Intro
The MID function in Excel VBA is a powerful tool used for extracting a specified number of characters from a text string, starting from a specified position. This function is particularly useful when you need to manipulate or analyze text data within your Excel spreadsheets using VBA (Visual Basic for Applications). Understanding how to use the MID function can greatly enhance your ability to automate tasks and perform complex data manipulations in Excel.
The MID function in VBA is similar to its worksheet function counterpart but is used within VBA code. The syntax of the MID function in VBA is as follows:
MID(string, start, length)
string
is the text string from which you want to extract characters.start
is the position of the first character you want to extract.length
is the number of characters you want to extract.
For example, if you have the string "HelloWorld" and you want to extract "World", you would use the MID function like this:
Dim myString As String
myString = "HelloWorld"
Dim extractedString As String
extractedString = Mid(myString, 6, 5)
In this example, extractedString
would be "World" because the MID function starts extracting characters from the 6th position (since the count starts at 1) and extracts 5 characters.
Using MID in Practical Scenarios
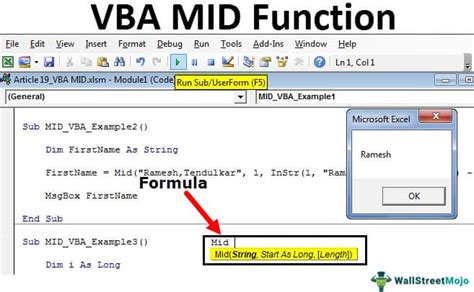
The MID function can be incredibly useful in a variety of scenarios, such as data cleaning, text manipulation, and even in creating custom functions to solve specific problems. For instance, if you have a list of full names and you want to extract the first name or the last name, you can use the MID function in conjunction with other string functions like INSTR to find the position of the space between the names.
Combining MID with Other Functions
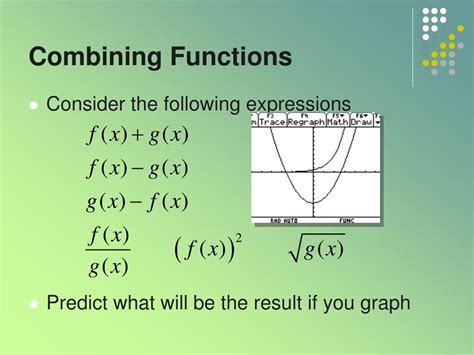
To enhance the utility of the MID function, you can combine it with other VBA string functions. For example, using the INSTR function to find the position of a specific character or substring within a string, and then using that position as the start argument in the MID function.
Dim fullName As String
fullName = "John Doe"
Dim spacePosition As Integer
spacePosition = InStr(1, fullName, " ")
Dim firstName As String
firstName = Mid(fullName, 1, spacePosition - 1)
Dim lastName As String
lastName = Mid(fullName, spacePosition + 1, Len(fullName) - spacePosition)
In this example, the code extracts the first name and the last name from a full name string by finding the position of the space character.
Common Errors and Troubleshooting
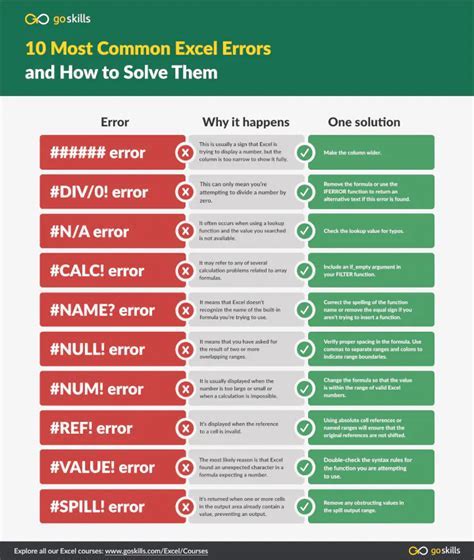
When using the MID function, one common error is specifying a start position that is greater than the length of the string, or specifying a length that exceeds the number of characters remaining in the string from the start position. This will not result in an error but will simply return a string that is shorter than requested or an empty string if the start position is beyond the end of the string.
