Intro
Learn how to clear clipboard using Excel VBA, optimizing workflow with macros, and related automation techniques for efficient data management and clipboard control.
Excel VBA is a powerful tool that allows users to automate tasks and create custom solutions within Excel. One common task that users may need to perform is clearing the clipboard. The clipboard is a temporary storage area that holds data that has been copied or cut from a document or other source. When you copy or cut data, it is stored in the clipboard until you paste it into a document or overwrite it with new data.
Clearing the clipboard can be useful in a variety of situations. For example, if you have sensitive data that you have copied or cut, you may want to clear the clipboard to prevent it from being accidentally pasted into another document. Additionally, if you are working with large amounts of data, clearing the clipboard can help to free up system resources and improve performance.
Fortunately, Excel VBA provides a simple way to clear the clipboard. You can use the following code to clear the clipboard:
Sub ClearClipboard()
Dim obj As New MSForms.DataObject
obj.Clear
Set obj = Nothing
End Sub
This code creates a new instance of the MSForms.DataObject
class, which represents the clipboard. The Clear
method is then used to clear the contents of the clipboard. Finally, the Set obj = Nothing
statement is used to release the object and free up system resources.
You can also use the following code to clear the clipboard:
Sub ClearClipboard()
Application.CutCopyMode = False
End Sub
This code sets the CutCopyMode
property of the Application
object to False
, which clears the clipboard.
You can call these macros from a button or shortcut to quickly clear the clipboard. Alternatively, you can add them to your Excel workbook's code module and run them as needed.
It's worth noting that these macros will only clear the clipboard within the Excel application. If you have copied or cut data from another application, these macros will not clear that data from the clipboard.
Here are some additional examples of how you can use Excel VBA to work with the clipboard:
- To copy data to the clipboard, you can use the
Range.Copy
method. For example:Range("A1").Copy
- To paste data from the clipboard, you can use the
Range.Paste
method. For example:Range("B1").Paste
- To check if the clipboard contains data, you can use the
Application.CutCopyMode
property. For example:If Application.CutCopyMode = True Then
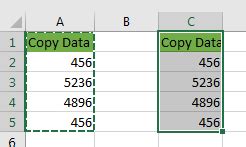
Benefits of Clearing the Clipboard
Clearing the clipboard can have several benefits, including:- Improved security: By clearing the clipboard, you can prevent sensitive data from being accidentally pasted into another document.
- Improved performance: Clearing the clipboard can help to free up system resources and improve performance, especially when working with large amounts of data.
- Reduced errors: Clearing the clipboard can help to prevent errors that can occur when data is accidentally pasted into the wrong location.
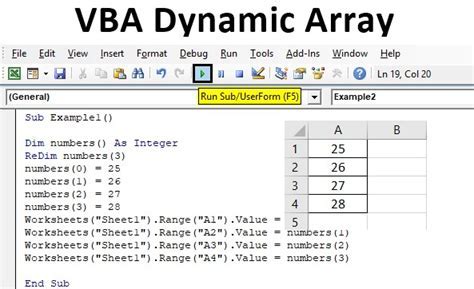
Common Uses of the Clipboard
The clipboard is a versatile tool that can be used in a variety of ways. Some common uses of the clipboard include:- Copying and pasting data between documents or applications
- Moving data from one location to another
- Storing temporary data that needs to be used later
- Sharing data between applications or users
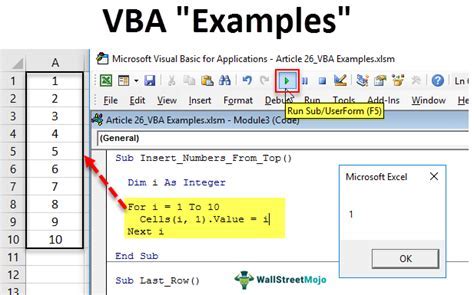
Best Practices for Working with the Clipboard
Here are some best practices to keep in mind when working with the clipboard:- Always clear the clipboard when you are finished using it to prevent sensitive data from being accidentally pasted into another document.
