Intro
Creating a new workbook in Excel using Visual Basic for Applications (VBA) is a straightforward process that can be accomplished with a few lines of code. This can be particularly useful for automating tasks, such as generating reports or creating templates, where you need to create new workbooks programmatically.
To start working with VBA in Excel, you first need to access the Visual Basic Editor. You can do this by pressing Alt
+ F11
or by navigating to the Developer tab (if available) and clicking on the Visual Basic button. If the Developer tab is not visible, you can add it by going to File > Options > Customize Ribbon and checking the Developer checkbox.
Creating a New Workbook
Once you have the Visual Basic Editor open, you can create a new module to write your code. To do this, right-click on any of the objects for your workbook in the Project Explorer on the left side, go to Insert, and then click on Module. This action creates a new module where you can write your VBA code.
Here's a simple example of VBA code that creates a new workbook:
Sub CreateNewWorkbook()
Workbooks.Add
End Sub
To run this code, press F5
while in the Visual Basic Editor with the module containing this code active, or close the Visual Basic Editor and run the macro from the Developer tab in Excel.
Saving the New Workbook
If you want to save the new workbook immediately after creating it, you can use the SaveAs
method. Here's how you can modify the previous code to save the workbook:
Sub CreateAndSaveNewWorkbook()
Dim newWorkbook As Workbook
Set newWorkbook = Workbooks.Add
newWorkbook.SaveAs "C:\Path\To\Save\YourWorkbook.xlsx"
End Sub
Make sure to replace "C:\Path\To\Save\YourWorkbook.xlsx"
with the actual path and filename you want to use.
Adding Worksheets to the New Workbook
After creating a new workbook, you might want to add worksheets to it. Here's an example of how to add a new worksheet:
Sub CreateNewWorkbookAndAddWorksheet()
Dim newWorkbook As Workbook
Set newWorkbook = Workbooks.Add
' Add a new worksheet
newWorkbook.Worksheets.Add
' Save the workbook
newWorkbook.SaveAs "C:\Path\To\Save\YourWorkbook.xlsx"
End Sub
Copying Data to the New Workbook
If you need to copy data from the current workbook to the new one, you can do so by referencing the worksheets and ranges directly:
Sub CreateNewWorkbookAndCopyData()
Dim sourceWorkbook As Workbook
Dim targetWorkbook As Workbook
Dim sourceSheet As Worksheet
Dim targetSheet As Worksheet
Set sourceWorkbook = ThisWorkbook
Set targetWorkbook = Workbooks.Add
Set sourceSheet = sourceWorkbook.Sheets("Sheet1")
Set targetSheet = targetWorkbook.Sheets(1)
' Copy data from source to target
sourceSheet.Range("A1:B10").Copy Destination:=targetSheet.Range("A1")
