Intro
The world of Excel VBA is vast and powerful, and one of the most useful tools within it is the Range.Find
method. This method allows you to search for specific data within a range of cells, making it incredibly useful for automating tasks and manipulating data. In this article, we'll delve into the details of how to use Range.Find
, its benefits, and provide practical examples to get you started.
When working with large datasets, finding specific information can be like looking for a needle in a haystack. That's where Range.Find
comes in – it enables you to quickly locate data, perform actions on it, and automate repetitive tasks. Whether you're a beginner or an advanced user, mastering Range.Find
can significantly enhance your productivity and efficiency in Excel.
Introduction to Range.Find
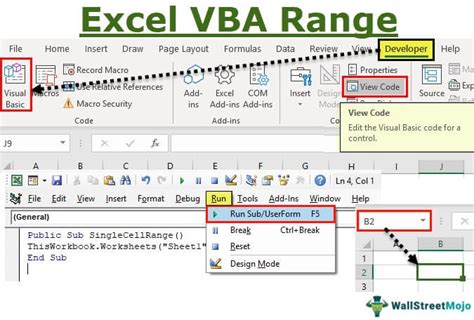
The Range.Find
method is part of the Excel VBA object model, which means it's accessible through Visual Basic for Applications, the programming language built into Excel. This method returns a Range
object that represents the first cell where the specified information is found. If no match is found, it returns Nothing
.
To use Range.Find
, you typically start by specifying the range of cells you want to search through. This can be a single cell, a row, a column, or any selection of cells. Then, you define what you're looking for, which can be a value, a format, or even a comment.
Basic Syntax of Range.Find
The basic syntax of `Range.Find` is as follows: ```vba Range.Find(What, After, LookIn, LookAt, SearchOrder, SearchDirection, MatchCase, MatchByte, SearchFormat) ``` - `What`: The value to search for. It can be a string, a number, or a date. - `After`: The cell after which to begin the search. This argument is useful for searching from a specific point in the range. - `LookIn`: Specifies whether to search in formulas, values, or comments. - `LookAt`: Determines whether to match the entire cell contents or just a part of it. - `SearchOrder`: Specifies whether to search by rows or columns. - `SearchDirection`: Determines the direction of the search (either forward or backward). - `MatchCase`: A boolean indicating whether the search should be case-sensitive. - `MatchByte`: Used for international support, this argument is not commonly used in English-speaking environments. - `SearchFormat`: Allows searching for specific formats.Practical Examples of Using Range.Find
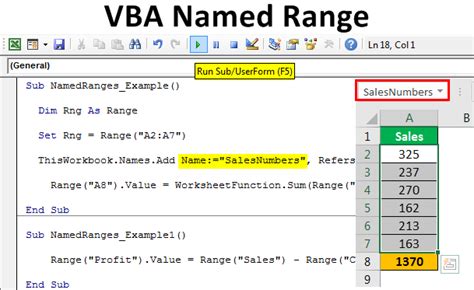
Let's look at a few examples to illustrate how Range.Find
can be used in real-world scenarios.
Example 1: Finding a Specific Value
Suppose you have a list of names in column A and you want to find a specific name, "John Doe".
Sub FindName()
Dim foundCell As Range
Set foundCell = Range("A1:A100").Find(What:="John Doe", LookIn:=xlValues)
If Not foundCell Is Nothing Then
MsgBox "Found at " & foundCell.Address
Else
MsgBox "Not found"
End If
End Sub
Example 2: Searching for a Format
You can also use Range.Find
to search for cells with a specific format, such as cells filled with a certain color.
Sub FindFormat()
Dim foundCell As Range
Set foundCell = Range("A1:A100").Find(What:="", SearchFormat:=True, LookIn:=xlFormatConditions)
If Not foundCell Is Nothing Then
MsgBox "Found at " & foundCell.Address
Else
MsgBox "Not found"
End If
End Sub
Benefits of Using Range.Find
The benefits of using `Range.Find` are numerous: - **Efficiency**: It saves time by automating the search process. - **Accuracy**: Reduces the chance of human error in finding data. - **Flexibility**: Can be used in a variety of scenarios, from simple value searches to complex format searches.Common Challenges and Solutions
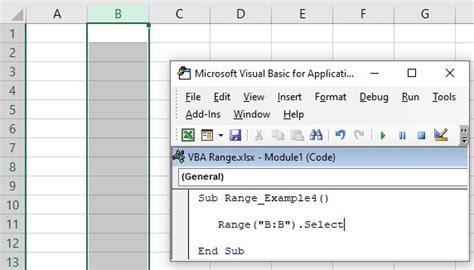
While Range.Find
is a powerful tool, there are some common challenges users face:
- Nothing is Found: Ensure that the
LookIn
parameter is correctly set (e.g.,xlValues
orxlFormulas
) and that the search value matches the data type in the cells. - Incorrect Results: Check the
LookAt
parameter. Setting it toxlWhole
will match the entire cell contents, whilexlPart
will match any part of the cell contents.
