Intro
Clear Excel clipboard with VBA, optimizing spreadsheet performance by deleting cached data, and improving macro functionality with related keywords like Excel VBA codes and clipboard management.
The clipboard in Excel is a useful tool that allows users to copy and paste data, formulas, and even charts and images. However, when working with VBA (Visual Basic for Applications), it's often necessary to clear the clipboard to prevent unintended pasting of data or to free up system resources. In this article, we'll explore how to clear the Excel clipboard using VBA.
Excel's clipboard is a temporary storage area that holds the data or object that you've copied or cut. When you copy or cut something in Excel, it's stored in the clipboard, and you can then paste it into another location. However, if you're working with VBA, you may need to clear the clipboard to prevent it from interfering with your code or to ensure that your code runs smoothly.
Why Clear the Clipboard?
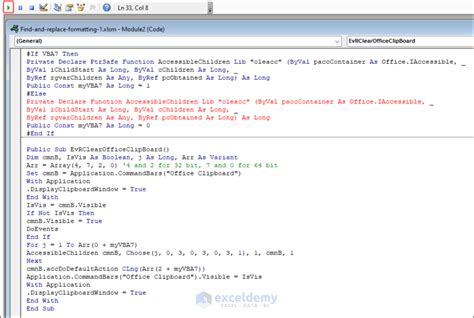
There are several reasons why you might want to clear the clipboard in Excel using VBA. Here are a few:
- Prevent unintended pasting: If you're working with VBA and you've copied some data or an object, you might accidentally paste it into the wrong location. By clearing the clipboard, you can prevent this from happening.
- Free up system resources: If you're working with large amounts of data or complex objects, copying and pasting can consume a lot of system resources. Clearing the clipboard can help free up these resources and improve performance.
- Ensure code runs smoothly: If you're running a VBA script that involves copying and pasting data, clearing the clipboard can help ensure that the code runs smoothly and without errors.
How to Clear the Clipboard in VBA
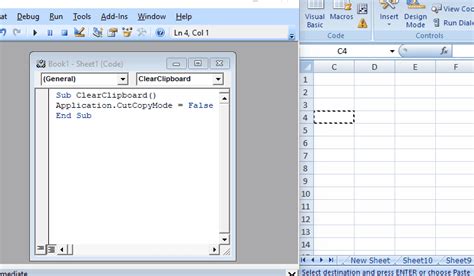
To clear the clipboard in VBA, you can use the following code:
Sub ClearClipboard()
Dim obj As New MSForms.DataObject
obj.Clear
Set obj = Nothing
End Sub
This code creates a new instance of the MSForms.DataObject
class, which represents the clipboard. The Clear
method is then used to clear the clipboard, and the object is set to Nothing
to release any system resources.
You can also use the following code to clear the clipboard:
Sub ClearClipboard()
Application.CutCopyMode = False
End Sub
This code sets the CutCopyMode
property of the Application
object to False
, which clears the clipboard.
Example Use Cases
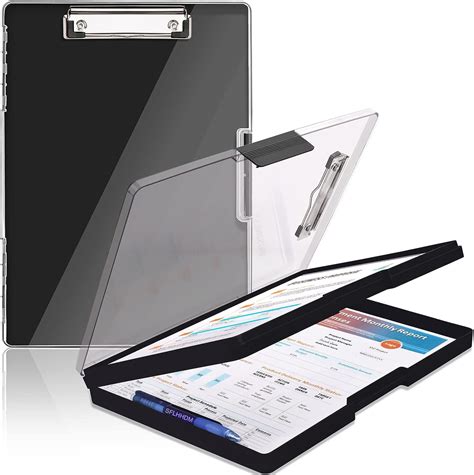
Here are a few example use cases for clearing the clipboard in VBA:
- Automating tasks: If you're automating tasks in Excel using VBA, you might want to clear the clipboard at the beginning of each task to ensure that the task runs smoothly and without errors.
- Working with large datasets: If you're working with large datasets in Excel, you might want to clear the clipboard periodically to free up system resources and improve performance.
