Intro
Fix range errors with 5 expert solutions, troubleshooting tips, and error correction methods to resolve #RangeError issues in coding, programming, and data analysis, ensuring accurate calculations and reliable results.
The range error is a common issue that can occur in various programming languages, including JavaScript, Python, and others. It typically happens when a value is outside the expected or allowed range for a specific operation or function. This error can be frustrating, but fortunately, there are several ways to fix it. In this article, we will explore five ways to fix range errors, along with examples and explanations to help you understand the concepts better.
When working with programming languages, it's essential to understand the data types and their limitations. For instance, in JavaScript, the RangeError
object is used to represent an error when a value is not within the expected range. Similarly, in Python, the ValueError
exception is raised when a function or operation receives an argument with an incorrect value. By understanding these concepts, you can write more robust and error-free code.
To fix range errors, you need to identify the source of the problem and then apply the appropriate solution. This might involve validating user input, using try-catch blocks, or modifying your code to handle unexpected values. In the following sections, we will delve into these solutions and provide examples to illustrate each point.
Understanding Range Errors
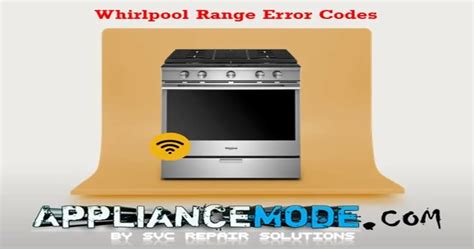
Range errors can occur in various situations, such as when working with arrays, strings, or numbers. For example, if you try to access an array index that is out of bounds, you will get a range error. Similarly, if you pass a value to a function that is outside the expected range, you will encounter a range error. To fix these errors, you need to understand the underlying causes and then apply the appropriate solutions.
Validating User Input
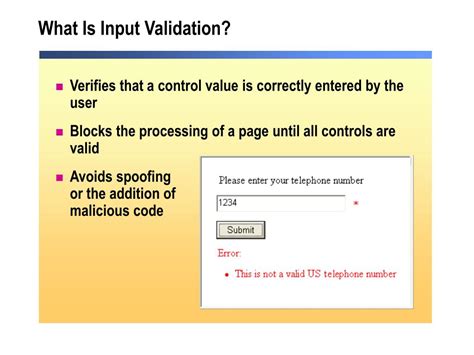
One of the primary causes of range errors is invalid user input. When users enter values that are outside the expected range, your code may throw a range error. To prevent this, you can validate user input using various techniques, such as checking the data type, range, and format. For example, you can use JavaScript's typeof
operator to check the data type of a variable, or use regular expressions to validate the format of a string.
Here are some examples of validating user input:
- Check if a value is a number using
typeof
operator:if (typeof value === 'number')
- Validate the range of a value using conditional statements:
if (value >= 0 && value <= 100)
- Use regular expressions to validate the format of a string:
if (/^[a-zA-Z]+$/.test(input))
Using Try-Catch Blocks
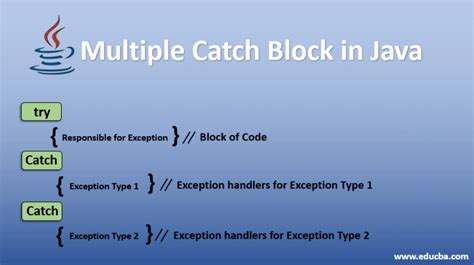
Try-catch blocks are another effective way to handle range errors. By wrapping your code in a try-catch block, you can catch any exceptions that occur and handle them accordingly. This approach allows you to provide a more robust and user-friendly experience, as you can display error messages or take alternative actions when an exception occurs.
Here is an example of using try-catch blocks:
try {
// Code that may throw a range error
var result = calculateValue(input);
} catch (error) {
// Handle the error
if (error instanceof RangeError) {
console.log('Range error occurred');
} else {
console.log('Unknown error occurred');
}
}
Modifying Code to Handle Unexpected Values

In some cases, you may need to modify your code to handle unexpected values. This can involve adding checks for invalid values, using default values, or providing alternative actions. By handling unexpected values, you can prevent range errors and ensure that your code runs smoothly.
