Intro
Finding the last row in Excel using VBA is a common task, especially when working with dynamic data ranges. It's essential to accurately determine the last row to avoid errors or to ensure that your macros process all relevant data. Here's how you can do it:
When finding the last row, it's crucial to consider the specific column you're interested in, as the last row can vary across different columns in a worksheet. The most common approach is to find the last row in a specific column or across the entire worksheet.
Finding the Last Row in a Specific Column
To find the last row in a specific column, you can use the Cells.Find
method or the Range.End
method. Here's how you can implement these methods:
Using Cells.Find
Sub FindLastRowUsingFind()
Dim lastRow As Long
lastRow = Cells.Find(What:="*", SearchOrder:=xlRows, SearchDirection:=xlPrevious, LookIn:=xlValues).Row
' Alternatively, to search in a specific column, say column A
lastRow = Cells.Find(What:="*", SearchOrder:=xlByColumns, SearchDirection:=xlPrevious, LookIn:=xlValues, SearchFormat:=False).Row
MsgBox "Last Row: " & lastRow
End Sub
However, the above code might not work as expected if you're searching in a specific column and that column is empty. A more reliable way to search in a specific column is:
Sub FindLastRowInColumnA()
Dim lastRow As Long
lastRow = Cells(Rows.Count, "A").End(xlUp).Row
MsgBox "Last Row in Column A: " & lastRow
End Sub
Using Range.End
This method is straightforward and efficient for finding the last row in a specific column:
Sub FindLastRowUsingEnd()
Dim lastRow As Long
lastRow = Cells(Rows.Count, "A").End(xlUp).Row
MsgBox "Last Row in Column A: " & lastRow
End Sub
Finding the Last Row Across the Entire Worksheet
If you need to find the last row that contains any data across the entire worksheet, you can use the following approach:
Sub FindLastRowInWorksheet()
Dim lastRow As Long
lastRow = Cells.Find(What:="*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious).Row
MsgBox "Last Row in Worksheet: " & lastRow
End Sub
Or, if you prefer a method that doesn't rely on Find
:
Sub FindLastRowAlternative()
Dim lastRow As Long
If Application.WorksheetFunction.CountA(Cells) = 0 Then
lastRow = 1
Else
lastRow = Cells(Rows.Count, Columns.Count).End(xlUp).Row
End If
MsgBox "Last Row in Worksheet: " & lastRow
End Sub
Practical Advice
- Specific Column vs. Entire Worksheet: Always consider whether you need to find the last row in a specific column or across the entire worksheet. This determines which method you should use.
- Handling Empty Worksheets: If there's a chance the worksheet could be empty, include a check to handle this scenario and avoid errors.