Tips for Effective Use
- **Specify the Search Range**: Clearly define the range you're searching within to avoid unnecessary searches and improve performance. - **Understand the Parameters**: Take the time to understand what each parameter does, especially `LookIn`, `LookAt`, and `SearchOrder`.Conclusion and Next Steps
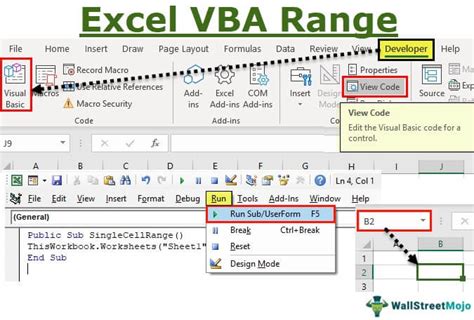
Mastering the Range.Find
method in Excel VBA can significantly enhance your ability to automate tasks and manipulate data. Whether you're dealing with simple datasets or complex spreadsheets, understanding how to effectively use Range.Find
can save you time and increase your productivity.
To further your learning, practice using Range.Find
in different scenarios, exploring its various parameters and how they can be combined to achieve specific goals. Additionally, consider delving into other Excel VBA methods and objects to expand your skill set and tackle more complex challenges.
Excel VBA Range Find Gallery
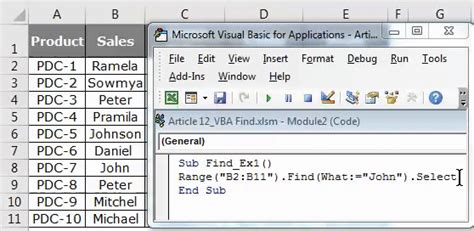
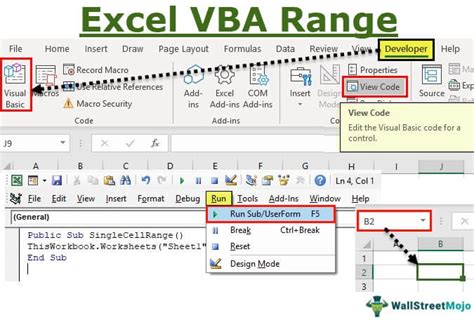
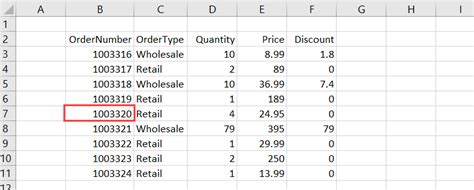
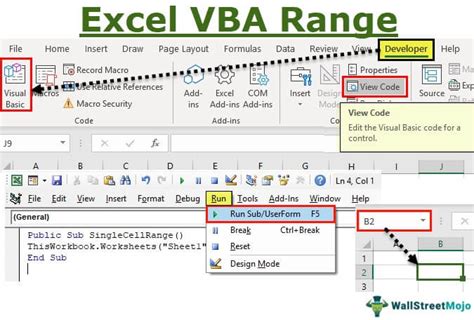
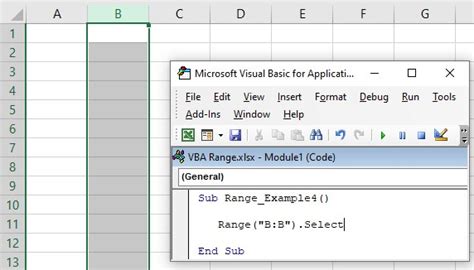
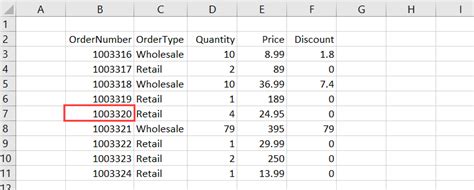
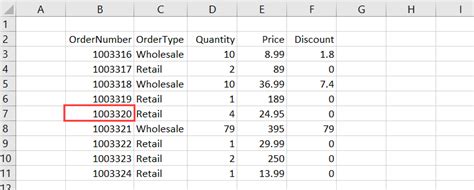
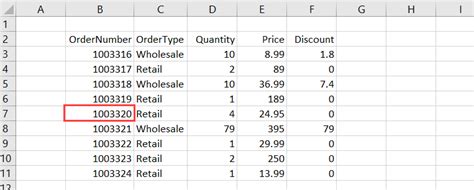
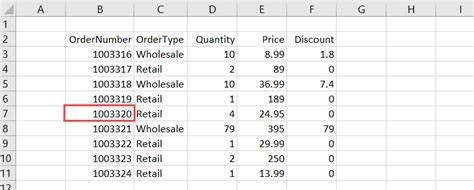
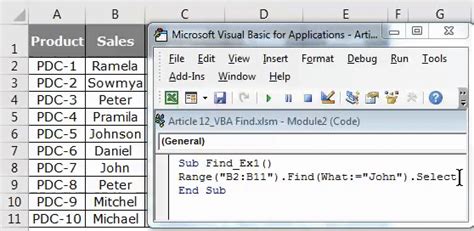
What is the purpose of the Range.Find method in Excel VBA?
+The Range.Find method is used to search for specific data within a range of cells in Excel, allowing for automation of tasks and manipulation of data.
How do I specify the search range in Range.Find?
+You specify the search range by defining the range of cells you want to search through, such as Range("A1:A100").
What is the difference between xlWhole and xlPart in the LookAt parameter?
+xlWhole matches the entire cell contents, while xlPart matches any part of the cell contents.
We hope this comprehensive guide to Range.Find
in Excel VBA has been informative and helpful. Whether you're just starting out with VBA or looking to expand your skills, mastering this method can open up new possibilities for automating tasks and working with data in Excel. Share your experiences, ask questions, or suggest topics for future articles in the comments below.