Intro
The world of Visual Basic for Applications (VBA) is a vast and powerful one, allowing users to automate and enhance their interactions with Microsoft Office applications. Among the numerous functions and methods available in VBA, the Match
function stands out for its utility in searching for specific values within ranges or arrays. This function is a part of the Excel VBA library and is particularly useful for tasks that require finding the relative position of an item in a list.
Understanding how to use the Match
function effectively can significantly improve the efficiency and accuracy of your VBA scripts. Here are five tips to help you master the Match
function in VBA:
Introduction to the Match Function
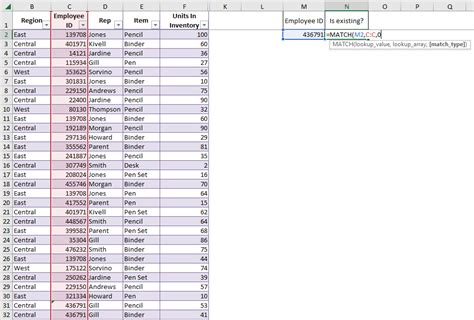
The Match
function in VBA is used to find the relative position of a value within a range or an array. Its basic syntax is Match(lookup_value, lookup_array, [match_type])
, where lookup_value
is the value you want to find, lookup_array
is the range or array you're searching in, and match_type
is an optional argument that specifies whether you want an exact match or an approximate match.
Tip 1: Understanding Match Types
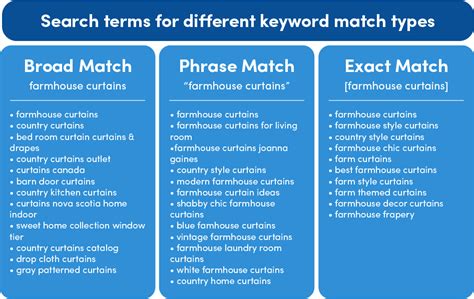
The match_type
argument in the Match
function allows you to specify how you want to match the lookup_value
with values in the lookup_array
. There are three types of matches you can specify:
1
or omitted: Finds an exact match. If no exact match is found, it returns a #N/A error.0
: Finds an exact match. If no exact match is found, it returns a #N/A error. This is the default behavior.-1
: Finds a value that is less than thelookup_value
. If no such value exists, it returns a #N/A error.1
: Finds a value that is greater than thelookup_value
. If no such value exists, it returns a #N/A error.
Exact Match Example
When looking for an exact match, you can simply use the `Match` function without specifying the `match_type`, or you can explicitly set it to `0`.Sub ExactMatchExample()
Dim lookupValue As Variant
Dim lookupArray As Range
Dim matchResult As Variant
lookupValue = "Apple"
Set lookupArray = Range("A1:A10")
matchResult = Application.Match(lookupValue, lookupArray, 0)
If Not IsError(matchResult) Then
MsgBox "Found at position: " & matchResult
Else
MsgBox "Not found"
End If
End Sub
Tip 2: Handling Errors
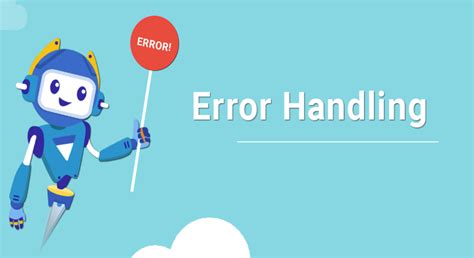
The Match
function returns a #N/A error if it cannot find the lookup_value
in the lookup_array
. To handle this, you can use the IsError
function in VBA to check if the result of the Match
function is an error.
Sub ErrorHandlerExample()
Dim lookupValue As Variant
Dim lookupArray As Range
Dim matchResult As Variant
lookupValue = "Orange"
Set lookupArray = Range("A1:A10")
matchResult = Application.Match(lookupValue, lookupArray, 0)
If IsError(matchResult) Then
MsgBox "The value was not found."
Else
MsgBox "The value was found at position: " & matchResult
End If
End Sub
Tip 3: Searching in Arrays
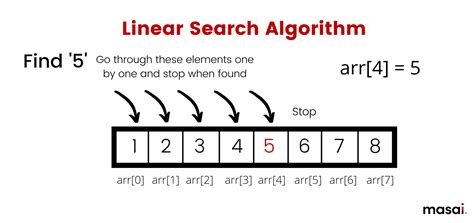
Besides searching in ranges, the Match
function can also be used to search within arrays. This is particularly useful when you need to work with data that is already loaded into memory as an array.