- Performance: For very large datasets, some methods might be slower than others.
Range.End(xlUp)
is generally efficient and reliable.
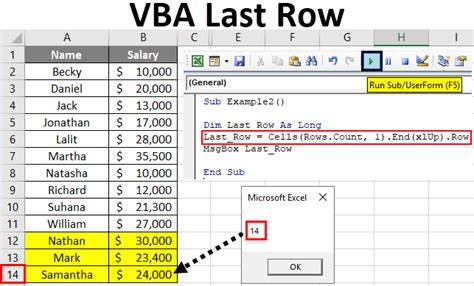
Example Use Cases
- Data Processing: When automating data processing tasks, finding the last row is crucial to ensure all data is included.
- Dynamic Charts: To create dynamic charts that update automatically when new data is added, finding the last row helps in setting the chart's data range.
- Automated Reporting: In automated reporting, accurately identifying the last row ensures that reports include all relevant data up to the last entry.
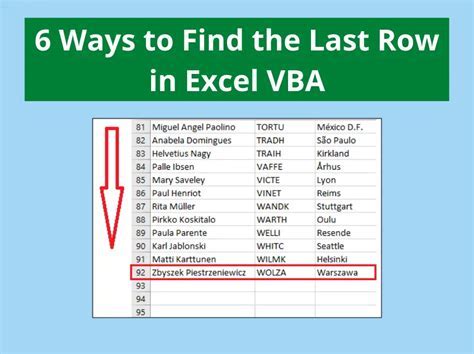
Gallery of Excel VBA Last Row Examples
Excel VBA Last Row Image Gallery
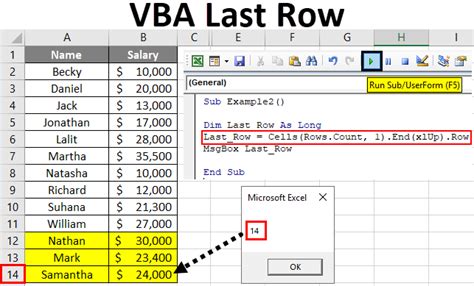
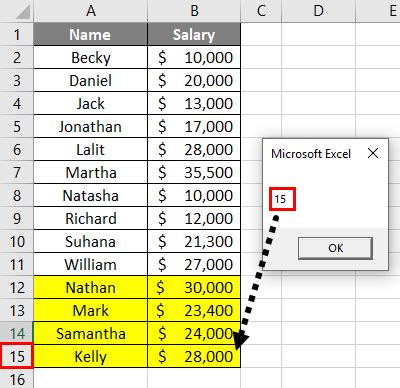
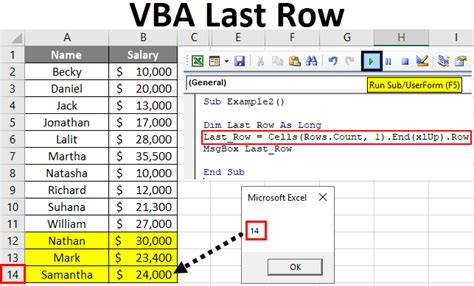
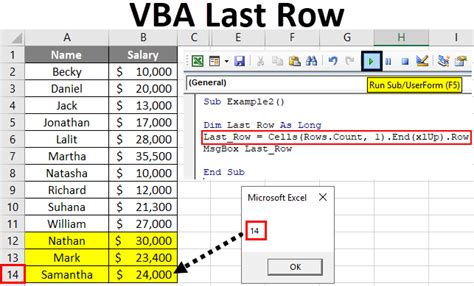
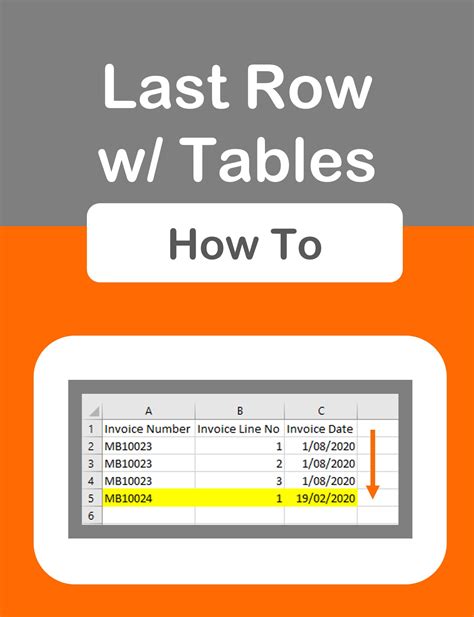
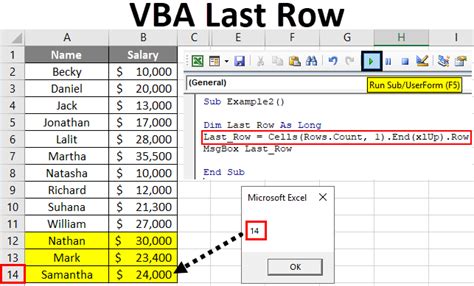
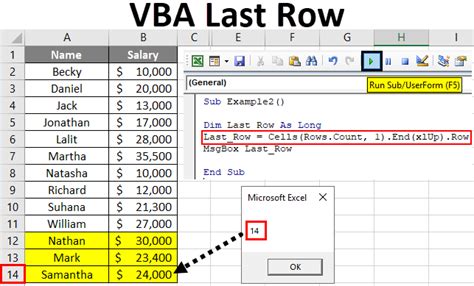
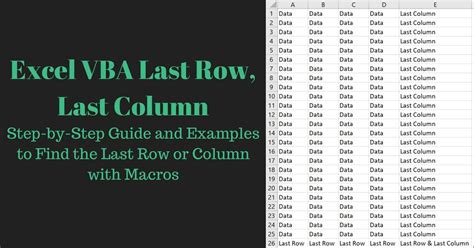
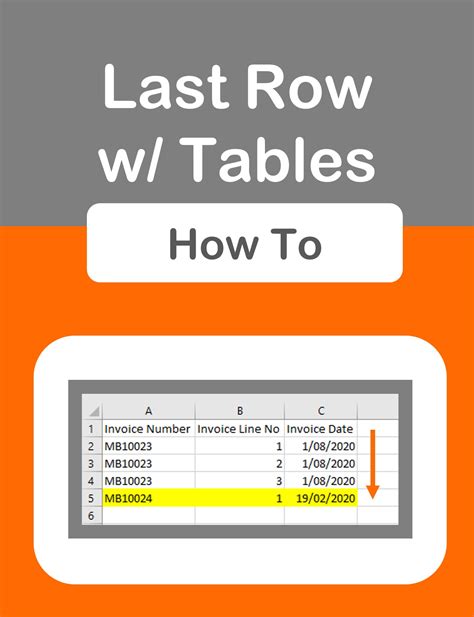
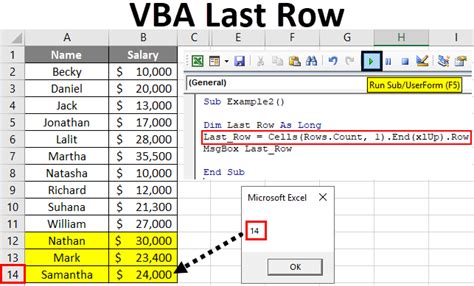
FAQs
What is the most efficient way to find the last row in Excel VBA?
+The most efficient way is often using `Cells(Rows.Count, "A").End(xlUp).Row` for a specific column or `Cells.Find` for the entire worksheet.
How do I handle an empty worksheet when finding the last row?
+Check if the worksheet is empty using `Application.WorksheetFunction.CountA(Cells) = 0`, and if so, set the last row to 1 or handle it as per your requirement.
Can I use these methods in any Excel version?
+Yes, these methods are compatible with most versions of Excel that support VBA, including Excel 2010, 2013, 2016, 2019, and Excel for Office 365.
Finding the last row in Excel VBA is a fundamental skill that can greatly enhance your ability to automate tasks and work efficiently with data in Excel. By mastering these techniques, you can create more robust and dynamic VBA applications that adapt to changing data environments. Whether you're processing data, creating reports, or automating tasks, accurately identifying the last row is a crucial step.
We hope this comprehensive guide has provided you with the insights and practical examples you need to find the last row in Excel VBA effectively. If you have any further questions or need more specific guidance, don't hesitate to reach out. Share your experiences or tips on finding the last row in Excel VBA in the comments below, and help build a community of Excel power users.