To troubleshoot issues with the MID function, ensure that your start and length arguments are correct and that the string you are operating on is not empty or null.
Best Practices for Using MID
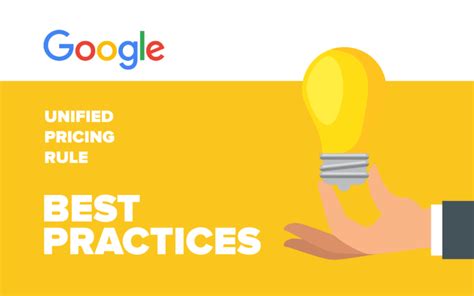
- Always validate the input string and the start and length parameters to ensure they are within valid ranges.
- Consider using other string functions in combination with MID to achieve more complex text manipulations.
- Keep your code readable by using meaningful variable names and commenting your code, especially when performing complex operations.
Advanced Applications of MID
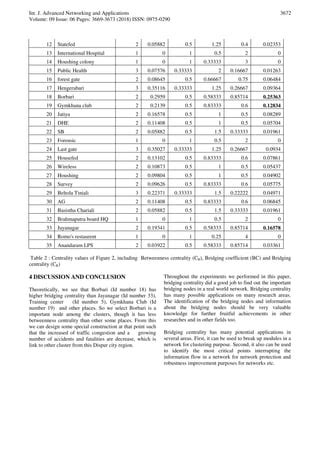
The MID function can be used in more advanced applications such as parsing complex strings, extracting data from poorly formatted sources, or even in generating strings according to specific patterns. Its versatility and simplicity make it a fundamental tool in the arsenal of any VBA developer working with text data.
Gallery of MID Function Examples
MID Function Examples
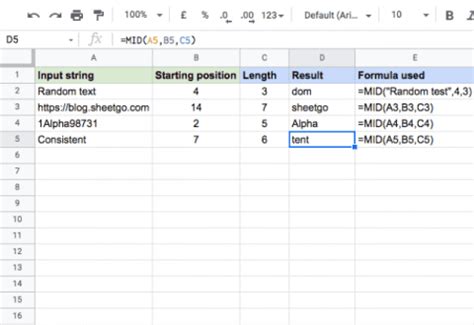
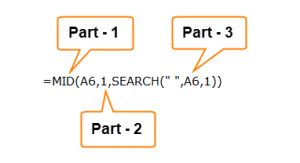
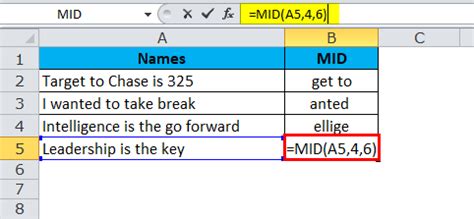
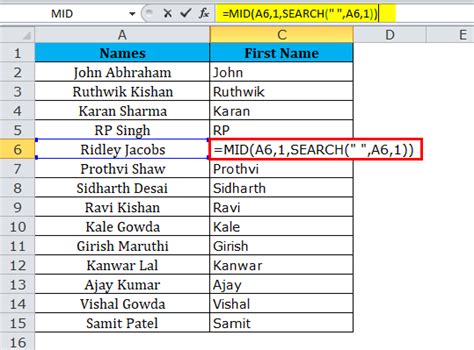
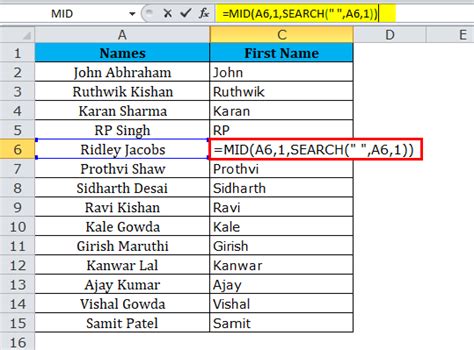
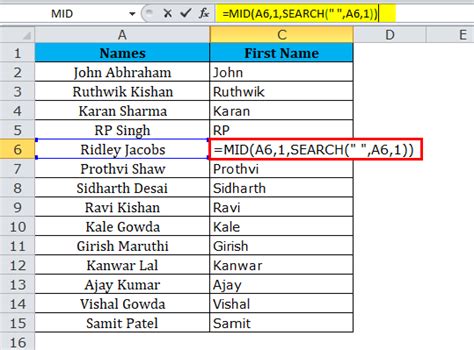
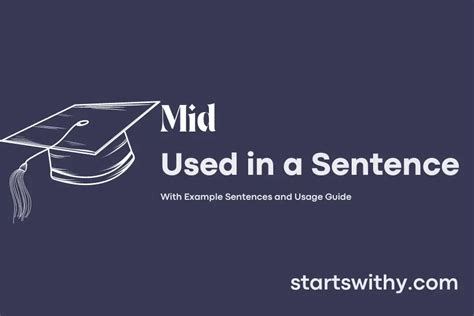
