Intro
The importance of filtering data in Excel cannot be overstated, especially when dealing with large datasets. Filtering helps in narrowing down the data to only the relevant information, making analysis and decision-making more efficient. Excel's built-in filter feature is powerful, but for those who need more advanced control, Excel VBA (Visual Basic for Applications) offers a robust solution. VBA allows users to automate filtering tasks, making repetitive processes easier and faster. For instance, when working with daily reports or frequent data updates, having a script that can automatically filter data based on specific criteria can save a significant amount of time.
In many cases, the ability to clear filters becomes as crucial as applying them. Filters are meant to be temporary views of the data, and once the analysis is done, it's often necessary to remove these filters to get back to the full dataset. Excel VBA provides several ways to achieve this, ranging from simple commands to more complex scripts that can handle multiple worksheets or workbooks. Understanding how to clear filters using VBA can enhance productivity and reduce the risk of errors that might occur from manual operations.
Automating tasks such as filtering and clearing filters can significantly improve workflow efficiency. For professionals who work with data regularly, learning VBA can be a valuable skill. It not only helps in performing repetitive tasks quickly but also enables the creation of custom tools and interfaces tailored to specific needs. Moreover, when it comes to sharing workbooks, having automated processes can make the data analysis process smoother for colleagues or clients who may not be familiar with the dataset or the required analysis steps.
Understanding Excel VBA Basics
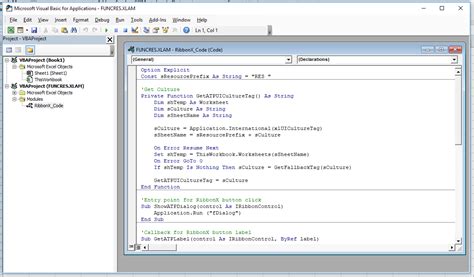
Before diving into the methods of clearing filters, it's essential to have a basic understanding of Excel VBA. VBA is accessed through the Developer tab in Excel, which might need to be enabled if it's not already visible. Once in the VBA editor, users can write, edit, and run macros. A macro is essentially a series of instructions that Excel executes. For clearing filters, these instructions can be as simple as selecting a range and applying a command to clear filters.
Method 1: Clearing Filters from a Specific Range
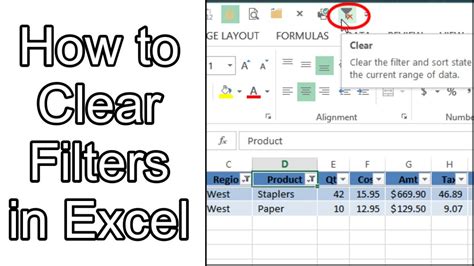
One of the straightforward methods to clear filters is by specifying the range from which the filters should be removed. This can be particularly useful when dealing with datasets that are structured in a specific way, such as having headers in the first row. The VBA code for this would involve selecting the range and then using the AutoFilter
method with the Clear
argument.
Sub ClearFiltersFromRange()
Range("A1:E1").AutoFilter 'Assuming filters are applied to columns A through E
Range("A1:E1").AutoFilter 'This line clears the filters
End Sub
However, the above code might not work as expected because simply calling AutoFilter
again does not clear the filters. Instead, you should use:
Sub ClearFiltersFromRange()
Range("A1:E1").AutoFilter 'Apply filter
Range("A1:E1").AutoFilter 'This does not clear filters, instead use:
ActiveSheet.AutoFilterMode = False 'This line clears all filters from the sheet
End Sub
Method 2: Clearing All Filters from the Active Sheet
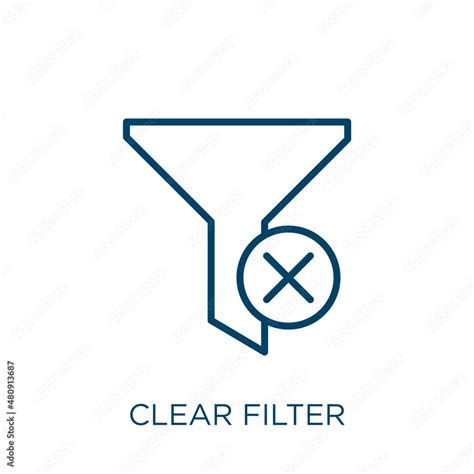
Sometimes, it's more efficient to clear all filters from the active sheet rather than specifying a range. This approach is useful when working with multiple datasets on the same sheet, and filters have been applied to different ranges. The VBA code to achieve this involves setting the AutoFilterMode
of the active sheet to False
.
