Intro
Optimize Excel sheets with VBA set column width, adjusting cell sizes, and formatting spreadsheets for better data visibility and readability, using macros and worksheet automation techniques.
Setting the column width in Excel using VBA (Visual Basic for Applications) can be a useful skill for automating tasks and customizing your spreadsheets. Column widths are crucial for ensuring that data is properly displayed and readable within your Excel worksheets. Here's how you can set column widths using VBA, along with explanations and examples to guide you through the process.
To set the column width in VBA, you typically use the ColumnWidth
property of the Range
object. This property allows you to specify the width of a column in points (1 point = 1/72 inch). Below are the basic steps and examples to get you started.
Basic Syntax
The basic syntax to set the column width is as follows:
Worksheets("Sheet1").Columns("A").ColumnWidth = 10
In this example, Sheet1
is the name of the worksheet where you want to adjust the column width, A
is the column letter you want to adjust, and 10
is the width in points you want to set.
Setting Column Width for Multiple Columns
If you need to set the width for multiple columns at once, you can specify a range of columns. For example:
Worksheets("Sheet1").Columns("A:C").ColumnWidth = 10
This will set the width of columns A, B, and C to 10 points.
Autofit Column Width
Sometimes, you might want to automatically adjust the column width to fit the contents of the cells. You can do this using the AutoFit
method:
Worksheets("Sheet1").Columns("A").AutoFit
This will automatically adjust the width of column A to fit the longest entry in that column.
Practical Example
Let's say you have a spreadsheet named DataSheet
and you want to set the width of column A to 15 points, and then autofit the width of column B to ensure it's wide enough to display all the data without truncation. Here's how you can do it:
Sub AdjustColumnWidths()
With Worksheets("DataSheet")
.Columns("A").ColumnWidth = 15
.Columns("B").AutoFit
End With
End Sub
This VBA subroutine, AdjustColumnWidths
, first sets the width of column A to 15 points and then autofits column B.
Tips for Working with Column Widths
- Using Loops: If you need to set the width of multiple columns based on certain conditions, consider using a
For
loop to iterate over the columns. - AutoFit vs. Manual Width: While
AutoFit
is convenient, it might not always produce the desired result, especially if your cells contain long formulas or if you're working with wrapped text. In such cases, manually setting the column width might be more appropriate. - Points vs. Characters: Remember that column widths are set in points, not characters. The width in characters can vary depending on the font used in the cells.
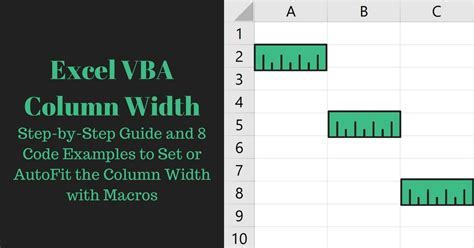
Advanced Techniques
For more complex scenarios, such as dynamically adjusting column widths based on the contents of cells or applying different widths to different parts of your spreadsheet, you might need to use more advanced VBA techniques, such as conditional statements or loops.
