Intro
Generate random numbers in Excel with VBA Random Number Generator, using macros and scripting to create unpredictable sequences, including integer and decimal numbers, for simulations, modeling, and data analysis.
The world of Visual Basic for Applications (VBA) is a powerful tool for automating tasks in Microsoft Office applications. One of the most useful features in VBA is the ability to generate random numbers, which can be applied in a wide range of scenarios, from simulations and modeling to games and statistical analysis. In this article, we will delve into the realm of VBA random number generators, exploring their importance, how they work, and providing practical examples to get you started.
Random number generation is a crucial aspect of many applications, including scientific research, engineering, and finance. It allows developers to introduce variability into their models, making them more realistic and reliable. In VBA, random number generation can be used to create simulations, generate test data, or even create games. The importance of random number generators cannot be overstated, as they provide a way to mimic real-world uncertainty and randomness.
VBA provides several ways to generate random numbers, including the use of the Rnd
function and the Randomize
statement. The Rnd
function returns a random number between 0 and 1, while the Randomize
statement is used to initialize the random number generator with a seed value. By using these functions, developers can create complex simulations and models that take into account the inherent randomness of real-world phenomena.
VBA Random Number Generator Functions

The Rnd
function is the most commonly used function for generating random numbers in VBA. It returns a random number between 0 and 1, which can then be scaled and shifted to generate numbers within a specific range. The Randomize
statement, on the other hand, is used to initialize the random number generator with a seed value. This ensures that the random numbers generated are truly random and not reproducible.
Using the Rnd Function
The `Rnd` function is easy to use and provides a simple way to generate random numbers. To use the `Rnd` function, simply call it in your VBA code, like this: `randomNumber = Rnd()`. This will generate a random number between 0 and 1, which can then be used in your application.VBA Random Number Generator Examples
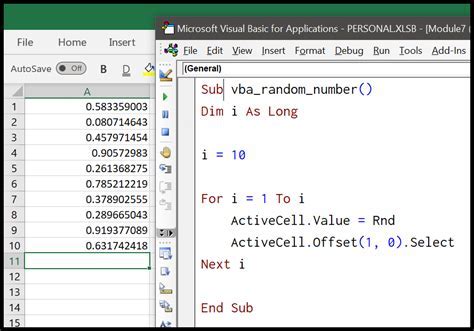
Here are a few examples of how to use the Rnd
function to generate random numbers in VBA:
- Generate a random integer between 1 and 10:
randomNumber = Int((10 * Rnd) + 1)
- Generate a random decimal number between 0 and 10:
randomNumber = (10 * Rnd)
- Generate a random boolean value (True or False):
randomBoolean = (Rnd > 0.5)
Seeding the Random Number Generator
To ensure that the random numbers generated are truly random and not reproducible, it's essential to seed the random number generator with a unique value. This can be done using the `Randomize` statement, like this: `Randomize`. The `Randomize` statement uses the system clock to generate a unique seed value, which is then used to initialize the random number generator.VBA Random Number Generator Best Practices
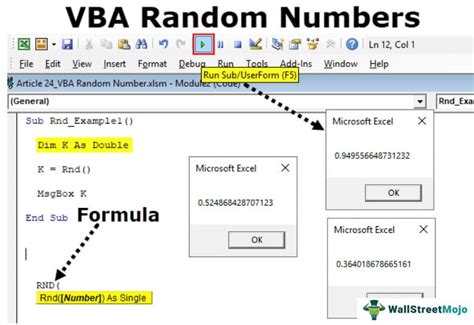
When using the VBA random number generator, there are several best practices to keep in mind:
- Always seed the random number generator with a unique value using the
Randomize
statement. - Use the
Rnd
function to generate random numbers, rather than trying to create your own random number algorithm. - Be careful when using the
Rnd
function to generate random integers, as the results may not be uniformly distributed.
Common Pitfalls to Avoid
When using the VBA random number generator, there are several common pitfalls to avoid: * Not seeding the random number generator with a unique value, resulting in reproducible random numbers. * Using the `Rnd` function to generate random numbers without scaling and shifting the results to the desired range. * Trying to create your own random number algorithm, rather than using the built-in `Rnd` function.VBA Random Number Generator Applications
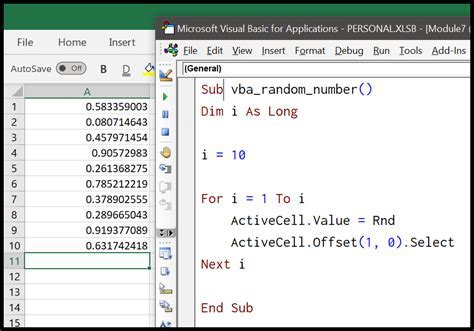
The VBA random number generator has a wide range of applications, including:
- Simulations and modeling: Random number generation can be used to introduce variability into simulations and models, making them more realistic and reliable.
- Games: Random number generation can be used to create games that involve chance and uncertainty, such as card games or dice games.
- Statistical analysis: Random number generation can be used to generate test data for statistical analysis, allowing developers to test their models and algorithms.
Real-World Examples
Here are a few real-world examples of how the VBA random number generator can be used: * A financial model that uses random number generation to simulate stock prices and portfolio returns. * A game that uses random number generation to determine the outcome of player actions, such as a card game or a dice game. * A statistical analysis that uses random number generation to generate test data, allowing developers to test their models and algorithms.VBA Random Number Generator Gallery
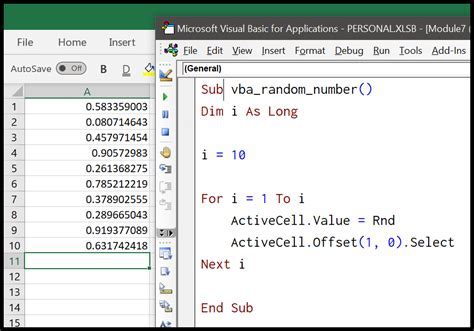
VBA Random Number Generator Image Gallery
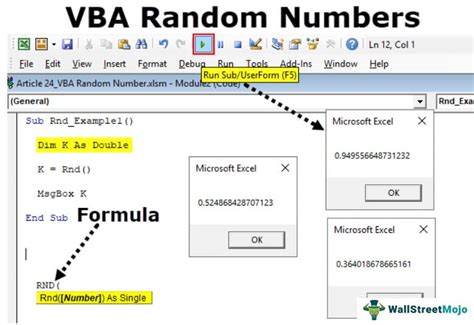
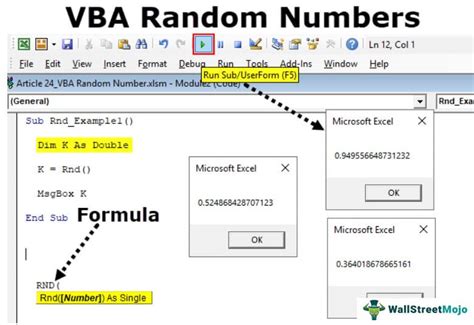

