Intro
Converting strings to integers is a common task in VBA programming, often necessary when working with data that comes in as text but needs to be processed numerically. VBA provides several methods to achieve this conversion, including the use of functions like CInt()
, CLng()
, Val()
, and Int()
. Each of these functions has its own specific use cases and limitations.
When converting strings to integers, it's crucial to ensure that the string can be successfully converted to a numeric value. If the string contains non-numeric characters (excluding the leading or trailing spaces and a possible minus sign at the beginning), attempting to convert it to an integer will result in a runtime error. Therefore, it's often a good practice to validate the string before attempting the conversion.
Using CInt()
CInt()
is one of the most commonly used functions to convert a string to an integer. It converts an expression to a variant of subtype Integer. Note that CInt()
can only handle values within the range of -32,768 to 32,767.
Dim myString As String
Dim myInteger As Integer
myString = "100"
myInteger = CInt(myString)
Debug.Print myInteger ' Outputs: 100
Using CLng()
If you need to convert a string to a long integer (which can handle larger numbers, ranging from -2,147,483,648 to 2,147,483,647), you can use CLng()
.
Dim myString As String
Dim myLong As Long
myString = "30000"
myLong = CLng(myString)
Debug.Print myLong ' Outputs: 30000
Using Val()
The Val()
function converts a string to a numeric value. It's more flexible than CInt()
or CLng()
because it can handle decimal points and returns a Double
value, which can then be converted to an integer if needed. However, it stops reading the string when it encounters a character that cannot be interpreted as a number.
Dim myString As String
Dim myNumber As Double
myString = "100.50"
myNumber = Val(myString)
Debug.Print myNumber ' Outputs: 100.5
To convert the result to an integer, you can use CInt()
or CLng()
on the result of Val()
.
myInteger = CInt(Val(myString))
Error Handling
When converting strings to integers, it's essential to implement error handling to manage cases where the conversion might fail. VBA's On Error
statement can be used to catch and handle errors.
On Error Resume Next
myInteger = CInt(myString)
If Err.Number <> 0 Then
Debug.Print "Error converting string to integer."
Err.Clear
End If
On Error GoTo 0
Alternatively, you can use IsNumeric()
to check if a string can be converted to a number before attempting the conversion.
If IsNumeric(myString) Then
myInteger = CInt(myString)
Else
Debug.Print "The string cannot be converted to a number."
End If
Conclusion and Further Steps
Converting strings to integers in VBA is straightforward using functions like CInt()
, CLng()
, and Val()
. However, it's crucial to validate the input and handle potential errors to ensure robust and reliable code. Whether you're working with user input, data from external sources, or internal calculations, careful consideration of the conversion process will help you avoid common pitfalls and write more effective VBA scripts.
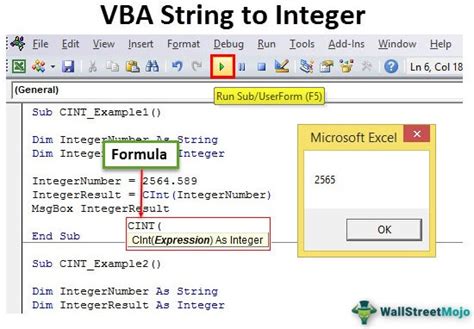
Best Practices for String to Integer Conversion
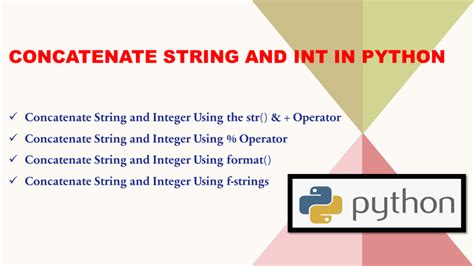
When converting strings to integers, following best practices can significantly improve the reliability and performance of your VBA code. This includes validating user input, using appropriate error handling mechanisms, and choosing the correct conversion function based on the expected range of values.
Validation and Error Handling
Validating the input string before attempting conversion is key. This can be done using the IsNumeric()
function, which checks if a string can be evaluated as a number.
If IsNumeric(myString) Then
' Conversion is safe
Else
' Handle the error
End If
Additionally, using On Error
statements or error handling blocks can help manage runtime errors that may occur during the conversion process.
