Intro
Learn to check if a file exists in VBA using efficient code methods, including file system object and directory functions, to streamline your Excel macros and avoid errors with file existence checks.
Checking if a file exists in VBA is a crucial task for many applications, such as data import/export, file management, and automation. It helps prevent errors that could occur when trying to access or manipulate files that do not exist. VBA, or Visual Basic for Applications, is a programming language developed by Microsoft, and it is widely used for creating and automating tasks in Microsoft Office applications like Excel, Word, and Access.
Importance of Checking File Existence
Before performing operations like opening, reading, writing, or deleting a file, it's essential to verify that the file exists. This check ensures that your VBA script or program runs smoothly and doesn't encounter runtime errors. For instance, attempting to open a file that doesn't exist will result in an error, potentially stopping your script's execution.
Methods to Check if a File Exists in VBA
There are several methods to check if a file exists in VBA. Here are a few common approaches:
1. Using the Dir
Function
The Dir
function is a simple and straightforward way to check if a file exists. It returns the name of a file if it exists; otherwise, it returns an empty string.
Sub CheckFileExists()
Dim filePath As String
filePath = "C:\path\to\your\file.txt"
If Dir(filePath) <> "" Then
MsgBox "The file exists."
Else
MsgBox "The file does not exist."
End If
End Sub
2. Using the FileExists
Function from the FileSystemObject
The FileSystemObject
provides a more robust way to interact with the file system, including checking if a file exists. You need to set a reference to the Scripting.Runtime
library in your VBA project to use this method.
Sub CheckFileExistsFSO()
Dim fso As Object
Dim filePath As String
Set fso = CreateObject("Scripting.FileSystemObject")
filePath = "C:\path\to\your\file.txt"
If fso.FileExists(filePath) Then
MsgBox "The file exists."
Else
MsgBox "The file does not exist."
End If
Set fso = Nothing
End Sub
To set a reference to the Scripting.Runtime
library, follow these steps:
- Open the Visual Basic Editor.
- Click
Tools
>References
in the menu. - In the References dialog box, scroll down and check
Microsoft Scripting Runtime
. - Click
OK
.
3. Using Late Binding without Setting References
If you prefer not to set a reference to the Scripting.Runtime
library, you can use late binding. This method is useful when distributing your VBA project, as it doesn't require the recipient to set any references.
Sub CheckFileExistsLateBinding()
Dim fso As Object
Dim filePath As String
Set fso = CreateObject("Scripting.FileSystemObject")
filePath = "C:\path\to\your\file.txt"
If fso.FileExists(filePath) Then
MsgBox "The file exists."
Else
MsgBox "The file does not exist."
End If
Set fso = Nothing
End Sub
This method is essentially the same as the FileSystemObject
method but uses late binding (CreateObject
instead of New
keyword), which means you don't need to set a reference to the library in your VBA project.
Practical Examples and Advice
When checking if a file exists, consider the following best practices:
- Always specify the full path to the file to avoid confusion with files having the same name in different directories.
- Be aware of file system permissions; even if a file exists, your script might not have permission to access it.
- Use error handling (
On Error
statements) to gracefully manage situations where files are expected but not found.
Gallery of File Management in VBA
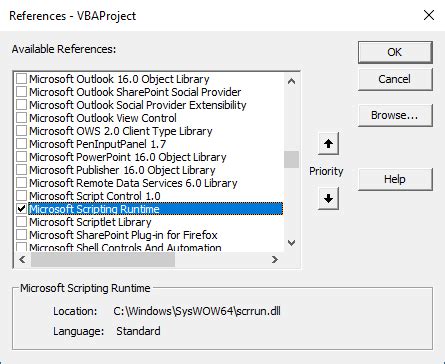
Gallery Section
VBA File Management Image Gallery
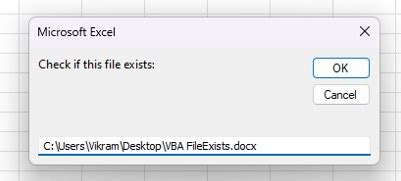
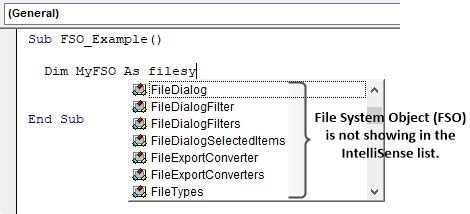
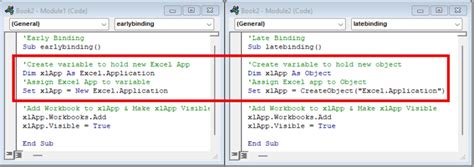
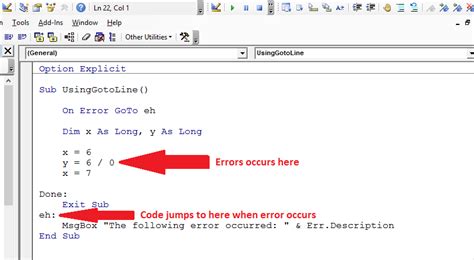

