Intro
Master Index and Match in VBA with expert techniques, leveraging lookup functions, data manipulation, and Excel automation for efficient data analysis and processing.
The world of VBA (Visual Basic for Applications) is a powerful tool for automating tasks in Microsoft Office applications, particularly in Excel. Two of the most useful functions in VBA for data manipulation and analysis are the Index and Match functions. These functions are crucial for looking up and retrieving data from large datasets, making them indispensable for data analysis and reporting.
In this article, we will delve into the details of using Index and Match in VBA, exploring their syntax, applications, and examples. We will also discuss best practices for implementing these functions effectively in your VBA code.
Introduction to Index and Match
The Index and Match functions are often used together in VBA to perform lookups and retrieve data from tables or ranges. The Match function is used to find the relative position of a value within a range, while the Index function returns a value at a specified position within a range.
Match Function
The Match function in VBA returns the relative position of a value within a range. The syntax for the Match function is:
Match(lookup_value, lookup_array, [match_type])
lookup_value
is the value you want to look up.lookup_array
is the range of cells where you want to look up the value.[match_type]
is optional and specifies whether you want an exact match or an approximate match.
Index Function
The Index function in VBA returns a value at a specified position within a range. The syntax for the Index function is:
Index(range, row_num, [col_num])
range
is the range of cells from which you want to return a value.row_num
is the row number of the value you want to return.[col_num]
is optional and specifies the column number if the range is more than one column wide.
Using Index and Match Together
When used together, the Index and Match functions can look up a value in one column of a table and return a corresponding value from another column. This is particularly useful for data analysis and reporting.
The general syntax for combining Index and Match is:
Index(return_range, Match(lookup_value, lookup_array, [match_type]), [col_num])
return_range
is the range from which you want to return a value.lookup_value
is the value you want to look up.lookup_array
is the range where you want to look up the value.[match_type]
is optional and specifies whether you want an exact match or an approximate match.[col_num]
is optional and specifies the column number if the return range is more than one column wide.
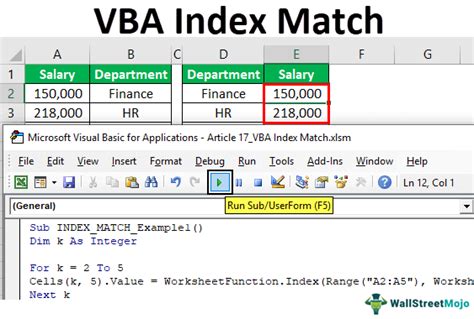
Practical Examples
Let's consider some practical examples to illustrate how to use Index and Match in VBA.
Example 1: Looking Up a Value
Suppose you have a table with employee names in column A and their corresponding IDs in column B. You want to find the ID of an employee named "John Doe".
Sub FindEmployeeID()
Dim lookupValue As String
Dim lookupArray As Range
Dim returnRange As Range
lookupValue = "John Doe"
Set lookupArray = Range("A1:A10") ' Range with employee names
Set returnRange = Range("B1:B10") ' Range with employee IDs
Dim employeeID As Variant
employeeID = Application.Index(returnRange, Application.Match(lookupValue, lookupArray, 0))
MsgBox "The ID of " & lookupValue & " is " & employeeID
End Sub
Example 2: Looking Up a Value with Multiple Criteria
Sometimes, you may need to look up a value based on multiple criteria. While the Index and Match functions can handle this by using an array formula, in VBA, it's more straightforward to use a loop or the Filter
function if you're using Excel 2019 or later.
However, for simplicity and compatibility, let's use a loop to look up a value based on two criteria.