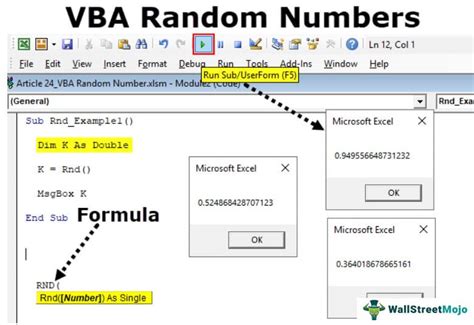
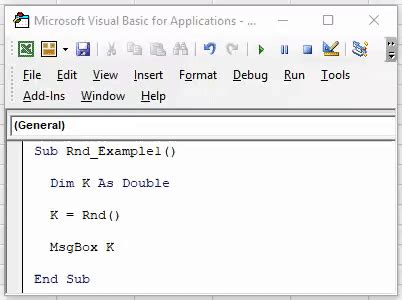
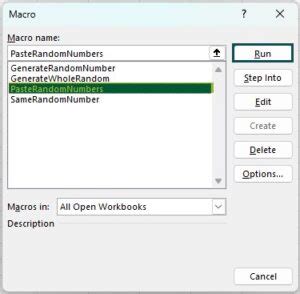


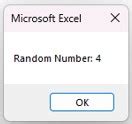
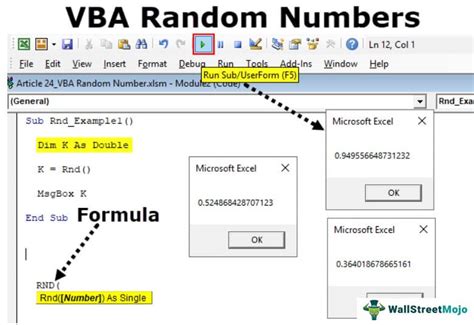
VBA Random Number Generator FAQs
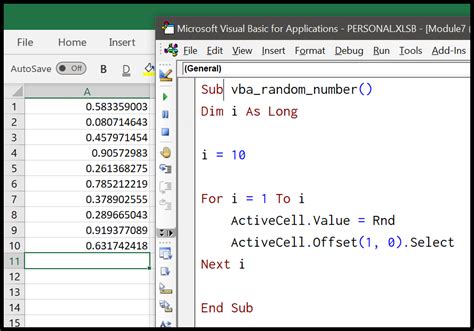
What is the purpose of the VBA random number generator?
+The VBA random number generator is used to introduce variability into simulations and models, making them more realistic and reliable.
How do I use the Rnd function to generate random numbers?
+To use the Rnd function, simply call it in your VBA code, like this: `randomNumber = Rnd()`. This will generate a random number between 0 and 1, which can then be scaled and shifted to the desired range.
What is the importance of seeding the random number generator?
+Seeding the random number generator with a unique value ensures that the random numbers generated are truly random and not reproducible.
In conclusion, the VBA random number generator is a powerful tool for introducing variability into simulations and models. By using the Rnd
function and seeding the random number generator with a unique value, developers can create complex and realistic models that take into account the inherent randomness of real-world phenomena. Whether you're a seasoned developer or just starting out with VBA, the random number generator is an essential tool to have in your toolkit. So why not give it a try and see what kind of creative and innovative applications you can come up with? Share your thoughts and experiences with us in the comments below, and don't forget to share this article with your friends and colleagues who may be interested in learning more about the VBA random number generator.