Intro
Discover 5 ways to concatenate strings, arrays, and data with ease, using techniques like looping, merging, and joining, to simplify data manipulation and improve coding efficiency.
In the realm of programming and data manipulation, concatenation stands out as a fundamental operation. It involves combining two or more strings, arrays, or other data structures into a single entity. This process is crucial in various applications, from building dynamic text outputs in web development to merging datasets in data analysis. Understanding the different methods to concatenate can significantly enhance one's ability to manipulate and transform data efficiently. Whether you're working with strings, arrays, or data frames, mastering concatenation techniques is indispensable for any aspiring programmer or data scientist.
The importance of concatenation cannot be overstated, as it allows for the creation of complex data structures from simpler ones, facilitating more sophisticated data analysis and presentation. For instance, in web development, concatenating strings can help in dynamically generating HTML content based on user input or database records. Similarly, in data science, concatenating arrays or data frames is essential for combining datasets from different sources, a common requirement in data preprocessing stages.
As technology advances and the volume of data grows, the need for efficient data manipulation techniques becomes more pressing. Concatenation, being a core operation, has seen various implementations across different programming languages and tools. Each method has its unique characteristics, advantages, and use cases, making it essential to explore and understand the different ways to concatenate data.
Introduction to Concatenation Methods
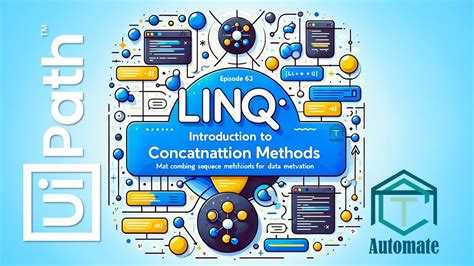
The world of concatenation is diverse, with methods ranging from simple string concatenation in programming languages like Python and Java, to more complex operations involving arrays and data frames in libraries such as Pandas and NumPy. Each of these methods serves a specific purpose and is tailored to handle different types of data and operational requirements.
1. String Concatenation
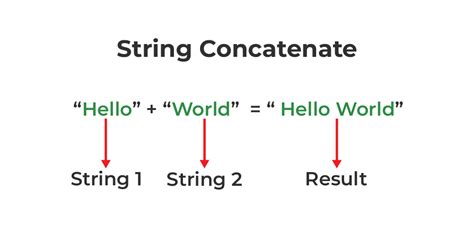
String concatenation is one of the most basic forms of concatenation and is supported by virtually all programming languages. It involves joining two or more strings together to form a new string. This operation can be performed using operators (like the "+" operator in Python or Java) or through built-in string methods.
For example, in Python, you can concatenate two strings "Hello" and "World" using the "+" operator:
greeting = "Hello" + " " + "World"
print(greeting) # Outputs: Hello World
Benefits of String Concatenation
