Intro
Learn 2 ways to remove characters from strings, including trimming and replacing, to improve text data quality with efficient string manipulation techniques.
The art of removing characters from a string is a crucial skill in programming, and it can be achieved in multiple ways. In this article, we will delve into the world of string manipulation and explore two primary methods for removing characters from a string. Whether you are a seasoned developer or just starting out, this article will provide you with a comprehensive understanding of the techniques involved.
The importance of removing characters from a string cannot be overstated. In many applications, strings are used to store and manipulate data, and the ability to remove unwanted characters is essential for ensuring data accuracy and integrity. From removing punctuation and special characters to deleting entire substrings, the techniques outlined in this article will equip you with the skills necessary to tackle even the most complex string manipulation tasks.
In the world of programming, strings are a fundamental data type, and the ability to manipulate them is essential for any developer. The two methods for removing characters from a string that we will explore in this article are the use of the replace() method and the use of regular expressions. Both methods have their strengths and weaknesses, and understanding when to use each is crucial for effective string manipulation.
Method 1: Using the Replace() Method
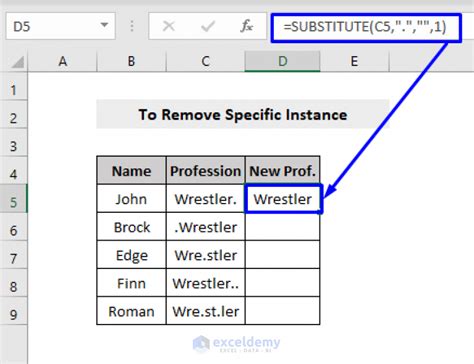
For example, if we want to remove all occurrences of the character "a" from the string "banana", we can use the replace() method as follows:
string = "banana"
new_string = string.replace("a", "")
print(new_string) # Output: "bnn"
As you can see, the replace() method is a simple and effective way to remove characters from a string. However, it has some limitations, such as only replacing the first occurrence of the character if the second argument is not specified.
Method 2: Using Regular Expressions

For example, if we want to remove all non-alphanumeric characters from a string, we can use the following regex pattern:
import re
string = "Hello, World! 123"
new_string = re.sub(r'\W+', '', string)
print(new_string) # Output: "HelloWorld123"
In this example, the regex pattern \W+
matches one or more non-alphanumeric characters, and the replace() method replaces these characters with an empty string, effectively removing them from the original string.
Benefits of Using Regular Expressions
Regular expressions offer several benefits when it comes to removing characters from a string. They provide a flexible and powerful way to search for patterns in strings and can be used to remove complex patterns that would be difficult to achieve using the replace() method alone.Some of the benefits of using regular expressions include:
- Flexibility: Regular expressions can be used to search for a wide range of patterns in strings, from simple characters to complex patterns.
- Power: Regular expressions provide a powerful way to manipulate strings and can be used to perform complex operations such as validation and extraction.
- Efficiency: Regular expressions can be more efficient than using the replace() method alone, especially when dealing with large strings or complex patterns.
Common Use Cases for Removing Characters
Removing characters from a string is a common operation in many applications, and there are several use cases where this technique is particularly useful. Some of the most common use cases include:- Data cleaning: Removing unwanted characters from data is an essential step in data cleaning and preprocessing.
- Text processing: Removing characters from text is a common operation in text processing and natural language processing applications.
- Validation: Removing characters from input data is often used as a form of validation to ensure that the data conforms to a specific format or pattern.
Best Practices for Removing Characters
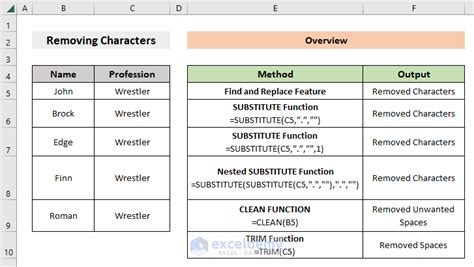
- Use the replace() method for simple replacements: The replace() method is a simple and efficient way to remove characters from a string, and it should be used for simple replacements.
- Use regular expressions for complex patterns: Regular expressions provide a powerful way to search for complex patterns in strings and should be used for more complex operations.
- Test your code: It is essential to test your code thoroughly to ensure that it is working as expected and that it is not introducing any unintended consequences.
Common Mistakes to Avoid
When removing characters from a string, there are several common mistakes to avoid. Some of the most common mistakes include:- Using the wrong method: Using the wrong method for removing characters can lead to unexpected results and can make your code more complex than it needs to be.
- Not testing your code: Failing to test your code thoroughly can lead to bugs and unintended consequences.
- Not considering edge cases: Failing to consider edge cases can lead to bugs and unintended consequences.
