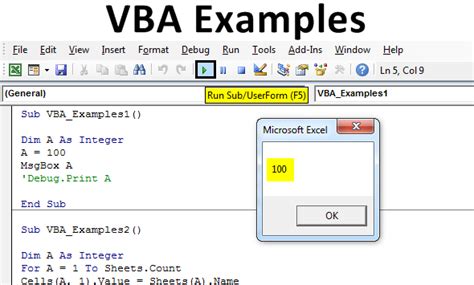
What is VBA used for in Microsoft Office applications?
+VBA is used for creating and automating tasks, enhancing productivity, and customizing the application's behavior to meet specific needs.
How do I send values from VBA to a worksheet?
+You can send values from VBA to a worksheet by using the Range object, variables, arrays, or loops, depending on the specific requirements of your task.
What are some common applications of VBA in real-world scenarios?
+VBA is commonly used in data analysis, automation of repetitive tasks, creating custom tools and interfaces, and integrating different applications and systems.
In conclusion, mastering the art of sending values in VBA is a powerful skill that can significantly enhance your productivity and capabilities when working with Microsoft Office applications. Whether you're automating tasks, creating custom interfaces, or performing complex data analyses, understanding how to effectively use VBA to send values is a fundamental aspect of achieving your goals. We invite you to share your experiences, ask questions, or provide feedback on this article, and we look forward to continuing the conversation on how to leverage VBA for maximum benefit.