Intro
Combine data with VBA, merging multiple sheets into one sheet efficiently, using Excel macros and scripting to automate tasks, synchronize worksheets, and consolidate data.
Merging multiple sheets into one sheet is a common task in Excel, and it can be accomplished using VBA (Visual Basic for Applications). This process can save you a significant amount of time, especially when dealing with large datasets. In this article, we will explore how to merge multiple sheets into one sheet using VBA, including the benefits, working mechanisms, steps, and practical examples.
When working with Excel, you often find yourself dealing with multiple worksheets that contain related data. For instance, you might have sales data for different regions, each on its own sheet. To get a comprehensive view or to perform analysis, it's helpful to have all this data in one place. Manually copying and pasting data from each sheet into a new sheet can be tedious and prone to errors. This is where VBA comes into play, offering a powerful and efficient solution to merge these sheets automatically.
Benefits of Using VBA for Merging Sheets
Using VBA to merge multiple sheets into one offers several benefits:
- Efficiency: It saves time by automating the process, especially when dealing with a large number of sheets or datasets.
- Accuracy: By reducing manual intervention, it minimizes the chance of human error, such as missing data or incorrect pasting.
- Flexibility: VBA scripts can be easily modified to accommodate different merging requirements, such as merging based on specific criteria or handling different data formats.
Working Mechanism of VBA Scripts for Merging Sheets
VBA scripts for merging sheets typically work by iterating through each worksheet in a workbook, selecting all data (or specific ranges), and then pasting this data into a target worksheet. The script can be designed to append data below existing data in the target sheet, ensuring that all data from multiple sheets is compiled into one continuous dataset.
Steps to Merge Multiple Sheets into One Using VBA
Here are the basic steps to create a VBA script for merging sheets:
- Open Excel: Start by opening your Excel workbook that contains the multiple sheets you want to merge.
- Access VBA Editor: Press
Alt + F11
to open the VBA Editor. - Insert Module: In the VBA Editor, right-click on any of the objects for your workbook in the "Project" window. Choose
Insert
>Module
to insert a new module. - Write the VBA Script: In the module window, you will write your VBA code. Below is a simple example of a script that merges data from all sheets into one sheet named "MasterSheet".
Sub MergeSheets()
Dim ws As Worksheet
Dim targetWorksheet As Worksheet
' Set target worksheet
Set targetWorksheet = ThisWorkbook.Worksheets("MasterSheet")
' Initialize row counter for target sheet
Dim targetRow As Long
targetRow = 1
' Loop through all worksheets
For Each ws In ThisWorkbook.Worksheets
' Skip the target worksheet to avoid infinite loop
If ws.Name <> targetWorksheet.Name Then
' Copy data from current worksheet
ws.Cells.Copy
' Paste data into target worksheet
targetWorksheet.Rows(targetRow).Paste
' Increment target row to avoid overwriting
targetRow = targetRow + ws.UsedRange.Rows.Count
' Clear clipboard
Application.CutCopyMode = False
End If
Next ws
End Sub
- Run the Script: With your script written, you can run it by pressing
F5
while in the VBA Editor with the module containing your script active, or by closing the VBA Editor and using the "Developer" tab in Excel to run the macro.
Practical Examples and Statistical Data
In real-world scenarios, the need to merge sheets arises frequently. For example, in a sales department, you might have separate sheets for different sales channels (online, offline, wholesale). To analyze overall sales performance, merging these sheets into one provides a comprehensive view, allowing for easier analysis and reporting.
Tips for Optimizing VBA Scripts
- Error Handling: Always include error handling in your scripts to manage unexpected issues, such as a worksheet not being found.
- Performance: For large datasets, consider optimizing your script to reduce processing time, such as by turning off screen updating or using more efficient data transfer methods.
- Security: Be aware of Excel's macro security settings, which might prevent your scripts from running. Ensure that macros are enabled for your workbook.
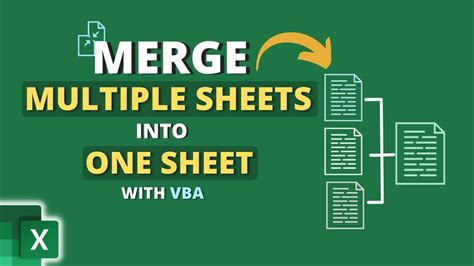
Benefits of Merging Sheets
Merging sheets offers several benefits, including simplified data analysis and reporting. With all your data in one place, you can easily apply filters, sorting, and grouping to understand your data better.
