Intro
Conditional formatting is a powerful tool in Excel that allows users to highlight cells based on specific conditions, making it easier to analyze and understand data. Visual Basic for Applications (VBA) can be used to create and manage conditional formatting rules in Excel. In this article, we will explore the importance of VBA for conditional formatting and how it can be used to automate and enhance the formatting of Excel spreadsheets.
Conditional formatting is a crucial aspect of data analysis, as it enables users to quickly identify trends, patterns, and anomalies in their data. By applying conditional formatting rules, users can highlight cells that meet specific conditions, such as values above or below a certain threshold, or cells that contain specific text or formulas. This can help users to focus on the most important data and make informed decisions.
VBA can be used to create and manage conditional formatting rules in Excel, allowing users to automate the formatting process and apply complex rules to their data. With VBA, users can create macros that apply conditional formatting rules to specific ranges or worksheets, or even entire workbooks. This can save time and improve the consistency of formatting across multiple spreadsheets.
Benefits of Using VBA for Conditional Formatting
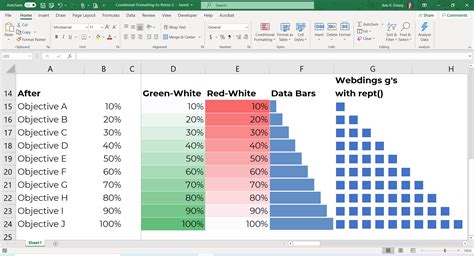
There are several benefits to using VBA for conditional formatting, including:
- Automation: VBA can automate the formatting process, saving time and reducing the risk of errors.
- Consistency: VBA can apply consistent formatting rules across multiple spreadsheets, ensuring that all data is presented in a uniform manner.
- Flexibility: VBA can be used to create complex formatting rules that are not possible with Excel's built-in conditional formatting tools.
- Customization: VBA can be used to create custom formatting rules that meet the specific needs of a user or organization.
How to Use VBA for Conditional Formatting
To use VBA for conditional formatting, users must first open the Visual Basic Editor in Excel. This can be done by pressing Alt + F11 or by navigating to the Developer tab and clicking on the Visual Basic button.Once the Visual Basic Editor is open, users can create a new module by clicking on the Insert menu and selecting Module. This will create a new module where users can write their VBA code.
Creating Conditional Formatting Rules with VBA
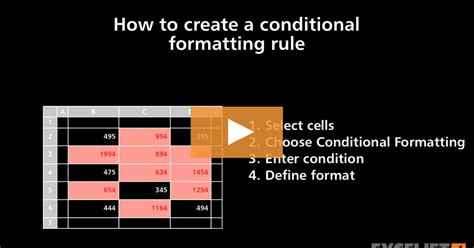
To create a conditional formatting rule with VBA, users must use the FormatConditions object. This object allows users to create and manage formatting rules for a specific range or worksheet.
Here is an example of how to create a simple conditional formatting rule with VBA:
Sub CreateConditionalFormattingRule()
Dim rng As Range
Set rng = Range("A1:A10")
With rng
.FormatConditions.Add Type:=xlCellValue, Operator:=xlGreater, Formula1:="=10"
.FormatConditions(.FormatConditions.Count).SetFirstPriority
.FormatConditions(1).Font.Color = vbRed
.FormatConditions(1).Interior.Color = vbYellow
End With
End Sub
This code creates a conditional formatting rule that highlights cells in the range A1:A10 that have a value greater than 10. The rule is applied to the font and interior of the cells, with the font color set to red and the interior color set to yellow.
Applying Conditional Formatting Rules to Multiple Ranges
VBA can also be used to apply conditional formatting rules to multiple ranges or worksheets. This can be done by using the FormatConditions object in combination with the Range object.Here is an example of how to apply a conditional formatting rule to multiple ranges:
Sub ApplyConditionalFormattingRule()
Dim rng1 As Range
Dim rng2 As Range
Set rng1 = Range("A1:A10")
Set rng2 = Range("B1:B10")
With rng1
.FormatConditions.Add Type:=xlCellValue, Operator:=xlGreater, Formula1:="=10"
.FormatConditions(.FormatConditions.Count).SetFirstPriority
.FormatConditions(1).Font.Color = vbRed
.FormatConditions(1).Interior.Color = vbYellow
End With
With rng2
.FormatConditions.Add Type:=xlCellValue, Operator:=xlGreater, Formula1:="=20"
.FormatConditions(.FormatConditions.Count).SetFirstPriority
.FormatConditions(1).Font.Color = vbBlue
.FormatConditions(1).Interior.Color = vbGreen
End With
End Sub
This code applies two separate conditional formatting rules to the ranges A1:A10 and B1:B10. The first rule highlights cells in the range A1:A10 that have a value greater than 10, while the second rule highlights cells in the range B1:B10 that have a value greater than 20.
