Intro
Discover 5 ways to remove left characters, including trimming whitespace, using string functions, and regex techniques to optimize text data, improve formatting, and enhance data cleansing and processing efficiency.
The ability to remove left characters from a string is a fundamental operation in programming and text processing. It can be useful in a variety of scenarios, such as data cleaning, string manipulation, and formatting. Whether you're working with strings in a programming language, using a text editor, or manipulating data in a spreadsheet, understanding how to remove characters from the left side of a string can be quite beneficial. Here, we'll explore five different ways to achieve this, covering various tools and programming languages to cater to different needs and environments.
Removing left characters can be necessary for several reasons, such as correcting improperly formatted data, removing unwanted prefixes, or simply reorganizing text to fit specific requirements. The method you choose can depend on the context in which you're working, the tools available to you, and your personal preference or familiarity with certain programming languages or software.
Understanding the Need to Remove Left Characters
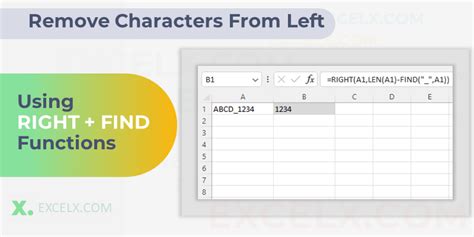
Before diving into the methods, it's essential to understand the scenarios where removing left characters is necessary. In data processing, for instance, you might encounter strings with leading spaces or characters that need to be removed for the data to be correctly analyzed or displayed. Similarly, in web development, removing left characters can be part of validating user input or formatting output to match specific design requirements.
Method 1: Using Programming Languages

Many programming languages offer built-in functions or methods to manipulate strings, including removing characters from the left. For example, in Python, you can use the lstrip()
method to remove leading characters from a string. If you want to remove a specific number of characters from the left, you can use slicing, such as string[5:]
to remove the first five characters.
Example in Python
# Remove all leading spaces
string = " Hello World"
print(string.lstrip()) # Output: "Hello World"
# Remove the first 5 characters
string = "Hello World"
print(string[5:]) # Output: " World"
Method 2: Using Text Editors
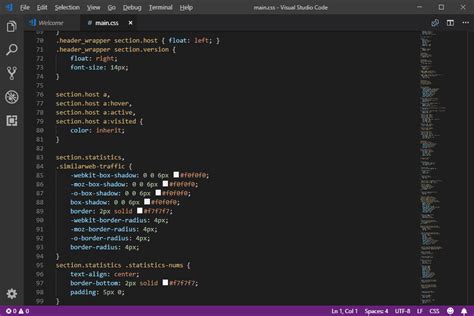
Text editors like Notepad++, Sublime Text, or Visual Studio Code offer powerful features for text manipulation, including regular expressions that can be used to remove characters from the left side of strings. For instance, using a regular expression to match and remove leading characters can be an efficient way to process large amounts of text.
Using Regular Expressions
Regular expressions can be used in many text editors and programming languages to find and replace patterns in text. To remove characters from the left, you can use a pattern that matches one or more characters from the start of the string.
Method 3: Using Spreadsheet Software
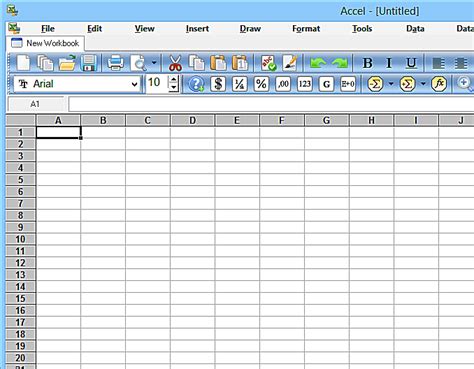
In spreadsheet software like Microsoft Excel or Google Sheets, you can use formulas to manipulate text. The RIGHT
function, combined with the LEN
function, can be used to remove characters from the left side of a string. For example, =RIGHT(A1,LEN(A1)-5)
removes the first 5 characters from the string in cell A1.
Example in Excel
=RIGHT(A1,LEN(A1)-5)
Method 4: Using Online Tools
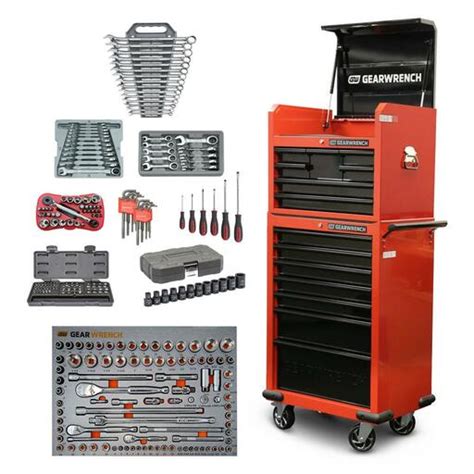
There are many online tools and websites that offer text manipulation services, including removing characters from strings. These tools can be handy for quick operations without the need to open a text editor or programming environment.
Method 5: Using Command Line Tools

For those comfortable with the command line, tools like sed
or awk
can be used to remove characters from the left side of strings. These tools are particularly useful for batch processing large numbers of files or streams of text.
Example Using Sed
echo "Hello World" | sed 's/^....//'
This command removes the first four characters from the string "Hello World", resulting in "o World".
