Intro
Creating a new sheet in Excel using VBA (Visual Basic for Applications) is a straightforward process that can be accomplished with a few lines of code. This can be particularly useful when automating tasks or creating templates where the number of sheets needed can vary. Below, we'll explore how to create a new sheet, how to name it, and how to position it within your workbook.
Why Use VBA to Create a New Sheet?
Before diving into the code, it's worth considering why you might want to use VBA for this task. While Excel's UI allows you to easily insert new sheets, VBA provides the power to automate this process. This can be useful in a variety of scenarios, such as:
- Automatically generating reports that require separate sheets for different data sets.
- Creating templates where the user needs to be able to dynamically add new sheets based on certain conditions.
- Automating the setup of a new project workbook that requires a specific structure.
Basic Code to Create a New Sheet
To create a new sheet, you can use the Worksheets.Add
method. Here's a basic example:
Sub CreateNewSheet()
Worksheets.Add
End Sub
This code will add a new sheet to your workbook. However, it doesn't specify where the new sheet should be inserted or what it should be named.
Specifying the Position of the New Sheet
If you want to control where the new sheet is inserted, you can use the After
or Before
argument with the Worksheets.Add
method. For example, to insert a new sheet after the last existing sheet:
Sub CreateNewSheetAfterLast()
Worksheets.Add After:=Worksheets(Worksheets.Count)
End Sub
Or, to insert it before the first sheet:
Sub CreateNewSheetBeforeFirst()
Worksheets.Add Before:=Worksheets(1)
End Sub
Naming the New Sheet
Immediately after creating a new sheet, you can name it using the Name
property. Here's how you can modify the previous example to also name the sheet:
Sub CreateAndNameNewSheet()
Dim newSheet As Worksheet
Set newSheet = Worksheets.Add(After:=Worksheets(Worksheets.Count))
newSheet.Name = "MyNewSheet"
End Sub
Practical Example: Creating Multiple Sheets with a Loop
Sometimes, you might need to create multiple sheets based on certain conditions or inputs. You can use a loop to achieve this. Here's an example that creates sheets named "Day1", "Day2", etc., up to "Day7":