Managing Conditional Formatting Rules with VBA
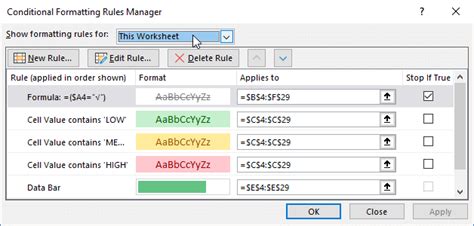
VBA can also be used to manage conditional formatting rules, including deleting, modifying, and prioritizing rules.
Here is an example of how to delete a conditional formatting rule with VBA:
Sub DeleteConditionalFormattingRule()
Dim rng As Range
Set rng = Range("A1:A10")
With rng
.FormatConditions.Delete
End With
End Sub
This code deletes all conditional formatting rules applied to the range A1:A10.
Best Practices for Using VBA for Conditional Formatting
When using VBA for conditional formatting, there are several best practices to keep in mind, including:- Use meaningful variable names and comments to make your code easy to understand and maintain.
- Use the FormatConditions object to create and manage formatting rules, rather than relying on Excel's built-in conditional formatting tools.
- Test your code thoroughly to ensure that it works as intended and does not produce any errors.
- Use error handling to catch and handle any errors that may occur when running your code.
Common Errors and Troubleshooting
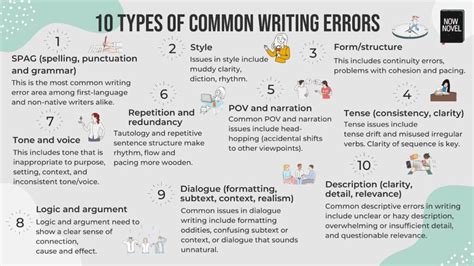
When using VBA for conditional formatting, there are several common errors and troubleshooting issues to be aware of, including:
- Errors when creating or deleting formatting rules, such as "Runtime error 1004: Application-defined or object-defined error".
- Errors when applying formatting rules to multiple ranges, such as "Runtime error 91: Object variable or With block variable not set".
- Issues with formatting rules not being applied correctly, such as rules being overridden by other formatting rules.
To troubleshoot these issues, users can try the following:
- Check the syntax of their code to ensure that it is correct and free of errors.
- Use the Debug.Print statement to print the value of variables and expressions to the Immediate window, helping to identify where errors are occurring.
- Use the Step Into and Step Over buttons to step through their code line by line, helping to identify where errors are occurring.
Conditional Formatting Image Gallery
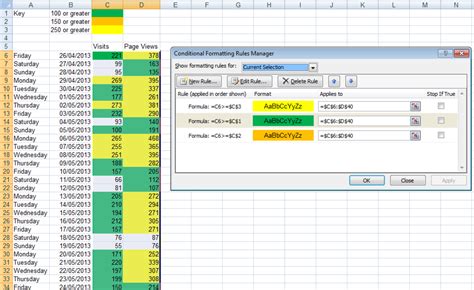
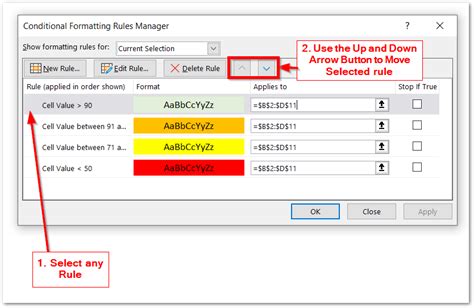
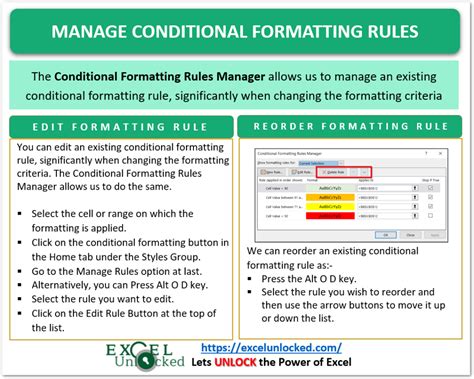
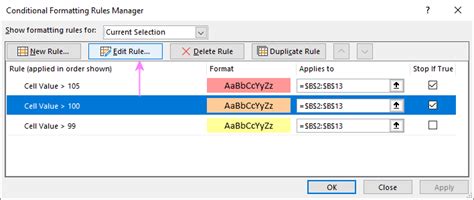
