Intro
Fix Excel Subscript Out Of Range errors with expert solutions, troubleshooting tips, and VBA code fixes, resolving runtime and compilation issues in Excel macros and worksheets.
The "Excel Subscript Out of Range" error is a common issue that many users encounter when working with Excel, particularly when using macros or Visual Basic for Applications (VBA) code. This error typically occurs when your code attempts to access an array or a collection with an index that does not exist. For instance, if you have an array with 5 elements ( indexed from 0 to 4), trying to access the 6th element (index 5) would result in a "Subscript Out of Range" error because the index is beyond the defined range of the array.
Understanding and resolving this error requires a basic knowledge of how arrays and collections work in VBA, as well as how to debug your code. Here's a comprehensive guide to help you navigate through this issue:
What Causes the "Subscript Out of Range" Error?
- Array Indexing: In VBA, arrays are 0-based by default, meaning the first element of an array is at index 0, and the last element is at an index equal to the array's length minus one. If you try to access an element outside this range, you'll get the error.
- Collection Indexing: Similar to arrays, collections also have indexes. However, collections can be 1-based or 0-based depending on how they are defined. Accessing an index that does not exist in the collection results in the error.
- Workbook or Worksheet Objects: When working with Excel objects like workbooks or worksheets, attempting to access a non-existent object (e.g., a worksheet that hasn't been added yet) can also trigger this error.
How to Fix the "Subscript Out of Range" Error
1. Check Array Bounds
Before accessing an array, ensure you know its bounds. You can use UBound()
and LBound()
functions to get the upper and lower bounds of an array.
Dim myArray(1 to 5) As Integer
' Attempting to access myArray(6) would result in an error
2. Validate Collection Existence
For collections, ensure the item exists before trying to access it.
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
' Safe to access ws here
Next ws
' Trying to access ThisWorkbook.Worksheets(100) if there are less than 100 sheets would cause an error
3. Use Error Handling
Implement error handling to gracefully manage and debug errors.
On Error Resume Next
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("NonExistentSheet")
If ws Is Nothing Then
MsgBox "Worksheet does not exist."
End If
On Error GoTo 0
4. Dynamically Size Arrays
Use ReDim
to dynamically adjust the size of an array based on the data you're working with.
Dim dataArray() As Variant
ReDim dataArray(1 To rowCount) ' Assuming rowCount is the number of rows you need
Best Practices to Avoid the Error
- Bounds Checking: Always check the bounds of arrays and collections before accessing them.
- Option Explicit: Use
Option Explicit
at the top of your modules to require explicit variable declaration, helping catch typos and undefined variables. - Error Handling: Implement robust error handling to manage unexpected errors gracefully.
- Debugging: Use the VBA debugger to step through your code, examine variables, and identify where the error occurs.
Troubleshooting Steps
- Identify the Line Causing the Error: Use the debugger to pinpoint the exact line where the error occurs.
- Check Variable Values: Examine the values of variables involved in the line causing the error.
- Verify Object Existence: If working with objects, ensure they exist and are properly set before use.
- Review Array and Collection Indexing: Double-check that you're accessing arrays and collections within their valid index ranges.
Conclusion and Next Steps
The "Subscript Out of Range" error in Excel VBA, while frustrating, is often straightforward to resolve once you understand its causes and how to properly manage arrays, collections, and object references. By following best practices such as bounds checking, using error handling, and debugging your code thoroughly, you can write more robust VBA scripts and avoid this error. Remember, practice makes perfect, so continue to work on your VBA skills and explore more advanced topics to become proficient in automating tasks in Excel.
