Intro
Learn Excel VBA truncate number techniques, including rounding and formatting numbers, using VBA code and functions like Int, Fix, and FormatNumber to simplify numerical data in spreadsheets.
The world of Excel VBA is a powerful tool for automating tasks and manipulating data. One common task that users often need to perform is truncating numbers, which involves removing the decimal part of a number without rounding it. In this article, we will explore the importance of truncating numbers in Excel VBA, the benefits of using VBA for this task, and provide a step-by-step guide on how to truncate numbers using VBA.
Truncating numbers is a crucial task in various fields, such as finance, engineering, and science, where precision is paramount. By truncating numbers, users can ensure that their calculations are accurate and consistent. Moreover, truncating numbers can help to simplify complex calculations and reduce errors. In Excel VBA, truncating numbers can be achieved using various methods, including using built-in functions, creating custom functions, and using VBA code.
One of the primary benefits of using VBA for truncating numbers is the level of control it provides. With VBA, users can create custom functions that can be tailored to their specific needs, allowing for greater flexibility and precision. Additionally, VBA code can be used to automate the truncation process, saving time and reducing the risk of errors. In this article, we will explore the different methods of truncating numbers in Excel VBA, including using the Int
function, the Fix
function, and creating custom functions.
Understanding Truncation in Excel VBA
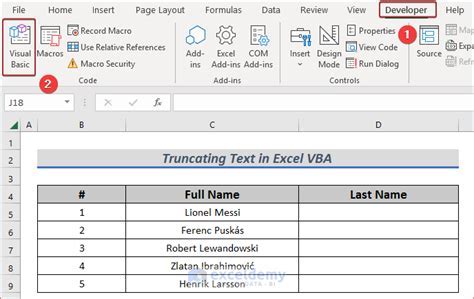
Before we dive into the different methods of truncating numbers, it's essential to understand what truncation means in the context of Excel VBA. Truncation refers to the process of removing the decimal part of a number without rounding it. For example, if we have the number 12.75, truncating it would result in 12, not 13. In Excel VBA, truncation can be achieved using various methods, including using built-in functions, creating custom functions, and using VBA code.
Using Built-in Functions
Excel VBA provides several built-in functions that can be used to truncate numbers, including the `Int` function and the `Fix` function. The `Int` function returns the largest integer that is less than or equal to the specified number, while the `Fix` function returns the largest integer that is less than or equal to the specified number. Both functions can be used to truncate numbers, but they differ in their behavior when dealing with negative numbers.Truncating Numbers using VBA Code
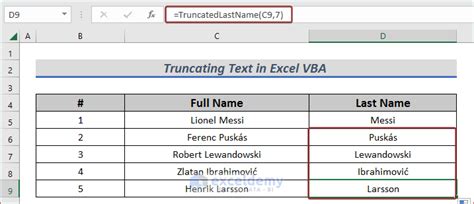
Truncating numbers using VBA code is a straightforward process that involves using the Int
or Fix
function. Here's an example of how to truncate a number using VBA code:
Sub TruncateNumber()
Dim num As Double
num = 12.75
truncatedNum = Int(num)
MsgBox truncatedNum
End Sub
This code defines a subroutine called TruncateNumber
that takes a number, truncates it using the Int
function, and displays the result in a message box.
Creating Custom Functions
In addition to using built-in functions and VBA code, users can also create custom functions to truncate numbers. Custom functions provide greater flexibility and can be tailored to specific needs. Here's an example of how to create a custom function to truncate numbers: ```vb Function Truncate(num As Double) As Integer Truncate = Int(num) End Function ``` This code defines a custom function called `Truncate` that takes a number as input, truncates it using the `Int` function, and returns the result.Benefits of Truncating Numbers in Excel VBA
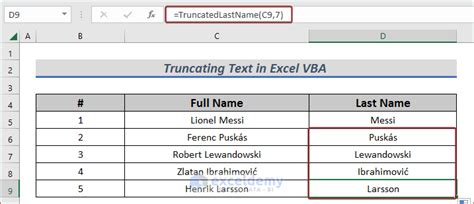
Truncating numbers in Excel VBA provides several benefits, including:
- Accuracy: Truncating numbers ensures that calculations are accurate and consistent.
- Simplification: Truncating numbers can simplify complex calculations and reduce errors.
- Flexibility: Custom functions and VBA code provide greater flexibility and can be tailored to specific needs.
- Automation: VBA code can be used to automate the truncation process, saving time and reducing the risk of errors.
Common Applications of Truncation
Truncation is a common task in various fields, including:- Finance: Truncation is used to calculate interest rates, investment returns, and other financial metrics.
- Engineering: Truncation is used to calculate measurements, distances, and other engineering metrics.
- Science: Truncation is used to calculate scientific metrics, such as temperatures, pressures, and velocities.
