Intro
The world of Visual Basic for Applications (VBA) is a powerful tool for automating tasks in Microsoft Office applications, particularly in Excel. One of the fundamental aspects of working with data in VBA is handling dates. Dates are crucial for various purposes, such as scheduling, data analysis, and reporting. VBA offers several built-in functions to manipulate and work with dates, making it easier to perform date-related tasks. In this article, we will delve into five essential VBA date functions that every developer should know.
VBA date functions are designed to simplify tasks such as calculating the difference between two dates, determining the current date, and formatting dates according to specific requirements. These functions are not only useful for basic date calculations but also for complex data analysis and automation tasks. By mastering these functions, developers can create more efficient and effective VBA applications.
The importance of understanding and utilizing VBA date functions cannot be overstated. They provide a foundation for more advanced date manipulation and analysis, enabling developers to tackle a wide range of tasks with confidence. Whether it's calculating ages, determining the number of days between two dates, or scheduling events, VBA date functions are indispensable tools in the world of Excel automation.
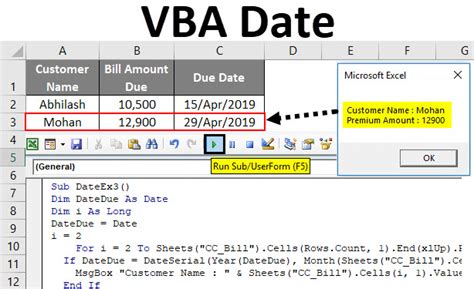
Introduction to VBA Date Functions
VBA date functions can be broadly categorized into several types, including functions for calculating dates, formatting dates, and determining the current date. Each of these categories contains a variety of functions that serve specific purposes. For instance, the Date()
function returns the current date, while the DateAdd()
function adds a specified interval to a date.
Benefits of Using VBA Date Functions
Using VBA date functions offers several benefits, including increased efficiency, accuracy, and flexibility. These functions are designed to handle date calculations and manipulations quickly and accurately, reducing the risk of human error. Moreover, they provide a flexible way to work with dates, allowing developers to perform complex date-related tasks with ease.
Working with the Date() Function
The Date()
function is one of the most basic yet essential VBA date functions. It returns the current date, which can be useful for a variety of purposes, such as logging events, scheduling tasks, or simply displaying the current date in a worksheet.
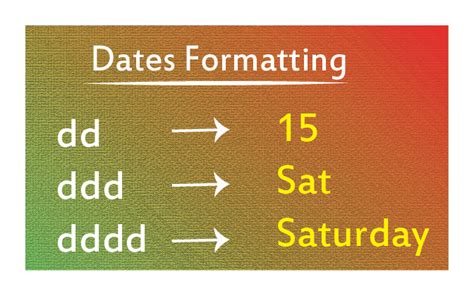
Example Usage of the Date() Function
To use the Date()
function, simply call it in your VBA code. For example:
Sub GetCurrentDate()
Dim currentDate As Date
currentDate = Date()
MsgBox "Today's date is: " & currentDate
End Sub
This code snippet declares a variable currentDate
of type Date
, assigns the current date to it using the Date()
function, and then displays the current date in a message box.
Understanding the DateAdd() Function
The DateAdd()
function is another powerful VBA date function that allows you to add a specified interval to a date. This function is particularly useful for calculating future or past dates based on a given date.
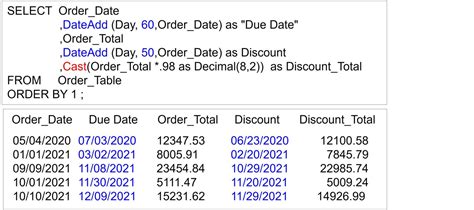
Syntax and Parameters of the DateAdd() Function
The syntax of the DateAdd()
function is as follows:
DateAdd(interval, number, date)
interval
: Specifies the interval to add. It can be one of the following:yyyy
for year,q
for quarter,m
for month,y
for day of year,d
for day,w
for weekday,ww
for week,h
for hour,n
for minute,s
for second.number
: The number of intervals to add. It can be positive (to add) or negative (to subtract).date
: The date to which the interval is added.
Example Usage of the DateAdd() Function
To add 30 days to the current date, you can use the following code:
Sub AddDaysToDate()
Dim currentDate As Date
Dim futureDate As Date
currentDate = Date()
futureDate = DateAdd("d", 30, currentDate)
MsgBox "The date 30 days from now is: " & futureDate
End Sub
This example calculates the date 30 days from the current date and displays it in a message box.
Using the DateDiff() Function
The DateDiff()
function calculates the difference between two dates in a specified interval. This function is useful for determining how many days, months, or years have passed between two dates.
