Intro
Counting cells by color in Google Sheets can be a useful feature, especially when you're working with datasets that use color coding to categorize or highlight important information. Unfortunately, Google Sheets doesn't have a built-in function like COUNTIF that directly counts cells based on their background color. However, there are workarounds and scripts that can help you achieve this functionality.
To start exploring how to count cells by color, it's essential to understand the basics of Google Sheets and how it handles conditional formatting and custom formulas. Conditional formatting allows you to change the background color of cells based on specific conditions, but it doesn't directly count cells. For counting, we often rely on formulas like COUNTIF or COUNTIFS, which are based on cell values rather than cell formats.
Understanding the Need for Counting by Color
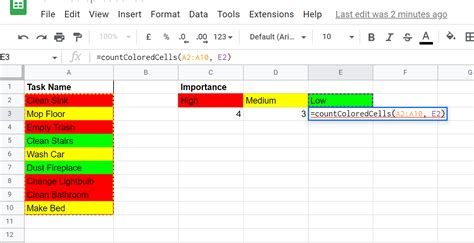
Before diving into the solutions, let's consider why counting cells by color is useful. In many datasets, colors are used to visually represent different statuses, categories, or priorities. For example, in a project management spreadsheet, tasks might be colored green for completed, yellow for in progress, and red for overdue. Being able to count these tasks by their color can provide quick insights into project status without having to manually filter or categorize each task.
Using Google Apps Script
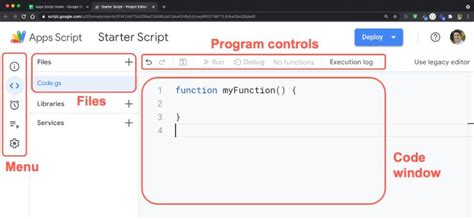
One of the most powerful ways to count cells by color in Google Sheets is by using Google Apps Script. This scripting platform allows you to create custom functions that can interact with your spreadsheet in ways that aren't possible with standard formulas. To count cells by color, you can write a script that iterates through a range of cells, checks the background color of each cell, and increments a counter for each color found.
Here's an example of how you might write such a script:
function countCellsByColor(color) {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var range = sheet.getDataRange();
var count = 0;
for (var i = 1; i <= range.getLastRow(); i++) {
for (var j = 1; j <= range.getLastColumn(); j++) {
if (sheet.getRange(i, j).getBackgroundColor() === color) {
count++;
}
}
}
return count;
}
This script defines a custom function countCellsByColor
that takes a color as an argument and returns the number of cells in the active sheet that have that background color.
Implementing the Script
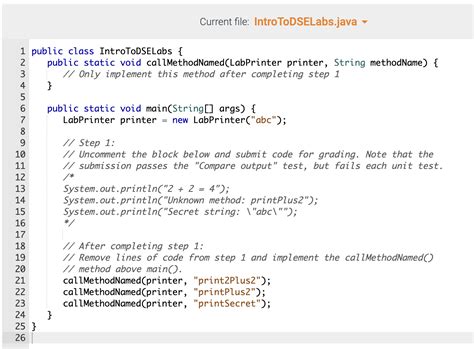
To use this script, follow these steps:
- Open your Google Sheet.
- Click on "Tools" > "Script editor" to open the Google Apps Script editor.
- Delete any code in the editor, and paste the script provided above.
- Save the script by clicking on the floppy disk icon or pressing Ctrl+S (or Cmd+S on a Mac).
- Go back to your Google Sheet.
- You can now use the
countCellsByColor
function in a cell like any other formula, specifying the color you want to count. For example,=countCellsByColor("#FF0000")
would count all cells with a red background.
Alternative Methods
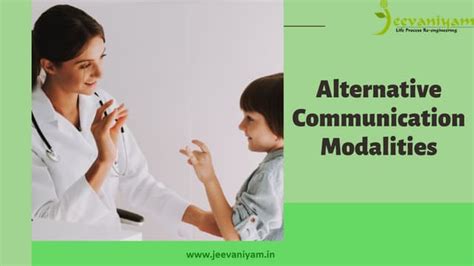
While Google Apps Script provides a powerful solution, there are alternative methods to achieve similar results without scripting:
- Using Filter Views: You can create filter views based on conditional formatting, but this won't give you a count directly.
- Helper Columns: You can use a helper column with a formula that checks the color and returns a value (e.g., 1 for the color of interest, 0 otherwise), and then sum this column. However, Google Sheets formulas cannot directly detect cell background colors.
Limitations and Considerations
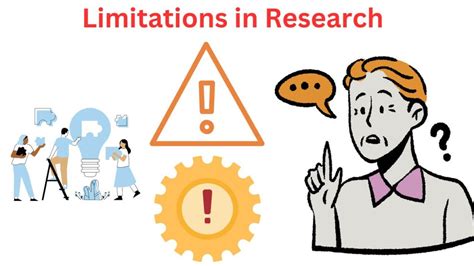
When working with scripts or formulas to count cells by color, consider the following:
- Performance: Scripts can be slower for very large datasets.
- Color Specification: Ensure you're using the correct color format (e.g., hex code) when specifying colors.
- Collaboration: Scripts are tied to the spreadsheet, so collaborators will need access to the script to use custom functions.