Sub CreateWeeklySheets()
Dim i As Integer
For i = 1 To 7
Dim newSheet As Worksheet
Set newSheet = Worksheets.Add(After:=Worksheets(Worksheets.Count))
newSheet.Name = "Day" & i
Next i
End Sub
Important Considerations
- Sheet Name Limitations: Excel has rules about what characters can and cannot be used in sheet names. For example, you cannot use the characters
/
,\
,?
,*
,[
, or]
. - Duplicate Names: You cannot have two sheets with the same name in a workbook. If you try to name a sheet with a name that already exists, Excel will throw an error.
- Maximum Number of Sheets: While the practical limit is very high, there is a maximum number of sheets you can have in a workbook, which is 255 in older versions of Excel and much higher in newer versions.
Embedding Images
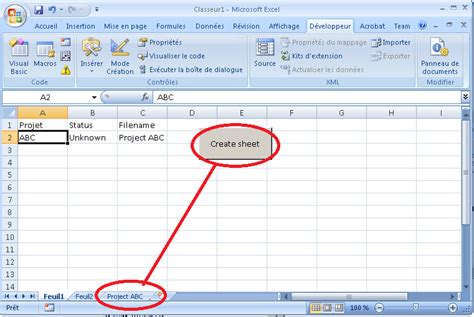
Gallery of Excel VBA Images
Excel VBA Gallery
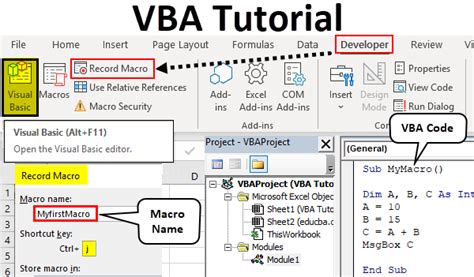
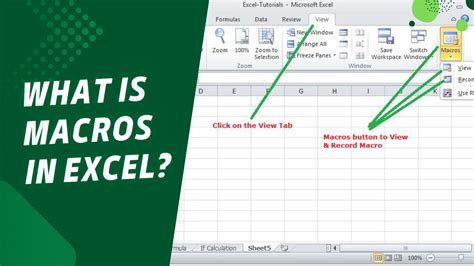
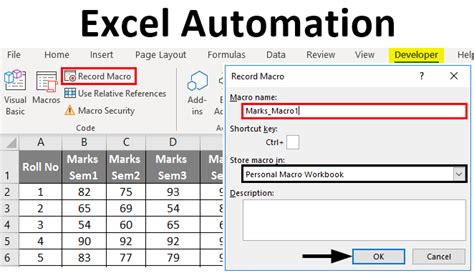
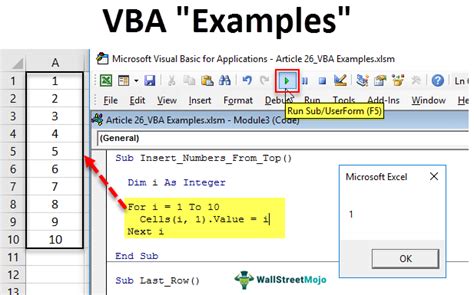
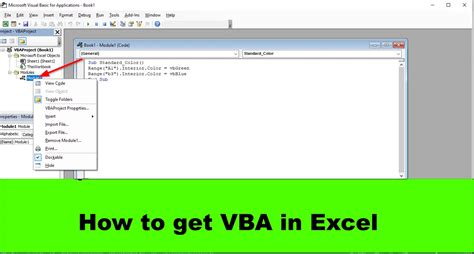
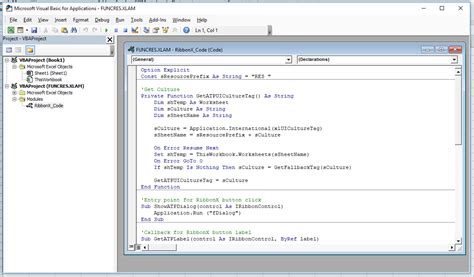
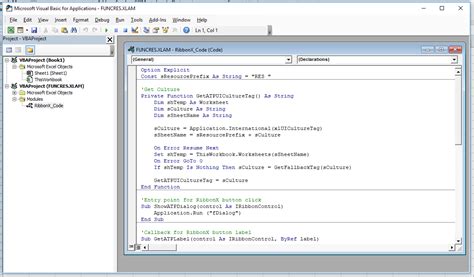
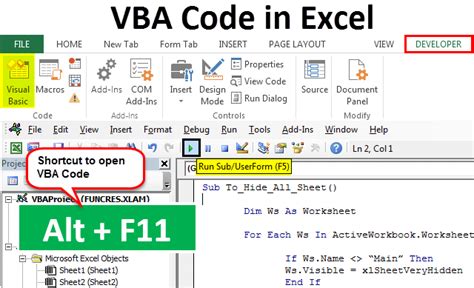
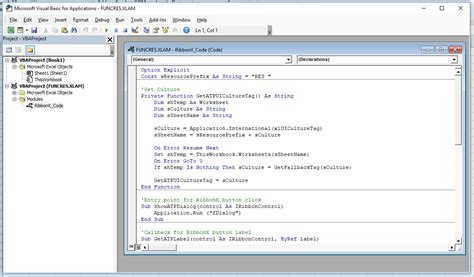
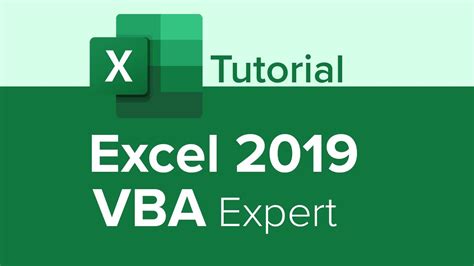
FAQs
What is Excel VBA?
+Excel VBA stands for Visual Basic for Applications, which is a programming language used to create and automate tasks in Excel.
How do I access VBA in Excel?
+You can access VBA in Excel by pressing Alt + F11 or by navigating to the Developer tab and clicking on Visual Basic.
Can I use VBA to automate tasks in other Office applications?
+Yes, VBA can be used to automate tasks in other Office applications such as Word, PowerPoint, and Outlook.
In conclusion, using VBA to create new sheets in Excel provides a powerful tool for automating tasks and customizing your workbooks. Whether you're looking to streamline your workflow, create complex templates, or simply need more control over your spreadsheets, VBA is an excellent skill to develop. Remember to always consider the limitations and best practices when working with VBA to ensure your code is efficient, readable, and maintainable. If you have any questions or need further assistance with creating new sheets or any other VBA-related topic, don't hesitate to reach out.