Intro
Master VBA Excel sheet name manipulation with our expert guide, covering sheet naming conventions, renaming sheets using VBA code, and dynamically updating sheet names with macros and Excel formulas.
Working with Excel sheet names in VBA can be a crucial aspect of automating tasks and managing workbooks efficiently. Excel sheet names are used to identify and reference specific sheets within a workbook, making it easier to perform operations on those sheets using VBA. In this article, we will delve into the world of VBA Excel sheet names, exploring how to work with them, rename sheets, and use them in various VBA operations.
Understanding Excel Sheet Names in VBA
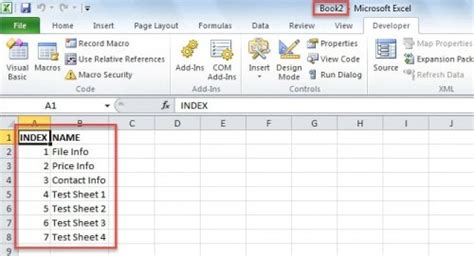
Excel sheet names are the names given to the sheets in an Excel workbook. These names are used to reference the sheets in VBA code, allowing you to perform various operations such as copying data, formatting cells, and more. By default, Excel assigns generic names to new sheets, such as "Sheet1", "Sheet2", etc. However, you can rename these sheets to make them more descriptive and easier to work with in your VBA code.
Rename Excel Sheets Using VBA
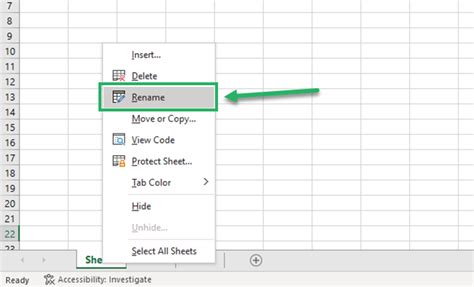
Renaming Excel sheets using VBA is a straightforward process. You can use the Name
property of the Worksheet
object to change the name of a sheet. For example:
Sub RenameSheet()
ThisWorkbook.Sheets("Sheet1").Name = "MyNewSheet"
End Sub
This code renames the sheet named "Sheet1" to "MyNewSheet".
Best Practices for Renaming Excel Sheets
When renaming Excel sheets, it's essential to follow some best practices to avoid errors and ensure that your VBA code works correctly. Here are some tips: * Avoid using special characters, such as!, @, #, $, etc., in your sheet names. * Keep your sheet names concise and descriptive. * Use a consistent naming convention throughout your workbook.Working with Excel Sheet Names in VBA
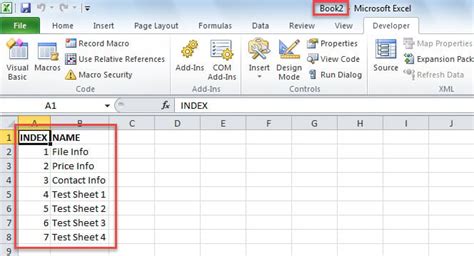
Once you have renamed your Excel sheets, you can start working with them in VBA. Here are some examples of how you can use Excel sheet names in your VBA code:
- Referencing a specific sheet:
ThisWorkbook.Sheets("MyNewSheet").Range("A1").Value = "Hello World"
- Looping through all sheets:
For Each ws In ThisWorkbook.Sheets: ws.Range("A1").Value = "Hello World": Next ws
- Checking if a sheet exists:
If ThisWorkbook.Sheets("MyNewSheet") Is Nothing Then MsgBox "Sheet not found"
Common Errors When Working with Excel Sheet Names
When working with Excel sheet names in VBA, you may encounter some common errors. Here are some tips to help you troubleshoot: * Make sure the sheet name is spelled correctly. * Check that the sheet name is not duplicated. * Use the `On Error Resume Next` statement to handle errors when working with sheet names.Using Excel Sheet Names in VBA Loops
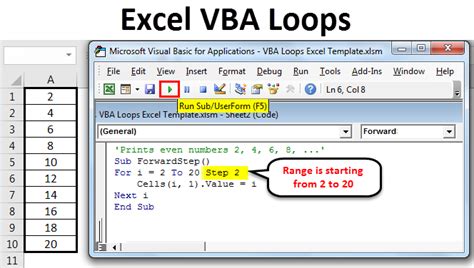
VBA loops are a powerful tool for automating tasks in Excel. You can use Excel sheet names in VBA loops to perform operations on multiple sheets. Here is an example:
Sub LoopThroughSheets()
For Each ws In ThisWorkbook.Sheets
ws.Range("A1").Value = "Hello World"
Next ws
End Sub
This code loops through all sheets in the workbook and sets the value of cell A1 to "Hello World".