Countif By Color Image Gallery
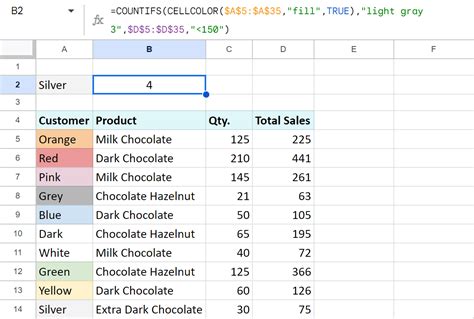
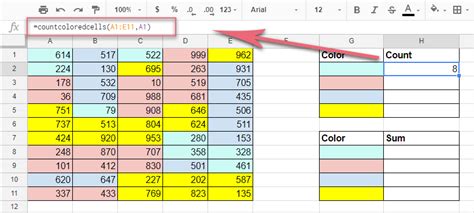

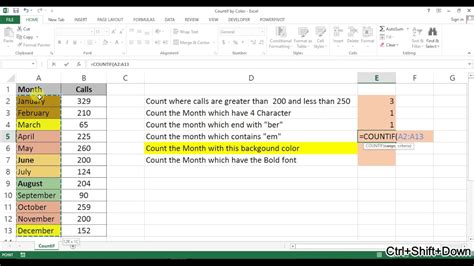
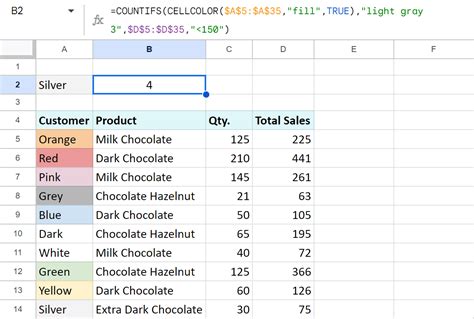
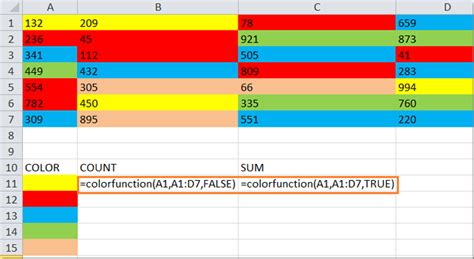
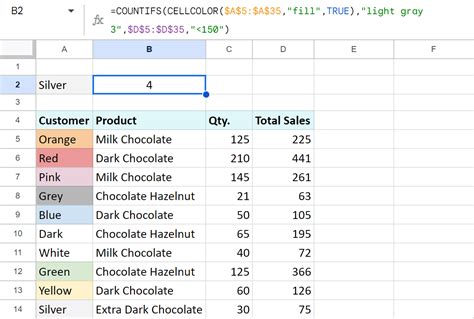
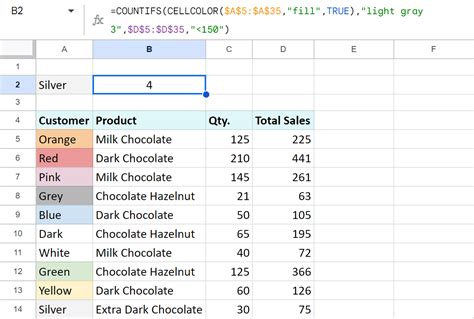
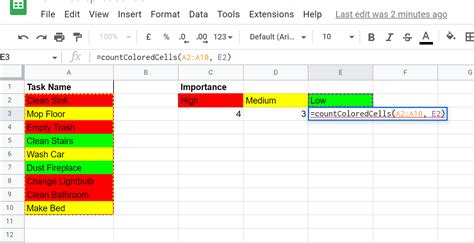
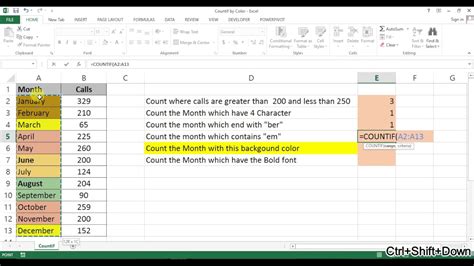
How do I count cells by color in Google Sheets without using scripts?
+Unfortunately, there isn't a direct way to count cells by color without using scripts. However, you can use helper columns with conditional formatting to achieve a similar result, albeit indirectly.
Can I use the COUNTIF function to count cells by color?
+No, the COUNTIF function in Google Sheets cannot directly count cells based on their background color. It's designed to count cells based on their values, not formats.
How do I specify the color in the countCellsByColor script?
+You specify the color by using its hex code. For example, to count cells with a red background, you would use "#FF0000" as the color argument in the script.
In conclusion, counting cells by color in Google Sheets can be achieved through the use of Google Apps Script, providing a powerful and customizable solution for data analysis and visualization. While there are limitations and considerations, especially regarding performance and collaboration, the ability to create custom functions like countCellsByColor
significantly enhances the capabilities of Google Sheets for users who rely on color coding in their spreadsheets. If you have any further questions or need more specific guidance on implementing these solutions, feel free to ask in the comments below.