The benefits of string concatenation include its simplicity and the ability to dynamically generate text based on variables or user inputs. However, for large-scale string manipulations, it can be less efficient due to the immutable nature of strings in many programming languages, leading to the creation of temporary strings during the concatenation process.2. Array Concatenation
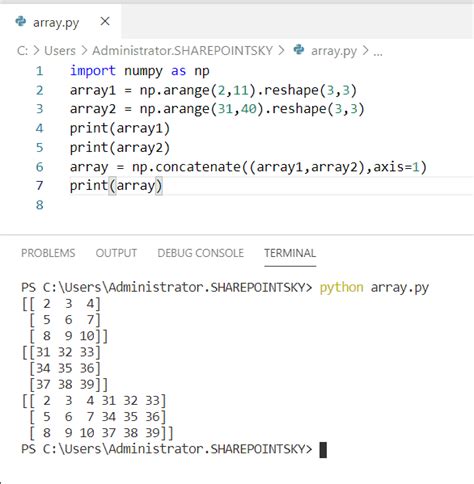
Array concatenation involves combining two or more arrays into a single array. This operation is crucial in data analysis and manipulation, especially when dealing with datasets from different sources. In JavaScript, for example, you can use the concat()
method to concatenate arrays:
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
let concatenatedArray = array1.concat(array2);
console.log(concatenatedArray); // Outputs: [1, 2, 3, 4, 5, 6]
Array Concatenation in Data Analysis
In the context of data analysis, array concatenation can be used to merge datasets. For instance, if you have two datasets representing sales figures from different regions, you can concatenate these arrays to get a comprehensive view of sales across all regions.3. Data Frame Concatenation

Data frame concatenation is a powerful operation in data science, particularly when working with libraries like Pandas in Python. It allows you to combine data frames either vertically (stacking) or horizontally (merging). The concat()
function in Pandas is used for this purpose:
import pandas as pd
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2'],
'C': ['C0', 'C1', 'C2'],
'D': ['D0', 'D1', 'D2']},
index=[0, 1, 2])
df2 = pd.DataFrame({'A': ['A3', 'A4', 'A5'],
'B': ['B3', 'B4', 'B5'],
'C': ['C3', 'C4', 'C5'],
'D': ['D3', 'D4', 'D5']},
index=[3, 4, 5])
concatenated_df = pd.concat([df1, df2])
print(concatenated_df)
Advantages of Data Frame Concatenation
The advantages of data frame concatenation include the ability to easily merge and manipulate large datasets, which is essential for data analysis and machine learning tasks. It provides a flexible way to handle data from different sources, allowing for efficient data preprocessing and preparation.4. Object Concatenation
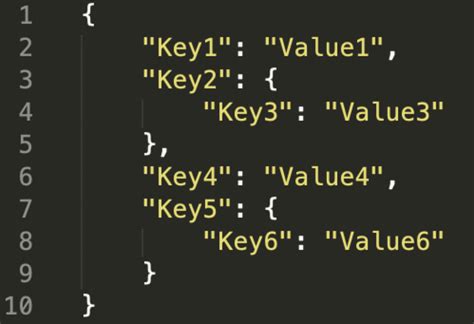
Object concatenation refers to the process of combining two or more objects into a single object. This can be particularly useful in JavaScript, where objects are commonly used to represent data:
let obj1 = {a: 1, b: 2};
let obj2 = {c: 3, d: 4};
let concatenatedObj = {...obj1,...obj2};
console.log(concatenatedObj); // Outputs: {a: 1, b: 2, c: 3, d: 4}
Use Cases for Object Concatenation
Object concatenation is useful in scenarios where you need to merge configuration objects, user data from different sources, or when working with state management in React applications.5. Concatenation in SQL