Sub FindValueWithMultipleCriteria()
Dim lookupValue1 As String
Dim lookupValue2 As String
Dim lookupRange As Range
Dim returnRange As Range
Dim i As Long
lookupValue1 = "Criteria1"
lookupValue2 = "Criteria2"
Set lookupRange = Range("A1:C10") ' Range with data including criteria columns
Set returnRange = Range("D1:D10") ' Range with return values
For i = 1 To lookupRange.Rows.Count
If lookupRange.Cells(i, 1).Value = lookupValue1 And lookupRange.Cells(i, 2).Value = lookupValue2 Then
MsgBox "The value for " & lookupValue1 & " and " & lookupValue2 & " is " & returnRange.Cells(i, 1).Value
Exit For
End If
Next i
End Sub
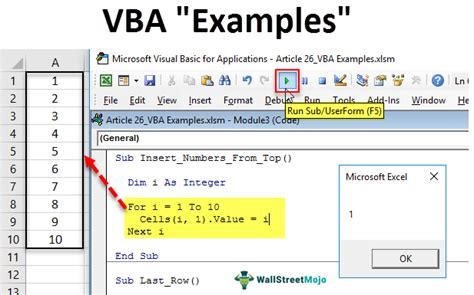
Best Practices
When using Index and Match in VBA, keep the following best practices in mind:
- Error Handling: Always include error handling to deal with situations where the lookup value is not found.
- Range References: Use explicit range references to avoid confusion and errors.
- Performance: For large datasets, consider using
Application.Index
andApplication.Match
to improve performance by minimizing the number of interactions with the Excel application. - Readability: Use descriptive variable names and comments to make your code readable and maintainable.
Advanced Techniques
For more advanced users, consider exploring the use of arrays and the Filter
function (in Excel 2019 and later) for more complex data manipulation tasks.
Using Arrays
Working with arrays in VBA can significantly improve performance when dealing with large datasets. You can load your data into arrays, perform operations, and then write the results back to the worksheet.
Sub UsingArrays()
Dim dataArray As Variant
Dim i As Long
' Load data into an array
dataArray = Range("A1:B10").Value
' Perform operations on the array
For i = LBound(dataArray) To UBound(dataArray)
' Example operation: double the value in the second column
dataArray(i, 2) = dataArray(i, 2) * 2
Next i
' Write the array back to the worksheet
Range("C1:D10").Value = dataArray
End Sub
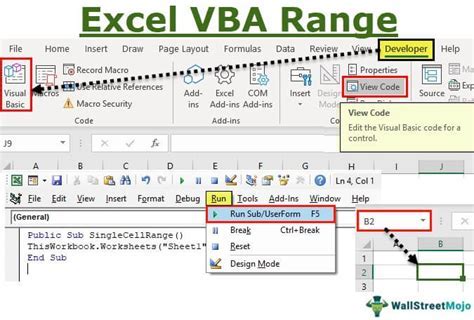
Using the Filter Function
The Filter
function, available in Excel 2019 and later, allows you to filter a range based on criteria. This can be a powerful tool for data analysis.
Sub UsingFilter()
Dim filteredData As Variant
' Filter a range based on criteria
filteredData = Application.Filter(Range("A1:C10"), "A1:A10", "Criteria1")
