Intro
Fix Java errors with ease, learn to handle else without if errors, and master exception handling, debugging, and coding best practices.
The "else without if" error in Java is a common issue that programmers encounter, especially when they are starting out with the language. This error occurs when the Java compiler encounters an "else" statement that is not associated with an "if" statement. In this article, we will delve into the reasons behind this error, how to identify it, and most importantly, how to fix it.
To understand the "else without if" error, it's essential to grasp the basic syntax and usage of "if" and "else" statements in Java. The "if" statement is used to execute a block of code if a certain condition is true. The "else" statement, on the other hand, is used in conjunction with the "if" statement to execute a different block of code if the condition is false.
Importance of Proper Syntax
The syntax of "if" and "else" statements in Java is straightforward. An "if" statement can stand alone, but an "else" statement must always be associated with an "if" statement. The general syntax is as follows:
if (condition) {
// code to be executed if condition is true
} else {
// code to be executed if condition is false
}
Causes of the Error
The "else without if" error typically arises from one of the following scenarios:
-
Missing or Misplaced If Statement: The most common cause is simply forgetting to include the "if" statement before the "else" statement or placing the "else" statement in a location where the compiler cannot associate it with an "if" statement.
-
Incorrect Bracketing: Java uses brackets
{}
to define blocks of code. If these brackets are not used correctly, it can lead to the compiler misinterpreting the structure of the "if" and "else" statements. -
Nested If-Else Statements: When using nested "if-else" statements, it can sometimes be confusing to match each "else" with its corresponding "if". This can lead to an "else" statement being associated with the wrong "if" statement or not being associated with any "if" statement at all.
Identifying the Error
When the Java compiler encounters an "else without if" error, it will typically report the error with a message indicating that it expected an "if" statement before the "else" statement. The exact wording of the error message may vary depending on the compiler being used.
Fixing the Error
To fix the "else without if" error, you need to ensure that every "else" statement is properly associated with an "if" statement. Here are some steps to follow:
-
Review Your Code: Carefully go through your code to identify the "else" statement that is causing the error.
-
Check for Missing If Statement: Make sure there is an "if" statement before the "else" statement. If the "if" statement is missing, add it.
-
Verify Bracketing: Ensure that the brackets
{}
are correctly used to define the blocks of code associated with the "if" and "else" statements. -
Correct Nested Statements: If you are using nested "if-else" statements, review them carefully to ensure that each "else" statement is correctly matched with its corresponding "if" statement.
Example
Here's a simple example of how the "else without if" error might occur and how to fix it:
// Incorrect code
int x = 5;
else {
System.out.println("x is less than 10");
}
// Corrected code
int x = 5;
if (x < 10) {
System.out.println("x is less than 10");
} else {
System.out.println("x is not less than 10");
}
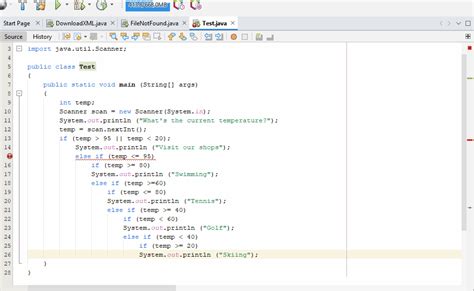
Best Practices
To avoid the "else without if" error, follow these best practices:
- Always ensure that every "else" statement is associated with an "if" statement.
- Use brackets
{}
consistently to define the scope of "if" and "else" blocks. - When using nested "if-else" statements, indent your code properly to make the structure clear.
Advanced Topics
For more complex scenarios involving "if-else" statements, such as using "else if" for multiple conditions or nested "if-else" statements, it's crucial to understand how the compiler interprets these structures. The key is to maintain clarity and readability in your code, which can be achieved through proper indentation, commenting, and bracketing.
Advanced If-Else Structures
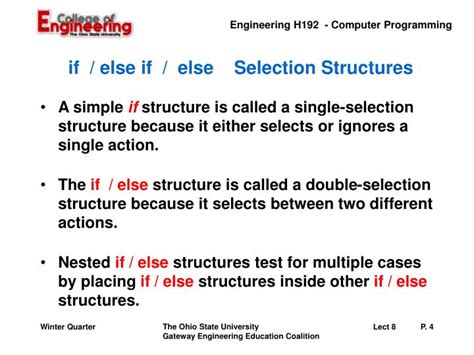
Conclusion and Next Steps
In conclusion, the "else without if" error in Java is a straightforward issue to resolve once you understand the causes and how to properly structure your "if" and "else" statements. By following the best practices outlined above and being mindful of the syntax and structure of your code, you can avoid this error and write more effective, error-free Java programs.
