Intro
When working with VBA in Microsoft Office applications, inserting a new line in a message box can be necessary for better readability and to convey more information effectively. However, VBA's message box function, MsgBox
, does not directly support multiline input through a specific parameter for line breaks. Instead, you can use a few different approaches to achieve the effect of a new line in your message box.
Using vbNewLine
The most straightforward way to insert a new line in a MsgBox
is by using the vbNewLine
constant. This constant represents a new line character and can be used within your string to create line breaks.
MsgBox "This is the first line" & vbNewLine & "This is the second line"
Using vbCrLf
Another way to achieve a line break is by using vbCrLf
, which represents a carriage return followed by a line feed. This is essentially doing the same thing as vbNewLine
but is more explicit about the characters being used.
MsgBox "This is the first line" & vbCrLf & "This is the second line"
Using Chr(13) & Chr(10)
For a more manual approach, you can use the ASCII characters for a carriage return (Chr(13)
) and a line feed (Chr(10)
), which together achieve the same effect as vbCrLf
.
MsgBox "This is the first line" & Chr(13) & Chr(10) & "This is the second line"
Practical Example
Let's say you want to display a message box that thanks a user for using your application and then provides additional information on how to contact support. You could structure your message like this:
Dim message As String
message = "Thank you for using our application!" & vbNewLine & _
"If you have any questions or need further assistance, please contact our support team at support@example.com."
MsgBox message, vbInformation, "Application Feedback"
This will display a message box with two lines of text, making the information easier to read and understand.
Embedding Images in VBA MsgBox
While MsgBox
itself does not support embedding images directly, you can create a custom user form to display images along with your text. This approach requires designing a form in the Visual Basic Editor, adding a PictureBox control, and then displaying the form instead of using MsgBox
.
- Insert a User Form: In the Visual Basic Editor, go to
Insert
>User Form
to create a new form. - Add a PictureBox: Drag and drop a PictureBox control from the Toolbox onto your form.
- Add a Label or TextBox: For displaying your text, you can use a Label or a TextBox, depending on your needs.
- Load the Image: Use the
LoadPicture
method to load an image into the PictureBox. - Show the Form: Instead of using
MsgBox
, show your custom form using theShow
method.
Gallery Section
VBA MsgBox Gallery
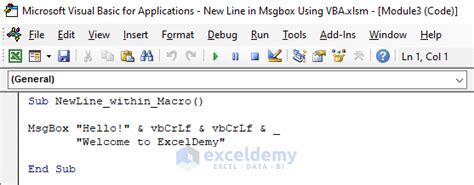
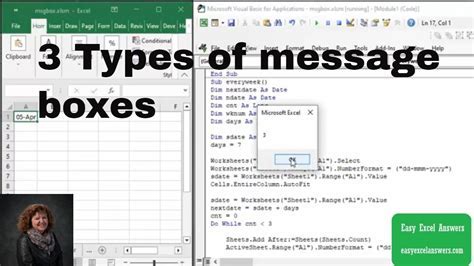
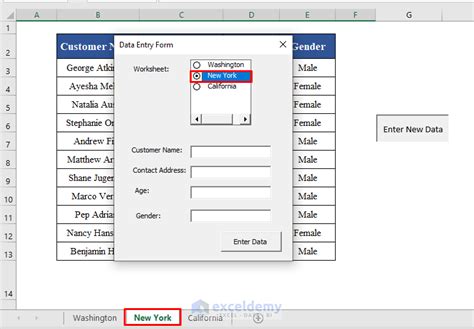
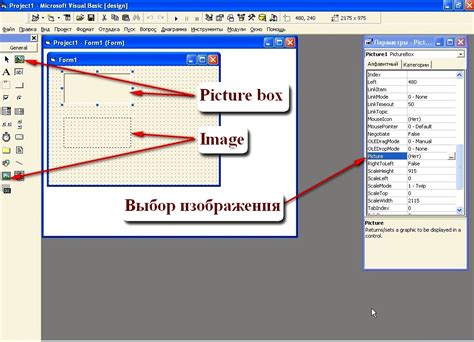
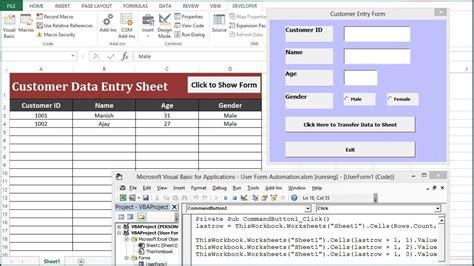
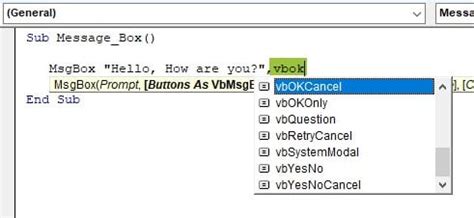
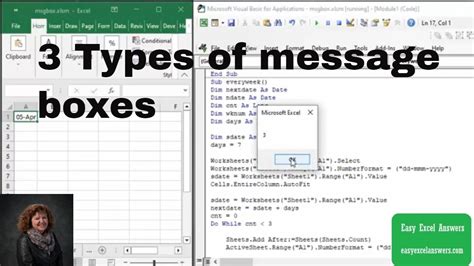
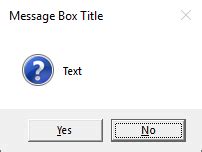
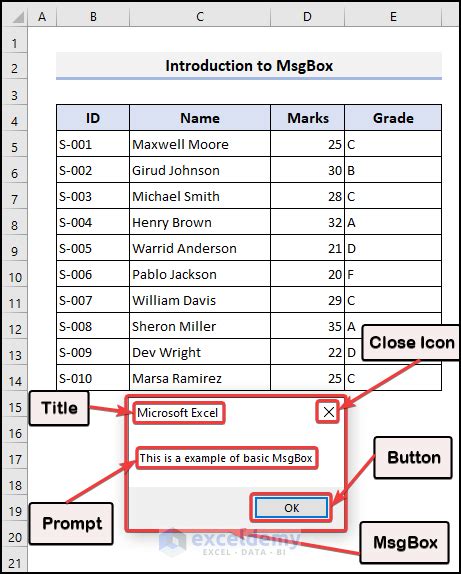
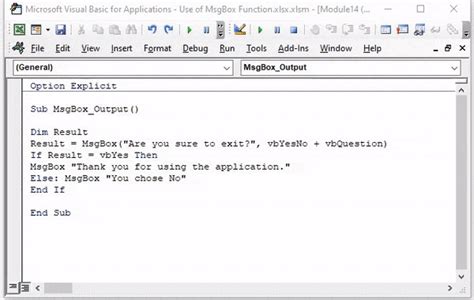
FAQs
How do I insert a new line in a VBA MsgBox?
+You can insert a new line in a VBA MsgBox by using the vbNewLine constant, vbCrLf, or Chr(13) & Chr(10) within your string.
Can I display images in a VBA MsgBox?
+While MsgBox itself does not support images, you can create a custom user form with a PictureBox to achieve this.
How do I create a custom user form in VBA?
+To create a custom user form, go to Insert > User Form in the Visual Basic Editor, and then design your form by adding controls such as PictureBox, Label, or TextBox.
In conclusion, working with VBA's MsgBox can be flexible when you know the right techniques, such as inserting new lines and potentially using custom forms for more complex messaging needs. Whether you're aiming to improve user experience with better-formatted messages or to display custom content like images, VBA provides the tools to achieve your goals. Feel free to experiment with different approaches and share your experiences or questions in the comments below.