Sub SearchingInArrays()
Dim myArray As Variant
Dim lookupValue As Variant
Dim matchResult As Variant
myArray = Array("Apple", "Banana", "Cherry")
lookupValue = "Banana"
matchResult = Application.Match(lookupValue, myArray, 0)
If Not IsError(matchResult) Then
MsgBox "Found at position: " & matchResult
Else
MsgBox "Not found"
End If
End Sub
Tip 4: Using Match with Other Functions
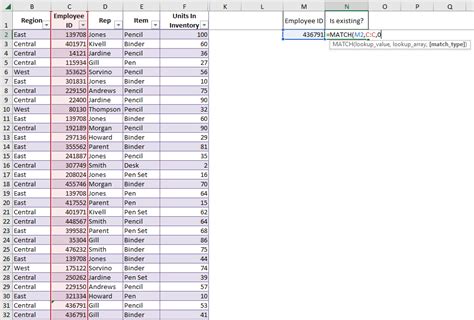
The Match
function can be combined with other Excel functions to achieve more complex tasks. For example, you can use it with the Index
function to return a value from a range based on its relative position.
Sub UsingMatchWithIndex()
Dim lookupValue As Variant
Dim lookupArray As Range
Dim returnArray As Range
Dim matchResult As Variant
lookupValue = "Pear"
Set lookupArray = Range("A1:A10")
Set returnArray = Range("B1:B10")
matchResult = Application.Match(lookupValue, lookupArray, 0)
If Not IsError(matchResult) Then
MsgBox Application.Index(returnArray, matchResult)
Else
MsgBox "Not found"
End If
End Sub
Tip 5: Performance Considerations
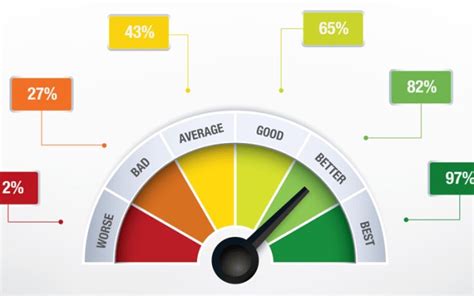
When using the Match
function, especially in loops or with large datasets, it's essential to consider performance. The Match
function can be slower than using Find
methods or looping through cells manually, especially if your dataset is very large. Always test your code with a representative dataset to ensure it performs as expected.
Gallery of Match Function Examples
Match Function Image Gallery