Common Errors and Solutions
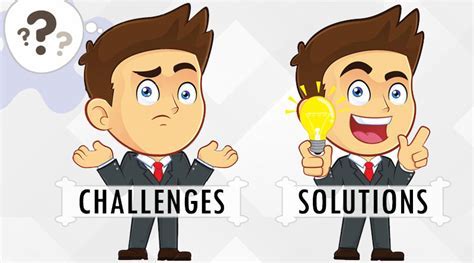
Several common errors can occur when converting strings to integers in VBA, including type mismatch errors, overflow errors, and errors due to non-numeric characters in the string.
- Type Mismatch Error: This occurs when the string cannot be converted to a number. Solution: Use
IsNumeric()
to check the string before conversion. - Overflow Error: This happens when the number is too large for the integer type. Solution: Use
CLng()
for larger numbers orCDbl()
for decimal numbers. - Non-Numeric Characters: If the string contains characters that cannot be interpreted as numbers, the conversion will fail. Solution: Clean the string before conversion or use
Val()
which ignores non-numeric characters after the number.
Optimizing Conversion Performance
For applications where performance is critical, optimizing the conversion process can be beneficial. This might involve minimizing the number of conversions, using the most appropriate conversion function for the task, and pre-validating data when possible.
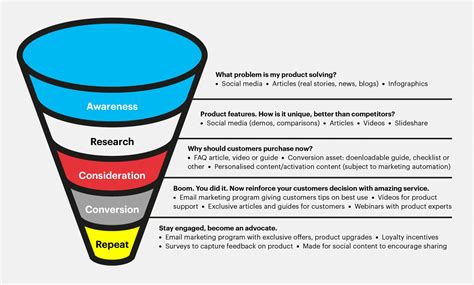
Advanced Topics and Considerations
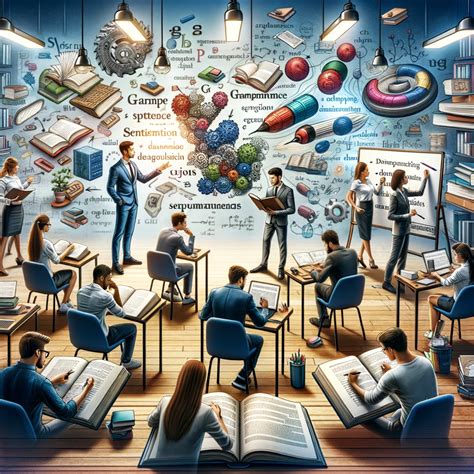
Beyond the basics of string to integer conversion, there are several advanced topics and considerations. These include handling international formats, dealing with very large numbers, and integrating conversion processes into larger data processing workflows.
International Formats and Localization
When working with strings that represent numbers in international formats, special considerations must be taken. For example, the decimal separator and thousand separator can vary by region.
' Replace commas with periods for conversion in some locales
