Intro
Discover 5 ways to refer cells in VBA, including range, offset, and index methods, to enhance Excel automation with cell references, macros, and spreadsheet programming.
Referencing cells in VBA is a fundamental concept when working with Excel using Visual Basic for Applications. It allows you to access and manipulate data within cells, which is crucial for creating dynamic and interactive Excel applications. There are several ways to refer to cells in VBA, each with its own advantages and use cases. Here, we'll explore five common methods to refer to cells in VBA, along with examples and explanations to help you understand when and how to use each method.
The importance of correctly referencing cells cannot be overstated. It's the basis upon which more complex operations are built, such as data manipulation, chart creation, and even user interface interactions. Whether you're a beginner looking to automate simple tasks or an advanced user aiming to create complex Excel applications, mastering cell references in VBA is essential.
Before diving into the specifics of each method, it's worth noting that the choice of method often depends on the specific requirements of your project. Factors such as the need for flexibility, performance, and readability can influence your decision. Additionally, understanding the differences between these methods can help you write more efficient and maintainable code.
Introduction to Referencing Cells
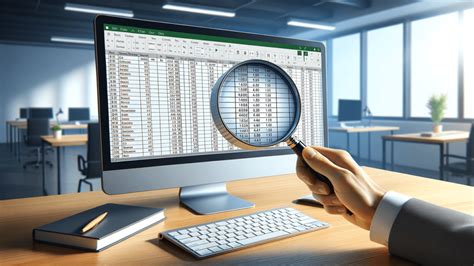
Referencing cells in VBA involves specifying the cell or range of cells you want to work with. This can be done in several ways, including using the cell's address, its position in a range, or by using named ranges. Each method has its own syntax and advantages, and choosing the right one depends on the context and requirements of your VBA script.
1. Using the Range Object
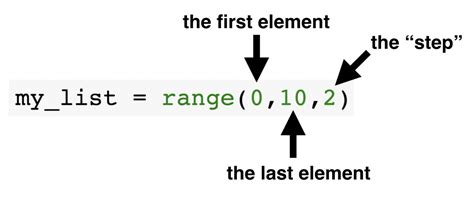
One of the most common ways to refer to cells in VBA is by using the Range
object. The Range
object represents a cell, a row, a column, a selection of cells, or a 3D range. You can specify a range by its address, such as "A1" for a single cell or "A1:B2" for a range of cells.
Sub ReferCellUsingRange()
' Refer to a single cell
Range("A1").Value = "Hello, World!"
' Refer to a range of cells
Range("A1:B2").Interior.Color = vbRed
End Sub
2. Using the Cells Property
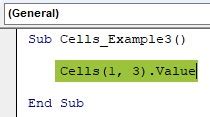
The Cells
property is another versatile way to refer to cells, especially when dealing with loops or dynamic cell references. It allows you to specify a cell by its row and column numbers, which can be particularly useful in loops.
Sub ReferCellUsingCells()
' Refer to a single cell
Cells(1, 1).Value = "Hello, World!"
' Refer to a range of cells using a loop
For i = 1 To 10
Cells(i, 1).Value = i
Next i
End Sub
3. Using Named Ranges
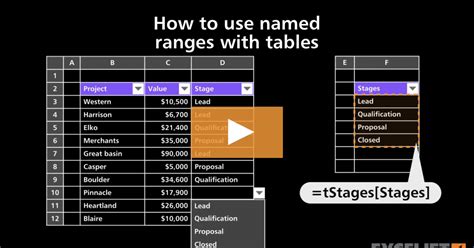
Named ranges provide a more readable and maintainable way to refer to cells or ranges. You can define a named range in the Excel interface or through VBA, and then use its name in your code.
Sub ReferCellUsingNamedRange()
' Define a named range
Range("A1").Name = "MyCell"
' Refer to the named range
Range("MyCell").Value = "Hello, World!"
End Sub
4. Using the Offset Property
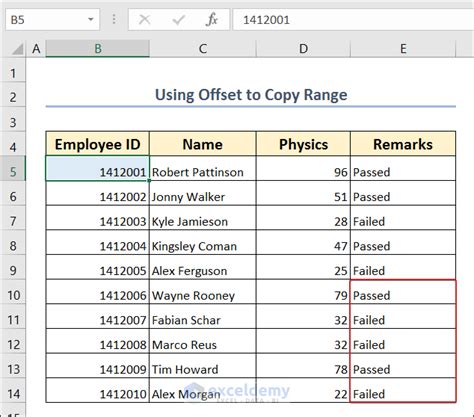
The Offset
property allows you to refer to a cell that is a specified number of rows and columns from a base cell. This can be useful for navigating relative to a known cell.
Sub ReferCellUsingOffset()
' Refer to a cell that is one row below and one column to the right of A1
Range("A1").Offset(1, 1).Value = "Hello, World!"
End Sub
5. Using the ActiveCell Property
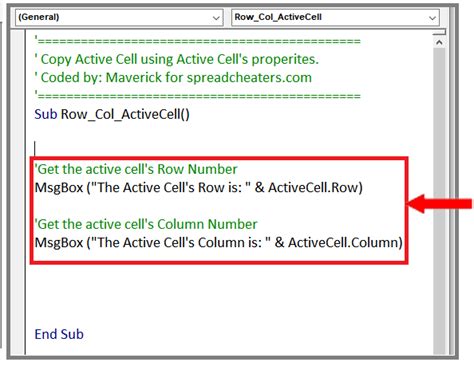
The ActiveCell
property refers to the currently active cell, which is the cell that is currently selected. This can be useful in applications where the user's selection is relevant.
