Intro
Unlock VBAs Match Function to simplify data lookup and retrieval, leveraging exact and approximate matching, wildcard characters, and array formulas for efficient data analysis and automation in Excel.
The VBA Match function is a powerful tool used in Excel Visual Basic for Applications (VBA) to find the relative position of a value within a range of cells. This function is often utilized in various Excel automation tasks, such as data manipulation, reporting, and more. Understanding how to use the VBA Match function can significantly enhance your ability to work with data in Excel using VBA.
The VBA Match function is similar to the Excel worksheet function MATCH, but it's used within VBA code. The syntax for the VBA Match function is as follows:
Match(lookup_value, lookup_array, [lookup_type])
lookup_value
is the value you want to search for.lookup_array
is the range of cells where you want to search for thelookup_value
.[lookup_type]
is optional and specifies whether you want an exact match or an approximate match. The possible values are:1
or omitted: Finds an exact match. If no exact match is found, it returns an error.0
: Finds an exact match. If no exact match is found, it returns an error. This is the same as omitting the lookup_type argument.-1
: Finds a value that is less than thelookup_value
. If no such value exists, it returns an error.1
: Finds a value that is greater than thelookup_value
. If no such value exists, it returns an error.
To illustrate the use of the VBA Match function, let's consider a simple example. Suppose we have a list of names in column A of a worksheet, and we want to find the position of a specific name, "John", within this list using VBA.
Sub FindPosition()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim lookupValue As String
lookupValue = "John"
Dim lookupArray As Range
Set lookupArray = ws.Range("A1:A10")
Dim position As Long
position = Application.Match(lookupValue, lookupArray, 0)
If Not IsError(position) Then
MsgBox "The name '" & lookupValue & "' is found at position " & position
Else
MsgBox "The name '" & lookupValue & "' is not found"
End If
End Sub
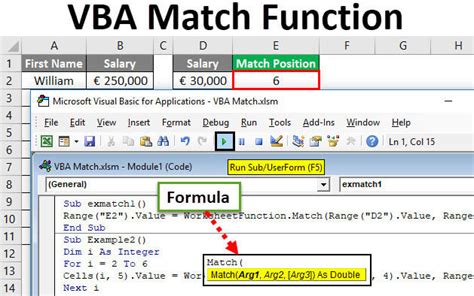
Benefits of Using the VBA Match Function
- Efficient Data Search: The VBA Match function allows for efficient searching of data within ranges. It's particularly useful when you need to locate specific values or perform actions based on the position of those values.
- Flexibility: The function can be used with various types of data, including strings, numbers, and dates, making it versatile for different applications.
- Error Handling: By using error handling techniques like
IsError
, you can manage situations where thelookup_value
is not found, making your code more robust.
Working Mechanisms
- Exact Match: The most common use of the Match function is to find an exact match. This requires setting the
lookup_type
to0
or omitting it. - Approximate Match: For approximate matches, you can use
-1
or1
as thelookup_type
, depending on whether you're looking for values less than or greater than thelookup_value
.
Steps for Implementation
- Define the Lookup Value: Clearly define what you're searching for.
- Specify the Lookup Array: Determine the range of cells where you want to perform the search.
- Choose the Lookup Type: Decide whether you need an exact or approximate match.
- Implement Error Handling: Use
IsError
or similar methods to handle cases where thelookup_value
is not found.
Practical Examples
- Data Validation: Use the Match function to check if a user-input value exists within a predefined list.
- Automated Reporting: Find and extract data based on specific criteria for report generation.
- Data Cleanup: Identify and remove duplicate entries by matching values within a dataset.
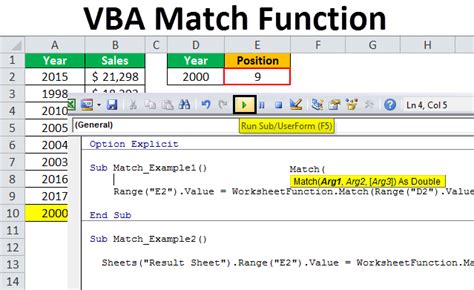
SEO Optimization for VBA Content
When creating content related to VBA, including discussions about the Match function, it's essential to optimize for search engines. This involves:
- Keyword Density: Ensuring that relevant keywords, such as "VBA Match function" and "Excel automation," appear throughout the content with a density of about 1-2%.
- Synonyms and Related Phrases: Using variations of the main keywords, like "VBA lookup" or "Excel VBA Match," to diversify the language and improve SEO relevance.
- Short Paragraphs: Keeping paragraphs concise to enhance readability and maintain user engagement.
