Intro
Working with Excel sheets by name using VBA (Visual Basic for Applications) can greatly enhance your ability to automate tasks, manipulate data, and interact with your spreadsheets in a more dynamic way. This approach is particularly useful when you're dealing with multiple sheets and need to perform actions based on the sheet's name rather than its position in the workbook.
Why Use Sheet Name?
Using a sheet's name instead of its index (position in the workbook) offers several advantages:
- Flexibility: If the order of sheets changes, your code won't break because it references sheets by name, not by position.
- Readability: Your code becomes more readable and understandable, as the sheet names clearly indicate what part of the data or functionality you're dealing with.
- Maintainability: If a sheet needs to be renamed for business or organizational reasons, you only need to update the name in your VBA code, rather than potentially reordering indexes throughout your macros.
Basic Operations with Sheets by Name
Here are some basic operations you can perform on sheets by name:
Activating a Sheet
To activate a sheet named "Summary", you can use the following code:
Worksheets("Summary").Activate
Selecting a Range
To select a range on a specific sheet:
Worksheets("Data").Range("A1:B2").Select
Copying Data
To copy data from one sheet to another:
Worksheets("Source").Range("A1").Copy Destination:=Worksheets("Target").Range("B1")
Hiding/Unhiding Sheets
To hide or unhide a sheet:
Worksheets("Confidential").Visible = False ' Hide
Worksheets("Confidential").Visible = True ' Unhide
Looping Through Sheets
Sometimes, you might need to perform an action on multiple sheets. You can loop through all sheets in a workbook like this:
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
' Perform an action on each sheet
ws.Range("A1").Value = "Processed"
Next ws
Or, if you want to target specific sheets by name, you can use an array of sheet names:
Dim targetSheets() As String
targetSheets = Array("Sheet1", "Sheet3", "Summary")
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If Not IsError(Application.Match(ws.Name, targetSheets, 0)) Then
' Perform an action on the targeted sheets
ws.Range("A1").Value = "Targeted"
End If
Next ws
Error Handling
When working with sheets by name, it's crucial to handle potential errors, such as attempting to access a sheet that doesn't exist. You can use On Error Resume Next
or check if a sheet exists before trying to access it:
If Evaluate("ISREF('" & sheetName & "'!A1)") Then
' Sheet exists, perform actions
Worksheets(sheetName).Activate
Else
MsgBox "The sheet '" & sheetName & "' does not exist.", vbExclamation
End If
Best Practices
- Consistency: Keep your sheet names consistent and avoid using special characters or spaces if possible.
- Comments: Use comments in your code to explain what each section is intended to do, especially when working with complex logic or multiple sheets.
- Testing: Always test your code in a non-production environment first to ensure it works as expected.
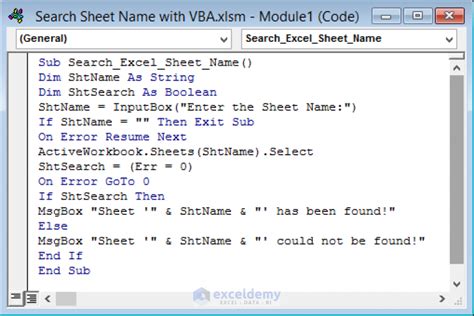
Advanced Topics
As you become more comfortable with VBA, you might explore more advanced topics such as:
- UserForms: Creating custom dialog boxes to interact with users.
- Classes: Using class modules to create custom objects.
- Error Handling: Implementing robust error handling mechanisms.
These topics can help you create more sophisticated and user-friendly applications within Excel.
