Intro
Discover how to count rows in Excel using VBA, including row counting methods, macros, and scripting techniques for efficient data management and automation.
When working with Excel VBA, being able to count rows is a fundamental task. It's essential for looping through data, applying changes, or simply understanding the scope of your dataset. Excel VBA provides several methods to count rows, each with its own use cases depending on what you're trying to achieve.
To begin with, let's consider the importance of accurately counting rows in Excel VBA. This skill is crucial for automating tasks, such as data analysis, report generation, and data manipulation. By mastering the art of row counting, you can create more efficient and dynamic VBA scripts that adapt to changing datasets.
Counting Rows in a Worksheet
One of the most common requirements is to count the number of rows used in a worksheet. This can be achieved in several ways:
1. Using Rows.Count
The Rows.Count
property returns the total number of rows in a worksheet. However, this method counts all rows, including empty ones, up to the maximum limit of Excel.
Sub CountAllRows()
Dim totalRows As Long
totalRows = Rows.Count
MsgBox "Total Rows: " & totalRows
End Sub
2. Using UsedRange.Rows.Count
The UsedRange
property returns a Range object that represents the used range in the worksheet. This method counts rows that have been used (i.e., contain data or formatting), which is often more useful than counting all rows.
Sub CountUsedRows()
Dim usedRows As Long
usedRows = UsedRange.Rows.Count
MsgBox "Used Rows: " & usedRows
End Sub
3. Finding the Last Row with Data
Often, you need to find the last row that contains data. This can be particularly useful for looping through data or applying formulas.
Sub FindLastRow()
Dim lastRow As Long
lastRow = Cells.Find(What:="*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious).Row
MsgBox "Last Row with Data: " & lastRow
End Sub
Practical Examples and Applications
Counting rows is not just about knowing how many rows you have; it's about applying that knowledge to real-world problems. Here are a few scenarios:
- Looping Through Data: If you need to perform an action on each row of data, knowing the last row with data is crucial.
Sub LoopThroughData()
Dim lastRow As Long
lastRow = Cells.Find(What:="*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious).Row
For i = 1 To lastRow
' Perform your action here
Cells(i, 1).Value = "Processed"
Next i
End Sub
- Dynamic Chart Updates: If you have a chart that needs to update dynamically based on the data range, counting rows helps you define the chart's data source range.
Sub UpdateChartSource()
Dim lastRow As Long
lastRow = Cells.Find(What:="*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious).Row
' Assuming your chart data is in column A
Range("A1:A" & lastRow).Select
' Update chart source here
End Sub
Best Practices and Common Mistakes
When counting rows in Excel VBA, keep the following best practices and common mistakes in mind:
- Avoid Hard-Coding: Unless you're certain your data will never exceed a certain number of rows, avoid hard-coding row numbers into your scripts.
- Use Meaningful Variable Names: Naming your variables (e.g.,
lastRowWithUserData
) can make your code easier to understand and maintain. - Test Your Code: Always test your code with different datasets to ensure it works as expected, especially when dealing with edge cases like empty worksheets or single-row datasets.
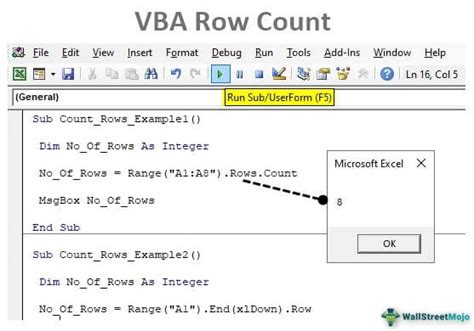
Advanced Techniques
For more complex scenarios, such as dealing with multiple worksheets, workbooks, or specific data types, you might need to employ more advanced techniques:
- Counting Rows Across Multiple Worksheets: If you need to count rows across all worksheets in a workbook, you can loop through each worksheet and sum up the used rows.
Sub CountRowsAcrossSheets()
Dim ws As Worksheet
Dim totalRows As Long
For Each ws In ThisWorkbook.Worksheets
totalRows = totalRows + ws.UsedRange.Rows.Count
Next ws
MsgBox "Total Rows Across All Sheets: " & totalRows
End Sub
- Handling Different Data Types: Depending on your data, you might need to count rows based on specific conditions, such as rows containing numbers, text, or dates.