myString = Replace(myString, ",", ".")
myNumber = Val(myString)
Gallery of String to Integer Conversion Examples
String to Integer Conversion Gallery
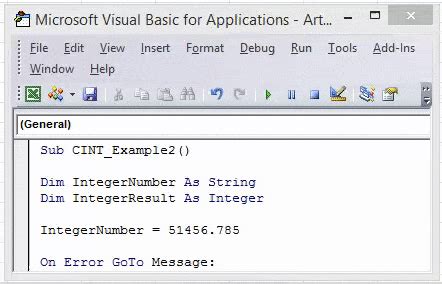
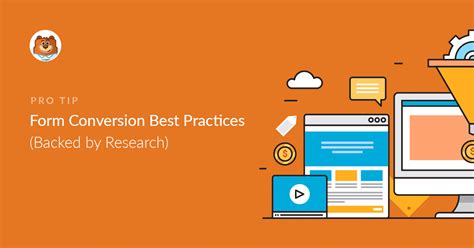
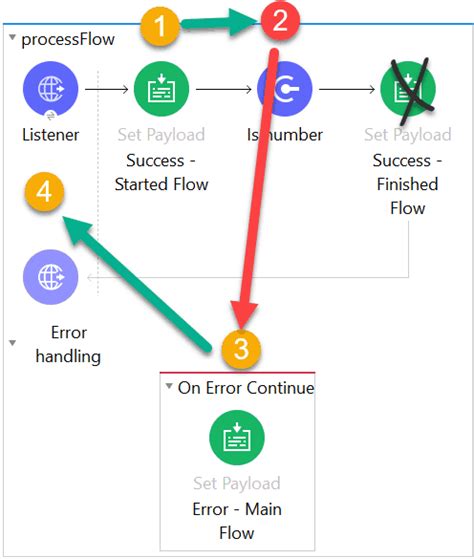
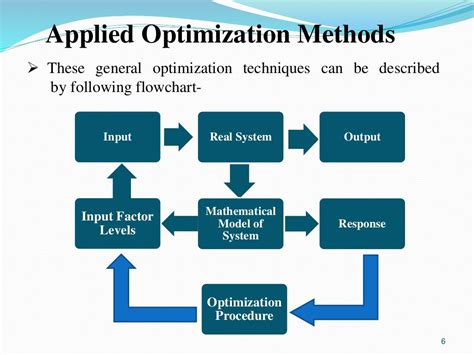
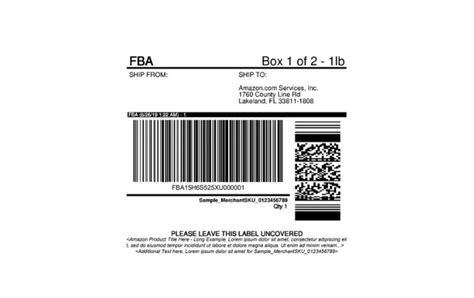
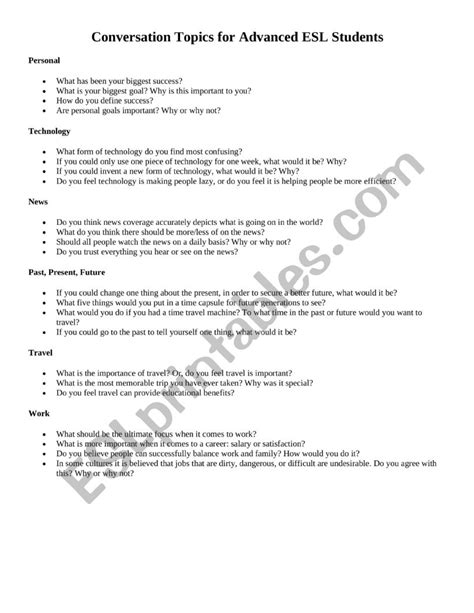
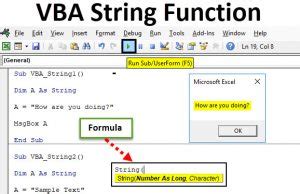
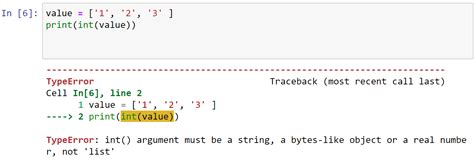
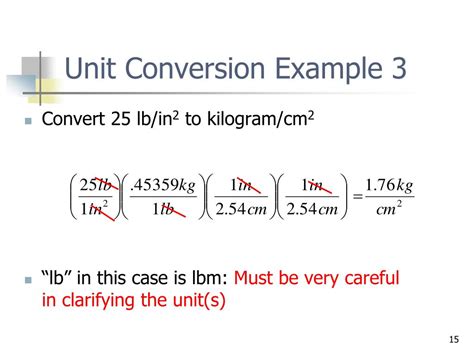
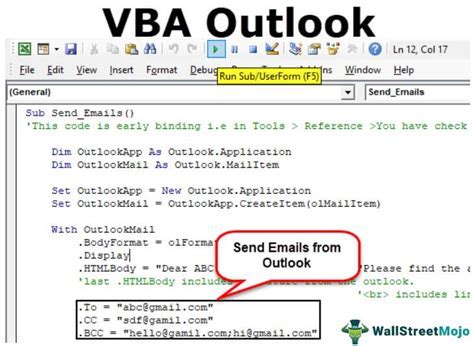
What is the purpose of converting strings to integers in VBA?
+The purpose is to enable numerical operations on data that is initially in string format, allowing for calculations, comparisons, and other operations that require numeric values.
How do I handle errors when converting strings to integers?
+You can use error handling mechanisms such as `On Error` statements or check if a string is numeric using `IsNumeric()` before attempting the conversion.
What is the difference between CInt() and CLng()?
+`CInt()` converts to an integer, which has a smaller range (-32,768 to 32,767), while `CLng()` converts to a long integer, which has a larger range (-2,147,483,648 to 2,147,483,647).
We hope this comprehensive guide to converting strings to integers in VBA has been informative and helpful. Whether you're a beginner or an experienced programmer, understanding the nuances of data type conversion is crucial for writing effective and reliable code. If you have any further questions or need more specific examples, don't hesitate to ask. Share your experiences and tips for converting strings to integers in the comments below.