Sub ClearAllFiltersFromActiveSheet()
ActiveSheet.AutoFilterMode = False
End Sub
This method is straightforward and quickly removes all filters, allowing you to start fresh with your data analysis.
Method 3: Clearing Filters Based on Criteria
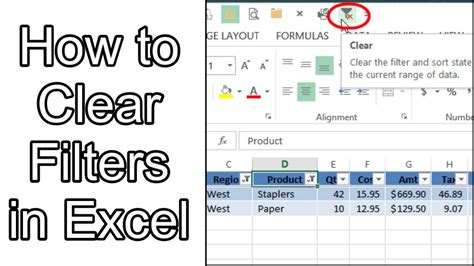
In some scenarios, you might want to clear filters based on specific criteria, such as clearing filters from columns that have a certain header. This requires a bit more complex VBA code that loops through the columns, checks the header, and then clears the filter if the condition is met.
Sub ClearFiltersBasedOnCriteria()
Dim header As Range
For Each header In Range("A1:E1").Cells 'Assuming headers are in the first row
If header.Value = "SpecificHeader" Then 'Replace "SpecificHeader" with your criteria
header.AutoFilter
header.AutoFilter 'This does not actually clear, use the next line instead
'ActiveSheet.AutoFilterMode = False 'Uncomment to clear all filters
'Or, apply filter again with no criteria to "clear" it
End If
Next header
End Sub
However, the above code has its limitations, as simply calling AutoFilter
again does not remove the filter. A more accurate approach would involve checking if a filter is applied and then clearing it, which can be complex and is generally more straightforward to clear all filters at once.
Method 4: Using a Button to Clear Filters
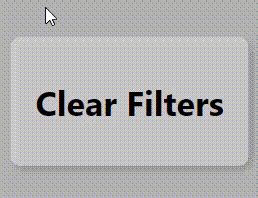
For users who frequently need to clear filters, assigning a macro to a button can be very convenient. This method involves creating a button in Excel, assigning the macro to clear filters to this button, and then clicking the button whenever filters need to be cleared.
- Go to the Developer tab and click on the "Insert" button in the Controls group.
- Select "Command Button" under the ActiveX Controls group.
- Draw the button on your sheet.
- Right-click the button and select "View Code."
- In the VBA editor, paste the code to clear filters, such as
ActiveSheet.AutoFilterMode = False
. - Close the VBA editor, and the button is now ready to use.
Method 5: Clearing Filters Across Multiple Worksheets
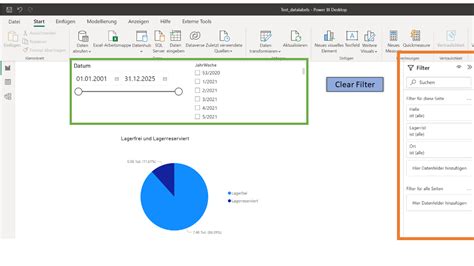
When working with workbooks that contain multiple worksheets, and filters have been applied across these sheets, it can be tedious to manually clear filters from each sheet. VBA provides a solution to clear filters across all worksheets in a workbook with a single macro.
Sub ClearFiltersAcrossAllSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.AutoFilterMode = False
Next ws
End Sub
This macro loops through each worksheet in the active workbook and clears all filters.
