Intro
Deleting a worksheet in Excel can be a straightforward process, but it can also be automated using Excel VBA (Visual Basic for Applications) for more complex tasks or when dealing with multiple worksheets. Understanding how to delete worksheets using VBA can be particularly useful for managing large workbooks, automating repetitive tasks, or creating custom applications within Excel.
The importance of knowing how to delete worksheets with Excel VBA lies in its ability to streamline workflow, reduce manual errors, and enhance productivity. Whether you're a beginner looking to automate simple tasks or an advanced user seeking to create complex applications, mastering VBA techniques such as worksheet deletion is essential. This article will guide you through the process, providing step-by-step instructions, practical examples, and tips for managing worksheets effectively with Excel VBA.
Introduction to Excel VBA
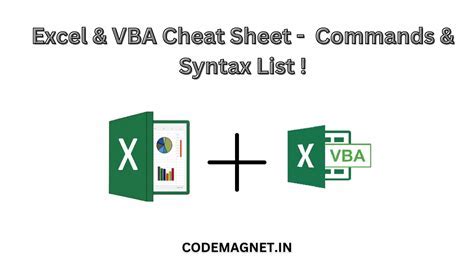
Excel VBA is a powerful tool that allows users to create and automate tasks within Excel. It uses the Visual Basic programming language to create procedures, functions, and applications that can interact with Excel worksheets, making it an indispensable skill for anyone looking to automate tasks or create custom Excel applications.
Why Delete Worksheets with VBA?
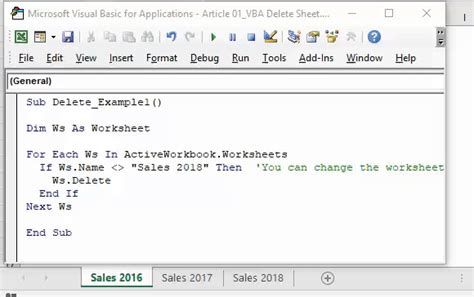
Deleting worksheets using VBA offers several advantages, including the ability to automate the process, apply conditions for deletion (e.g., deleting worksheets based on their names or contents), and integrate worksheet deletion into larger VBA scripts. This can be particularly useful in scenarios where manual deletion would be time-consuming or prone to errors.
Basic Steps to Delete a Worksheet
Before diving into the VBA code, it's essential to understand the basic steps involved in deleting a worksheet manually:
- Select the worksheet you want to delete.
- Right-click on the worksheet tab and choose "Delete Sheet."
- Confirm the deletion in the dialog box that appears.
Deleting Worksheets with Excel VBA
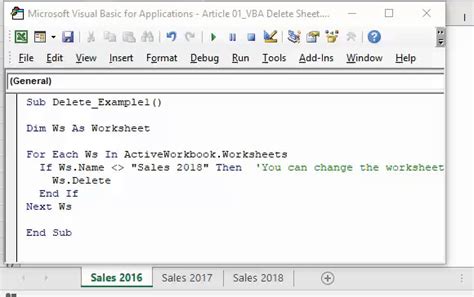
To delete a worksheet using VBA, you can use the following code as a starting point:
Sub DeleteWorksheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1") ' Change "Sheet1" to the name of your worksheet
Application.DisplayAlerts = False ' This line suppresses the confirmation dialog
ws.Delete
Application.DisplayAlerts = True ' This line re-enables alerts
End Sub
This code defines a subroutine named DeleteWorksheet
that deletes a worksheet named "Sheet1" in the active workbook. The Application.DisplayAlerts = False
line is used to suppress the confirmation dialog that Excel typically displays when deleting a worksheet, allowing the deletion to occur silently.
Conditional Deletion of Worksheets
One of the powerful features of VBA is the ability to apply conditions to the deletion process. For example, you might want to delete all worksheets that start with a certain name or contain specific data. Here’s an example of how to delete worksheets based on their names:
Sub DeleteWorksheetsByName()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If ws.Name Like "Temp*" Then ' Deletes worksheets whose names start with "Temp"
Application.DisplayAlerts = False
ws.Delete
Application.DisplayAlerts = True
End If
Next ws
End Sub
This code loops through all worksheets in the workbook and deletes any worksheet whose name starts with "Temp".