Best Practices for Truncating Numbers in Excel VBA
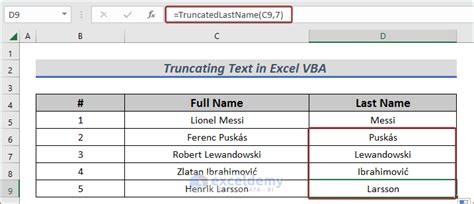
When truncating numbers in Excel VBA, it's essential to follow best practices to ensure accuracy and consistency. Here are some tips:
- Use built-in functions: Use built-in functions, such as the
Int
function, to truncate numbers. - Create custom functions: Create custom functions to provide greater flexibility and tailor the truncation process to specific needs.
- Use VBA code: Use VBA code to automate the truncation process and reduce the risk of errors.
- Test and validate: Test and validate the truncation process to ensure accuracy and consistency.
Gallery of Excel VBA Truncate Number
Excel VBA Truncate Number Image Gallery
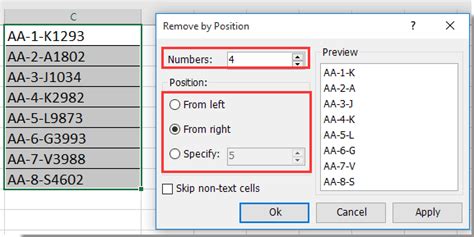
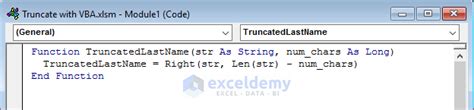
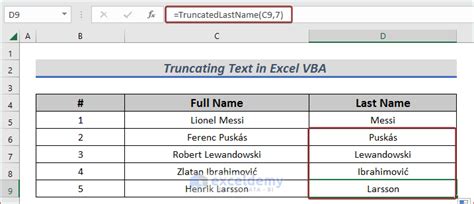
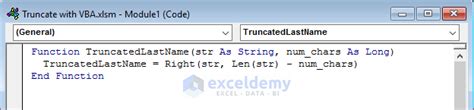
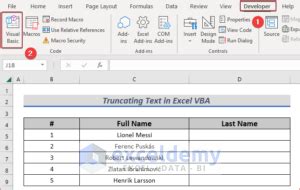
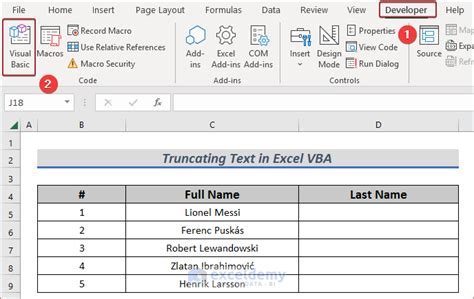
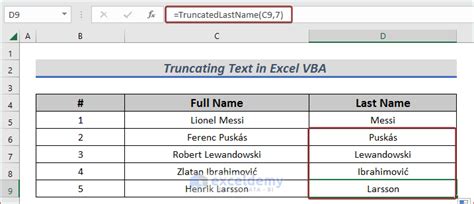
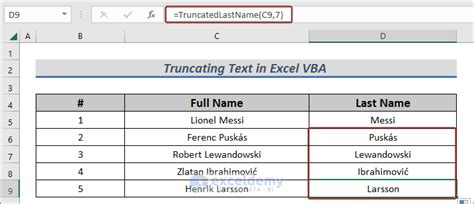
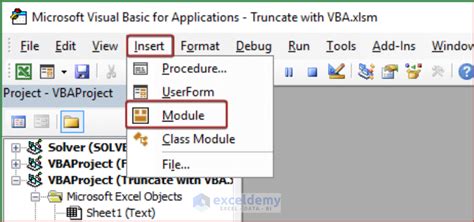
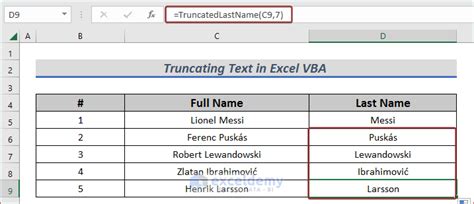
Frequently Asked Questions
What is truncation in Excel VBA?
+Truncation in Excel VBA refers to the process of removing the decimal part of a number without rounding it.
How do I truncate a number in Excel VBA?
+You can truncate a number in Excel VBA using the `Int` function, the `Fix` function, or by creating a custom function.
What are the benefits of truncating numbers in Excel VBA?
+The benefits of truncating numbers in Excel VBA include accuracy, simplification, flexibility, and automation.
How do I create a custom function to truncate numbers in Excel VBA?
+You can create a custom function to truncate numbers in Excel VBA by using the `Function` statement and the `Int` function.
What are some common applications of truncation in Excel VBA?
+Common applications of truncation in Excel VBA include finance, engineering, and science.
In conclusion, truncating numbers in Excel VBA is a powerful tool that can help users achieve accuracy, simplification, and flexibility in their calculations. By using built-in functions, creating custom functions, and following best practices, users can ensure that their truncation process is efficient and effective. Whether you're a beginner or an advanced user, this article has provided you with the knowledge and skills to truncate numbers like a pro. So, go ahead and start truncating those numbers, and take your Excel VBA skills to the next level! We encourage you to comment, share this article, or take specific actions to improve your Excel VBA skills.