- Use the
Application.CutCopyMode
property to check if the clipboard contains data before attempting to paste it. - Use the
Range.Copy
andRange.Paste
methods to copy and paste data, rather than using theApplication.Clipboard
object.
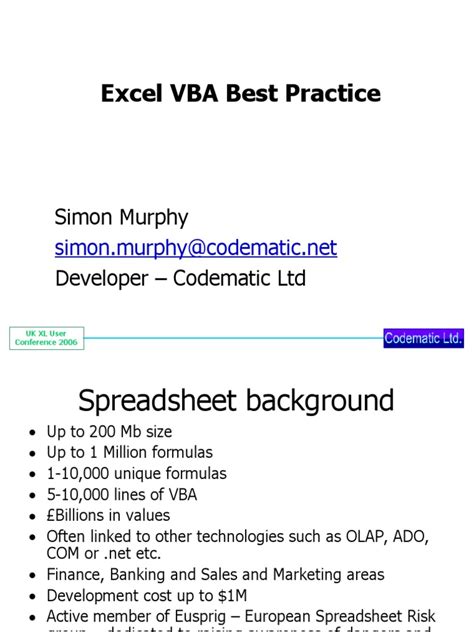
Troubleshooting Common Issues
Here are some common issues that you may encounter when working with the clipboard, along with some troubleshooting tips:- If the clipboard is not clearing, check to make sure that you are using the correct code to clear the clipboard.
- If data is not being copied or pasted correctly, check to make sure that the range you are trying to copy or paste is valid and that the data is in the correct format.
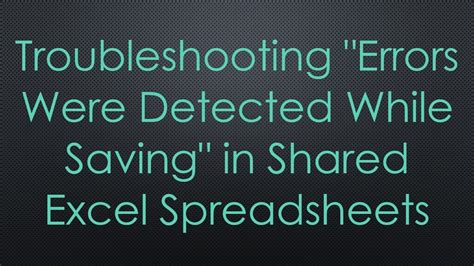
Conclusion and Final Thoughts
In conclusion, clearing the clipboard is an important task that can help to improve security, performance, and reduce errors. By using the `MSForms.DataObject` class or the `Application.CutCopyMode` property, you can easily clear the clipboard and prevent sensitive data from being accidentally pasted into another document. Remember to always follow best practices when working with the clipboard, and troubleshoot common issues as needed.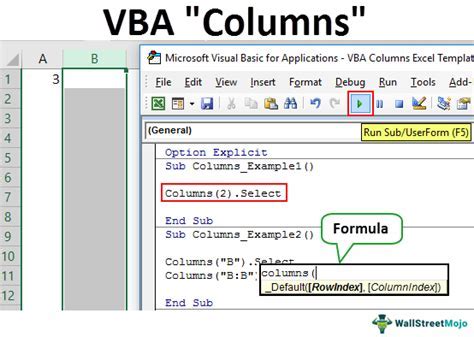
Excel VBA Gallery
