Intro
Master Excel Clear Contents VBA with 5 efficient methods, including range selection, cell formatting, and macro automation, to boost spreadsheet productivity and data management using Visual Basic for Applications.
Excel is a powerful tool used for data analysis, visualization, and management. One of the essential skills for working efficiently in Excel is mastering its Visual Basic for Applications (VBA) capabilities. VBA allows users to automate tasks, create custom tools, and interact with Excel in a more personalized way. Among the various tasks that can be automated, clearing contents from cells is a common requirement. This can be necessary for preparing worksheets for new data, removing obsolete information, or resetting templates. In this article, we will delve into five ways to clear contents using Excel VBA, exploring the different methods and their applications.
When working with Excel VBA, understanding the various methods to clear cell contents is crucial. The most common methods include using the ClearContents
method, the Clear
method, deleting cells, using the Range
object to specify which cells to clear, and utilizing loops for more complex clearing tasks. Each of these methods has its own use cases and advantages, depending on what exactly you need to achieve.
Understanding the Basics of Excel VBA
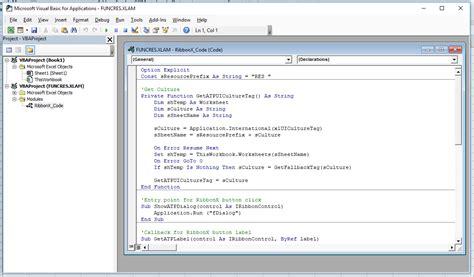
Before diving into the specifics of clearing contents, it's essential to have a basic understanding of how Excel VBA works. VBA is accessed through the Visual Basic Editor, which can be opened by pressing Alt + F11
or by navigating to the Developer tab in Excel and clicking on Visual Basic. From here, you can insert modules, write macros, and interact with Excel's objects, such as worksheets and ranges.
Method 1: Using the ClearContents
Method
ClearContents
Method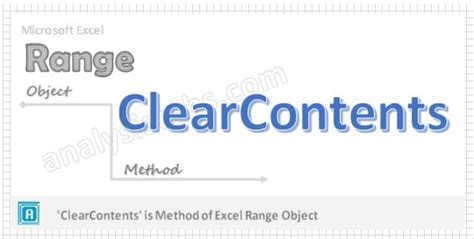
The ClearContents
method is specifically designed to remove the contents of a cell or a range of cells without affecting their formatting. This method is useful when you want to remove data from cells but keep any formatting that has been applied, such as font colors, borders, or number formatting. The syntax for this method is straightforward: Range("A1").ClearContents
, where Range("A1")
specifies the cell or range you want to clear.
Example of ClearContents
Method
ClearContents
MethodSub ClearContentsExample()
Range("A1:B2").ClearContents
End Sub
This example clears the contents of the range A1:B2
without affecting any formatting.
Method 2: Using the Clear
Method
Clear
Method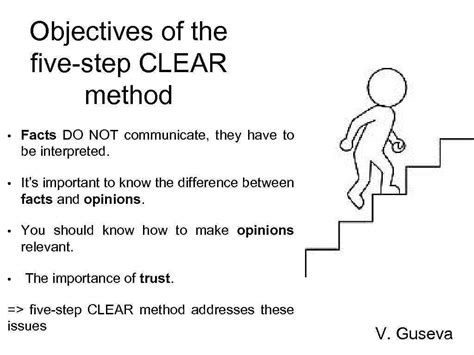
The Clear
method is more comprehensive than ClearContents
. It not only removes the contents of the cells but also clears any formatting. This method is useful when you want to completely reset cells to their default state. The syntax is similar to ClearContents
: Range("A1").Clear
.
Example of Clear
Method
Clear
MethodSub ClearExample()
Range("A1:B2").Clear
End Sub
This example clears both the contents and formatting of the range A1:B2
.
Method 3: Deleting Cells
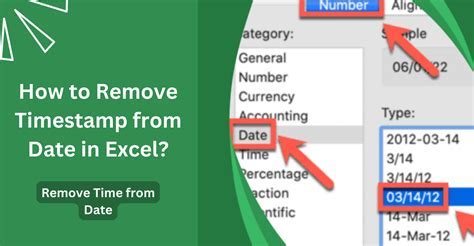
Deleting cells is another way to remove contents, but it also shifts cells around to fill the gap, which can be undesirable in some situations. However, it can be useful when you need to remove not just the contents but the cells themselves. The syntax for deleting cells is Range("A1").Delete
.