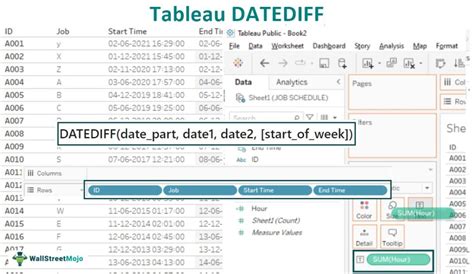
Syntax and Parameters of the DateDiff() Function
The syntax of the DateDiff()
function is as follows:
DateDiff(interval, date1, date2, [firstdayofweek], [firstweekofyear])
interval
: Specifies the interval to use when calculating the difference. It can be one of the following:yyyy
for year,q
for quarter,m
for month,y
for day of year,d
for day,w
for weekday,ww
for week,h
for hour,n
for minute,s
for second.date1
anddate2
: The two dates to calculate the difference between.[firstdayofweek]
: Optional. Specifies the first day of the week. If not specified, Sunday is assumed.[firstweekofyear]
: Optional. Specifies the first week of the year. If not specified, the first week is assumed to start on January 1.
Example Usage of the DateDiff() Function
To calculate the number of days between two dates, you can use the following code:
Sub CalculateDaysBetweenDates()
Dim startDate As Date
Dim endDate As Date
Dim daysBetween As Long
startDate = #1/1/2022#
endDate = #12/31/2022#
daysBetween = DateDiff("d", startDate, endDate)
MsgBox "There are " & daysBetween & " days between the start and end dates."
End Sub
This example calculates the number of days between January 1, 2022, and December 31, 2022, and displays the result in a message box.
Utilizing the DatePart() Function
The DatePart()
function returns a specified part of a date, such as the year, month, or day. This function is useful for extracting specific components of a date.
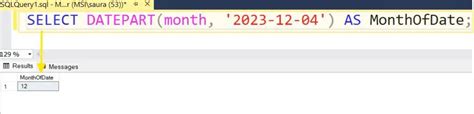
Syntax and Parameters of the DatePart() Function
The syntax of the DatePart()
function is as follows:
DatePart(interval, date, [firstdayofweek], [firstweekofyear])
interval
: Specifies the part of the date to return. It can be one of the following:yyyy
for year,q
for quarter,m
for month,y
for day of year,d
for day,w
for weekday,ww
for week,h
for hour,n
for minute,s
for second.date
: The date from which to extract the part.[firstdayofweek]
: Optional. Specifies the first day of the week. If not specified, Sunday is assumed.[firstweekofyear]
: Optional. Specifies the first week of the year. If not specified, the first week is assumed to start on January 1.
Example Usage of the DatePart() Function
To extract the year from a date, you can use the following code:
Sub ExtractYearFromDate()
Dim currentDate As Date
Dim year As Integer
currentDate = Date()
year = DatePart("yyyy", currentDate)
MsgBox "The current year is: " & year
End Sub
This example extracts the year from the current date and displays it in a message box.
Employing the DateSerial() Function
The DateSerial()
function returns a date given the year, month, and day. This function is useful for creating dates from separate year, month, and day components.
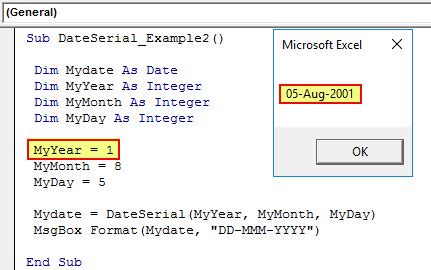
Syntax and Parameters of the DateSerial() Function
The syntax of the DateSerial()
function is as follows:
DateSerial(year, month, day)
year
: The year of the date.month
: The month of the date.day
: The day of the date.
Example Usage of the DateSerial() Function
To create a date from separate year, month, and day components, you can use the following code:
Sub CreateDateFromComponents()
Dim year As Integer
Dim month As Integer
Dim day As Integer
Dim dateCreated As Date
year = 2022
month = 12
day = 25
dateCreated = DateSerial(year, month, day)
MsgBox "The created date is: " & dateCreated
End Sub
This example creates a date from the year 2022, month 12, and day 25, and displays the created date in a message box.