Gallery of Excel VBA Filter Examples
Excel VBA Filter Gallery
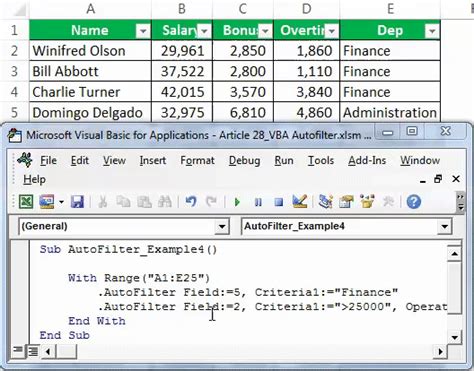
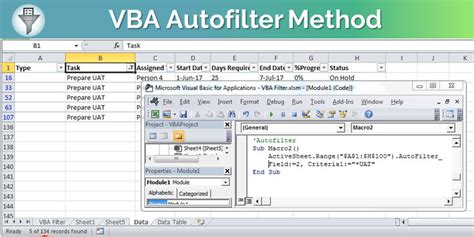
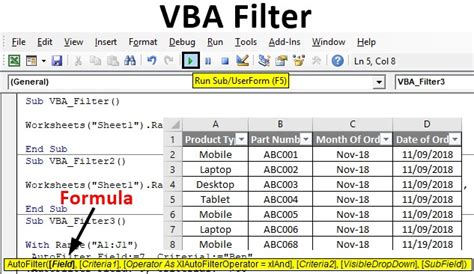
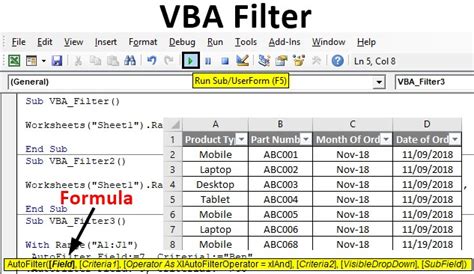
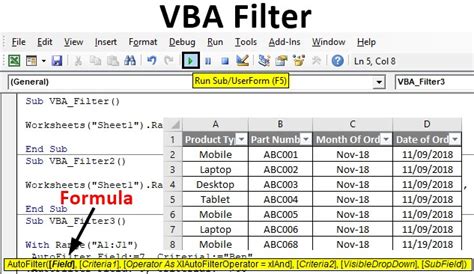
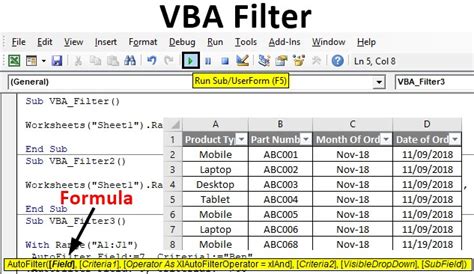
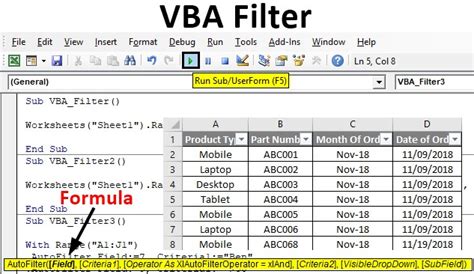
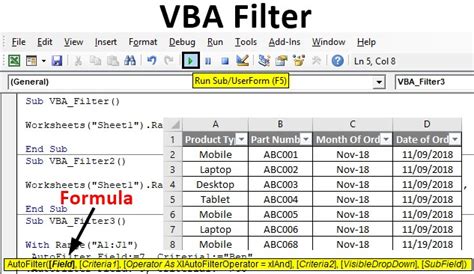
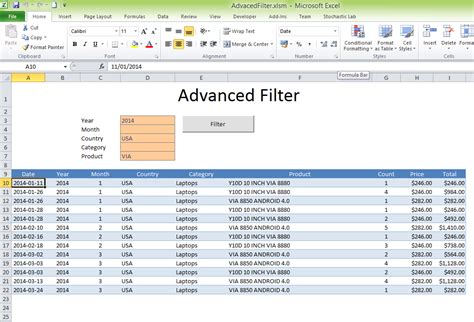
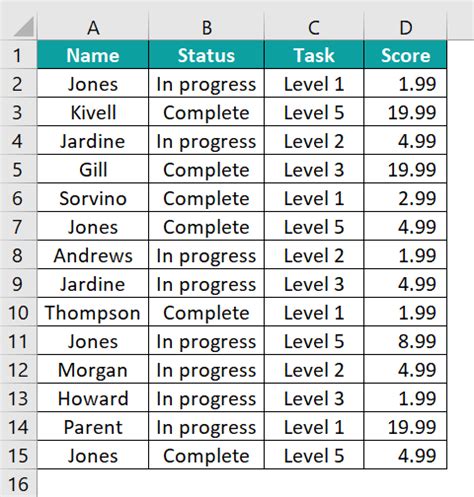
How do I enable the Developer tab in Excel?
+To enable the Developer tab, go to File > Options > Customize Ribbon, check the Developer checkbox, and click OK.
What is the difference between clearing filters and removing filters?
+Clearing filters removes the filtered view but keeps the filter criteria. Removing filters completely deletes the filter, including its criteria.
Can I use VBA to filter data based on multiple criteria?
+Yes, VBA allows you to filter data based on multiple criteria using the `AutoFilter` method with the `Criteria1` and `Operator` arguments for multiple conditions.
In conclusion, mastering the art of clearing filters in Excel using VBA can significantly enhance your productivity and data analysis capabilities. Whether you're working with simple datasets or complex, multi-sheet workbooks, understanding how to automate the process of clearing filters can save time and reduce errors. By applying the methods outlined in this article, you can take your Excel skills to the next level and make data analysis more efficient. Feel free to share your experiences or ask questions about using VBA for filtering and clearing filters in the comments below.