Common Challenges and Solutions
One common challenge when merging sheets is dealing with inconsistent data formats. For example, date formats might differ between sheets. To solve this, you can include data formatting steps in your VBA script to ensure consistency.
Conclusion and Next Steps
Merging multiple sheets into one using VBA is a powerful technique for managing and analyzing data in Excel. By following the steps and examples outlined above, you can create your own VBA scripts tailored to your specific needs. Remember to always test your scripts in a safe environment before applying them to critical data.

Advanced VBA Techniques for Merging Sheets
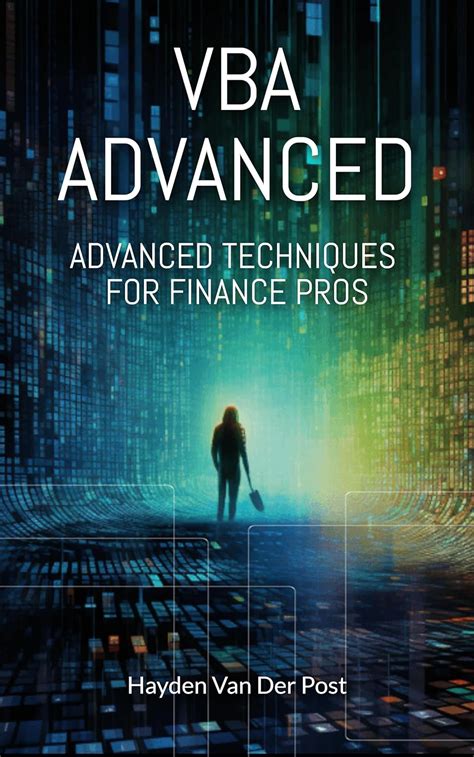
For more complex merging tasks, such as merging based on specific criteria or handling duplicates, you can use advanced VBA techniques. These include using arrays to store and manipulate data, employing SQL queries to merge data, and utilizing Excel's built-in functions for data manipulation.
Using Arrays for Data Manipulation
Arrays can be used to store data from multiple sheets and then manipulate this data before pasting it into the target sheet. This approach is particularly useful for large datasets, as it can improve performance by reducing the number of interactions with the Excel interface.Best Practices for Writing VBA Scripts
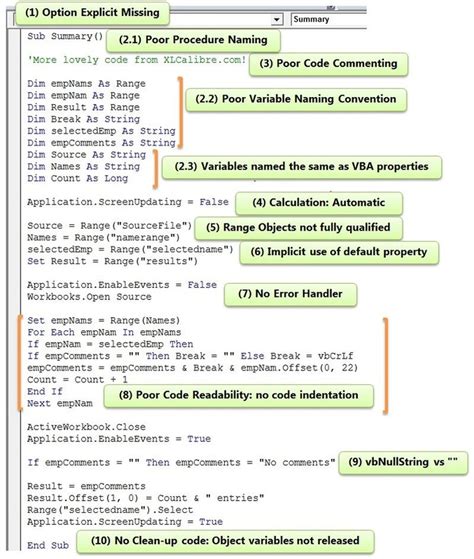
When writing VBA scripts, it's essential to follow best practices to ensure your scripts are efficient, readable, and maintainable. This includes using clear variable names, commenting your code, and testing your scripts thoroughly.