VBA Date Functions Image Gallery
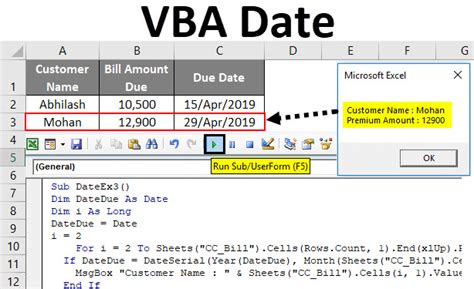
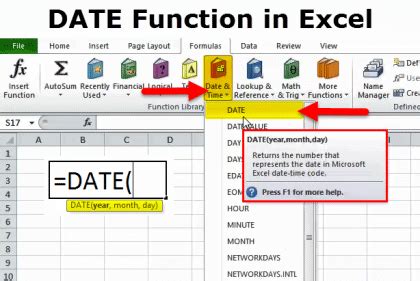
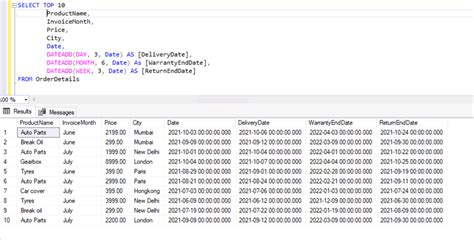
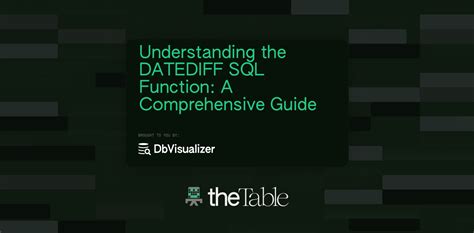
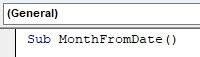
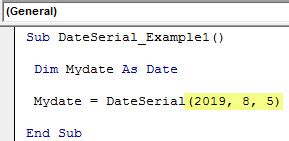
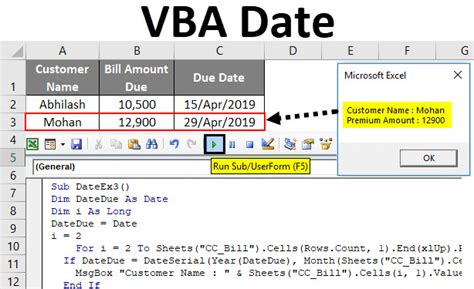
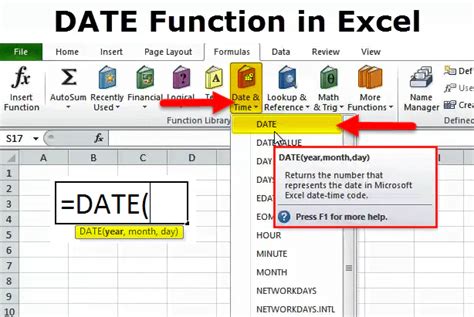
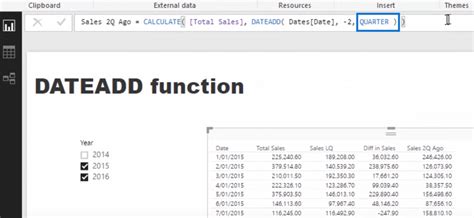
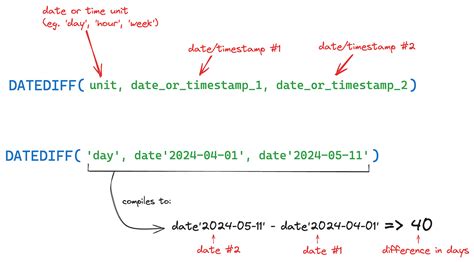
What is the purpose of the Date() function in VBA?
+The Date() function returns the current date, which can be useful for logging events, scheduling tasks, or displaying the current date in a worksheet.
How do I use the DateAdd() function to add a specified interval to a date?
+The DateAdd() function adds a specified interval to a date. For example, DateAdd("d", 30, Date()) adds 30 days to the current date.
What is the difference between the DateDiff() and DateAdd() functions?
+The DateDiff() function calculates the difference between two dates in a specified interval, while the DateAdd() function adds a specified interval to a date.
How do I extract a specific part of a date using the DatePart() function?
+The DatePart() function returns a specified part of a date, such as the year, month, or day. For example, DatePart("yyyy", Date()) extracts the year from the current date.
What is the purpose of the DateSerial() function in VBA?
+The DateSerial() function returns a date given the year, month, and day. It is useful for creating dates from separate year, month, and day components.
In conclusion, mastering VBA date functions is essential for any developer working with Excel automation. These functions provide a powerful toolset for manipulating and analyzing dates, enabling developers to create more efficient and effective VBA applications. By understanding and utilizing these functions, developers can tackle a wide range of date-related tasks with confidence. Whether you're a beginner or an experienced developer, exploring the world of VBA date functions can help you unlock new possibilities in Excel automation. We invite you to share your experiences, ask questions, or provide feedback on this article, and we look forward to hearing from you.