Intro
Convert PDF to Excel with VBA, leveraging macros and scripting to extract data, automate tasks, and integrate PDF content into spreadsheets for efficient data analysis and management.
The ability to convert PDF files to Excel spreadsheets is a valuable skill, especially for those working with large datasets or financial information. Visual Basic for Applications (VBA) is a powerful tool that can be used to automate this process, saving time and reducing the risk of errors. In this article, we will explore the importance of converting PDF to Excel, the benefits of using VBA, and provide a step-by-step guide on how to achieve this using VBA.
Converting PDF files to Excel spreadsheets is a common task in many industries, including finance, accounting, and data analysis. PDF files are often used to share and distribute reports, invoices, and other documents, but they can be difficult to work with when it comes to data analysis. Excel, on the other hand, is a powerful tool for data analysis and manipulation, making it an ideal format for working with large datasets. By converting PDF files to Excel, users can easily extract and manipulate the data, perform calculations, and create charts and graphs.
VBA is a programming language developed by Microsoft that is used to create and automate tasks in Microsoft Office applications, including Excel. VBA is a powerful tool that can be used to automate repetitive tasks, create custom tools and interfaces, and even interact with other applications. When it comes to converting PDF files to Excel, VBA can be used to automate the process, making it faster and more efficient.
Benefits of Using VBA to Convert PDF to Excel
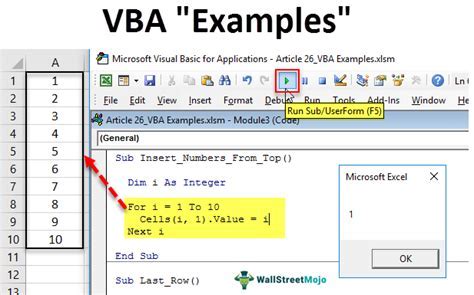
There are several benefits to using VBA to convert PDF files to Excel. One of the main advantages is speed. VBA can automate the process of converting PDF files to Excel, saving time and reducing the risk of errors. Additionally, VBA can be used to create custom tools and interfaces, making it easier to work with PDF files and extract the data needed. VBA can also be used to interact with other applications, such as Adobe Acrobat, to automate the conversion process.
Another benefit of using VBA to convert PDF files to Excel is flexibility. VBA can be used to create custom scripts and macros that can be tailored to specific needs and requirements. This means that users can create scripts that are designed to work with specific types of PDF files or data, making it easier to extract and manipulate the data needed.
How to Use VBA to Convert PDF to Excel
To use VBA to convert PDF files to Excel, users will need to have Microsoft Excel and Adobe Acrobat installed on their computer. They will also need to have a basic understanding of VBA programming and how to create and run macros in Excel.The first step in using VBA to convert PDF files to Excel is to create a new macro in Excel. This can be done by opening the Visual Basic Editor in Excel and creating a new module. Once the module is created, users can start writing their VBA code.
One of the most common methods for converting PDF files to Excel using VBA is to use the Adobe Acrobat library. This library provides a set of functions and objects that can be used to interact with Adobe Acrobat and automate the conversion process.
To use the Adobe Acrobat library, users will need to add a reference to the library in their VBA project. This can be done by opening the Visual Basic Editor and selecting "Tools" > "References" from the menu. Once the references dialog box is open, users can scroll down and check the box next to "Adobe Acrobat xx.x Type Library", where "xx.x" is the version of Adobe Acrobat installed on their computer.
Step-by-Step Guide to Converting PDF to Excel using VBA
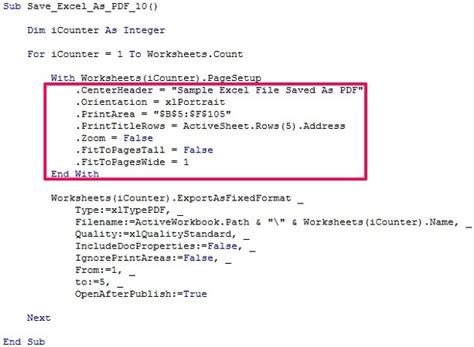
Here is a step-by-step guide to converting PDF files to Excel using VBA:
- Open the Visual Basic Editor in Excel and create a new module.
- Add a reference to the Adobe Acrobat library by selecting "Tools" > "References" from the menu and checking the box next to "Adobe Acrobat xx.x Type Library".
- Declare the necessary variables and objects, such as the PDF file and the Excel worksheet.
- Use the Adobe Acrobat library to open the PDF file and extract the data.
- Use VBA to create a new Excel worksheet and paste the extracted data into the worksheet.
- Use VBA to format the data and make any necessary adjustments.
- Save the Excel worksheet and close the PDF file.