Practical Applications
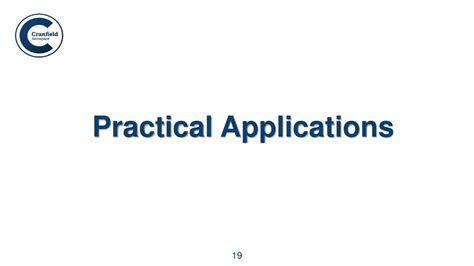
For those looking to dive deeper into Java programming and explore more advanced topics, there are numerous resources available, including tutorials, online courses, and comprehensive programming books. The key to mastering Java, like any programming language, is practice and persistence.
Further Learning
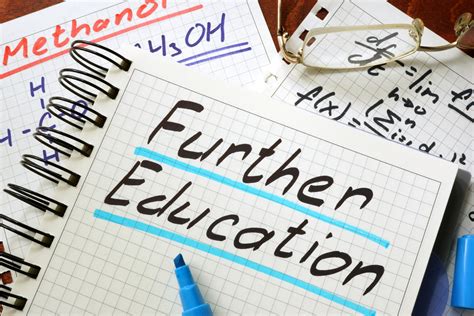
Engagement and Community
We invite you to share your experiences with the "else without if" error in Java, including any challenging scenarios you've faced and how you overcame them. Your insights can help others in the programming community. Feel free to comment below, share this article with fellow programmers, or take a moment to explore other related topics on our site.
Community Engagement
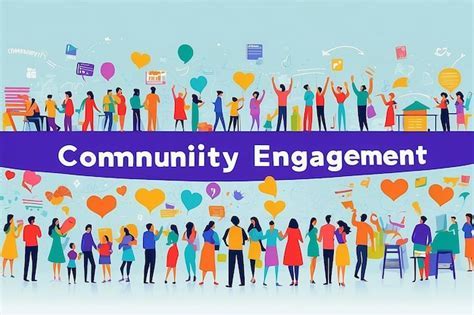
Gallery of Java Programming
Java Programming Image Gallery
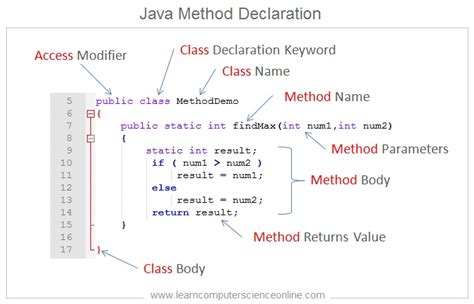