In SQL, concatenation is used to combine two or more strings into a single string. The syntax can vary between different SQL databases, with some using the CONCAT()
function, while others use the "+" operator or the "||" operator:
-- Using CONCAT() function
SELECT CONCAT('Hello', ' ', 'World') AS greeting;
-- Using || operator (in PostgreSQL)
SELECT 'Hello' || ' ' || 'World' AS greeting;
Importance of Concatenation in SQL
Concatenation in SQL is important for generating dynamic queries, creating reports, and manipulating data within the database itself. It allows for more flexible and powerful data manipulation and analysis directly within SQL queries.Concatenation Methods Image Gallery
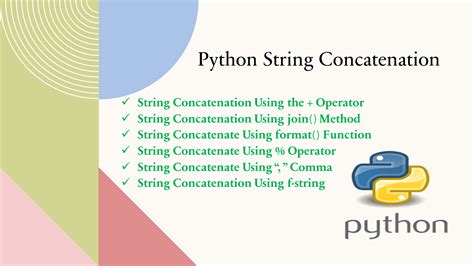
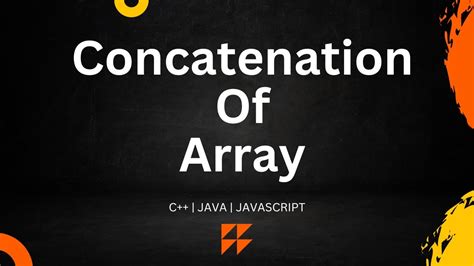
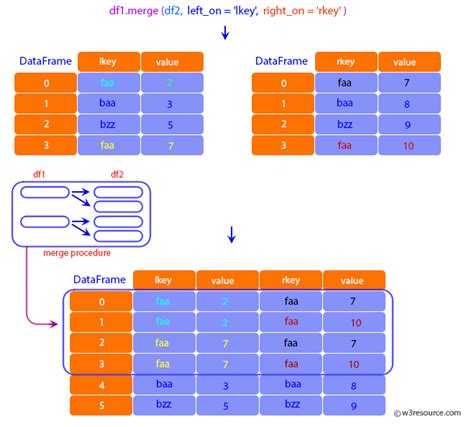

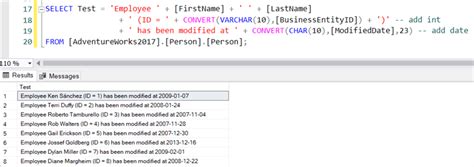

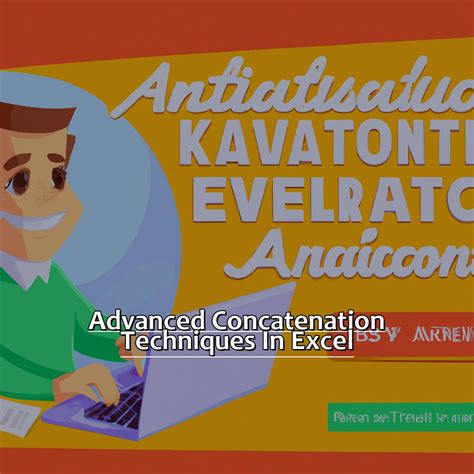
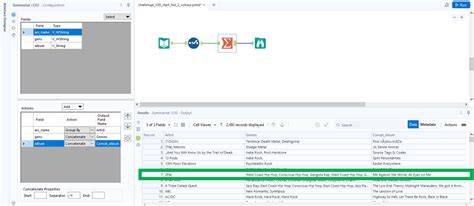
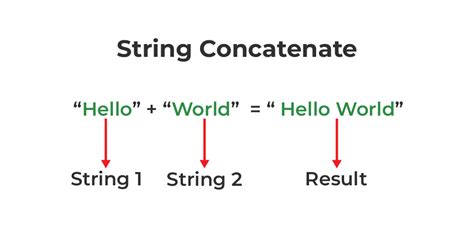
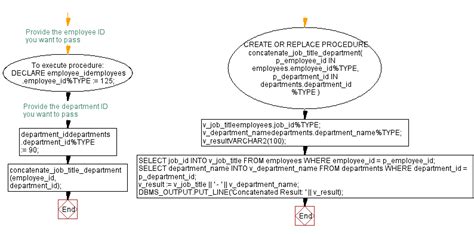
What is the purpose of concatenation in programming?
+Concatenation is used to combine two or more strings, arrays, or other data structures into a single entity, which is essential for various applications such as dynamic text generation, data analysis, and manipulation.
How does string concatenation differ from array concatenation?
+String concatenation involves joining strings, whereas array concatenation involves combining arrays. The methods and operators used for these operations can differ based on the programming language.
What are some common use cases for data frame concatenation?
+Data frame concatenation is commonly used in data analysis and science for merging datasets from different sources, creating comprehensive views of data, and preparing data for machine learning models.
In conclusion, mastering the art of concatenation is a vital skill for anyone involved in programming, data analysis, or any field that requires data manipulation. With its wide range of applications and the diversity of methods available across different programming languages and tools, understanding concatenation can significantly enhance one's ability to work with data efficiently. Whether you're concatenating strings, arrays, data frames, or objects, each method has its unique benefits and use cases, making it essential to explore and practice these techniques to become proficient in data manipulation and analysis. We invite you to share your thoughts on concatenation, its applications, and any challenges you've faced in the comments below, and don't forget to share this article with anyone who might benefit from learning about the powerful world of concatenation.