- Creating custom add-ins: If you're creating custom add-ins for Excel using VBA, you might want to clear the clipboard as part of the add-in's initialization routine to ensure that the add-in runs smoothly and without errors.
Best Practices for Clearing the Clipboard
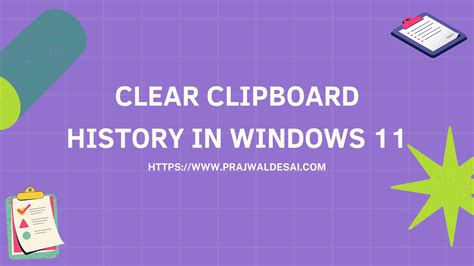
Here are a few best practices to keep in mind when clearing the clipboard in VBA:
- Clear the clipboard periodically: If you're working with large datasets or complex objects, it's a good idea to clear the clipboard periodically to free up system resources and improve performance.
- Use the
Clear
method: TheClear
method is the most reliable way to clear the clipboard in VBA. Avoid using other methods, such as setting theCutCopyMode
property toFalse
, as they may not work in all situations. - Test your code: Before deploying your VBA code, make sure to test it thoroughly to ensure that it works as expected and doesn't cause any unintended consequences.
Common Errors and Troubleshooting
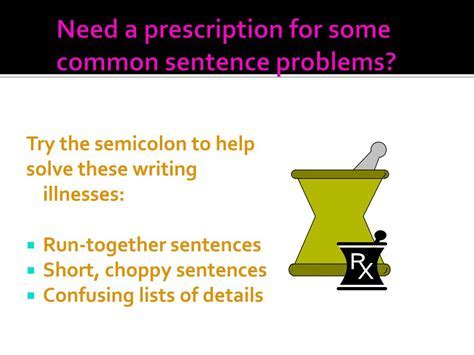
Here are a few common errors and troubleshooting tips to keep in mind when clearing the clipboard in VBA:
- Error 1004: This error occurs when the
Clear
method is called on an object that doesn't support it. Make sure to check the object's documentation to ensure that it supports theClear
method. - Error 438: This error occurs when the
Clear
method is called on an object that doesn't exist. Make sure to check that the object exists before calling theClear
method. - Clipboard not clearing: If the clipboard is not clearing as expected, make sure to check that the