Benefits of Using Excel Sheet Names in VBA Loops
Using Excel sheet names in VBA loops offers several benefits, including: * Increased efficiency: You can perform operations on multiple sheets with a single loop. * Improved accuracy: You can avoid errors by using the correct sheet names. * Flexibility: You can easily add or remove sheets from the loop as needed.Gallery of Excel Sheet Names
Excel Sheet Names Gallery
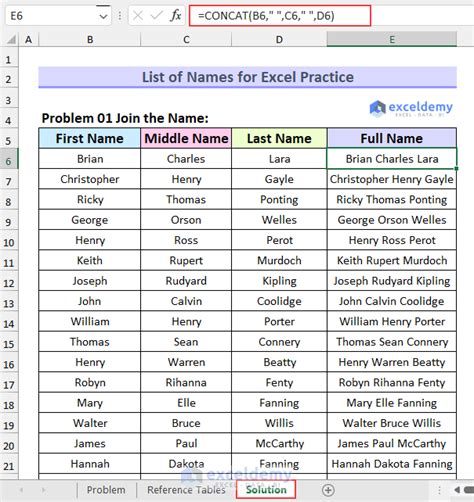
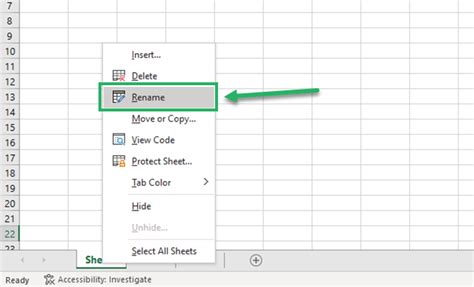
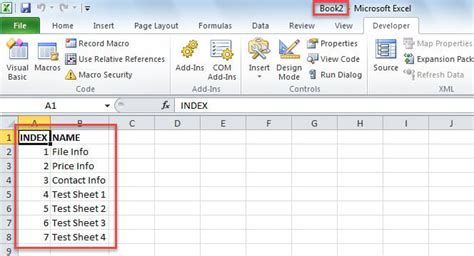
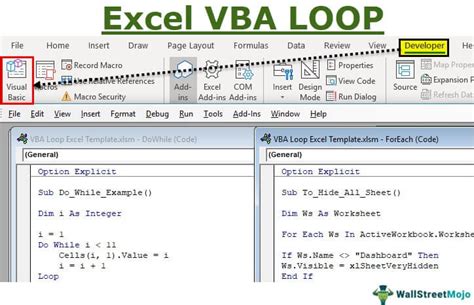
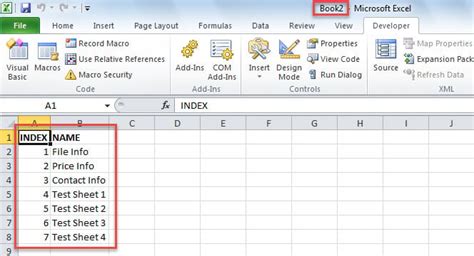
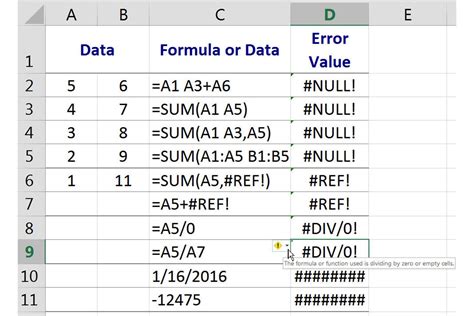
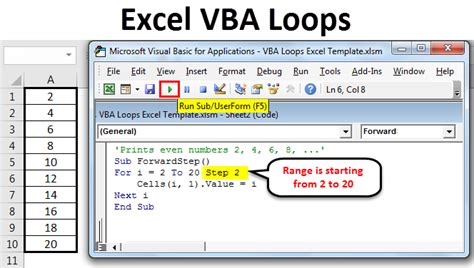
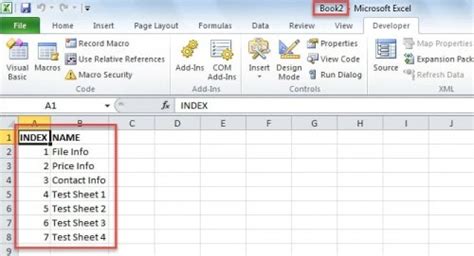
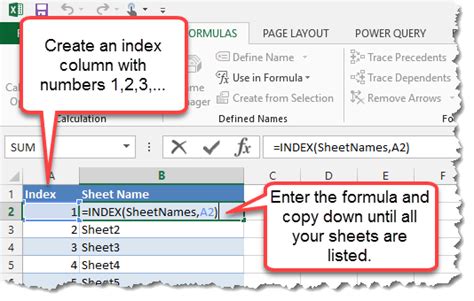
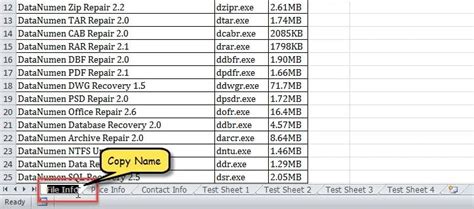
Frequently Asked Questions
How do I rename an Excel sheet using VBA?
+You can rename an Excel sheet using VBA by using the `Name` property of the `Worksheet` object. For example: `ThisWorkbook.Sheets("Sheet1").Name = "MyNewSheet"`
How do I loop through all sheets in an Excel workbook using VBA?
+You can loop through all sheets in an Excel workbook using VBA by using a `For Each` loop. For example: `For Each ws In ThisWorkbook.Sheets: ws.Range("A1").Value = "Hello World": Next ws`
What are some best practices for working with Excel sheet names in VBA?
+Some best practices for working with Excel sheet names in VBA include avoiding special characters, keeping sheet names concise and descriptive, and using a consistent naming convention throughout your workbook.
We hope this article has provided you with a comprehensive understanding of working with Excel sheet names in VBA. Whether you're a beginner or an experienced VBA user, mastering Excel sheet names is essential for automating tasks and managing workbooks efficiently. If you have any questions or need further assistance, please don't hesitate to comment below. Share this article with your friends and colleagues who may benefit from learning about Excel sheet names in VBA.