Here are some examples of modifying code to handle unexpected values:
- Use default values for missing or invalid input:
var value = input || 0;
- Provide alternative actions for invalid input:
if (input < 0) { return -input; }
- Use checks for invalid values:
if (isNaN(input)) { throw new Error('Invalid input'); }
Testing and Debugging
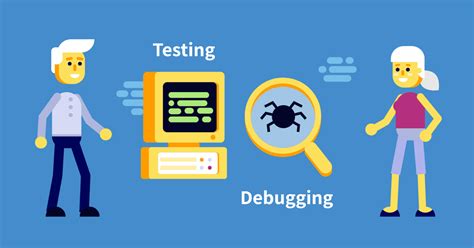
Testing and debugging are crucial steps in identifying and fixing range errors. By thoroughly testing your code, you can identify potential issues and fix them before they cause problems. Additionally, debugging tools can help you diagnose and fix range errors by providing detailed information about the error.
Here are some tips for testing and debugging:
- Use testing frameworks to write unit tests for your code
- Use debugging tools to diagnose and fix errors
- Test your code with different input values and edge cases
Best Practices for Preventing Range Errors
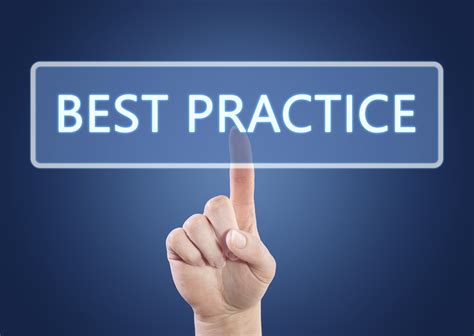
To prevent range errors, it's essential to follow best practices when writing code. This includes validating user input, using try-catch blocks, and modifying code to handle unexpected values. Additionally, testing and debugging can help you identify and fix potential issues before they cause problems.
Here are some best practices for preventing range errors:
- Validate user input thoroughly
- Use try-catch blocks to handle exceptions
- Modify code to handle unexpected values
- Test and debug code thoroughly
Range Error Image Gallery

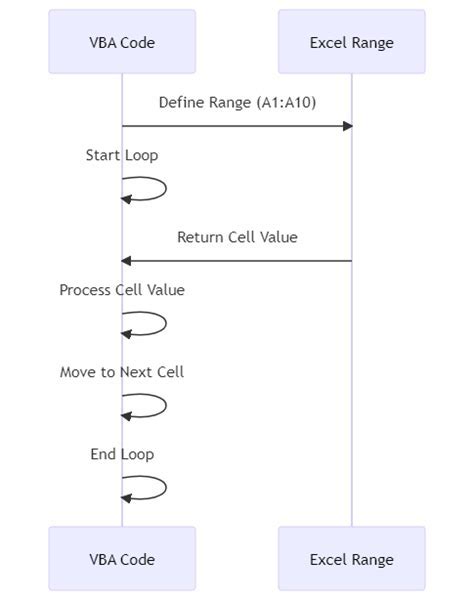
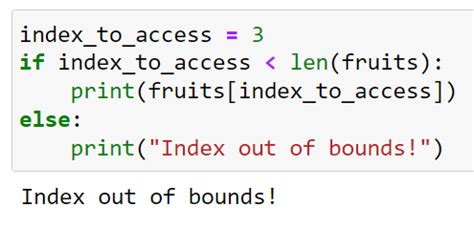
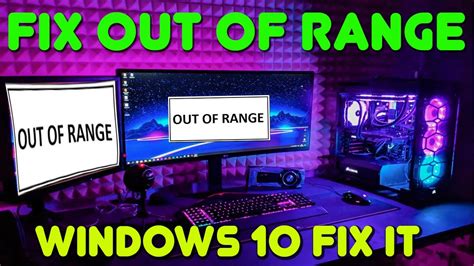

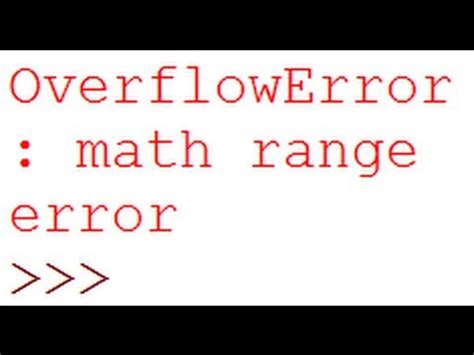