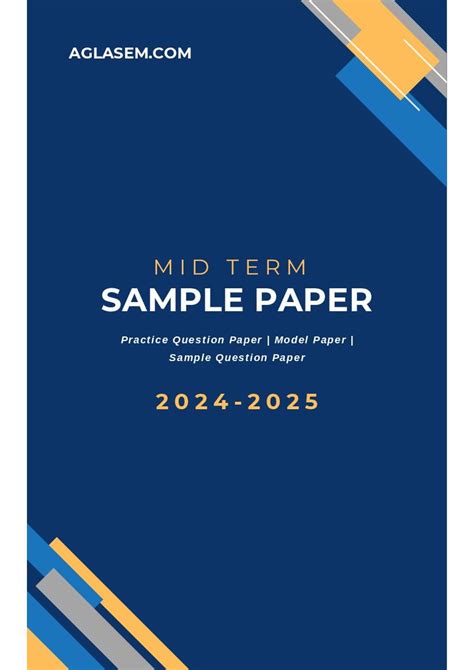
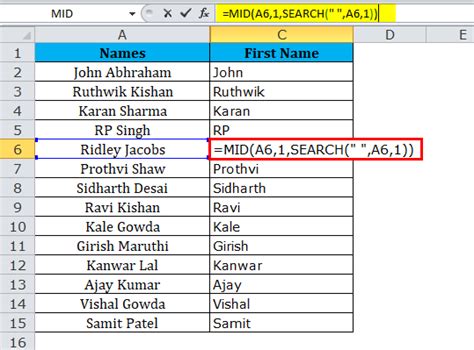
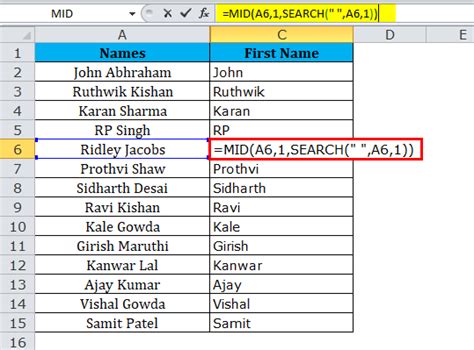
Frequently Asked Questions
What is the MID function used for in Excel VBA?
+The MID function is used to extract a specified number of characters from a text string, starting from a specified position.
How do I troubleshoot issues with the MID function?
+Ensure that your start and length arguments are correct and that the string you are operating on is not empty or null.
Can I use the MID function with other string functions?
+Yes, the MID function can be combined with other string functions like INSTR to achieve more complex text manipulations.
In conclusion, mastering the MID function in Excel VBA can significantly enhance your ability to manipulate and analyze text data within your spreadsheets. By understanding its syntax, common applications, and how to troubleshoot issues, you can leverage this powerful tool to automate tasks and solve complex problems. Whether you're a beginner or an advanced user, the MID function is an essential component of any VBA developer's toolkit. We invite you to share your experiences, ask questions, or provide tips on using the MID function in the comments below. Your engagement will help create a valuable resource for everyone looking to improve their skills in Excel VBA.