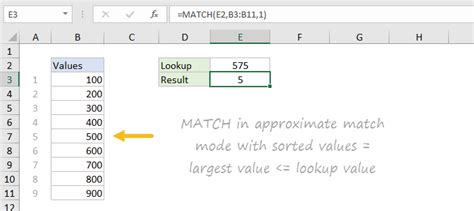
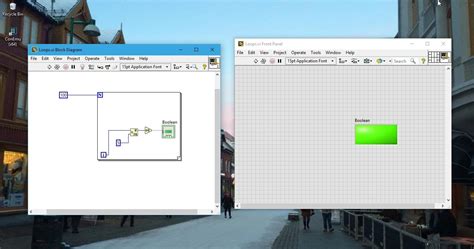
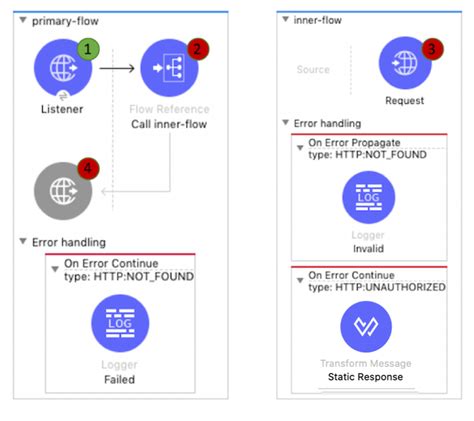
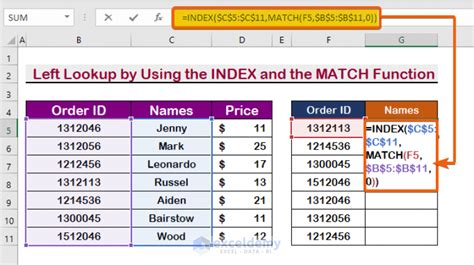
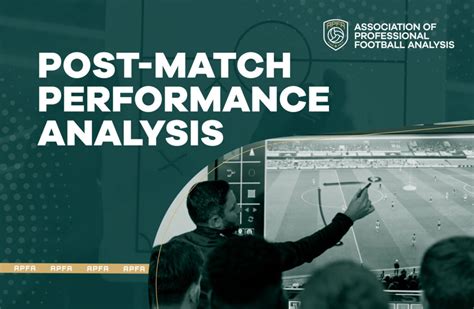
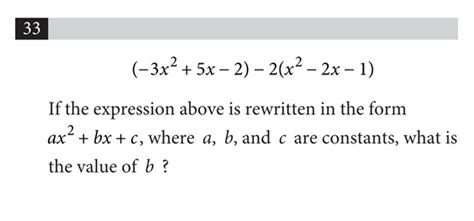
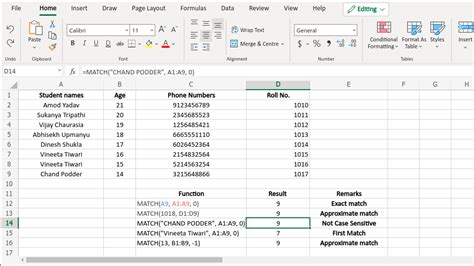
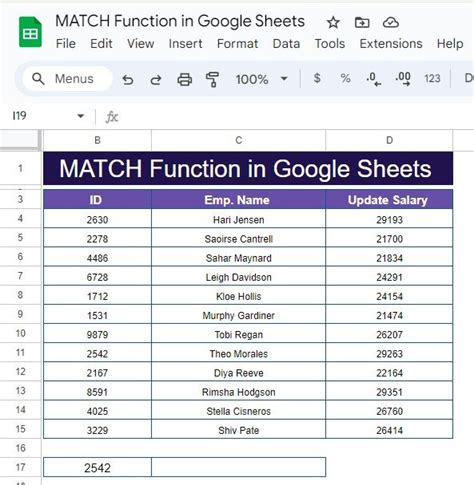
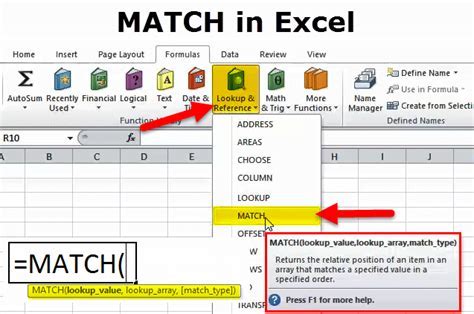
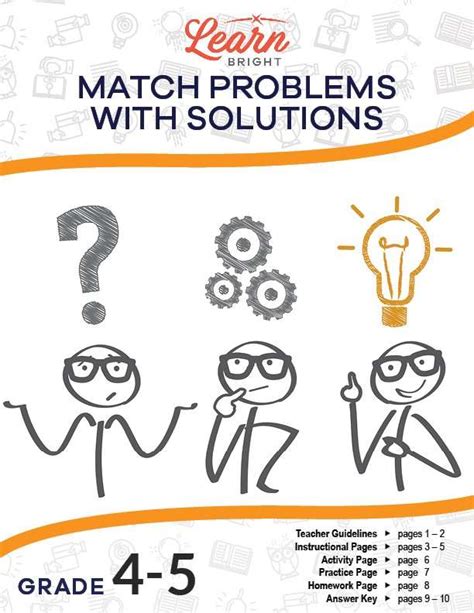
What is the purpose of the Match function in VBA?
+The Match function is used to find the relative position of a value within a range or an array.
How do I handle errors when using the Match function?
+You can use the IsError function to check if the result of the Match function is an error, indicating the value was not found.
Can the Match function be used with arrays?
+Yes, the Match function can be used to search for values within arrays, not just ranges.
In conclusion, mastering the Match
function in VBA can greatly enhance your ability to automate tasks and interact with data in Microsoft Office applications. By understanding the different match types, handling errors appropriately, and combining Match
with other functions, you can create powerful and efficient scripts. Remember to consider performance when working with large datasets and to always test your code thoroughly. With practice and experience, you'll find the Match
function to be an indispensable tool in your VBA toolkit. Feel free to comment below with any questions or share your own tips and experiences with using the Match
function in VBA.