Example of Deleting Cells
Sub DeleteCellsExample()
Range("A1:B2").Delete Shift:=xlToLeft
End Sub
This example deletes the cells in the range A1:B2
and shifts the remaining cells to the left to fill the gap.
Method 4: Using the Range
Object
Range
Object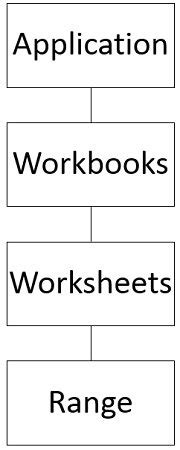
The Range
object is versatile and can be used in various ways to specify which cells to clear. You can use it to clear entire rows, columns, or any specific range. For example, Range("A:A").ClearContents
clears the entire column A, while Range("1:1").ClearContents
clears the entire first row.
Example of Using the Range
Object
Range
ObjectSub RangeObjectExample()
Range("A1", Range("A1").End(xlDown)).ClearContents
End Sub
This example clears the contents of all cells in column A from A1 down to the last cell with data.
Method 5: Utilizing Loops for Complex Clearing Tasks
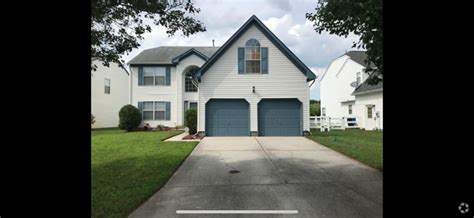
For more complex tasks, such as clearing contents based on certain conditions, loops can be incredibly useful. You can loop through each cell in a range and check if it meets a certain condition before clearing its contents.
Example of Using Loops
Sub LoopExample()
For Each cell In Range("A1:B2")
If cell.Value = "ClearMe" Then
cell.ClearContents
End If
Next cell
End Sub
This example loops through each cell in the range A1:B2
and clears the contents of any cell that contains the value "ClearMe".
Gallery of Excel VBA Clear Contents Methods:
Excel VBA Clear Contents Gallery
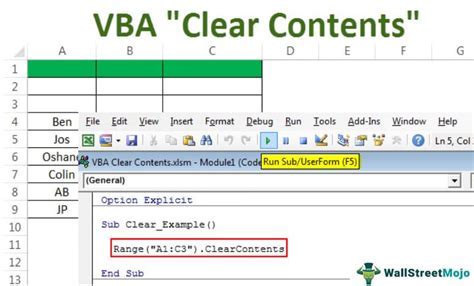
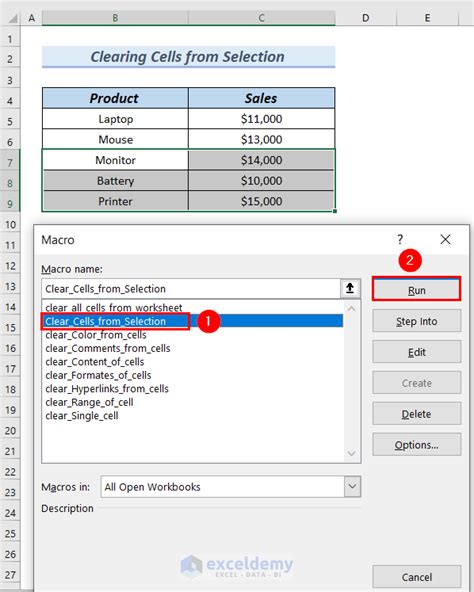
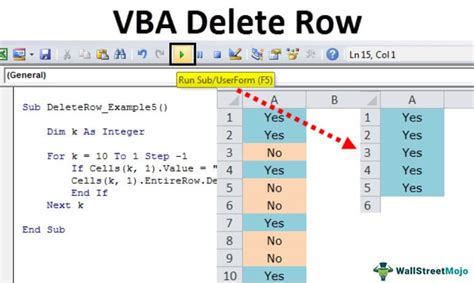