' Save the target workbook
targetWorkbook.SaveAs "C:\Path\To\Save\YourWorkbook.xlsx"
End Sub
This example copies data from the range A1:B10
in Sheet1
of the current workbook to the first sheet of the new workbook, starting from cell A1
.
Tips and Variations
- Working with Multiple Workbooks: When working with multiple workbooks, it's essential to keep track of which workbook and worksheet you're referencing to avoid errors.
- Error Handling: Always consider adding error handling to your code, especially when dealing with file operations like saving, to handle potential issues such as insufficient permissions or file already exists.
- Customization: Depending on your needs, you might want to customize the new workbook further, such as setting the worksheet layout, adding headers or footers, or applying specific formatting.
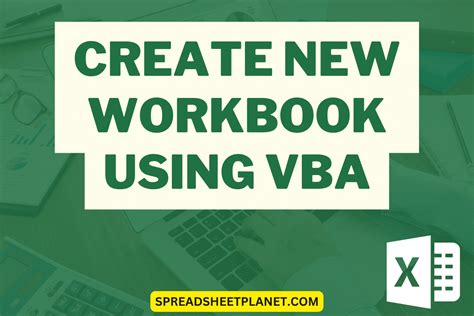
Gallery of Excel VBA Examples
VBA Excel Gallery
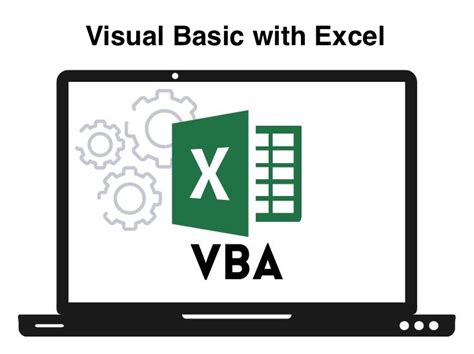
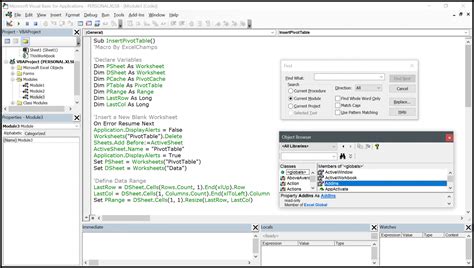
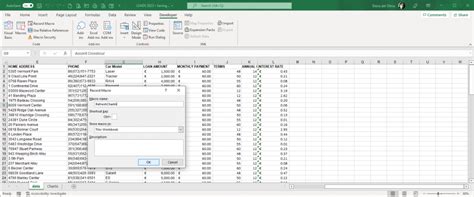
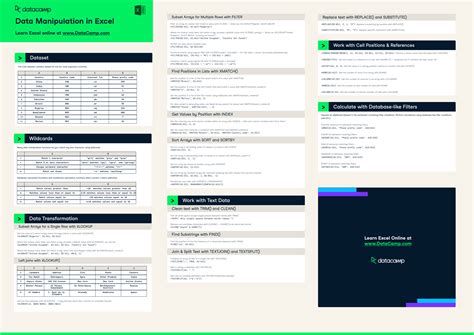
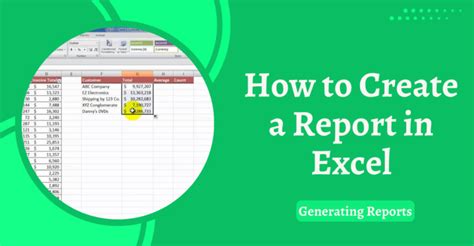
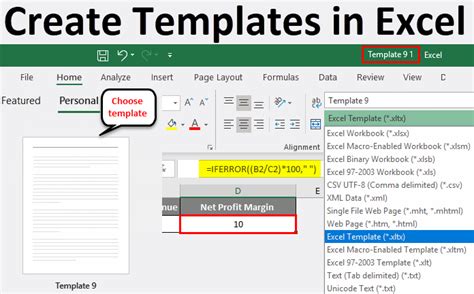
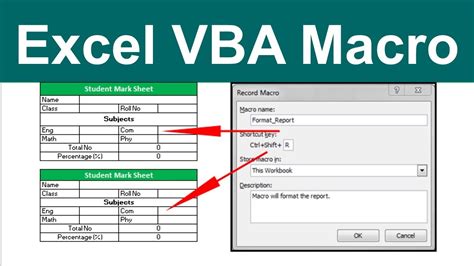
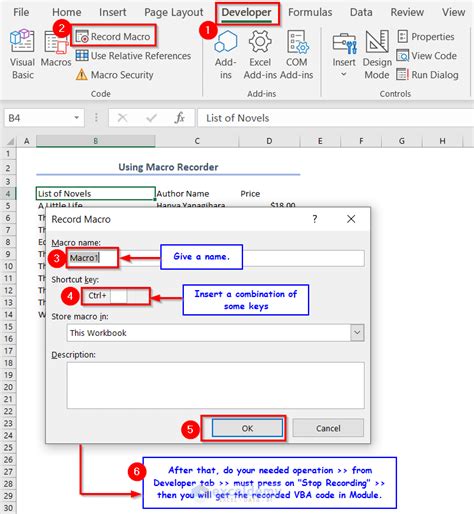
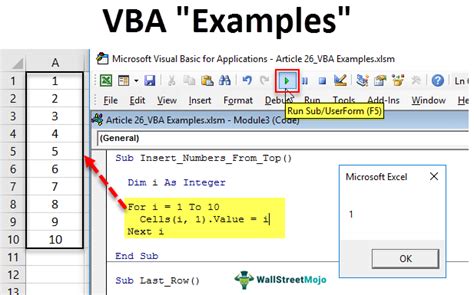
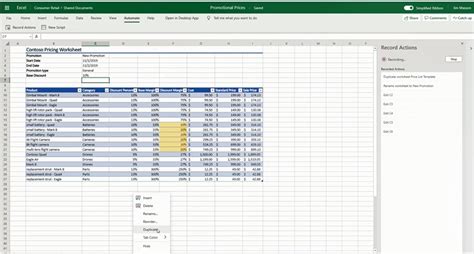
Frequently Asked Questions
What is VBA in Excel?
+VBA stands for Visual Basic for Applications. It's a programming language built into Excel that allows users to create and automate tasks, from simple macros to complex applications.
How do I enable the Developer tab in Excel?
+To enable the Developer tab, go to File > Options > Customize Ribbon, and then check the Developer checkbox in the list of available main tabs.
What are the benefits of using VBA in Excel?
+The benefits of using VBA in Excel include increased productivity through automation, the ability to perform complex tasks that are not possible with standard Excel functions, and enhanced customization capabilities.
If you've made it this far, you're likely interested in diving deeper into the world of Excel VBA. Whether you're looking to automate routine tasks, create complex applications, or simply explore the possibilities of what VBA can do, there's a wealth of information and resources available. From online tutorials and forums to books and courses, the opportunities to learn and grow with VBA are vast. So, take the next step, experiment with the code examples provided, and see where your journey with Excel VBA takes you. Don't hesitate to share your experiences, ask questions, or provide feedback in the comments below. Your input is invaluable in helping others navigate the fascinating world of Excel automation.