Intro
Master VBA with our guide on setting the active worksheet, featuring workbook navigation, sheet activation, and range selection using Excel VBA macros and worksheet objects.
Working with Excel VBA can be a powerful way to automate tasks and improve productivity. One fundamental aspect of VBA programming in Excel is the ability to interact with worksheets. Setting the active worksheet is a common requirement in many VBA scripts, allowing you to perform operations on a specific sheet.
The importance of being able to set the active worksheet cannot be overstated. It allows for precise control over where your macro operates, ensuring that changes are made to the intended worksheet. This is particularly useful in workbooks with multiple sheets, where targeting the wrong sheet could lead to errors or data loss.
Moreover, understanding how to set the active worksheet is a stepping stone to more complex VBA operations. It introduces you to the concept of object-oriented programming, where worksheets are objects that can be manipulated through code. As you delve deeper into VBA, you'll find that mastering these basics is crucial for more advanced tasks, such as data manipulation, chart creation, and even interacting with other Office applications.
In the following sections, we will explore how to set the active worksheet in Excel VBA, along with examples and practical advice on how to use this skill effectively in your macros.
Understanding the Basics of Worksheets in VBA
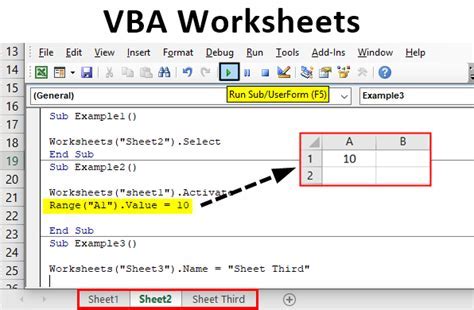
Before diving into how to set the active worksheet, it's essential to understand the basics of how worksheets are referenced in VBA. The Worksheets
collection contains all the worksheets in a workbook. You can access a specific worksheet by its name or index. For example, Worksheets("Sheet1")
references a worksheet named "Sheet1", while Worksheets(1)
references the first worksheet in the workbook.
Referencing Worksheets
You can reference worksheets in several ways: - By name: `Worksheets("SheetName")` - By index: `Worksheets(1)` - Using the `ActiveSheet` property: `ActiveSheet`Setting the Active Worksheet
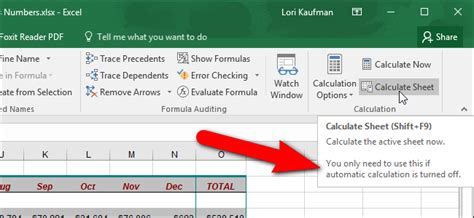
To set the active worksheet, you use the Activate
method. Here's how you can do it:
Sub SetActiveWorksheet()
Worksheets("Sheet1").Activate
End Sub
This code will activate the worksheet named "Sheet1". If "Sheet1" does not exist, VBA will throw an error.
Best Practices for Setting the Active Worksheet
- **Always** check if the worksheet exists before trying to activate it to avoid errors. - Use the worksheet's codename instead of its name when possible. The codename is the name that appears in the VBA editor's project explorer, and it does not change even if the worksheet's name is changed in Excel.Sub SafeActivateWorksheet()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If ws.Name = "Sheet1" Then
ws.Activate
Exit Sub
End If
Next ws
MsgBox "Worksheet not found."
End Sub
Working with Multiple Worksheets
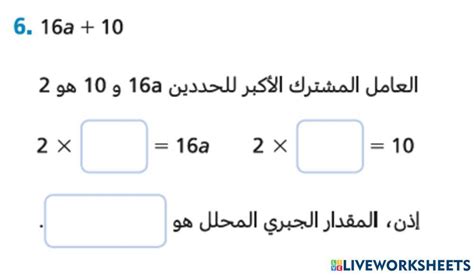
Often, you'll need to perform actions on multiple worksheets. You can loop through all worksheets in a workbook using a For Each
loop.
Sub LoopThroughWorksheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
' Perform action on ws
ws.Range("A1").Value = "Hello, World!"
Next ws
End Sub
Tips for Looping Through Worksheets
- Use `ThisWorkbook` to ensure you're working with the workbook that contains your macro, not the active workbook. - Consider disabling screen updating (`Application.ScreenUpdating = False`) before looping through worksheets to improve performance, especially if you're making many changes.Common Errors and Troubleshooting
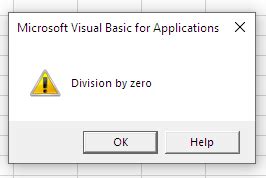
When working with worksheets in VBA, you might encounter several common errors:
- Worksheet not found: Ensure the worksheet name is spelled correctly and exists in the workbook.
- Permission errors: Check if the workbook or worksheet is protected.