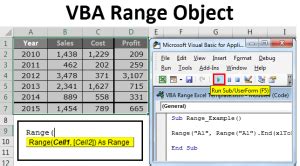
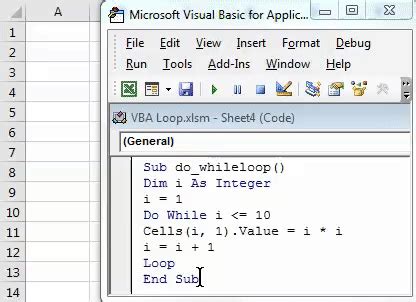
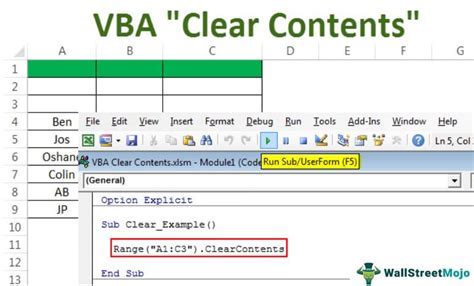
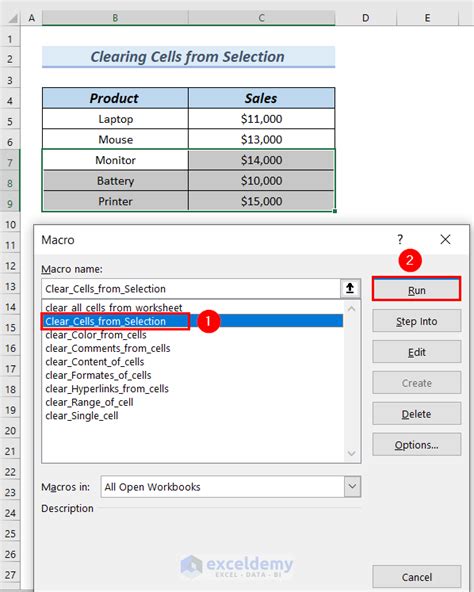
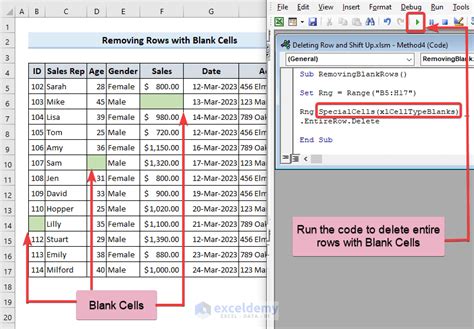

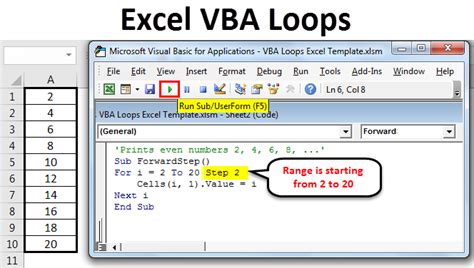
Frequently Asked Questions
What is the difference between `ClearContents` and `Clear` in Excel VBA?
+`ClearContents` removes the contents of cells but leaves formatting intact, while `Clear` removes both contents and formatting.
How do I clear an entire column or row using Excel VBA?
+You can use the `Range` object, such as `Range("A:A").ClearContents` for an entire column or `Range("1:1").ClearContents` for an entire row.
Can I use loops to clear contents based on conditions in Excel VBA?
+Yes, loops can be used to iterate through cells and clear contents based on specific conditions, such as cell values or formatting.
In conclusion, mastering the various methods to clear contents in Excel VBA is a valuable skill for any Excel user looking to automate tasks and work more efficiently. Whether you're using the ClearContents
method, the Clear
method, deleting cells, utilizing the Range
object, or employing loops for more complex tasks, understanding these methods can significantly enhance your productivity and ability to manage data in Excel. We encourage you to explore these methods further, practice implementing them in your own worksheets, and share your experiences or questions in the comments below. By doing so, you'll not only improve your Excel skills but also contribute to a community of learners and professionals seeking to leverage the full potential of Excel VBA.