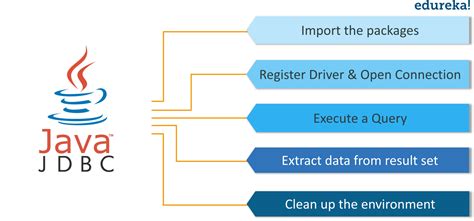
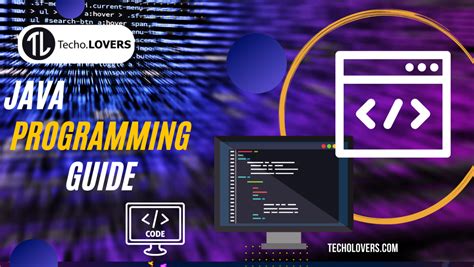
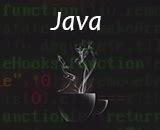
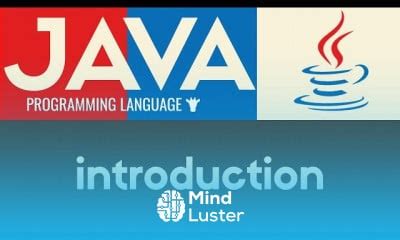
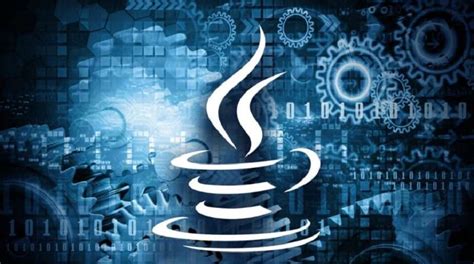
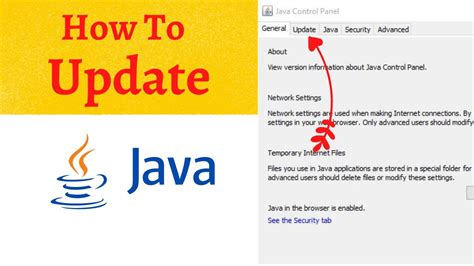
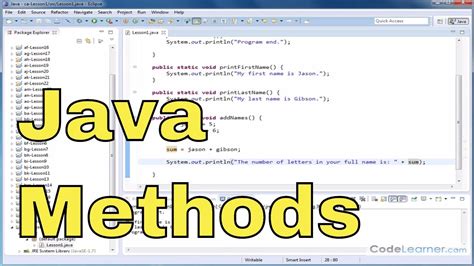
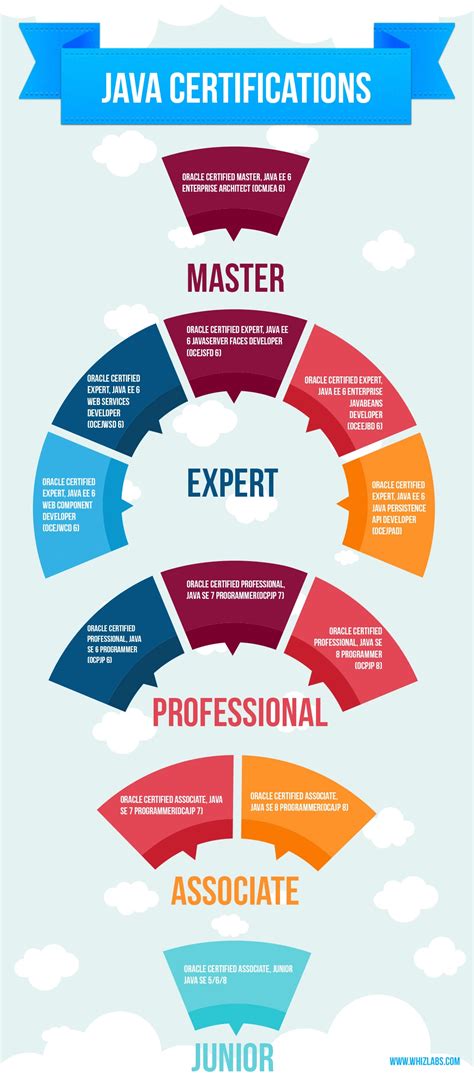
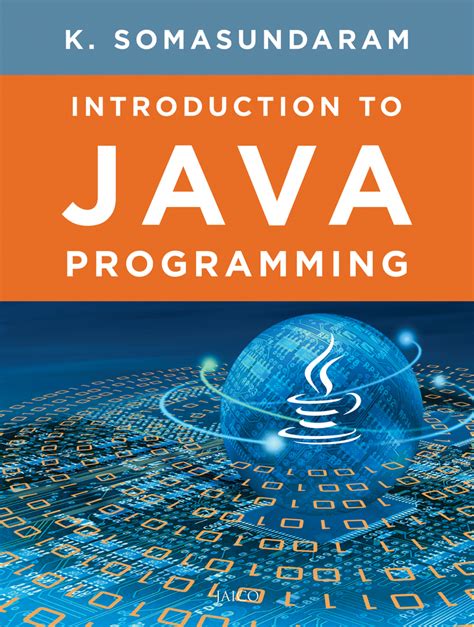
FAQs
What is the "else without if" error in Java?
+The "else without if" error occurs when the Java compiler encounters an "else" statement that is not associated with an "if" statement.
How do I fix the "else without if" error?
+To fix the error, ensure that every "else" statement is properly associated with an "if" statement, and verify that the brackets are correctly used.
What are some best practices to avoid the "else without if" error?
+Best practices include always associating "else" with "if", using brackets consistently, and maintaining code readability through proper indentation and commenting.