Sub ReferCellUsingActiveCell()
' Refer to the active cell
ActiveCell.Value = "Hello, World!"
End Sub
Gallery of Cell Referencing Methods
Cell Referencing Methods Image Gallery
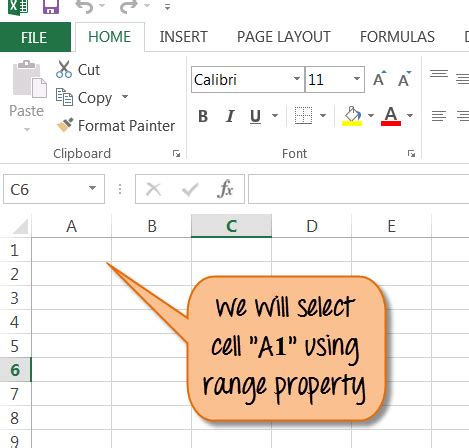

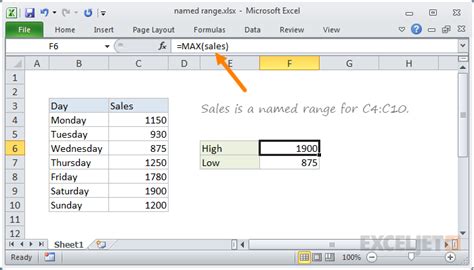
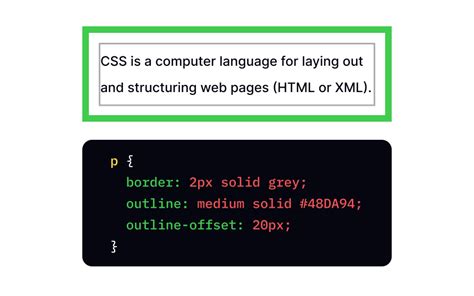
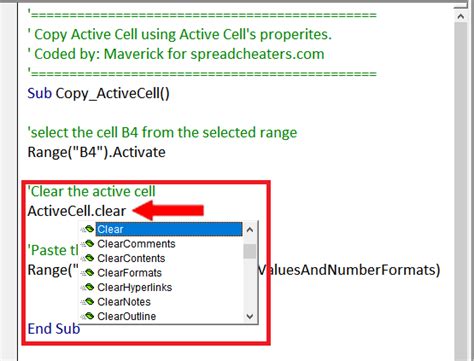
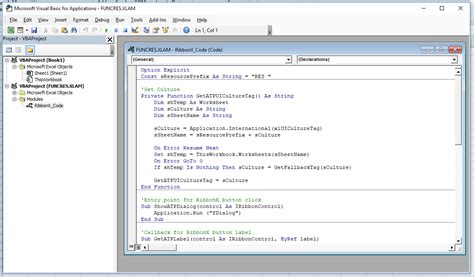
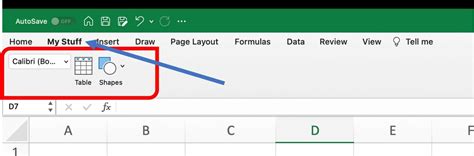
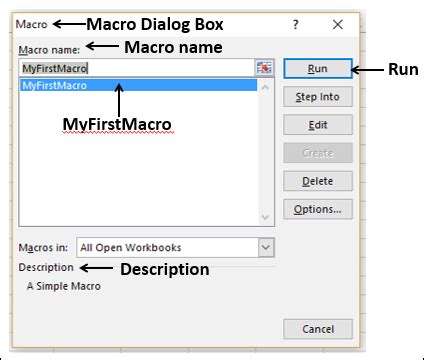
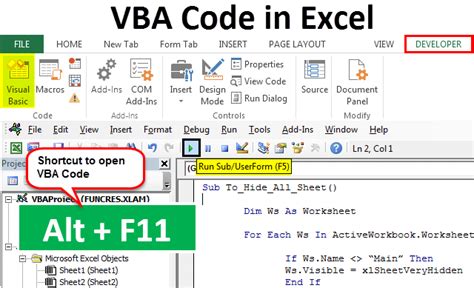
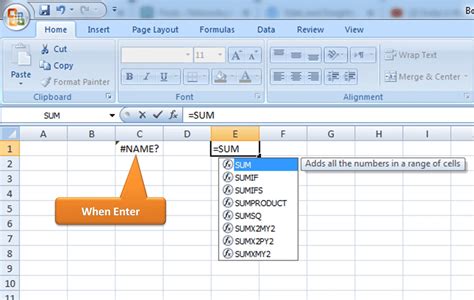
What is the difference between the Range and Cells properties in VBA?
+The Range property is used to refer to a cell or range of cells by its address, while the Cells property refers to a cell by its row and column numbers.
How do I define a named range in Excel using VBA?
+You can define a named range in VBA using the Name property of the Range object, such as Range("A1").Name = "MyRange".
What is the purpose of the Offset property in VBA?
+The Offset property is used to refer to a cell that is a specified number of rows and columns from a base cell.
In conclusion, mastering the various methods of referencing cells in VBA is crucial for any Excel automation task. By understanding the strengths and use cases of each method, you can write more efficient, readable, and maintainable code. Whether you're working with simple data manipulation or complex Excel applications, the ability to effectively reference cells is fundamental. We hope this guide has provided you with a comprehensive overview of the different methods available and has inspired you to explore the capabilities of VBA further. If you have any questions or would like to share your experiences with cell referencing in VBA, please don't hesitate to comment below. Your feedback is invaluable, and we look forward to hearing from you.