Intro
Discover how to find the last row in VBA using Excel macros, worksheets, and ranges, with tips on dynamic data and cell referencing.
Finding the last row in a spreadsheet is a common task when working with Excel VBA. This is often necessary to determine the range of data that needs to be processed or to dynamically adjust the range for operations like formatting, calculations, or data manipulation. The method to find the last row can vary depending on the specific requirements of your task, such as whether you're working with a specific column or the entire worksheet. Here's how you can find the last row in VBA:
Finding the Last Row in a Specific Column
If your data is primarily contained within a specific column, you can find the last row by looking for the last cell with data in that column. The following example finds the last row with data in column A:
Sub FindLastRowInColumn()
Dim lastRow As Long
lastRow = Cells(Rows.Count, "A").End(xlUp).Row
MsgBox "The last row with data in column A is: " & lastRow
End Sub
In this code:
Cells(Rows.Count, "A")
refers to the cell in the last possible row (based on Excel's row limit) in column A..End(xlUp)
moves up from that cell until it finds a cell with data, effectively finding the last row with data in column A..Row
returns the row number of that cell.
Finding the Last Row in the Entire Worksheet
If your data can be anywhere in the worksheet, you might want to find the last row considering all columns. This can be a bit trickier because Excel's UsedRange
property, which might seem like a straightforward solution, can sometimes return incorrect results due to formatting or other non-data changes in the worksheet. However, for many cases, using UsedRange
can be sufficient:
Sub FindLastRowInWorksheet()
Dim lastRow As Long
lastRow = ActiveSheet.UsedRange.Rows.Count + ActiveSheet.UsedRange.Row - 1
MsgBox "The last row in the worksheet is: " & lastRow
End Sub
Or, more accurately but also more complex, you can loop through columns to find the last row with data in any column:
Sub FindLastRowInAnyColumn()
Dim lastRow As Long
Dim i As Integer
lastRow = 1
For i = 1 To ActiveSheet.Columns.Count
If Cells(Rows.Count, i).End(xlUp).Row > lastRow Then
lastRow = Cells(Rows.Count, i).End(xlUp).Row
End If
Next i
MsgBox "The last row in any column is: " & lastRow
End Sub
Best Practices
- Specify worksheets: Instead of using
ActiveSheet
, it's better to specify the worksheet you're working with, e.g.,Worksheets("YourSheetName")
. - Error handling: Consider adding error handling, especially if your code might run on worksheets with no data or in unexpected states.
- Performance: For very large datasets, looping through all columns can be slow. Consider the structure of your data and whether there are more efficient methods to achieve your goals.
Gallery of Finding Last Row Methods
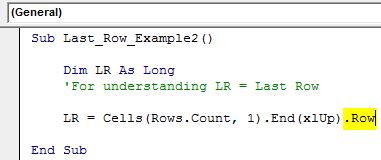
FAQs
What is the fastest way to find the last row in Excel VBA?
+Using `Cells(Rows.Count, "A").End(xlUp).Row` for a specific column is generally fast. For the entire worksheet, `UsedRange` can be quick but might not always be accurate.
How do I find the last row with data in any column?
+You can loop through all columns and check the last row with data in each, keeping track of the maximum row found.
Why might `UsedRange` not always give accurate results?
+`UsedRange` can include cells that have been formatted or contain formulas that don't display data, leading to incorrect last row detection.
Finding the last row in Excel VBA is a foundational skill for automating tasks and interacting with worksheet data. By understanding the different methods available and their strengths, you can write more effective and efficient VBA code. Whether you're processing data in a specific column or across the entire worksheet, being able to accurately determine the last row with data is crucial for ensuring your macros work as intended.