Best Practices for Managing Worksheets with VBA
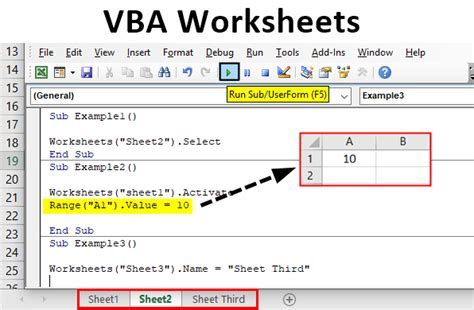
When managing worksheets with VBA, it’s crucial to follow best practices to ensure your code is efficient, readable, and error-free. Here are some tips:
- Always back up your workbook before running VBA scripts that modify or delete worksheets.
- Use meaningful variable names and comments to make your code understandable.
- Test your code in a non-production environment first.
- Consider using error handling to manage unexpected situations, such as a worksheet not being found.
Common Errors and Troubleshooting
When working with VBA to delete worksheets, you might encounter several common errors, such as:
- Runtime Error 1004: This error can occur if the worksheet you're trying to delete does not exist or if you're trying to delete a worksheet that is the only one in the workbook.
- Permission Errors: Sometimes, macros might be disabled, or the workbook might be open in a mode that prevents VBA from executing properly.
To troubleshoot these issues, ensure that your workbook is not protected, macros are enabled, and you have the necessary permissions to modify the workbook.
Conclusion and Next Steps
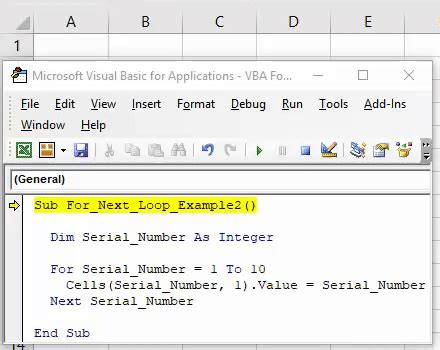
Deleting worksheets with Excel VBA is a powerful technique for automating tasks and managing large workbooks efficiently. By mastering this skill, you can significantly enhance your productivity and open up new possibilities for what you can achieve with Excel. Whether you're looking to automate simple tasks or create complex applications, understanding how to work with worksheets in VBA is a fundamental step.
For those looking to delve deeper into Excel VBA, exploring topics such as user form creation, data manipulation, and advanced error handling can provide a comprehensive understanding of what VBA can offer. Remember, practice is key, so start by applying these techniques to your everyday tasks and gradually move on to more complex projects.
