Intro
Pulling the first word of a sentence or a line of text can be useful in various text processing and analysis tasks. Here are 5 ways to achieve this using different programming languages and techniques:
The first method involves using regular expressions, which are powerful tools for matching patterns in text. By using a regular expression that matches the start of a string followed by one or more word characters, you can extract the first word from a sentence. This approach is versatile and can be applied in many programming languages that support regular expressions, such as Python, JavaScript, and Java.
Another approach is to use string splitting, where the sentence is split into words based on spaces or other delimiters. The first element of the resulting array or list will be the first word of the sentence. This method is straightforward and efficient, especially in languages like Python and JavaScript that have built-in support for string splitting.
For those working with databases or performing text analysis on large datasets, using SQL or dedicated text processing libraries like NLTK (Natural Language Toolkit) can be beneficial. SQL provides functions to manipulate strings, including extracting substrings, which can be used to pull the first word. NLTK, on the other hand, offers more sophisticated text processing capabilities, including tokenization, which can be used to identify and extract the first word of sentences.
In addition to these methods, using command-line tools like awk
or sed
can be very effective for text manipulation tasks, including extracting the first word from sentences. These tools are particularly useful when working with large text files or when you need to perform batch processing.
Lastly, for tasks that require a more manual or interactive approach, using spreadsheet software like Microsoft Excel or Google Sheets can be a good option. These applications provide functions to manipulate text within cells, including extracting parts of strings, which can be used to pull the first word from a sentence.
Introduction to Pulling First Words

Pulling the first word from a sentence is a fundamental task in text processing and analysis. It can be used in a variety of applications, from simple data cleaning to complex natural language processing tasks. Understanding the different methods available to achieve this can help in choosing the most appropriate approach based on the specific requirements of the task at hand.
Method 1: Using Regular Expressions
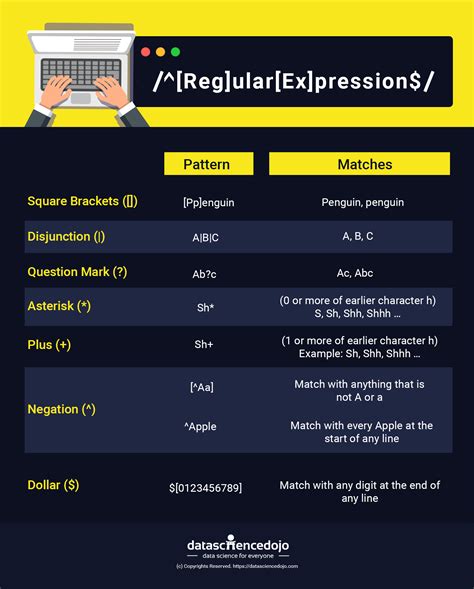
Regular expressions (regex) are a powerful tool for matching patterns in strings. They can be used to extract the first word from a sentence by matching the start of the string followed by one or more word characters. The pattern ^\w+
is commonly used for this purpose, where ^
denotes the start of the string, \w
matches any word character (equivalent to [a-zA-Z0-9_]
), and +
indicates one or more occurrences.
Here are some examples of how to use regular expressions in different programming languages to extract the first word:
- Python: Using the
re
module, you can extract the first word like this:import re; sentence = "Hello world"; first_word = re.match(r'\w+', sentence).group(); print(first_word)
- JavaScript: The
match()
method of the String object can be used with regex to find the first word:let sentence = "Hello world"; let firstWord = sentence.match(/^\w+/)[0]; console.log(firstWord);
Method 2: String Splitting
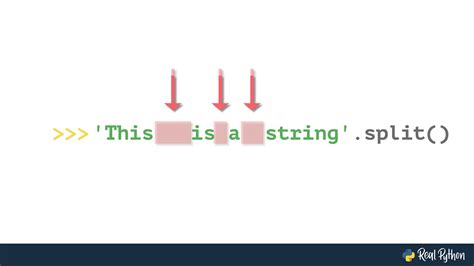
String splitting involves dividing a string into an array of substrings based on a specified delimiter. To extract the first word from a sentence, you can split the sentence into words using spaces as the delimiter and then take the first element of the resulting array.