Gallery of VBA Match Function Examples
VBA Match Function Image Gallery
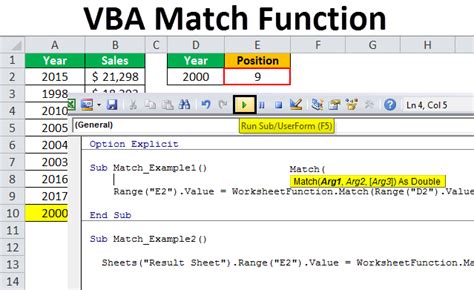
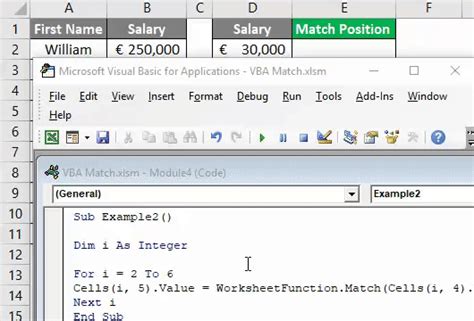
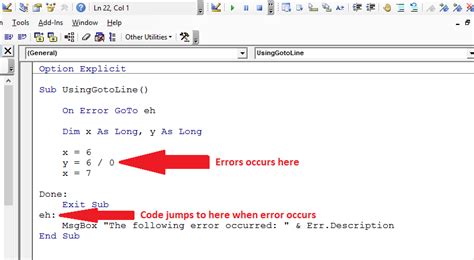
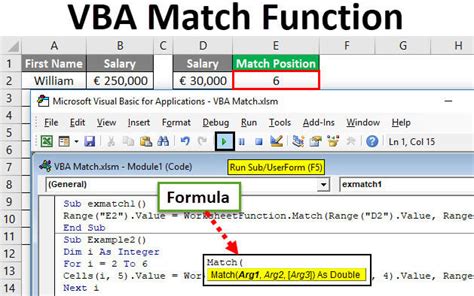
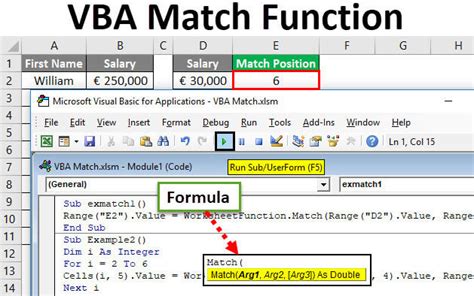

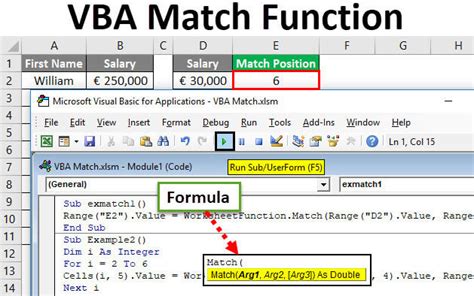
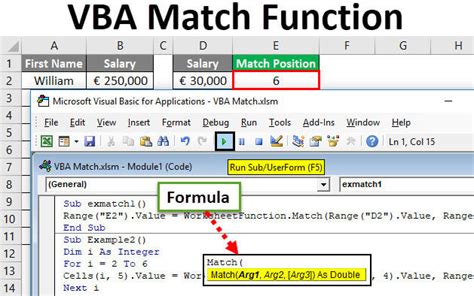
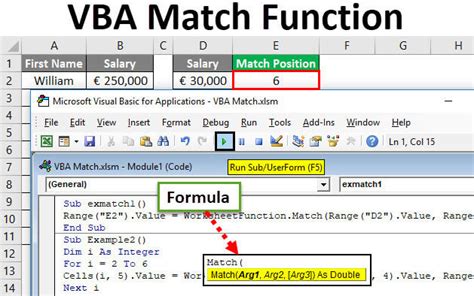
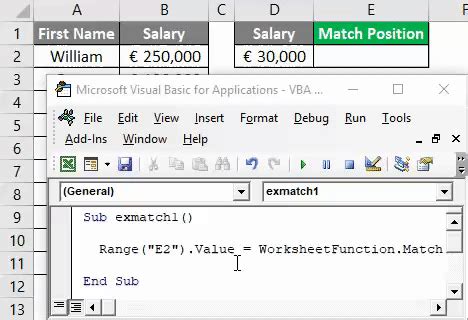
FAQs
What is the VBA Match function used for?
+The VBA Match function is used to find the relative position of a value within a range of cells in Excel.
How do I handle errors when using the VBA Match function?
+You can handle errors by using the `IsError` function to check if the Match function returns an error value, indicating that the lookup value was not found.
Can I use the VBA Match function for approximate matches?
+Yes, you can use the VBA Match function for approximate matches by specifying the `lookup_type` as `-1` for values less than the lookup value or `1` for values greater than the lookup value.
In conclusion, mastering the VBA Match function can significantly enhance your ability to automate tasks and manipulate data in Excel. By understanding its syntax, benefits, and how to implement it effectively, you can create more efficient and robust VBA scripts. Whether you're a beginner or an experienced VBA user, the Match function is a valuable tool to have in your arsenal. Feel free to share your experiences or ask questions about using the VBA Match function in the comments below.