Excel VBA Gallery
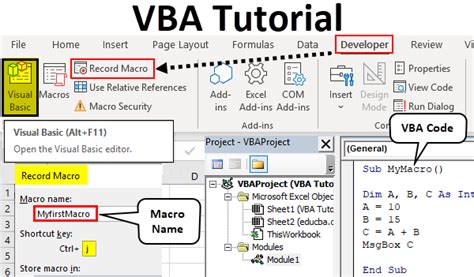
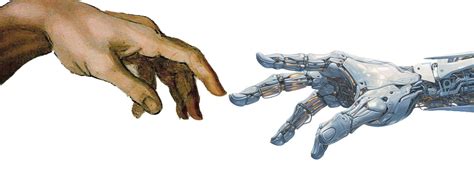
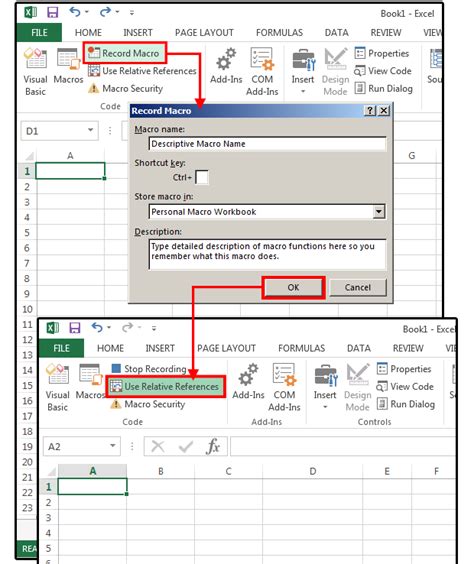
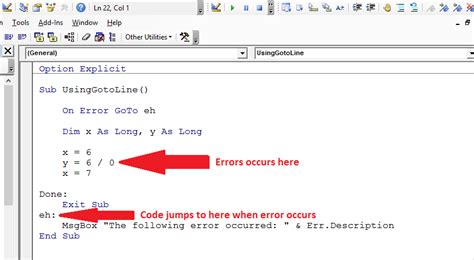
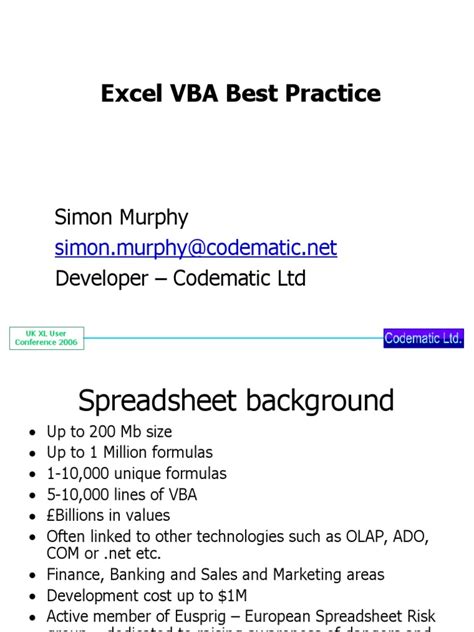
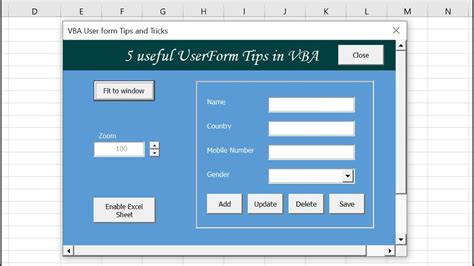
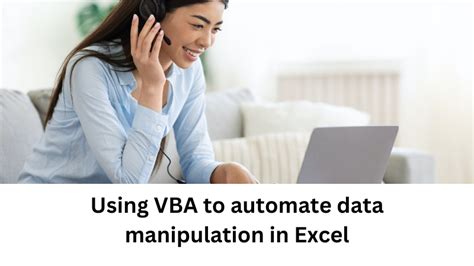

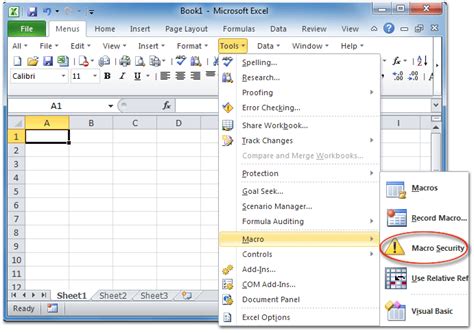
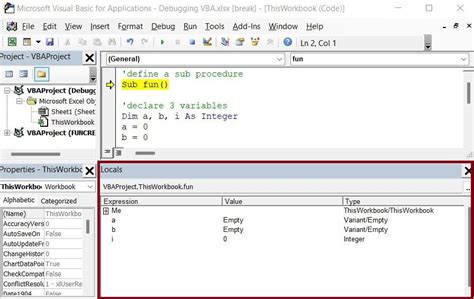
What is Excel VBA used for?
+Excel VBA is used for automating tasks, creating custom applications, and enhancing productivity within Excel.
How do I enable macros in Excel?
+To enable macros, go to the "Developer" tab in Excel, click on "Macro Security," and set the macro settings to "Enable all macros" or "Disable all macros except digitally signed macros."
Can I use VBA to delete multiple worksheets at once?
+Yes, you can use VBA to delete multiple worksheets by looping through the worksheets collection and applying the deletion condition to each worksheet.
How do I troubleshoot VBA errors in Excel?
+To troubleshoot VBA errors, use the Visual Basic Editor to step through your code line by line, check for syntax errors, and ensure that your variables are properly declared and initialized.
Is VBA compatible with all versions of Excel?
+VBA is compatible with most versions of Excel, but compatibility might vary depending on the specific version and the complexity of the VBA code.
We hope this comprehensive guide to deleting worksheets with Excel VBA has been informative and helpful. Whether you're a beginner or an advanced user, mastering VBA techniques can significantly enhance your productivity and open up new possibilities for what you can achieve with Excel. Feel free to share your thoughts, ask questions, or provide feedback in the comments below.