Examples include:
- Python:
sentence = "Hello world"; words = sentence.split(); first_word = words[0]; print(first_word)
- JavaScript:
let sentence = "Hello world"; let words = sentence.split(' '); let firstWord = words[0]; console.log(firstWord);
Method 3: Using SQL
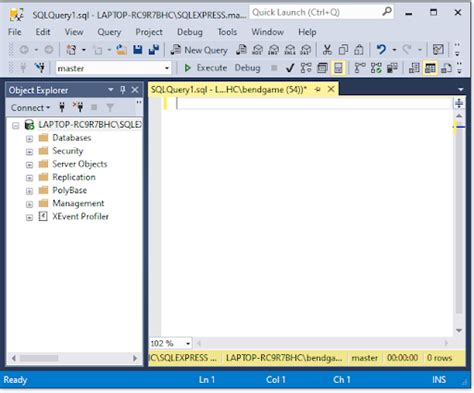
In SQL, you can use string functions to extract the first word from a sentence. The exact function may vary depending on the SQL dialect you are using. For example, in MySQL, you can use the SUBSTRING_INDEX
function to achieve this.
SELECT SUBSTRING_INDEX('Hello world', ' ', 1) AS first_word;
This will return Hello
, which is the first word of the sentence.
Method 4: Using NLTK
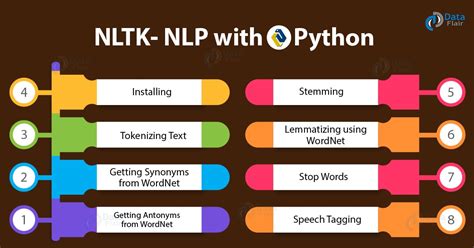
The Natural Language Toolkit (NLTK) is a comprehensive library of NLP tasks. It includes tools for tokenization, which is the process of breaking down text into words or tokens. You can use NLTK to extract the first word from a sentence by tokenizing the sentence and then selecting the first token.
import nltk
nltk.download('punkt') # Download the Punkt tokenizer models
sentence = "Hello world"
tokens = nltk.word_tokenize(sentence)
first_word = tokens[0]
print(first_word)
Method 5: Using Command-Line Tools
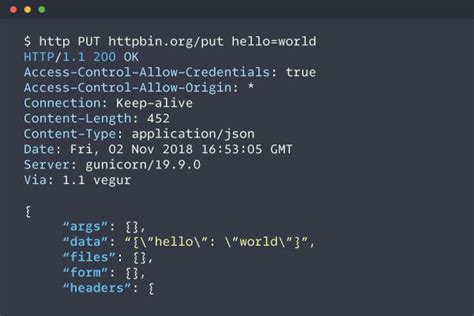
Command-line tools like awk
and sed
are very powerful for text manipulation tasks. They can be used to extract the first word from a sentence.
- Awk:
echo "Hello world" | awk '{print $1}'
- Sed:
echo "Hello world" | sed 's/.*//g'
These commands will output Hello
, which is the first word of the sentence.
Gallery of Text Processing Techniques