Gallery of VBA Column Width Examples
VBA Column Width Examples

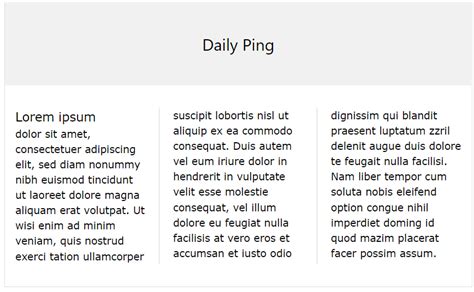
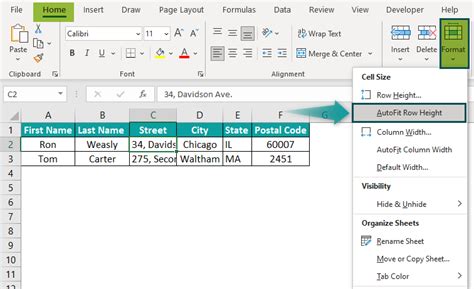
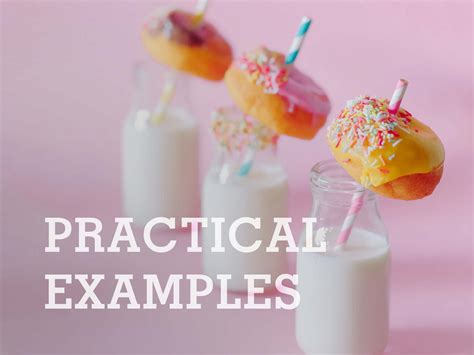
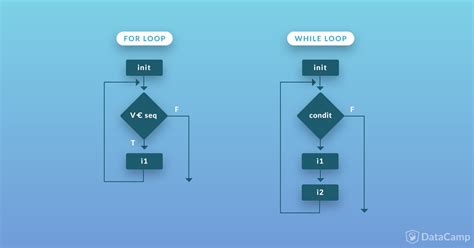

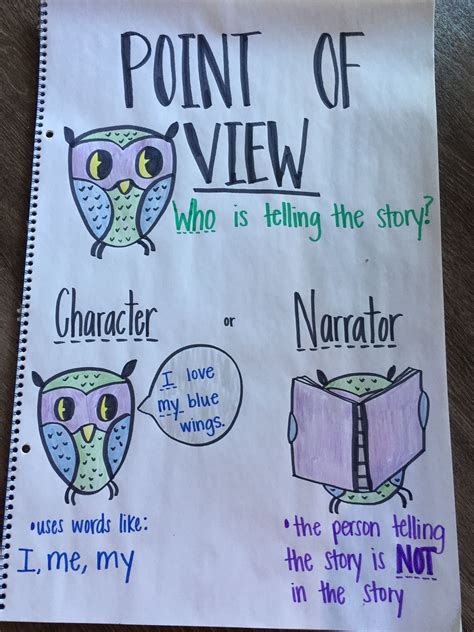
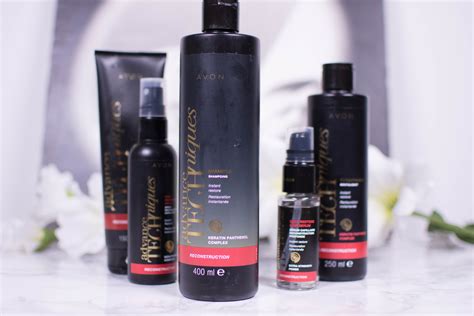
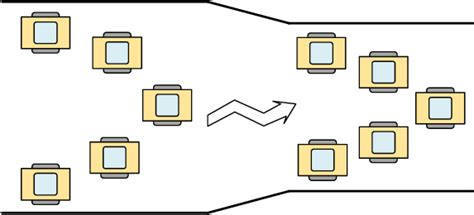
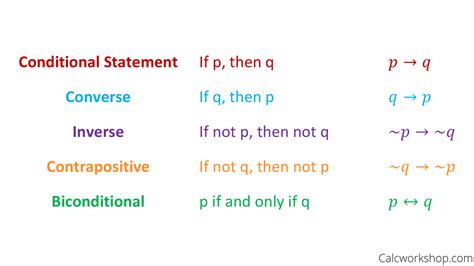
FAQs
How do I set the column width in VBA?
+You can set the column width using the `ColumnWidth` property of the `Range` object, such as `Worksheets("Sheet1").Columns("A").ColumnWidth = 10`.
How do I autofit the column width in VBA?
+You can autofit the column width by using the `AutoFit` method, such as `Worksheets("Sheet1").Columns("A").AutoFit`.
What is the difference between points and characters in column widths?
+Column widths are set in points (1 point = 1/72 inch), not characters. The width in characters can vary depending on the font used in the cells.
In conclusion, setting column widths in Excel using VBA is a straightforward process that can greatly enhance the readability and usability of your spreadsheets. By mastering the basic syntax and understanding how to apply it in different scenarios, you can efficiently manage your data and create more effective Excel worksheets. Whether you're working with simple spreadsheets or complex data analysis projects, being able to control column widths is an essential skill for any Excel user.