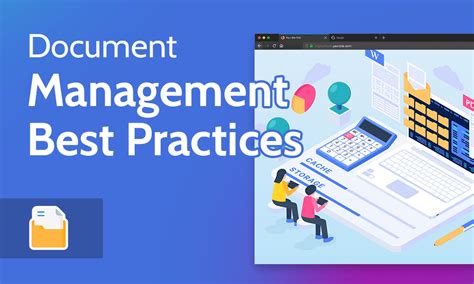
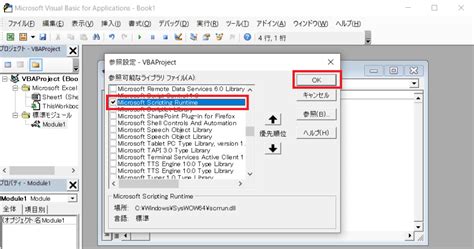

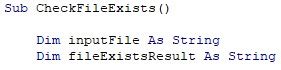

FAQs
What is the simplest way to check if a file exists in VBA?
+The simplest way is to use the `Dir` function, which returns the name of a file if it exists.
How do I use the `FileSystemObject` to check for file existence?
+First, set a reference to the `Scripting.Runtime` library, then create an instance of `FileSystemObject`, and use its `FileExists` method.
What is late binding in VBA, and how does it relate to checking file existence?
+Late binding in VBA refers to creating objects at runtime using `CreateObject` instead of setting references. It's useful for file existence checks when you don't want to set a reference to the `Scripting.Runtime` library.
Why is error handling important when checking if a file exists in VBA?
+Error handling is crucial because it allows your script to gracefully manage situations where files are expected but not found, preventing runtime errors and ensuring a smoother user experience.
Can I use these methods to check for folder existence as well?
+Yes, similar methods exist for checking folder existence, such as using the `Dir` function or the `FolderExists` method of the `FileSystemObject`.
In conclusion, checking if a file exists is a fundamental aspect of VBA programming that can help prevent errors and ensure the smooth execution of your scripts. By using methods like the Dir
function, FileSystemObject
, or late binding, you can effectively manage file existence checks in your VBA projects. Remember to always consider best practices such as specifying full file paths, being aware of file system permissions, and implementing robust error handling. With these strategies, you can write more reliable and efficient VBA code.
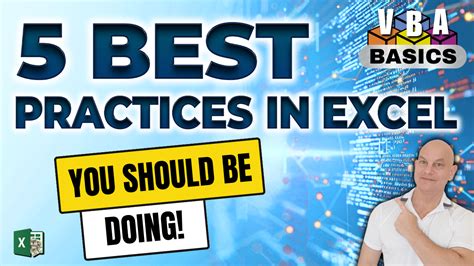
If you have any further questions or need more detailed explanations on any of the topics covered, feel free to ask in the comments. Sharing your experiences or tips on handling file existence checks in VBA can also be helpful for others.