Clear
method is being called correctly and that the object is being set toNothing
to release any system resources.
Clear Excel Clipboard Image Gallery
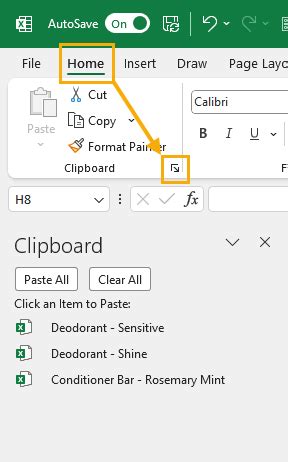

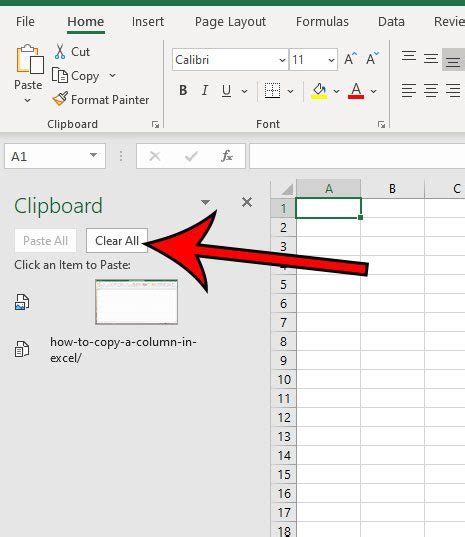
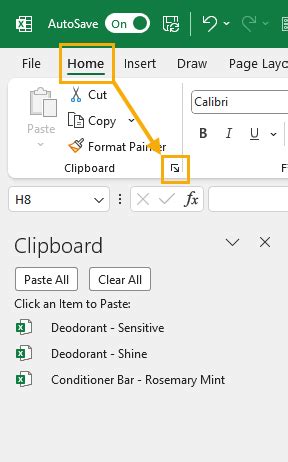
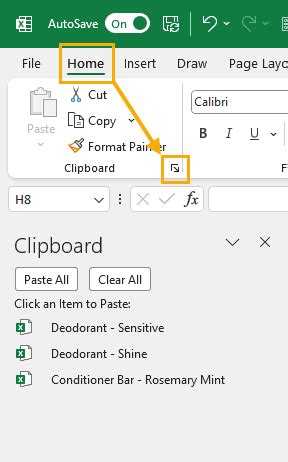

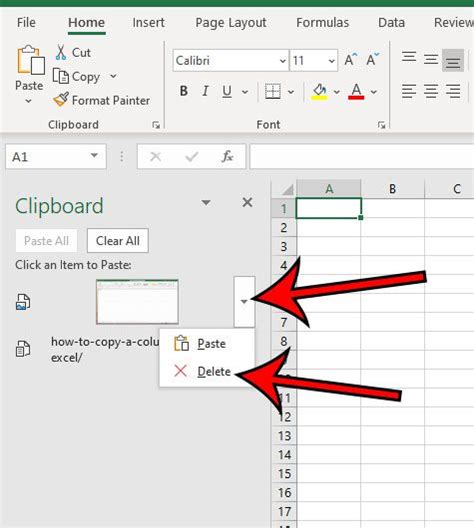
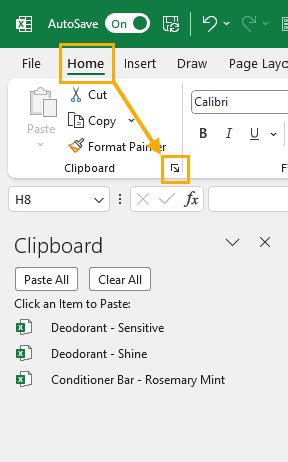
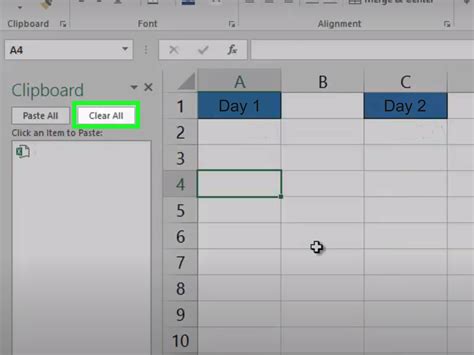
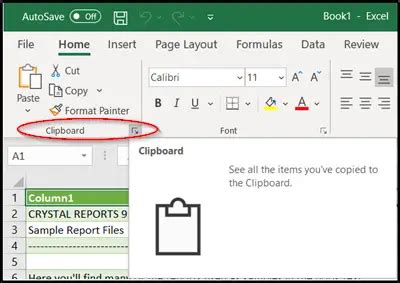
What is the purpose of clearing the clipboard in VBA?
+The purpose of clearing the clipboard in VBA is to prevent unintended pasting of data, free up system resources, and ensure that code runs smoothly.
How do I clear the clipboard in VBA?
+You can clear the clipboard in VBA by using the `Clear` method of the `MSForms.DataObject` class or by setting the `CutCopyMode` property of the `Application` object to `False`.
What are some common errors that occur when clearing the clipboard in VBA?
+Some common errors that occur when clearing the clipboard in VBA include Error 1004 and Error 438. These errors can be caused by calling the `Clear` method on an object that doesn't support it or by calling the `Clear` method on an object that doesn't exist.
If you have any questions or need further assistance with clearing the Excel clipboard using VBA, don't hesitate to ask. You can leave a comment below or reach out to us through our contact page. We're always happy to help. Additionally, if you found this article helpful, please share it with others who may benefit from it. Thank you for reading!