Intro
Entering numerical data is a common requirement in many applications, and ensuring that users can only input numbers is crucial for maintaining data integrity and preventing errors. In Visual Basic for Applications (VBA), you can use an InputBox to get user input, but by default, it accepts any type of input. To restrict the InputBox to accept numbers only, you can use a combination of VBA's InputBox function and some error handling. Here's how you can achieve this:
Basic Example of Restricting InputBox to Numbers Only
The following VBA code snippet demonstrates a basic way to ensure that the InputBox only accepts numbers:
Sub GetNumberInput()
Dim userInput As Variant
Do
userInput = InputBox("Please enter a number", "Number Input")
If IsNumeric(userInput) Then
' Input is a number, exit the loop
Exit Do
ElseIf userInput = "" Then
' User clicked Cancel, exit the loop
Exit Do
Else
' Input is not a number, show an error message
MsgBox "Invalid input. Please enter a number.", vbExclamation, "Error"
End If
Loop
If userInput <> "" Then
' User entered a valid number, you can use it here
MsgBox "You entered: " & userInput, vbInformation, "Success"
Else
' User clicked Cancel without entering anything
MsgBox "No input provided.", vbInformation, "No Input"
End If
End Sub
How It Works
- Looping Input: The code uses a
Do
loop to continuously prompt the user for input until a valid number is entered or the user clicks Cancel. - InputBox: The
InputBox
function is used to get user input. It returns a string, so we need to check if this string can be converted to a number. - IsNumeric Function: The
IsNumeric
function checks if the input string can be converted to a number. If it returnsTrue
, the input is a number, and we exit the loop. - Error Handling: If
IsNumeric
returnsFalse
, an error message is displayed, prompting the user to enter a valid number. - Cancel Button Handling: If the user clicks the Cancel button, the
InputBox
returns an empty string (""
), which is also handled by exiting the loop.
Advanced Example with More Validation
For more complex scenarios, you might want to validate the input further, such as checking if it's within a certain range or if it's an integer. Here's an example that checks for integer input within a specific range:
Sub GetIntegerInputWithinRange()
Dim userInput As Variant
Dim minVal As Integer: minVal = 1
Dim maxVal As Integer: maxVal = 100
Do
userInput = InputBox("Please enter an integer between " & minVal & " and " & maxVal, "Integer Input")
If userInput = "" Then
' User clicked Cancel, exit the loop
Exit Do
ElseIf IsNumeric(userInput) Then
If CInt(userInput) >= minVal And CInt(userInput) <= maxVal Then
' Input is a valid integer within the range, exit the loop
Exit Do
Else
' Input is a number but out of range, show an error message
MsgBox "Please enter an integer between " & minVal & " and " & maxVal, vbExclamation, "Out of Range"
End If
Else
' Input is not a number, show an error message
MsgBox "Invalid input. Please enter an integer.", vbExclamation, "Error"
End If
Loop
If userInput <> "" Then
' User entered a valid integer within the range, you can use it here
MsgBox "You entered: " & userInput, vbInformation, "Success"
Else
' User clicked Cancel without entering anything
MsgBox "No input provided.", vbInformation, "No Input"
End If
End Sub
Conclusion and Next Steps
Restricting the InputBox to accept numbers only in VBA involves using a loop to continuously prompt the user until valid input is provided, along with the IsNumeric
function to check if the input can be converted to a number. By incorporating more validation, such as range checking, you can ensure that your application receives the correct type of data from the user, enhancing its reliability and user experience. For further enhancements, consider exploring more advanced input validation techniques and error handling strategies in VBA.
