Intro
Learn 5 ways to remove trailing characters, spaces, and zeros in strings, using efficient methods and tools for data cleansing and string manipulation, including trimming and stripping techniques.
Removing trailing characters or spaces from a string is a common task in various programming languages and text editing scenarios. It is essential for data cleaning, ensuring consistency in formatting, and preventing errors that might arise from unwanted characters at the end of strings. This process can be achieved through different methods, depending on the context and the tools available. Here, we'll explore five ways to remove trailing characters or spaces, focusing on general approaches that can be adapted to various situations.
The importance of removing trailing characters cannot be overstated. In programming, these unwanted characters can lead to bugs, errors in data processing, or issues with data comparison and validation. In text editing, trailing spaces can affect the layout, especially when dealing with formatting-sensitive documents or web pages. Furthermore, in data analysis and science, clean data is crucial for accurate analysis and modeling, making the removal of trailing characters a preliminary step in data preprocessing.
Understanding the context in which trailing characters need to be removed is crucial. Different programming languages and software tools offer various methods and functions to achieve this. For instance, in programming languages like Python, JavaScript, and Java, there are built-in string methods that can trim or remove trailing characters. Similarly, in text editors and spreadsheet software like Microsoft Excel, there are specific functions and formulas that can be used to remove unwanted trailing spaces or characters.
Using String Methods in Programming Languages
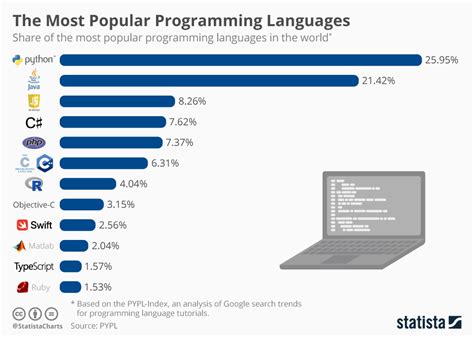
One of the most straightforward ways to remove trailing characters in programming is by using the built-in string methods provided by the language. For example, in Python, the strip()
, rstrip()
, and lstrip()
methods can be used to remove leading, trailing, or both leading and trailing characters from a string. The strip()
method removes both leading and trailing characters (spaces are default), lstrip()
removes leading characters, and rstrip()
removes trailing characters.
Example in Python
In Python, you can remove trailing spaces from a string using the `rstrip()` method. Here's an example: ```python my_string = "Hello, World! " clean_string = my_string.rstrip() print(clean_string) # Outputs: "Hello, World!" ```Regular Expressions for Complex Patterns
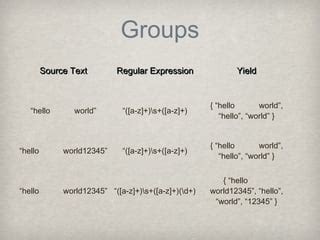
Regular expressions (regex) offer a powerful way to search for patterns in strings and can be used to remove trailing characters that match specific criteria. This method is particularly useful when dealing with complex patterns or when the trailing characters are not just spaces. In JavaScript, for example, you can use the replace()
method with a regex pattern to remove trailing characters.
Example in JavaScript
To remove trailing digits from a string in JavaScript, you can use the following regex: ```javascript let myString = "Hello123"; let cleanString = myString.replace(/[0-9]+$/, ''); console.log(cleanString); // Outputs: "Hello" ```Text Editing Software
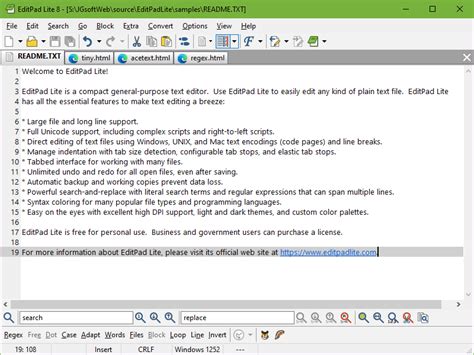
In text editing software like Notepad++, Sublime Text, or even Microsoft Word, there are often built-in functions or plugins available that can remove trailing spaces from documents. For instance, in Notepad++, you can use the "Trim Trailing and save" option under the "TextFX" menu to remove trailing spaces from the entire document.