' Do something with the filtered data
Range("D1:E10").Value = filteredData
End Sub
Gallery of VBA Index and Match Examples
VBA Index and Match Examples
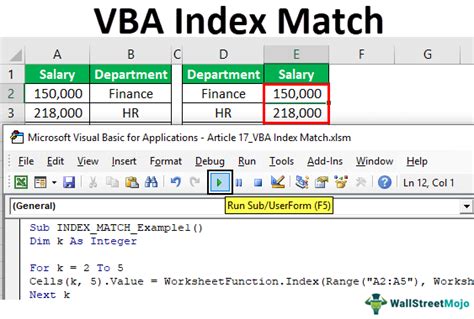
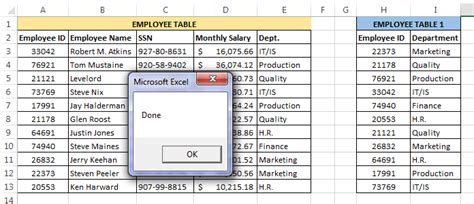
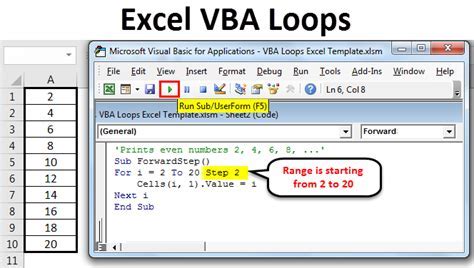
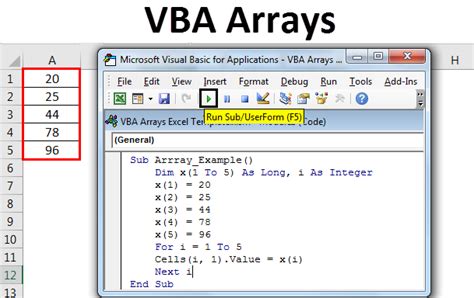
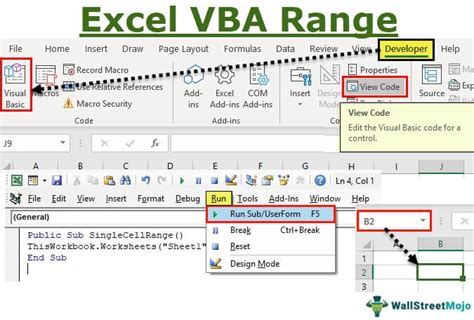
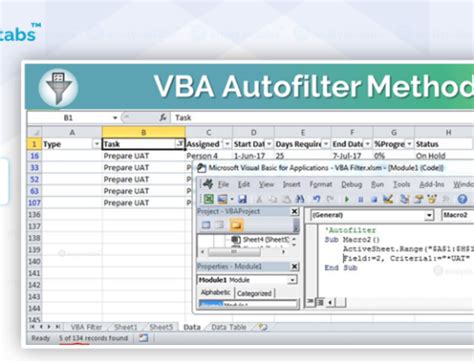

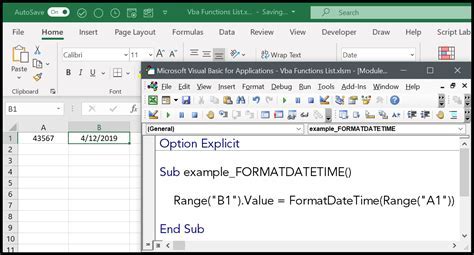
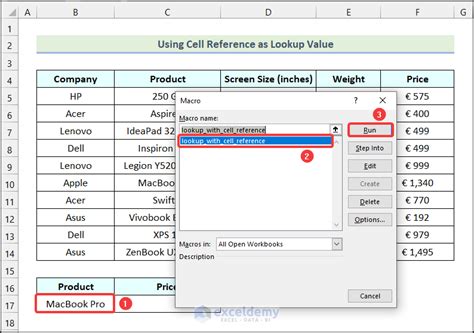
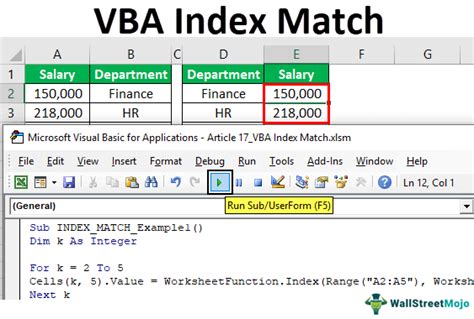
FAQs
What is the purpose of the Index function in VBA?
+The Index function in VBA returns a value at a specified position within a range.
How do I use the Match function in VBA to find a value?
+The Match function is used to find the relative position of a value within a range. It returns the position as a number that can then be used with the Index function to retrieve the actual value.
Can I use Index and Match together in VBA?
+Yes, Index and Match are often used together to look up a value in one column of a table and return a corresponding value from another column.
How do I handle errors when using Index and Match in VBA?
+Always include error handling to deal with situations where the lookup value is not found. This can be done using If statements to check if the Match function returns an error.
What are some best practices for using Index and Match in VBA?
+Best practices include using explicit range references, minimizing interactions with the Excel application for performance, and including error handling.
In conclusion, mastering the Index and Match functions in VBA is essential for efficient data analysis and manipulation in Excel. By understanding how to use these functions effectively, either alone or in combination, you can streamline your workflow and make your VBA scripts more powerful. Remember to always follow best practices and consider performance and readability when writing your code. Whether you're a beginner or an advanced user, the Index and Match functions are invaluable tools in your VBA toolkit.
We hope this comprehensive guide has been informative and helpful. If you have any further questions or would like to share your experiences with using Index and Match in VBA, please don't hesitate to comment below. Share this article with your colleagues and friends who might benefit from learning more about these powerful VBA functions.