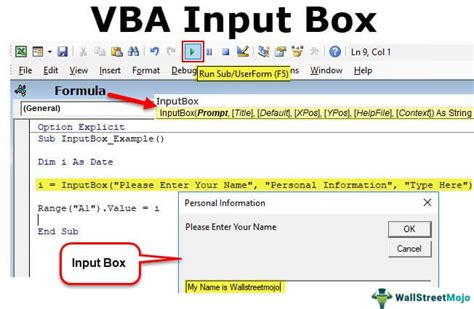
Understanding VBA InputBox
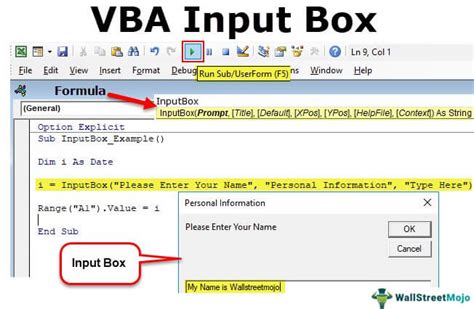
Advanced Input Validation
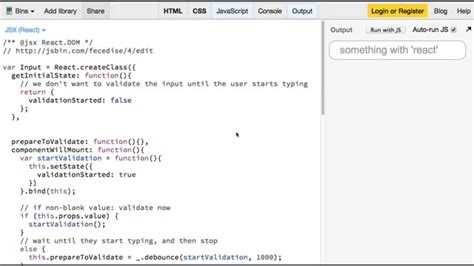
Error Handling in VBA
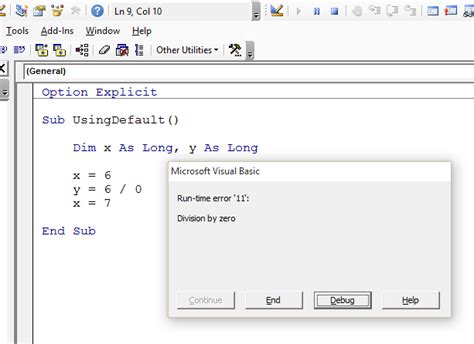
VBA InputBox Best Practices
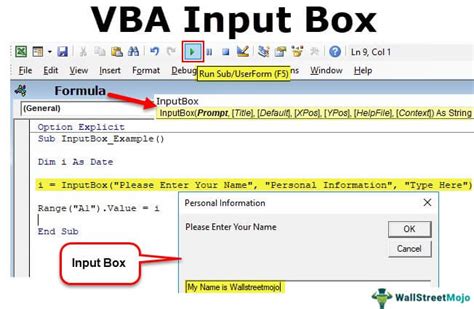
VBA for Beginners
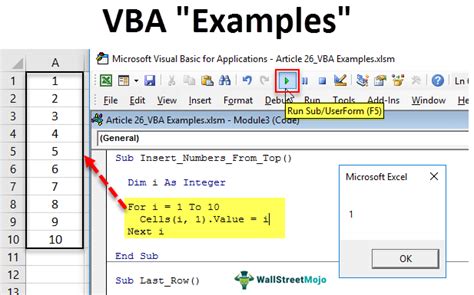
VBA InputBox Examples
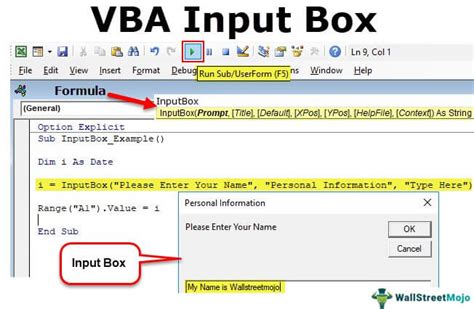
VBA Tutorials
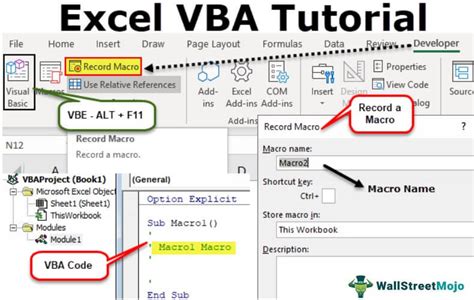
VBA InputBox Tips
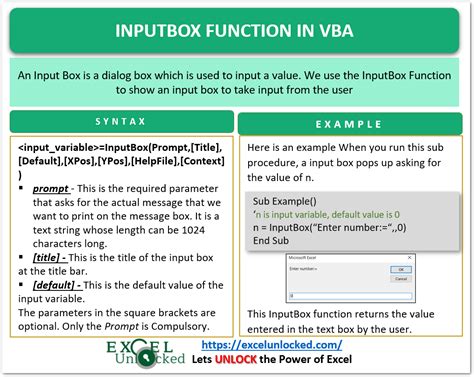
VBA Community
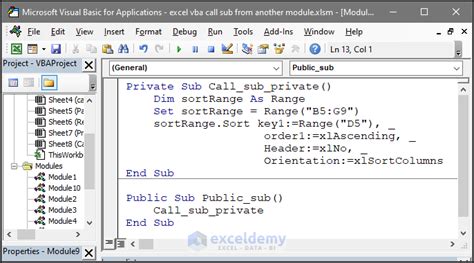
VBA InputBox Gallery
VBA InputBox Image Gallery
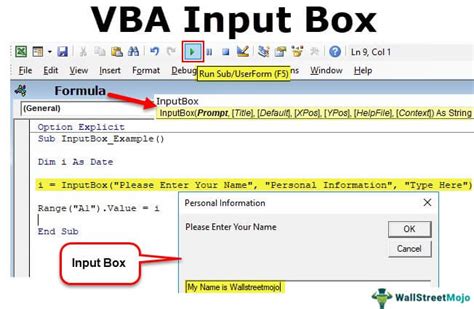
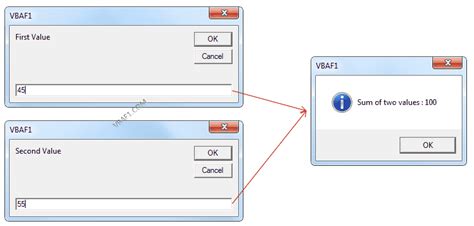
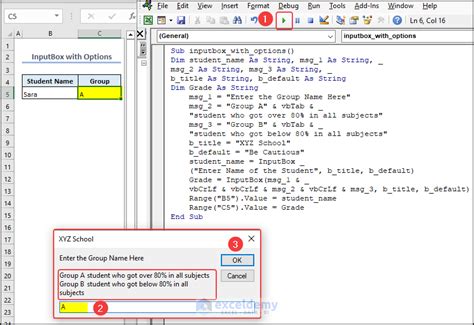
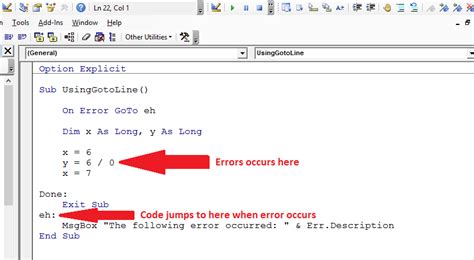
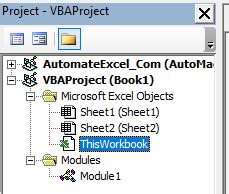
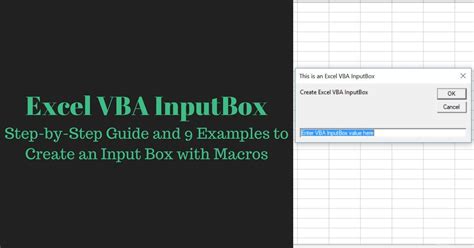
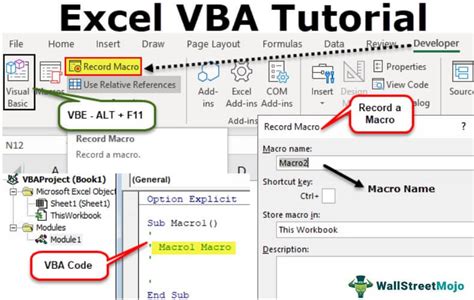
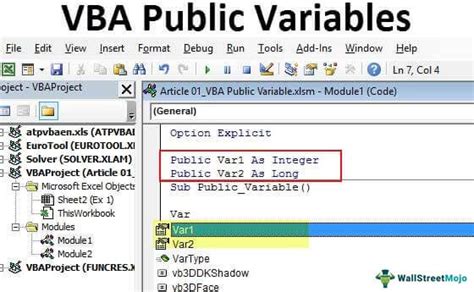
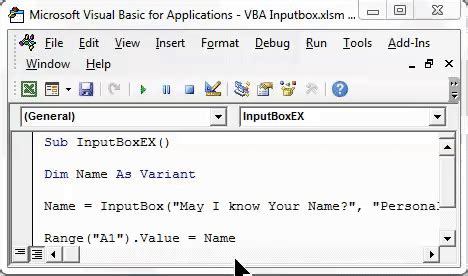
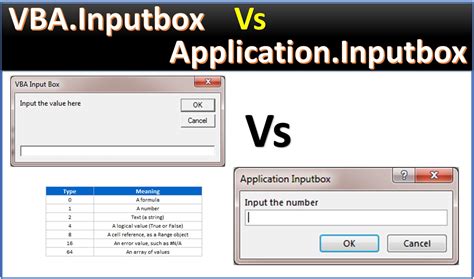
What is VBA InputBox used for?
+VBA InputBox is used to get user input in the form of text or numbers, allowing for interaction between the user and the VBA application.
How do I restrict InputBox to accept numbers only?
+You can use a loop with the IsNumeric function to check if the input is a number. If not, display an error message and prompt the user to enter a number.
Can I validate the input range in VBA InputBox?
+Yes, you can validate the input range by checking if the input number falls within a specified range. Use conditional statements to handle inputs outside the desired range.
To learn more about VBA and its applications, or to share your experiences with using VBA InputBox for number input, feel free to comment below. Your insights and questions are valuable to the community, and discussing them can lead to new ideas and solutions. If you found this article helpful, consider sharing it with others who might benefit from understanding how to restrict VBA InputBox to accept numbers only.