Text Processing Techniques Gallery
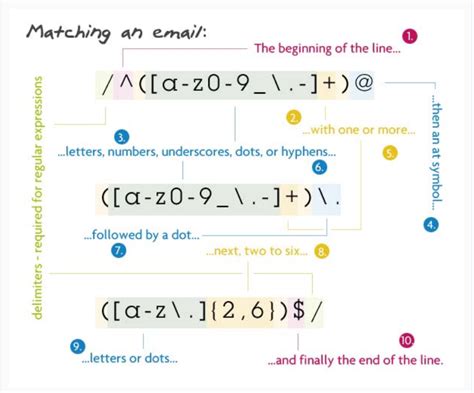
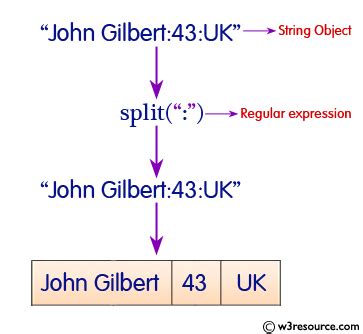
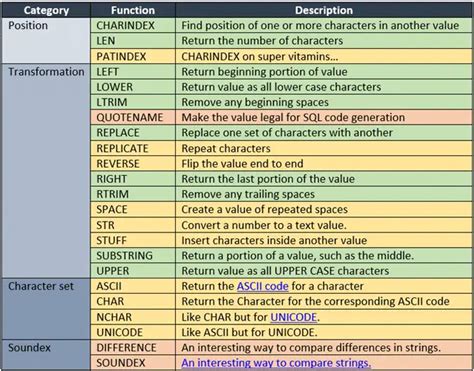
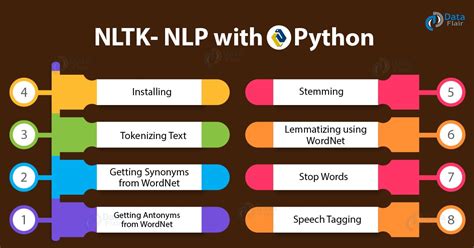
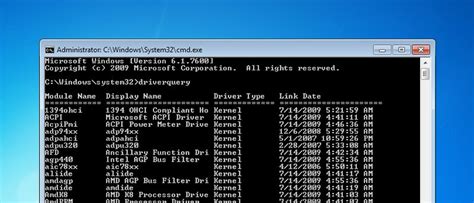
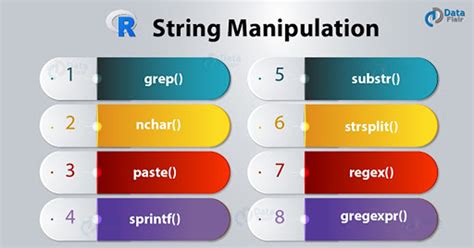
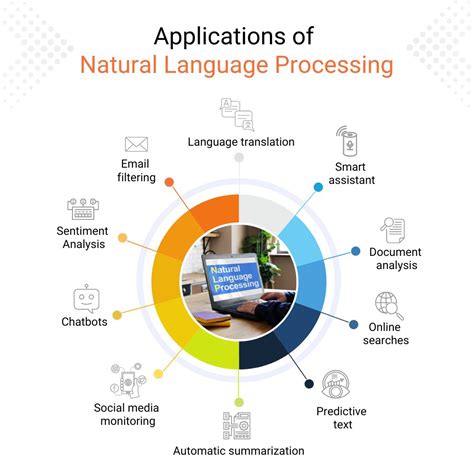
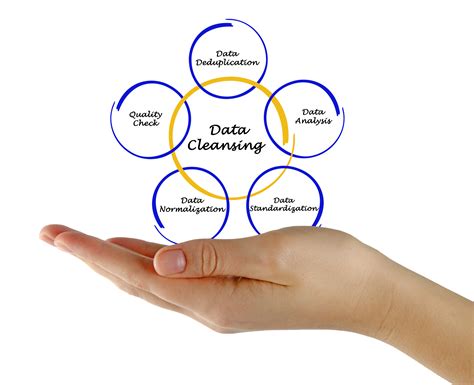
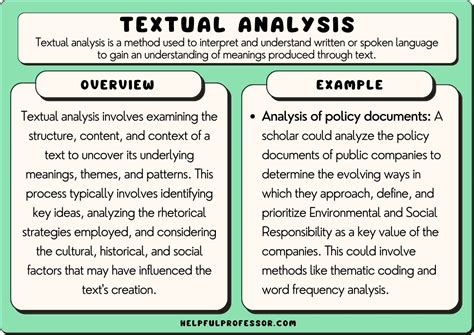
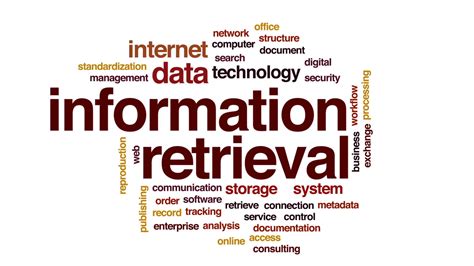
What is the most efficient way to extract the first word from a sentence?
+The most efficient way can depend on the context and the tools you are using. However, string splitting is often one of the simplest and most straightforward methods.
Can regular expressions be used for complex text manipulation tasks?
+Yes, regular expressions are very powerful and can be used for a wide range of text manipulation tasks, from simple to complex.
What is NLTK and how is it used in text processing?
+NLTK (Natural Language Toolkit) is a library used for natural language processing tasks. It includes tools for tokenization, stemming, tagging, parsing, and semantic reasoning.
If you've found this article helpful in understanding the different ways to pull the first word from a sentence, we invite you to share your thoughts and experiences with text processing tasks. Whether you're working on a project that involves natural language processing, data cleaning, or simply need to extract specific information from text, there are numerous tools and techniques at your disposal. Feel free to comment below and share this article with others who might find it useful.