Here is an example of VBA code that can be used to convert a PDF file to an Excel worksheet:
Sub ConvertPDFtoExcel()
' Declare the necessary variables and objects
Dim pdfFile As String
Dim excelWorksheet As Worksheet
Dim acrobatApp As Object
Dim acrobatDoc As Object
' Set the PDF file and Excel worksheet
pdfFile = "C:\Path\To\PDF\File.pdf"
Set excelWorksheet = ThisWorkbook.Worksheets("Sheet1")
' Create a new instance of Adobe Acrobat
Set acrobatApp = CreateObject("AcroExch.App")
Set acrobatDoc = CreateObject("AcroExch.PDDoc")
' Open the PDF file
acrobatDoc.Open pdfFile
' Extract the data from the PDF file
Dim pageNumber As Integer
For pageNumber = 1 To acrobatDoc.GetNumPages
Dim page As Object
Set page = acrobatDoc.AcquirePage(pageNumber)
Dim text As String
text = page.GetText
' Paste the extracted data into the Excel worksheet
excelWorksheet.Range("A1").Offset(pageNumber - 1, 0).Value = text
Next pageNumber
' Close the PDF file and Adobe Acrobat
acrobatDoc.Close
acrobatApp.Exit
' Save the Excel worksheet
ThisWorkbook.Save
End Sub
This code creates a new instance of Adobe Acrobat, opens the specified PDF file, extracts the data from each page, and pastes the extracted data into the specified Excel worksheet.
Common Issues and Troubleshooting
When using VBA to convert PDF files to Excel, there are several common issues that may arise. One of the most common issues is that the PDF file may not be properly formatted, making it difficult for VBA to extract the data.To troubleshoot this issue, users can try adjusting the formatting of the PDF file or using a different method to extract the data. Additionally, users can try using a different library or object to interact with Adobe Acrobat, such as the Acrobat SDK.
Another common issue is that the VBA code may not be properly configured, resulting in errors or unexpected behavior. To troubleshoot this issue, users can try debugging the code, checking for syntax errors, and ensuring that all variables and objects are properly declared.
Best Practices for Converting PDF to Excel using VBA
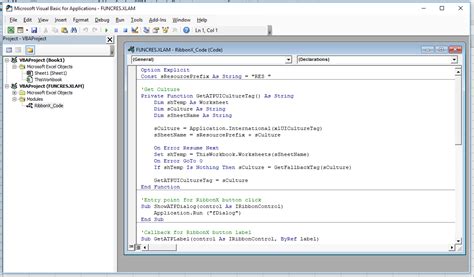
Here are some best practices to keep in mind when using VBA to convert PDF files to Excel:
- Use the Adobe Acrobat library to interact with Adobe Acrobat and automate the conversion process.
- Declare all variables and objects properly to avoid errors and unexpected behavior.
- Use error handling to catch and handle any errors that may arise during the conversion process.