Gallery of VBA Examples
VBA Examples Image Gallery
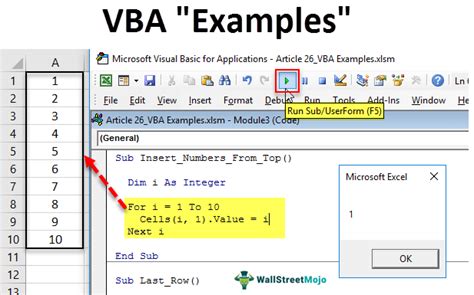
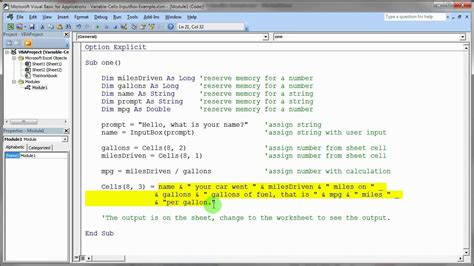
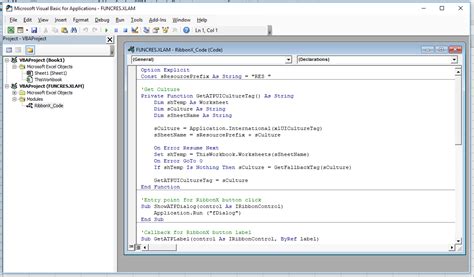
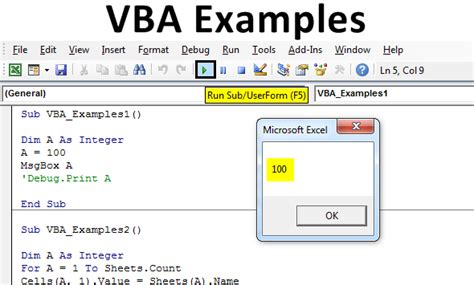
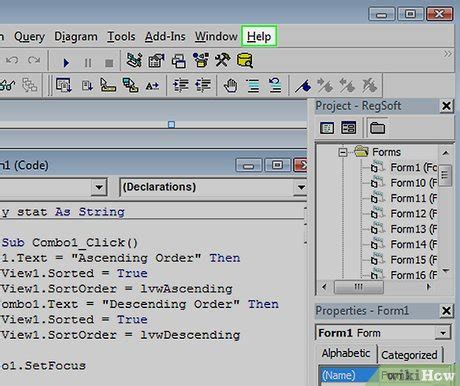
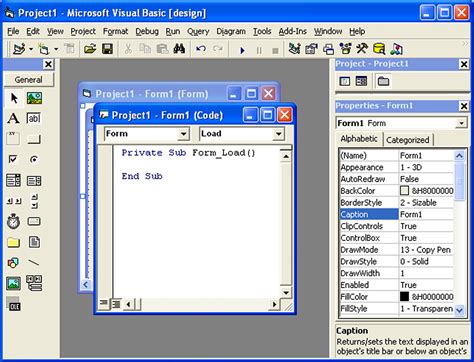
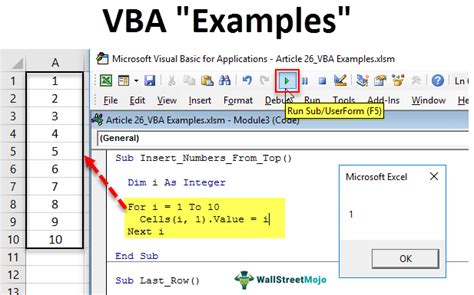
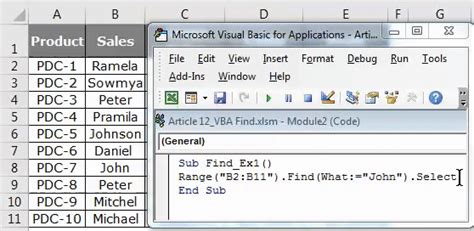
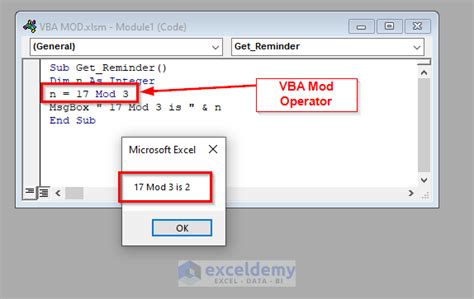
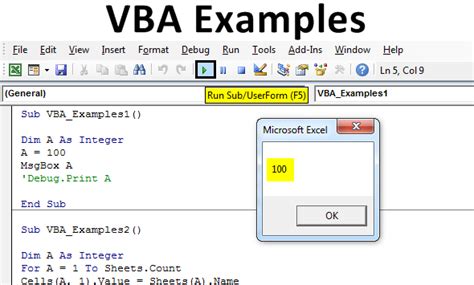
FAQs
How do I reference a sheet by name in VBA?
+You can reference a sheet by name using `Worksheets("SheetName")`, replacing "SheetName" with the actual name of your sheet.
What if the sheet I'm trying to access doesn't exist?
+You should implement error handling to check if the sheet exists before trying to access it. You can use `On Error Resume Next` or check with `Evaluate("ISREF('" & sheetName & "'!A1)")`.
How can I loop through all sheets in a workbook and perform an action?
+You can use a `For Each` loop with `ThisWorkbook.Worksheets` to iterate through all sheets and perform actions on each one.
In conclusion, working with sheets by name in VBA is a powerful way to automate and interact with your Excel spreadsheets. By mastering these techniques and best practices, you can create more efficient, flexible, and maintainable VBA applications. Whether you're a beginner or an advanced user, understanding how to effectively use sheet names in your code can significantly enhance your productivity and the functionality of your spreadsheets.