Gallery of Remove Left Characters
Remove Left Characters Image Gallery
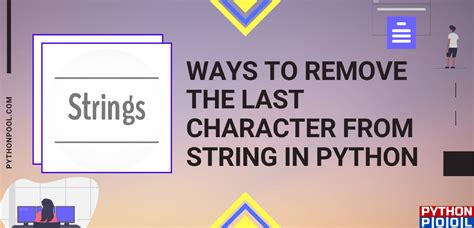
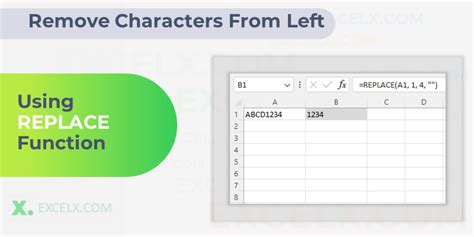
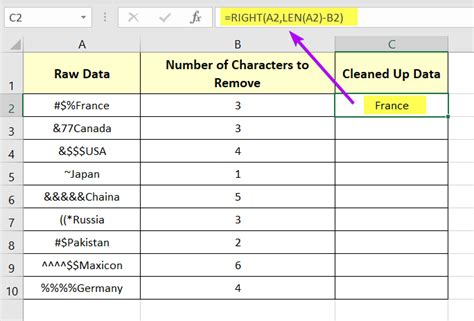
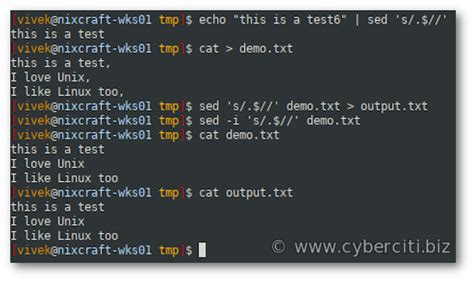
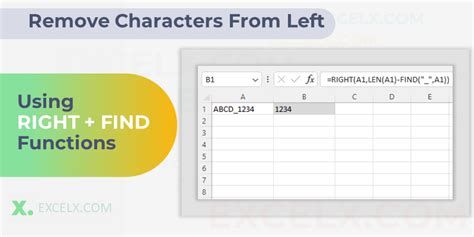
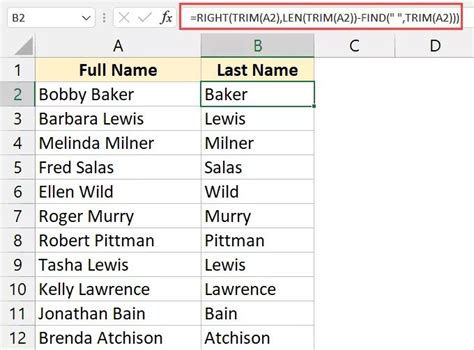
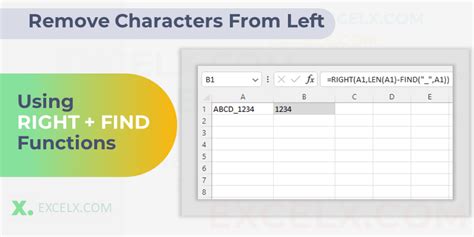
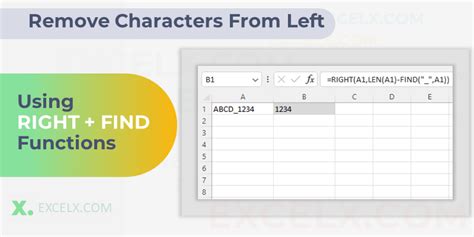
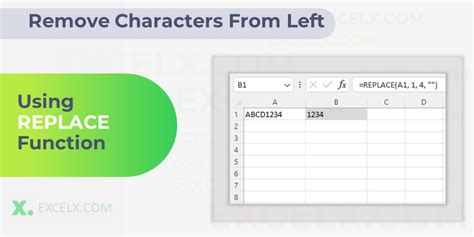
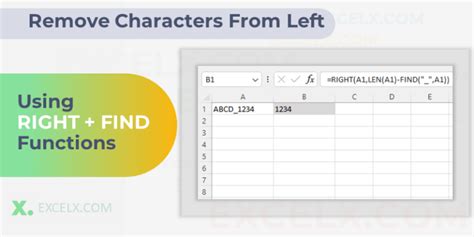
Frequently Asked Questions
What is the purpose of removing left characters?
+The purpose of removing left characters can vary, including data cleaning, string manipulation, and formatting. It's often necessary to remove unwanted prefixes or correct improperly formatted data.
How do I remove left characters in Python?
+In Python, you can use the `lstrip()` method to remove leading characters from a string. For removing a specific number of characters, slicing can be used, such as `string[5:]` to remove the first five characters.
Can I use online tools to remove left characters?
+Yes, there are many online tools available that offer text manipulation services, including removing characters from strings. These can be useful for quick operations without needing to open a text editor or programming environment.
In conclusion, removing left characters from strings is a versatile operation that can be achieved through various methods and tools, ranging from programming languages and text editors to spreadsheet software and online tools. Each method has its own advantages and is suited to different scenarios, making it important to choose the one that best fits your specific needs and environment. Whether you're a developer, data analyst, or simply someone looking to manipulate text, understanding how to remove characters from the left side of a string can be a valuable skill. We hope this article has provided you with the insights and techniques necessary to efficiently remove left characters and enhance your text manipulation capabilities. Feel free to share your experiences or ask further questions in the comments below.