Troubleshooting Tips
- Use the debugger to step through your code and identify where errors occur. - Check the Immediate window for error messages.Gallery of VBA Worksheet Examples
VBA Worksheet Examples
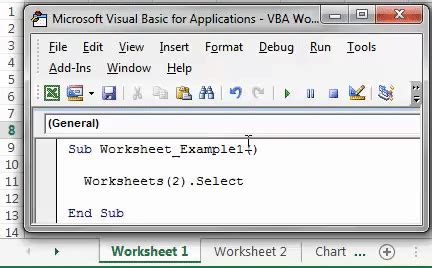
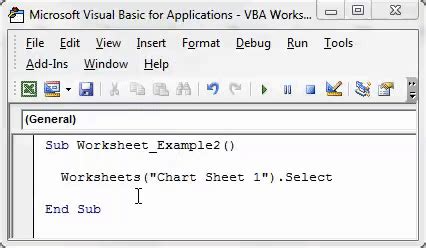
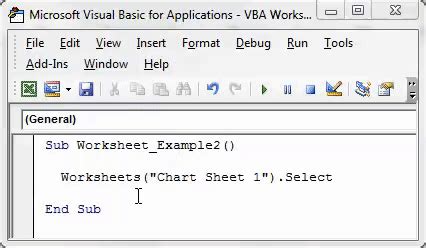
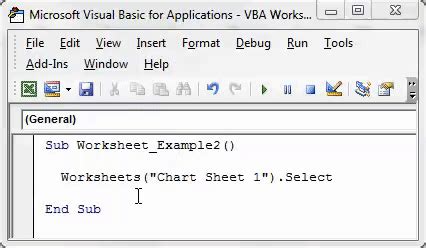
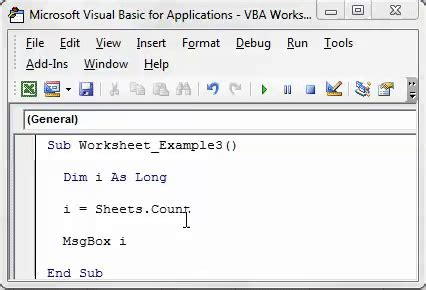
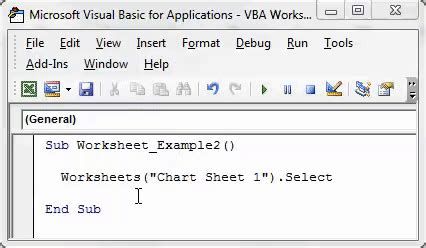
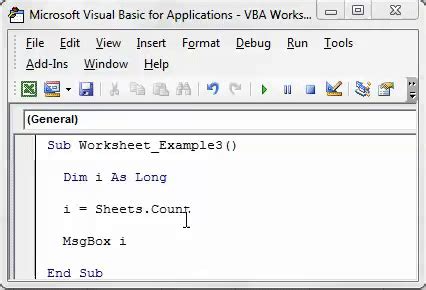
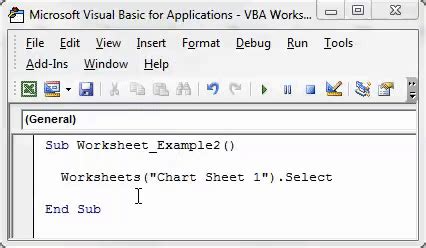
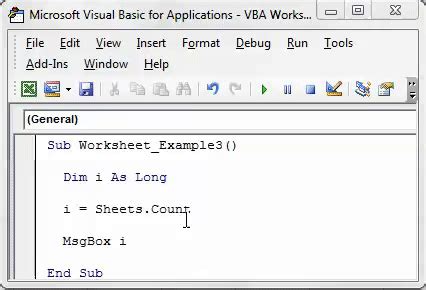
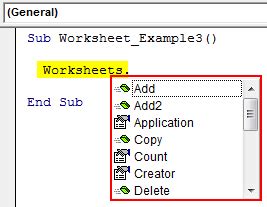
Frequently Asked Questions
How do I reference a worksheet in VBA?
+You can reference a worksheet by its name (e.g., `Worksheets("Sheet1")`) or by its index (e.g., `Worksheets(1)`).
What is the difference between a worksheet's name and its codename?
+A worksheet's name is what appears in Excel (e.g., "Sheet1"), while its codename is used in VBA (visible in the Project Explorer) and does not change even if the worksheet's name is changed in Excel.
How can I loop through all worksheets in a workbook?
+You can use a `For Each` loop, iterating over the `Worksheets` collection of the workbook (e.g., `For Each ws In ThisWorkbook.Worksheets`).
In conclusion, mastering the skill of setting the active worksheet in Excel VBA is fundamental for any automation task. By understanding how to reference, activate, and loop through worksheets, you can create powerful macros that automate complex tasks with precision. Whether you're a beginner looking to learn the basics or an advanced user seeking to refine your skills, the ability to work with worksheets in VBA is an indispensable tool in your Excel automation toolkit. We invite you to share your experiences, ask questions, or provide tips on working with worksheets in VBA in the comments below.