- Test the VBA code thoroughly to ensure that it is working properly and producing the desired results.
- Use comments and documentation to explain the code and make it easier to understand and maintain.
By following these best practices, users can ensure that their VBA code is efficient, effective, and easy to maintain.
Gallery of VBA PDF to Excel Examples
VBA PDF to Excel Image Gallery
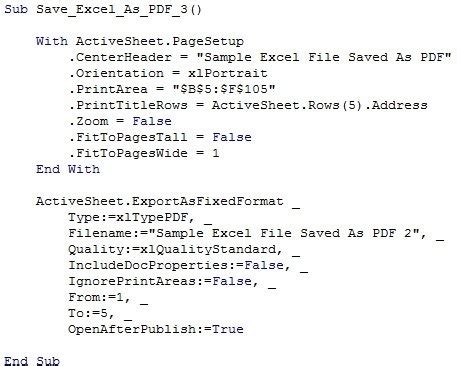
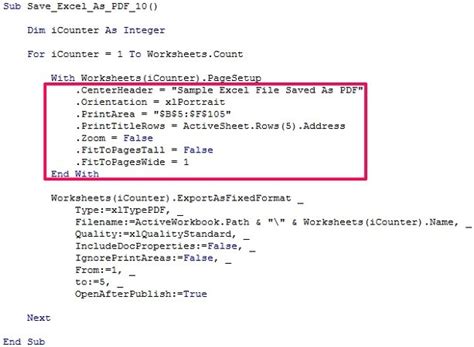
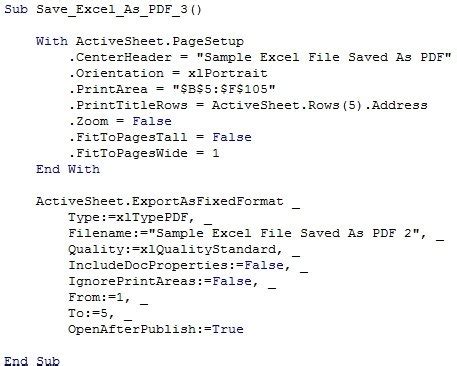
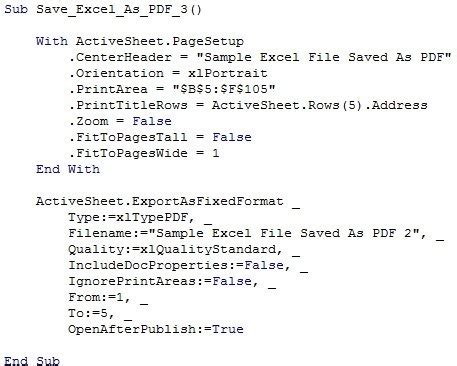
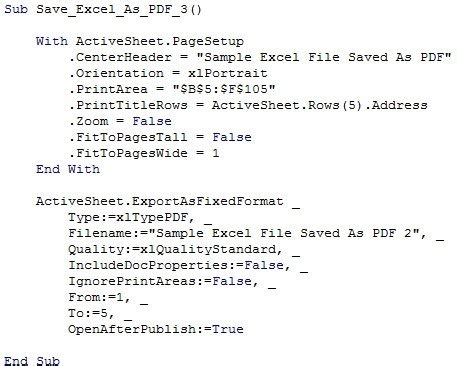
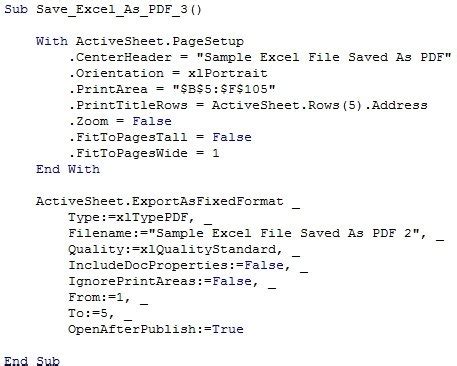
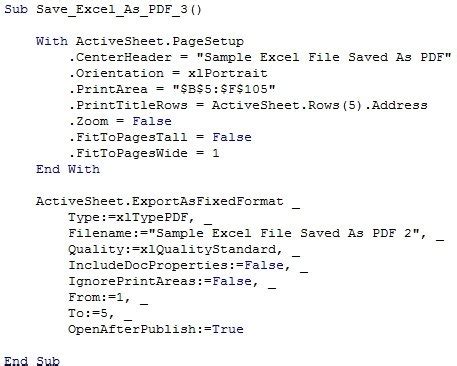
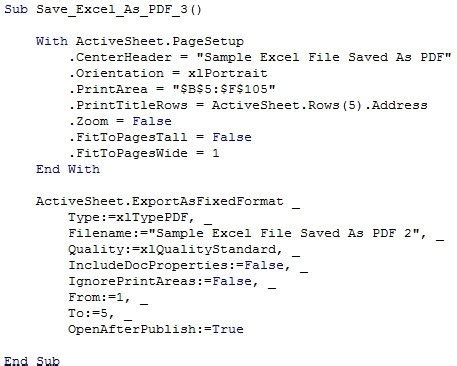
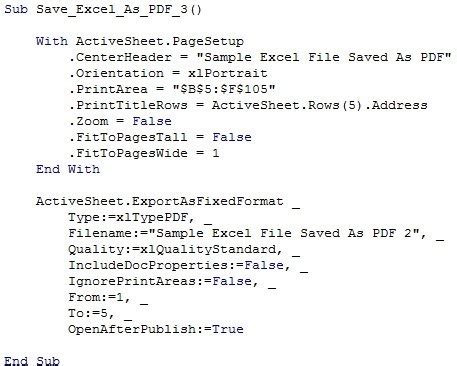
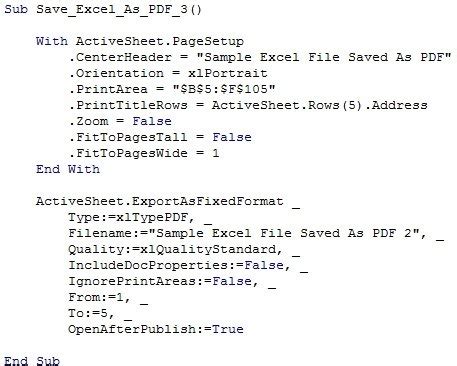
What is VBA and how is it used to convert PDF to Excel?
+VBA is a programming language developed by Microsoft that is used to create and automate tasks in Microsoft Office applications, including Excel. It can be used to convert PDF files to Excel by automating the process of extracting data from the PDF file and pasting it into an Excel worksheet.
What are the benefits of using VBA to convert PDF to Excel?
+The benefits of using VBA to convert PDF to Excel include speed, flexibility, and accuracy. VBA can automate the process of converting PDF files to Excel, making it faster and more efficient. It can also be used to create custom tools and interfaces, making it easier to work with PDF files and extract the data needed.
How do I troubleshoot common issues when using VBA to convert PDF to Excel?
+To troubleshoot common issues when using VBA to convert PDF to Excel, users can try adjusting the formatting of the PDF file, using a different method to extract the data, or debugging the VBA code. Additionally, users can try using a different library or object to interact with Adobe Acrobat, such as the Acrobat SDK.
In conclusion, converting PDF files to Excel spreadsheets is a valuable skill that can save time and reduce the risk of errors. VBA is a powerful tool that can be used to automate this process, making it faster and more efficient. By following the steps and best practices outlined in this article, users can create their own VBA scripts to convert PDF files to Excel and take advantage of the many benefits that VBA has to offer. We invite you to comment, share this article, or take specific actions to learn more about VBA and its applications in converting PDF files to Excel.