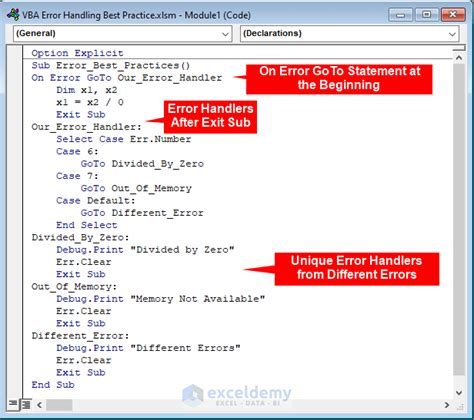
Understanding Arrays in VBA
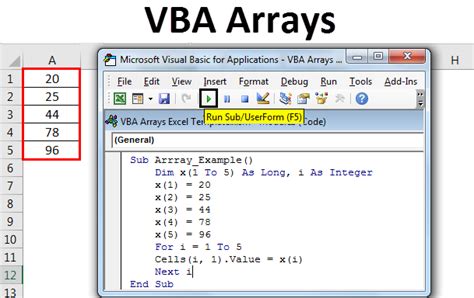
Working with Collections
Collections in VBA are useful for storing and manipulating groups of objects. Understanding how to work with collections can help you avoid the "Subscript Out of Range" error.
Debugging Techniques
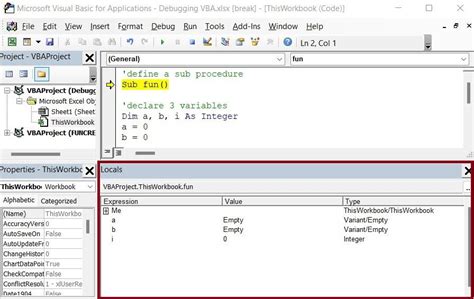
Advanced Error Handling
Error handling is crucial for making your VBA scripts robust. Learning advanced error handling techniques can help you manage errors more effectively.
Best Practices for VBA Coding
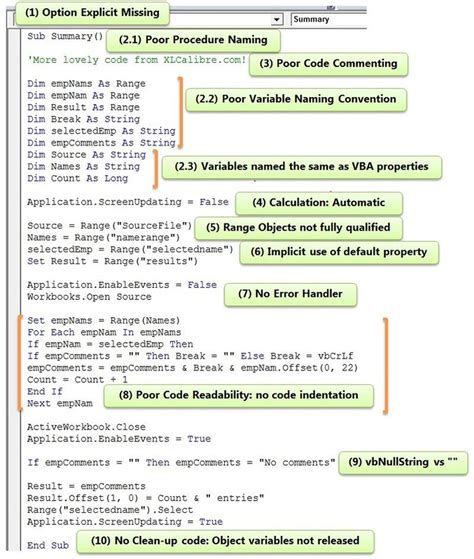
Gallery of Excel VBA Tips
Excel VBA Tips Image Gallery
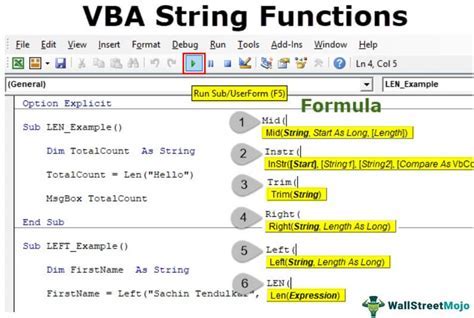
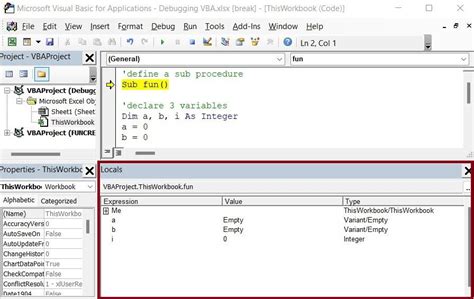
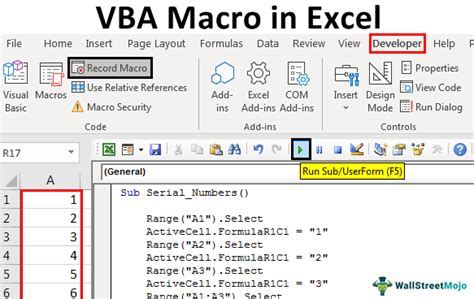
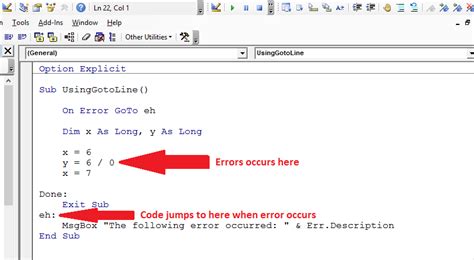
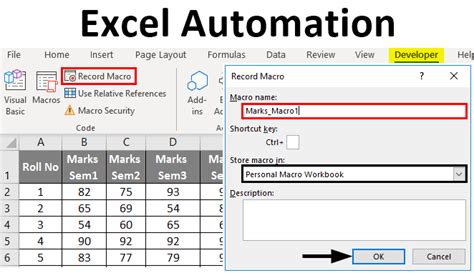
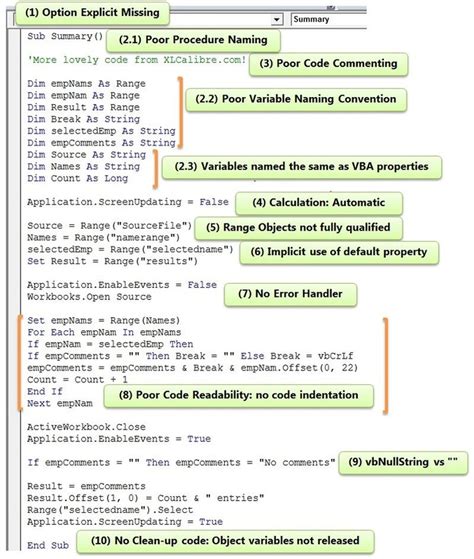
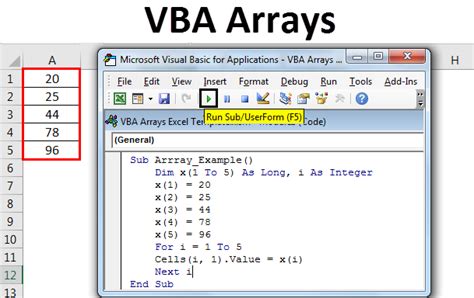
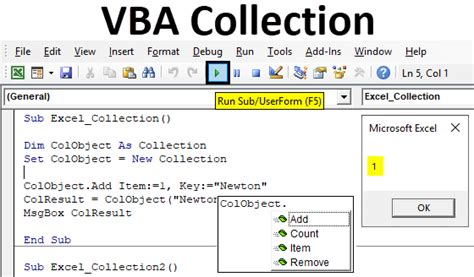
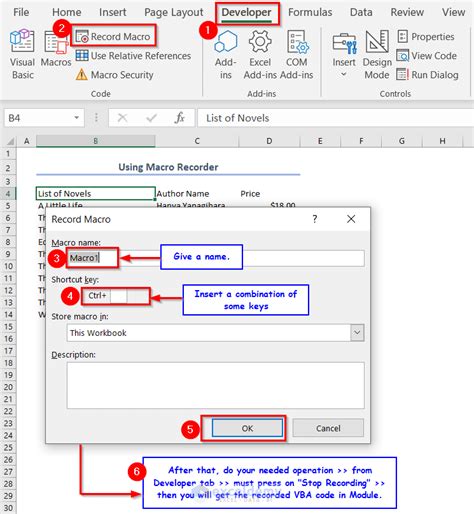
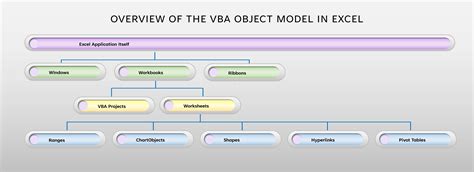
What is the "Subscript Out of Range" error in Excel VBA?
+The "Subscript Out of Range" error occurs when your VBA code attempts to access an array or collection with an index that does not exist.
How can I avoid the "Subscript Out of Range" error?
+To avoid this error, always check the bounds of arrays and collections before accessing them, use error handling, and ensure objects exist before trying to access them.
What are some best practices for working with arrays in VBA?
+Best practices include using `ReDim` to dynamically size arrays, checking array bounds with `UBound()` and `LBound()`, and avoiding hard-coded indices when possible.
We hope this comprehensive guide has helped you understand and resolve the "Subscript Out of Range" error in Excel VBA. Remember, mastering VBA takes time and practice, so don't be discouraged by errors—use them as opportunities to learn and improve your skills. If you have any further questions or need more specific guidance, feel free to ask in the comments below.