Sub CountRowsWithNumbers()
Dim lastRow As Long
Dim count As Long
lastRow = Cells.Find(What:="*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious).Row
For i = 1 To lastRow
If IsNumeric(Cells(i, 1).Value) Then
count = count + 1
End If
Next i
MsgBox "Rows with Numbers: " & count
End Sub
Gallery of Excel VBA Rows Count
Excel VBA Rows Count Image Gallery
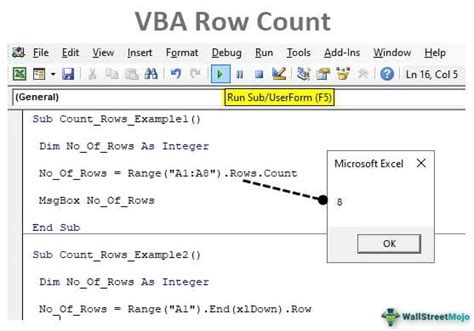
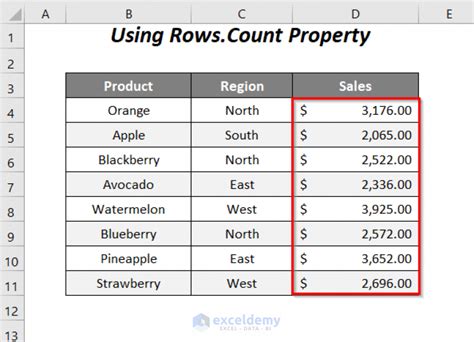
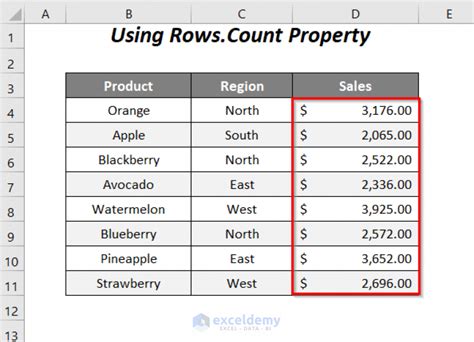
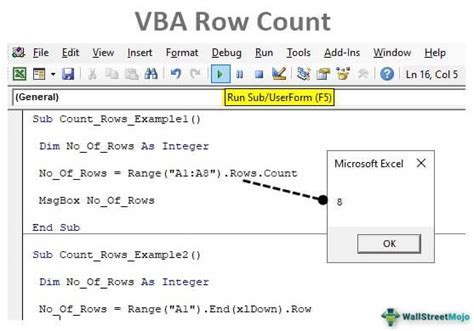
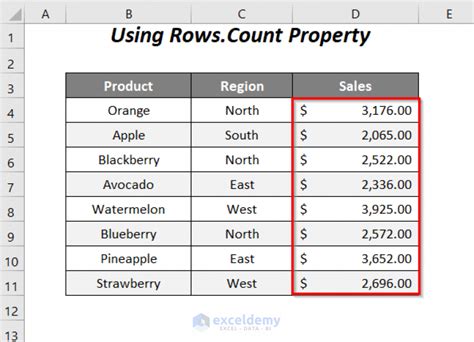
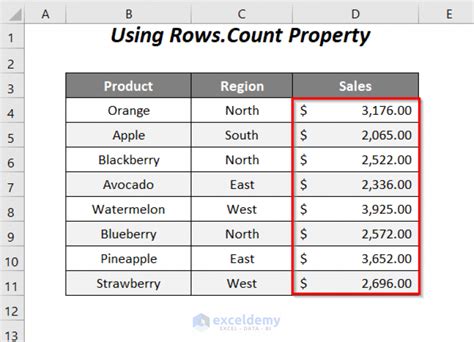
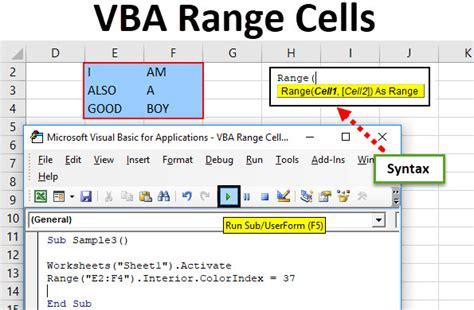
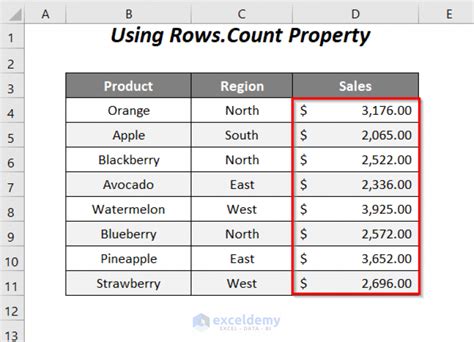
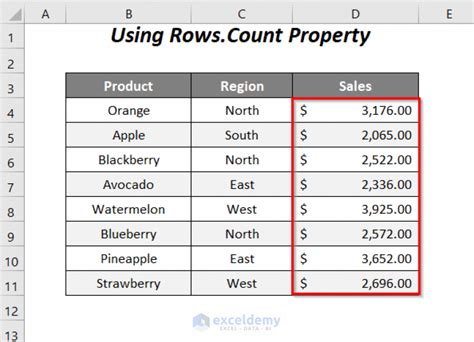
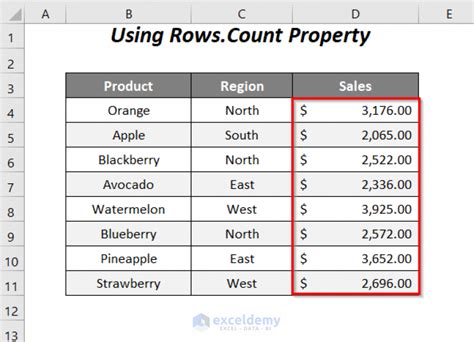
FAQs
What is the most efficient way to count rows in Excel VBA?
+The most efficient way often involves using the `UsedRange.Rows.Count` or finding the last row with data using `Cells.Find` method, depending on your specific needs.
How do I count rows across multiple worksheets in a workbook?
+You can achieve this by looping through each worksheet in the workbook and summing up the used rows in each sheet.
What are some common mistakes to avoid when counting rows in Excel VBA?
+Avoid hard-coding row numbers, use meaningful variable names, and always test your code with different datasets to ensure it works as expected.
As you delve into the world of Excel VBA, mastering the skill of counting rows will become increasingly important. Whether you're automating tasks, analyzing data, or simply organizing your worksheets, understanding how to efficiently count rows will make you more proficient and capable of handling complex tasks. Remember, practice makes perfect, so don't hesitate to experiment with different methods and techniques to find what works best for you.
In conclusion, the ability to count rows in Excel VBA is a fundamental skill that can significantly enhance your productivity and efficiency when working with spreadsheets. By following the guidelines, examples, and best practices outlined in this article, you'll be well on your way to becoming proficient in this essential aspect of Excel VBA programming.
Please feel free to share your thoughts, ask questions, or provide feedback on this article. Your input is invaluable in helping us create more informative and useful content for our readers.