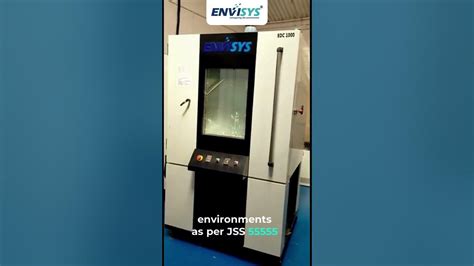
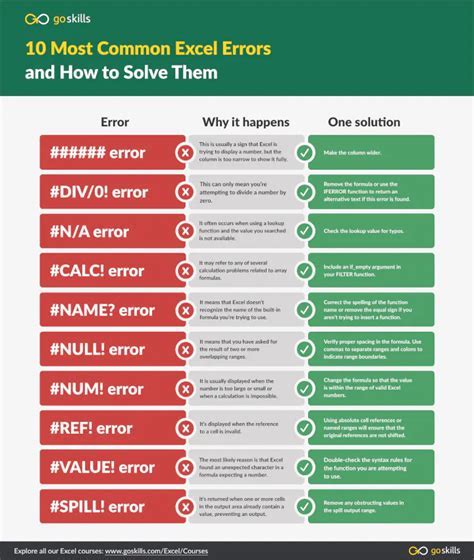
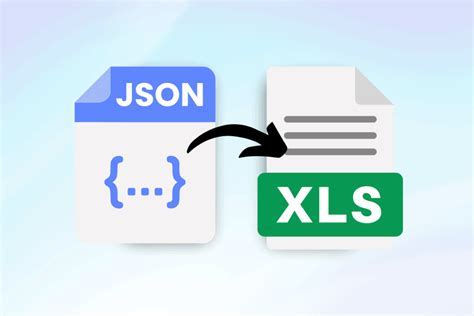
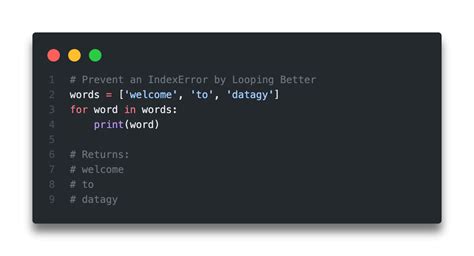
What is a range error?
+A range error is an error that occurs when a value is outside the expected or allowed range for a specific operation or function.
How can I fix a range error?
+To fix a range error, you can validate user input, use try-catch blocks, modify code to handle unexpected values, and test and debug your code thoroughly.
What are some common causes of range errors?
+Common causes of range errors include invalid user input, unexpected values, and code that is not designed to handle edge cases.
How can I prevent range errors?
+To prevent range errors, you can follow best practices such as validating user input, using try-catch blocks, modifying code to handle unexpected values, and testing and debugging your code thoroughly.
What are some tools and techniques for debugging range errors?
+Some tools and techniques for debugging range errors include using debugging tools, testing frameworks, and logging mechanisms to identify and diagnose the error.
In conclusion, range errors can be frustrating, but by understanding the causes and applying the right solutions, you can fix them and ensure that your code runs smoothly. By following best practices, validating user input, using try-catch blocks, modifying code to handle unexpected values, and testing and debugging thoroughly, you can prevent range errors and provide a more robust and user-friendly experience. If you have any questions or need further assistance, please don't hesitate to comment or share this article with others.