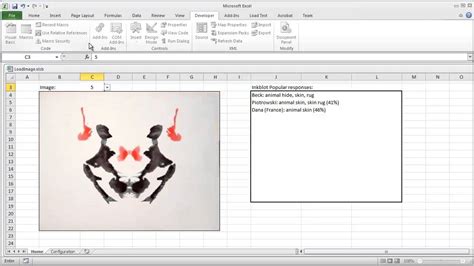

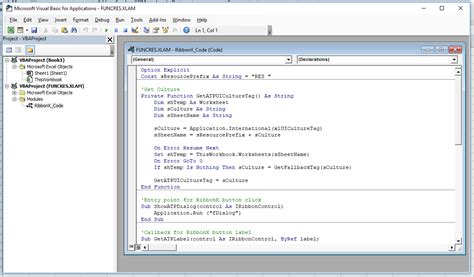
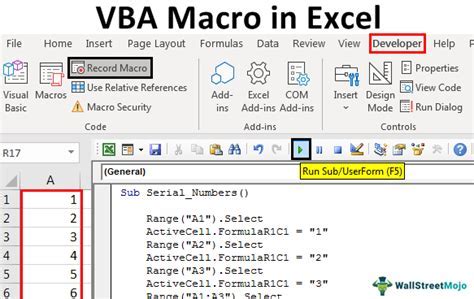
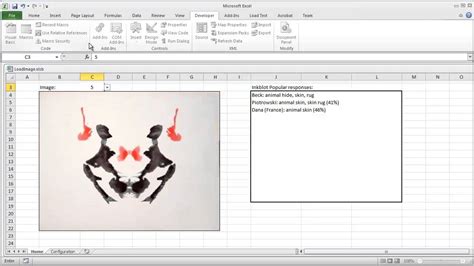
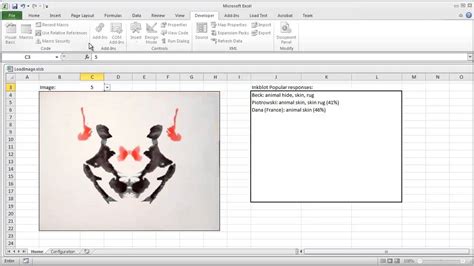
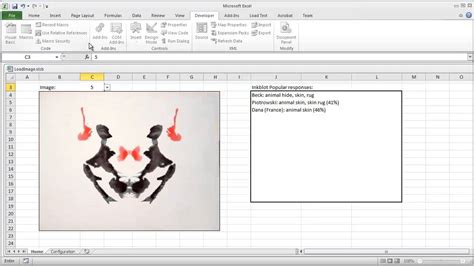
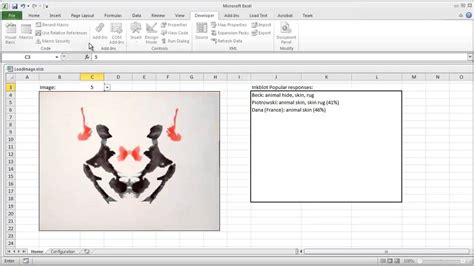
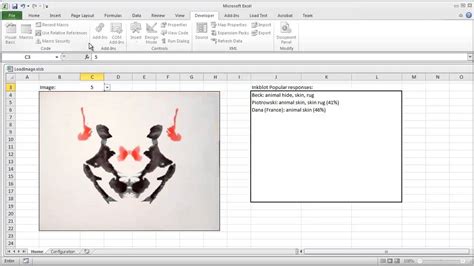
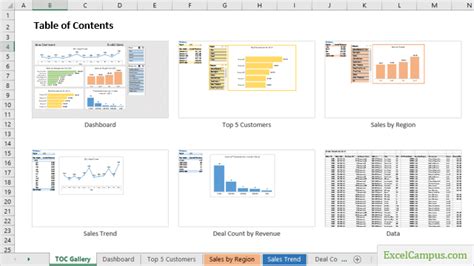
What is the purpose of clearing the clipboard in Excel VBA?
+The purpose of clearing the clipboard is to prevent sensitive data from being accidentally pasted into another document, and to improve performance by freeing up system resources.
How do I clear the clipboard in Excel VBA?
+You can clear the clipboard by using the `MSForms.DataObject` class or the `Application.CutCopyMode` property.
What are some best practices for working with the clipboard in Excel VBA?
+Some best practices include always clearing the clipboard when finished using it, using the `Application.CutCopyMode` property to check if the clipboard contains data, and using the `Range.Copy` and `Range.Paste` methods to copy and paste data.
If you have any further questions or need additional help with Excel VBA, please don't hesitate to ask. You can also share your own experiences or tips for working with the clipboard in the comments below.