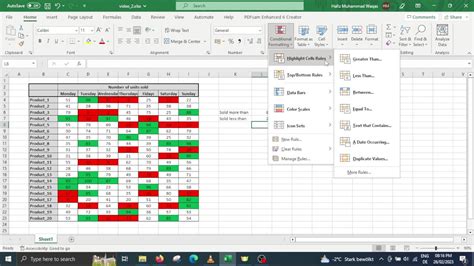
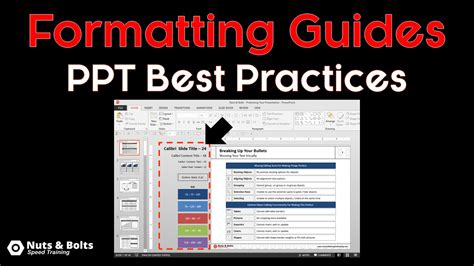
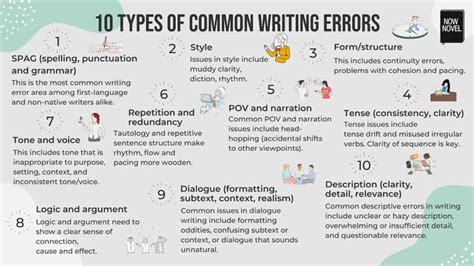
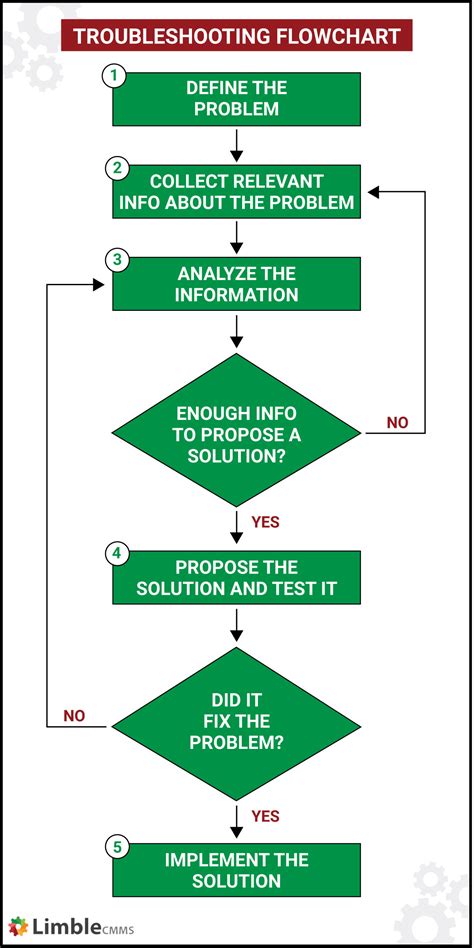
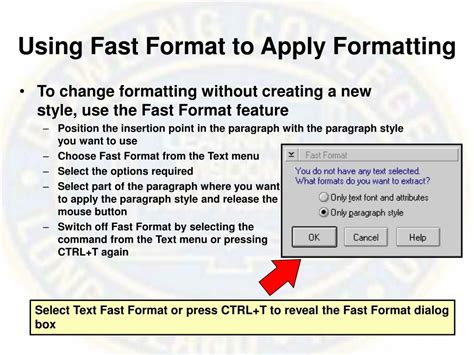
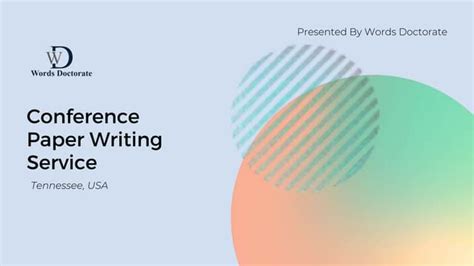
What is conditional formatting in Excel?
+Conditional formatting is a feature in Excel that allows users to highlight cells based on specific conditions, such as values above or below a certain threshold, or cells that contain specific text or formulas.
How do I create a conditional formatting rule in Excel?
+To create a conditional formatting rule in Excel, select the range of cells you want to format, go to the Home tab, click on the Conditional Formatting button, and select the type of rule you want to create.
Can I use VBA to create and manage conditional formatting rules in Excel?
+Yes, you can use VBA to create and manage conditional formatting rules in Excel. VBA provides a powerful and flexible way to automate and enhance the formatting of Excel spreadsheets.
What are some common errors and troubleshooting issues when using VBA for conditional formatting?
+Some common errors and troubleshooting issues when using VBA for conditional formatting include errors when creating or deleting formatting rules, issues with formatting rules not being applied correctly, and problems with VBA code not running as expected.
How can I learn more about using VBA for conditional formatting in Excel?
+There are many resources available to learn more about using VBA for conditional formatting in Excel, including online tutorials, videos, and forums. You can also try experimenting with different VBA code and techniques to see what works best for your specific needs.
In summary, VBA is a powerful tool for creating and managing conditional formatting rules in Excel. By using VBA, users can automate and enhance the formatting of their spreadsheets, making it easier to analyze and understand their data. Whether you are a beginner or an advanced user, VBA can help you to take your conditional formatting skills to the next level. We hope this article has provided you with a comprehensive overview of the benefits and techniques of using VBA for conditional formatting. If you have any questions or comments, please don't hesitate to share them with us.