Debugging VBA Scripts
Debugging is an essential part of the script development process. Excel's VBA Editor provides several tools for debugging, including the ability to step through your code line by line, set breakpoints, and use the Immediate window to execute commands and inspect variables.Merge Sheets Image Gallery
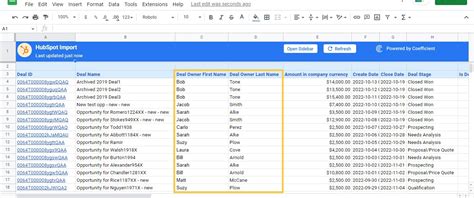
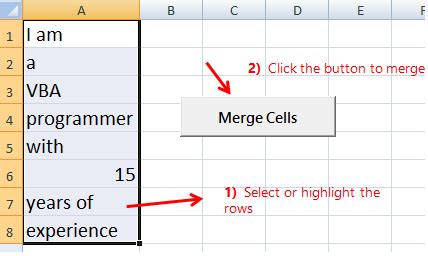
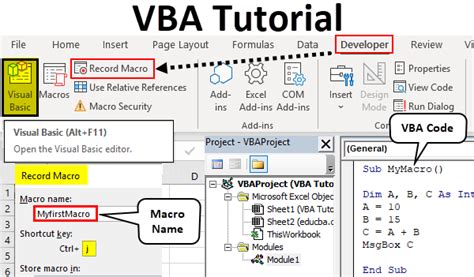
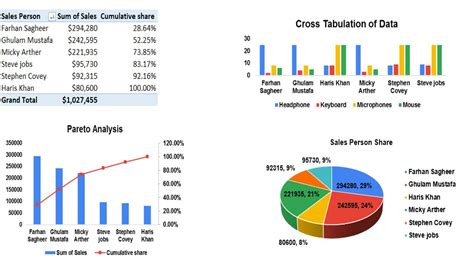
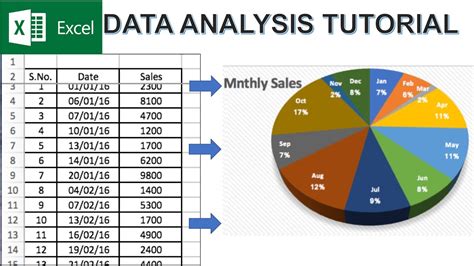
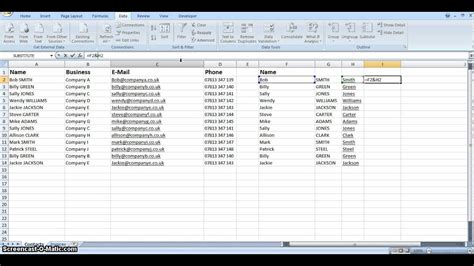
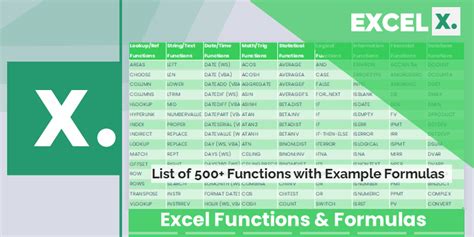
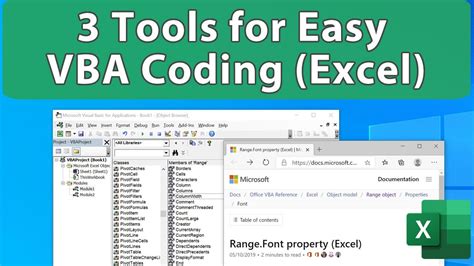
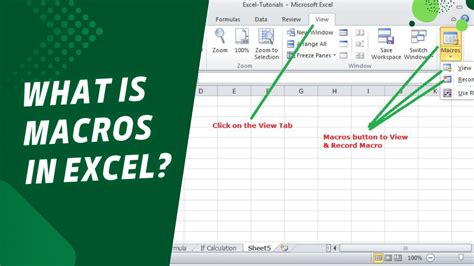
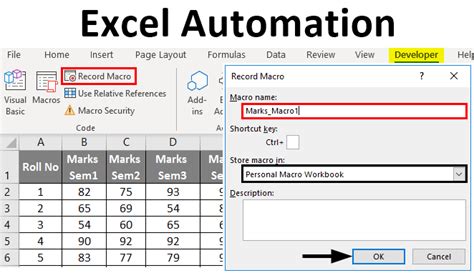
How do I enable macros in Excel?
+To enable macros in Excel, go to the "Developer" tab, click on "Macro Security," and then select the option to enable macros.
What are the benefits of using VBA for merging sheets?
+The benefits include efficiency, accuracy, and flexibility in managing and analyzing data across multiple sheets.
How can I troubleshoot issues with my VBA script?
+Use the VBA Editor's debugging tools, such as stepping through code, setting breakpoints, and using the Immediate window to inspect variables and execute commands.
We hope this comprehensive guide has provided you with the knowledge and tools necessary to merge multiple sheets into one using VBA. Whether you're a beginner or an advanced user, mastering VBA scripts can significantly enhance your productivity and data analysis capabilities in Excel. Feel free to share your experiences, ask questions, or provide feedback in the comments below.