Remove Characters Image Gallery
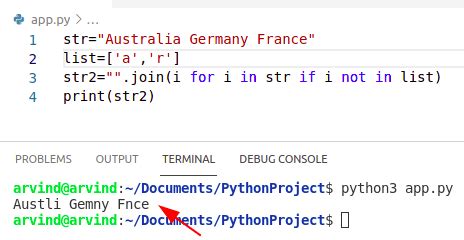
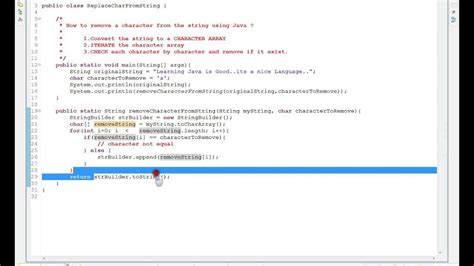
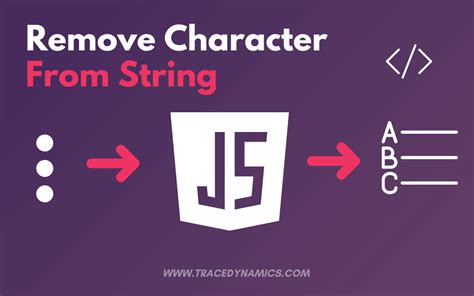
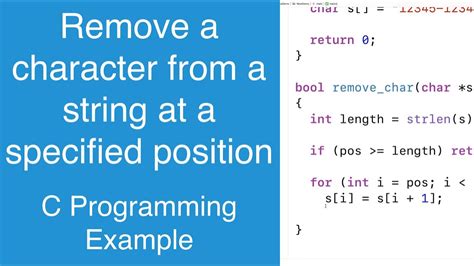
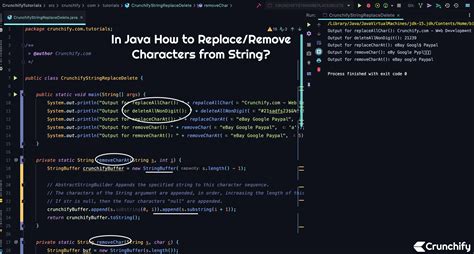
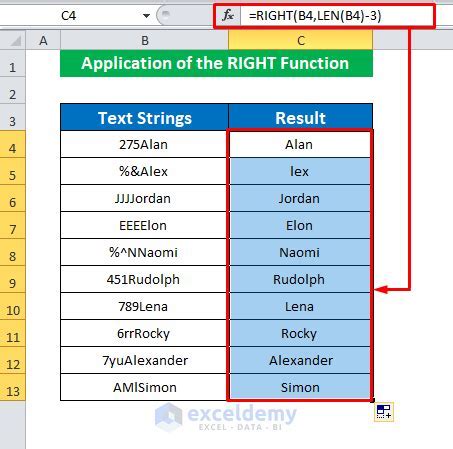
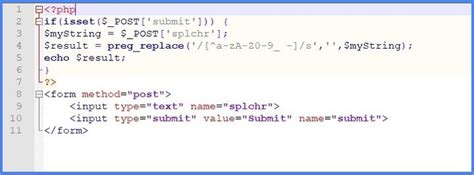
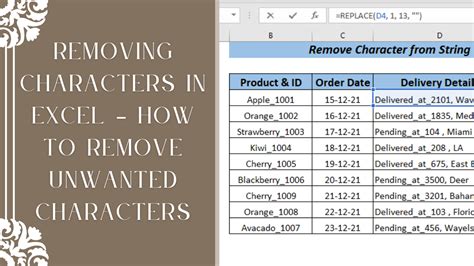
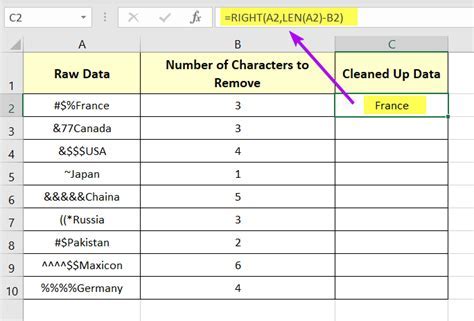
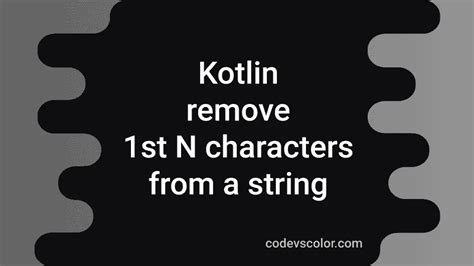
What is the best way to remove characters from a string?
+The best way to remove characters from a string depends on the specific use case and the programming language being used. The replace() method and regular expressions are two common methods used to remove characters from a string.
How do I remove all non-alphanumeric characters from a string?
+You can remove all non-alphanumeric characters from a string using regular expressions. The regex pattern \W+ matches one or more non-alphanumeric characters, and the replace() method can be used to replace these characters with an empty string.
What are some common use cases for removing characters from a string?
+Some common use cases for removing characters from a string include data cleaning, text processing, and validation. Removing characters from a string can be used to ensure that data conforms to a specific format or pattern.
How do I test my code to ensure that it is working correctly?
+You can test your code by using a combination of unit tests and integration tests. Unit tests can be used to test individual functions or methods, while integration tests can be used to test how different components of your code work together.
What are some common mistakes to avoid when removing characters from a string?
+Some common mistakes to avoid when removing characters from a string include using the wrong method, not testing your code, and not considering edge cases. Using the wrong method can lead to unexpected results, while not testing your code can lead to bugs and unintended consequences.
In conclusion, removing characters from a string is a crucial operation in many applications, and there are several methods that can be used to achieve this. The replace() method and regular expressions are two common methods used to remove characters from a string, and each has its strengths and weaknesses. By understanding the different methods available and how to use them effectively, you can ensure that your code is efficient, effective, and easy to maintain. Whether you are a seasoned developer or just starting out, this article has provided you with a comprehensive understanding of the techniques involved in removing characters from a string. We hope that you have found this article informative and helpful, and we encourage you to share your thoughts and experiences in the comments below.